JavaScript Unleashed: Your First Step into Coding!
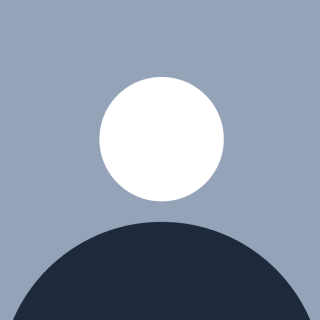
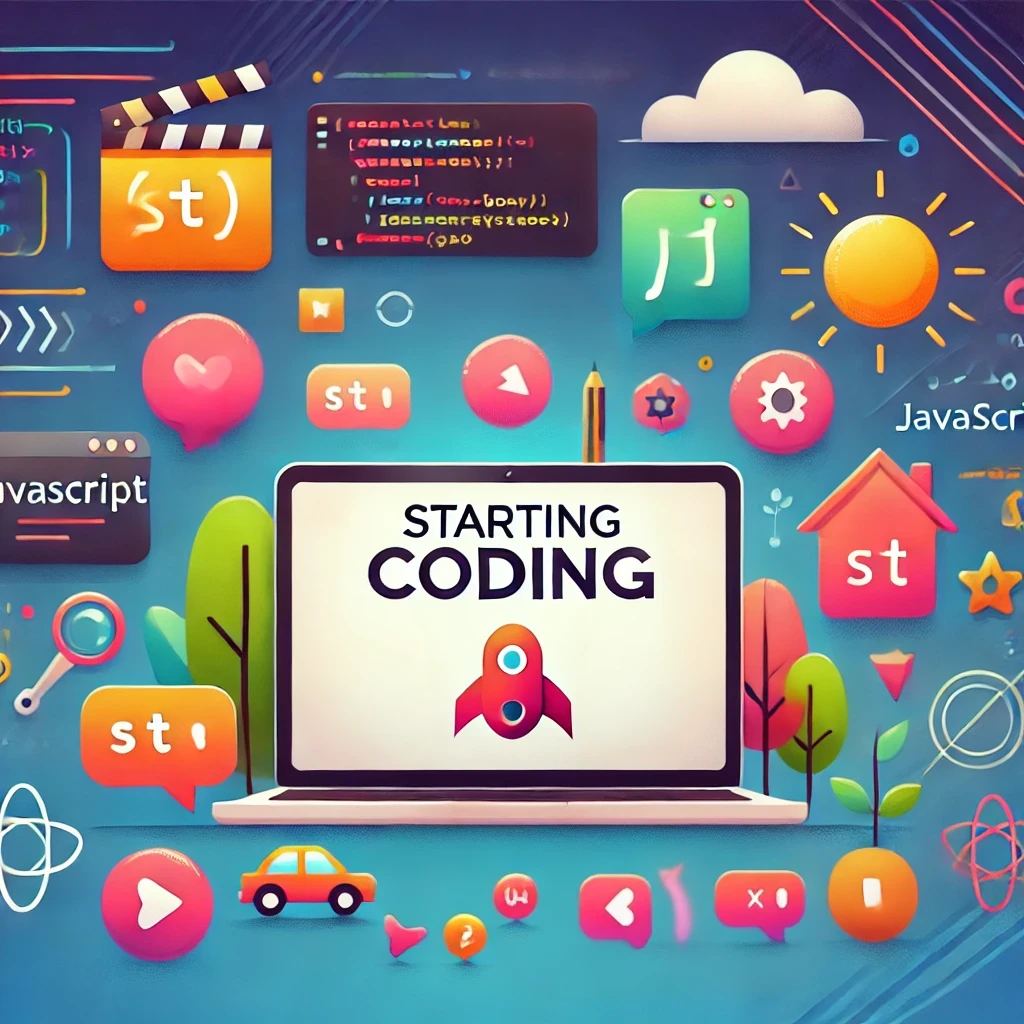
Introduction to JavaScript
JavaScript is a powerful programming language that plays a crucial role in web development, enabling developers to create interactive and dynamic web applications. From validating forms to creating real-time applications, JavaScript is the tool that makes websites come alive!
As you start your journey to learn JavaScript, envision it as a superpower that allows you to control the web. With this power, you can create anything from simple scripts to complex applications. Let's dive into the basics!
Simple JavaScript Syntax: Writing Your First Code
"Hello, World!"
The best way to start learning a new programming language is to write your first line of code. In JavaScript, a classic starting point is displaying "Hello, World!" on the console.
console.log("Hello, World!");
console.log()
is a built-in function that outputs the specified message to the console, making it essential for debugging and checking variable values during development.The message inside the parentheses,
"Hello, World!"
, is a string, which is a sequence of characters and a fundamental data type in JavaScript.
Flow of a Basic Program
To visualize the flow of our simple program, consider the following flowchart:
[ Start ]
|
V
[ Execute console.log("Hello, World!") ]
|
V
[ Display "Hello, World!" in Console ]
|
V
[ End ]
This flowchart illustrates that when the program starts, it executes the command to display "Hello, World!" and then ends.
Identifiers, Expressions, and Variables
Now that we have written our first line of code, let's explore how to store information in JavaScript using variables.
Declaring Variables
In JavaScript, you can declare a variable using the keywords var
, let
, or const
. Here’s how you can do it:
let superheroName = "Iron Man"; // Using let
const superheroPower = "Flight"; // Using const
Explanation of var
, let
, and const
:
var
:Scope: Function-scoped or globally-scoped. If declared inside a function, it can only be accessed within that function.
Hoisting:
var
declarations are hoisted to the top of their containing scope, meaning they can be referenced before they are declared (though they will beundefined
until the declaration).
console.log(hero); // Output: undefined
var hero = "Hulk";
console.log(hero); // Output: Hulk
let
:Scope: Block-scoped. This means it can only be accessed within the block it is defined in (e.g., within loops or conditionals).
No Hoisting:
let
is not accessible before its declaration in the block.
if (true) {
let hero = "Spider-Man";
console.log(hero); // Output: Spider-Man
}
console.log(hero); // ReferenceError: hero is not defined
const
:Scope: Block-scoped, similar to
let
.Immutability: Variables declared with
const
cannot be reassigned. However, if it's an object or array, the contents can be modified.
const sidekick = "Robin";
// sidekick = "Batgirl"; // TypeError: Assignment to constant variable.
const sidekickPowers = ["Agility", "Intelligence"];
sidekickPowers.push("Combat Skills"); // Allowed
console.log(sidekickPowers); // Output: ["Agility", "Intelligence", "Combat Skills"]
Superheroes
Think of variables as superheroes. Each superhero has a unique name and specific powers. Just like a superhero can change their outfit but retains their identity, a variable can change its value while keeping its name.
Superhero Name (Variable Name): Represents the identity of the superhero.
Superhero Power (Variable Value): Represents the ability or data that the superhero possesses.
Data Types
Understanding data types is crucial for working with variables in JavaScript. There are two categories of data types:
Primitive Data Types: These include:
String: Textual data (e.g.,
"Iron Man"
)Number: Numeric data (e.g.,
42
,3.14
)Boolean: Represents
true
orfalse
Null: Represents an intentional absence of any object value.
Undefined: A variable that has been declared but not assigned a value.
Reference Data Types: These include:
Objects: Collections of key-value pairs.
Arrays: Ordered lists of values.
Operators in JavaScript
JavaScript uses various operators to perform operations on variables and values.
Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations.
Addition (
+
)Subtraction (
-
)Multiplication (
*
)Division (
/
)Modulus (
%
): Returns the remainder of a division operation.
let a = 10;
let b = 5;
console.log(a + b); // Output: 15 (Addition)
console.log(a - b); // Output: 5 (Subtraction)
console.log(a * b); // Output: 50 (Multiplication)
console.log(a / b); // Output: 2 (Division)
console.log(a % b); // Output: 0 (Modulus)
Increment and Decrement Operators
These operators are used to increase or decrease a variable's value by one.
Increment (
++
): Increases the value by one.Decrement (
--
): Decreases the value by one.
let count = 5;
count++; // Increment
console.log(count); // Output: 6
count--; // Decrement
console.log(count); // Output: 5
Logical Operators
Logical operators are used to combine multiple boolean expressions.
AND (
&&
): Returns true if both operands are true.OR (
||
): Returns true if at least one operand is true.NOT (
!
): Reverses the logical state of its operand.
let isHero = true;
let isVillain = false;
console.log(isHero && isVillain); // Output: false (AND)
console.log(isHero || isVillain); // Output: true (OR)
console.log(!isHero); // Output: false (NOT)
Comparison Operators
Comparison operators are used to compare two values.
Equal (
==
): Checks if values are equal.Strict Equal (
===
): Checks if values and types are equal.Not Equal (
!=
): Checks if values are not equal.Strict Not Equal (
!==
): Checks if values and types are not equal.Greater Than (
>
): Checks if the left value is greater than the right value.Less Than (
<
): Checks if the left value is less than the right value.Greater Than or Equal To (
>=
): Checks if the left value is greater than or equal to the right value.Less Than or Equal To (
<=
): Checks if the left value is less than or equal to the right value.
console.log(5 == '5'); // Output: true (equal)
console.log(5 === '5'); // Output: false (strict equal)
console.log(5 != '5'); // Output: false (not equal)
console.log(5 !== '5'); // Output: true (strict not equal)
console.log(5 > 3); // Output: true (greater than)
console.log(5 < 3); // Output: false (less than)
Boolean Values
Boolean values can only be true
or false
. They are often the result of comparison and logical operations. Understanding booleans is essential for control flow in programming.
Challenge: Create Your Own Superhero Variable
Now it’s your turn! Create your own superhero variable by following these steps:
Choose a superhero name and their special power.
Declare two variables using
let
andconst
.Use arithmetic operators to display a message about your superhero.
Example:
let myHeroName = "Captain Marvel"; // Using let
const myHeroPower = "Super Strength"; // Using const
// Using an arithmetic operation to represent power level
let powerLevel = 100;
powerLevel += 50; // Increase power level
console.log("My superhero is " + myHeroName + " with the power of " + myHeroPower + ". Power Level: " + powerLevel);
Building the Foundation for Coding
In this section, we introduced the fundamentals of JavaScript, including syntax, variables, and the concept of identifiers. We also explored the different types of variables (var
, let
, and const
), data types, and various operators that will be crucial as you progress further in your JavaScript journey. Understanding these basics is essential for creating more complex applications and functions.
With these foundational skills, you are now equipped to tackle more advanced topics and create dynamic applications.
Next, we’ll tackle Data Types!
In the next article, we will delve deeper into the fascinating world of data types in JavaScript. Understanding the various data types is crucial for effective programming, as they dictate how you can manipulate and interact with data. We’ll explore primitive and reference types, their unique characteristics, and how to use them effectively in your code. Get ready to enhance your knowledge and become proficient in managing data in your JavaScript applications!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by