Python Functions: A Comprehensive Guide

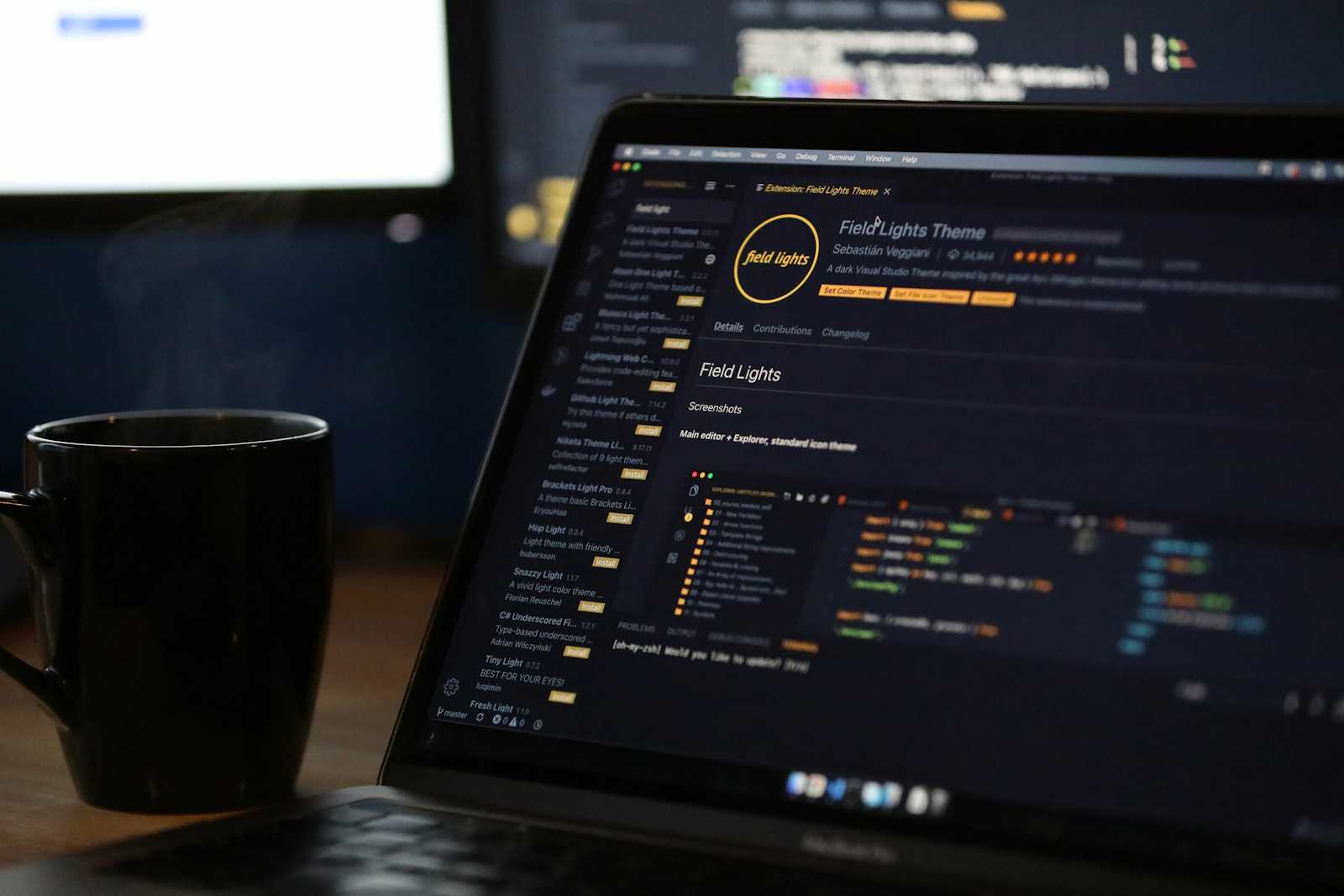
Introduction
In Python, a function is a reusable block of code that performs a specific task. Functions help break our program into smaller and modular chunks, making it more organized, manageable, and reusable. Python functions can accept inputs (parameters), perform computations, and return outputs (results).
Table of Contents
What is a Function?
A function is a block of organized, reusable code used to perform a single, related action. Functions provide better modularity and code reusability.
Key Concepts:
Function Definition: The block of code that performs a task.
Function Call: Executing the function to perform its task.
Parameters: Variables listed in the function definition.
Arguments: Values passed to the function when it is called.
Defining Functions
In Python, functions are defined using the def
keyword followed by the function name and parentheses ()
.
Syntax:
def function_name(parameters):
"""Docstring explaining the function."""
# Function body
return result
Example:
def greet(name):
"""Function to greet a person."""
return f"Hello, {name}!"
# Function call
message = greet("Alice")
print(message) # Output: Hello, Alice!
Types of Functions
Built-in Functions
Python provides a rich set of built-in functions that are always available.
Examples:
print()
: Prints the specified message.len()
: Returns the length of an object.type()
: Returns the type of an object.range()
: Generates a sequence of numbers.
Example Usage:
numbers = [1, 2, 3, 4, 5]
print(len(numbers)) # Output: 5
print(type(numbers)) # Output: <class 'list'>
User-defined Functions
Functions that users create to perform specific tasks.
Example:
def add_numbers(a, b):
"""Returns the sum of two numbers."""
return a + b
result = add_numbers(5, 3)
print(result) # Output: 8
Anonymous Functions (Lambda Functions)
Functions defined without a name using the lambda
keyword. They are often used for short, simple functions.
Syntax:
lambda arguments: expression
Example:
# Traditional function
def square(x):
return x * x
# Lambda function
square_lambda = lambda x: x * x
print(square(4)) # Output: 16
print(square_lambda(4)) # Output: 16
Use Case with map()
:
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda x: x * x, numbers))
print(squares) # Output: [1, 4, 9, 16, 25]
Recursive Functions
Functions that call themselves to solve a problem by breaking it down into smaller, more manageable problems.
Example: Calculating Factorial
def factorial(n):
"""Returns the factorial of a number."""
if n == 1:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
Higher-order Functions
Functions that take other functions as arguments or return functions as their result.
Examples:
Taking a Function as an Argument:
def apply_function(func, value): return func(value) def double(x): return x * 2 result = apply_function(double, 5) print(result) # Output: 10
Returning a Function:
def make_multiplier(n): def multiplier(x): return x * n return multiplier times3 = make_multiplier(3) print(times3(10)) # Output: 30
Generator Functions
Functions that return an iterator that yields a sequence of values using the yield
statement.
Example:
def fibonacci(n):
"""Generator function for Fibonacci series up to n."""
a, b = 0, 1
while a < n:
yield a
a, b = b, a + b
fib = fibonacci(10)
for num in fib:
print(num, end=" ") # Output: 0 1 1 2 3 5 8
Function Arguments
Positional Arguments
Arguments passed to a function in the correct positional order.
Example:
def power(base, exponent):
return base ** exponent
print(power(2, 3)) # Output: 8
Keyword Arguments
Arguments passed to a function by explicitly stating the parameter name.
Example:
def power(base, exponent):
return base ** exponent
print(power(exponent=3, base=2)) # Output: 8
Default Arguments
Parameters that assume a default value if no argument is provided.
Example:
def greet(name, message="Hello"):
return f"{message}, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
print(greet("Bob", "Good morning")) # Output: Good morning, Bob!
Variable-length Arguments (*args and **kwargs)
Functions can accept a variable number of arguments.
*args
: Non-keyworded variable-length argument list.**kwargs
: Keyworded variable-length argument list.
Understanding *args and **kwargs
Using *args
Purpose: To pass a variable number of non-keyworded arguments to a function.
*args
collects extra positional arguments as a tuple.
Example:
def sum_all(*args):
"""Returns the sum of all arguments."""
total = 0
for num in args:
total += num
return total
print(sum_all(1, 2, 3)) # Output: 6
print(sum_all(4, 5, 6, 7, 8)) # Output: 30
Explanation:
The function
sum_all
can accept any number of arguments.args
is a tuple of the positional arguments.
Using **kwargs
Purpose: To pass a variable number of keyworded arguments to a function.
**kwargs
collects extra keyword arguments as a dictionary.
Example:
def print_info(**kwargs):
"""Prints key-value pairs passed as keyword arguments."""
for key, value in kwargs.items():
print(f"{key}: {value}")
print_info(name="Alice", age=30, city="New York")
# Output:
# name: Alice
# age: 30
# city: New York
Explanation:
The function
print_info
accepts any number of keyword arguments.kwargs
is a dictionary of the keyword arguments.
Combining *args and **kwargs
You can use both *args
and **kwargs
in the same function.
Order of Parameters:
Formal positional arguments.
*args
Keyword-only arguments
**kwargs
Example:
def func_example(a, b, *args, **kwargs):
print(f"a: {a}")
print(f"b: {b}")
print(f"args: {args}")
print(f"kwargs: {kwargs}")
func_example(1, 2, 3, 4, 5, x=6, y=7)
# Output:
# a: 1
# b: 2
# args: (3, 4, 5)
# kwargs: {'x': 6, 'y': 7}
Examples and Use Cases
Example 1: Function with Default and Variable Arguments
def make_pizza(size, *toppings):
"""Summarize the pizza we are about to make."""
print(f"\nMaking a {size}-inch pizza with the following toppings:")
for topping in toppings:
print(f"- {topping}")
make_pizza(12, 'pepperoni')
make_pizza(16, 'mushrooms', 'green peppers', 'extra cheese')
Output:
Making a 12-inch pizza with the following toppings:
- pepperoni
Making a 16-inch pizza with the following toppings:
- mushrooms
- green peppers
- extra cheese
Example 2: Function with **kwargs to Build a Dictionary
def build_profile(first, last, **user_info):
"""Build a dictionary containing everything we know about a user."""
profile = {'first_name': first, 'last_name': last}
profile.update(user_info)
return profile
user_profile = build_profile('albert', 'einstein', location='princeton', field='physics')
print(user_profile)
Output:
{
'first_name': 'albert',
'last_name': 'einstein',
'location': 'princeton',
'field': 'physics'
}
Example 3: Unpacking Arguments
- Unpacking with
*
and**
when calling functions.
Example with Lists and Tuples:
def multiply(a, b, c):
return a * b * c
numbers = [2, 3, 4]
print(multiply(*numbers)) # Output: 24
Example with Dictionaries:
def introduce(name, age):
print(f"My name is {name} and I'm {age} years old.")
person = {'name': 'Alice', 'age': 30}
introduce(**person) # Output: My name is Alice and I'm 30 years old.
Quick Revision Notes
Functions: Reusable blocks of code that perform specific tasks.
Defining Functions: Use
def
keyword followed by function name and parameters.Types of Functions:
Built-in Functions: Provided by Python (e.g.,
print()
,len()
).User-defined Functions: Created by users.
Anonymous Functions: Created using
lambda
.Recursive Functions: Functions that call themselves.
Higher-order Functions: Functions that take or return other functions.
Generator Functions: Use
yield
to return a sequence of values.
Function Arguments:
Positional Arguments: Based on the order of parameters.
Keyword Arguments: Specified by parameter names.
Default Arguments: Parameters with default values.
Variable-length Arguments: Use
*args
and**kwargs
to accept varying numbers of arguments.
*args
:Collects extra positional arguments into a tuple.
Use when you want to pass a variable number of positional arguments.
**kwargs
:Collects extra keyword arguments into a dictionary.
Use when you want to pass a variable number of keyword arguments.
Order of Parameters in Function Definition:
Regular positional parameters.
*args
(non-keyworded variable-length arguments).Keyword-only parameters.
**kwargs
(keyworded variable-length arguments).
Unpacking Arguments:
Use
*
to unpack sequences (lists, tuples) into positional arguments.Use
**
to unpack dictionaries into keyword arguments.
Conclusion
Understanding functions and their types is fundamental to programming in Python. Functions allow for modular, reusable, and organized code. By mastering function arguments, including *args
and **kwargs
, you can write flexible functions that handle varying numbers of inputs, making your code more adaptable and efficient.
Subscribe to my newsletter
Read articles from Sai Prasanna Maharana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
