Mastering Multi-Container Magic: Docker Compose Tips Every DevOps Engineer Should Know

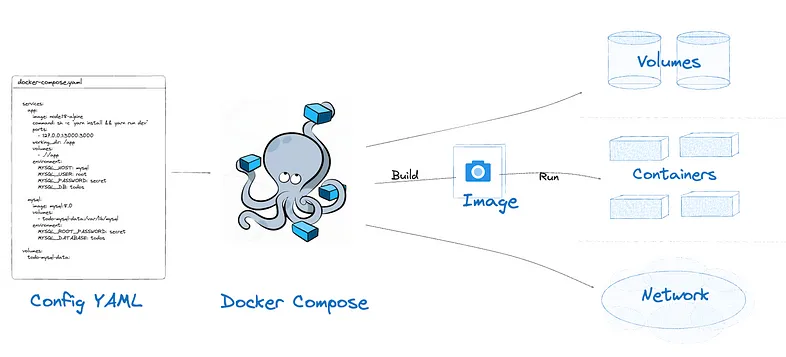
Alright, DevOps engineers! You’ve likely heard about Docker Compose and Dockerfiles a thousand times, but maybe you're still a bit puzzled about what each one does and when to use them. Don’t worry—you’re not alone. Let’s dive deep into Docker Compose, the tool for managing multi-container applications, and clear up the confusion between Dockerfiles and Docker Compose.
First, What’s the Difference Between Dockerfile and Docker Compose?
Here’s the golden rule:
A Dockerfile is like a recipe. It defines how to build a single container. It’s all about building the image.
Docker Compose is like a full-course meal. It’s a tool for managing multiple containers, setting them up, and linking them so they work together as a complete system.
If you need a single container, a Dockerfile will do. But for applications with multiple services, Docker Compose is your friend.
Imagine you’re building a web app. You might have:
A web server container,
A database container, and
A cache container.
With Docker Compose, you can spin up all these containers in one go. Neat, right?
Setting Up Your Environment with Docker Compose
Let’s dive into how Docker Compose makes life easier. Suppose you’re working on a project that needs three services: an app service, a database service, and a Redis cache. Let’s say the app is a basic Python Flask application.
Step 1: Write Dockerfiles for Each Service
For each service, you’ll need a Dockerfile. Each Dockerfile is like a custom blueprint for a container. Let’s start with the one for the Flask app.
Create a Dockerfile in your app’s directory:
FROM python:3.8
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
This Dockerfile:
Starts from the Python 3.8 image,
Sets the working directory to /app,
Copies the app files, installs dependencies, and
Runs app.py.
Step 2: Write Your Docker Compose File
Now we’re ready for the docker-compose.yml file, which defines the whole system. It’s like saying, “Hey, Docker, spin up all these containers at once and make them talk to each other.”
Here’s what your docker-compose.yml might look like:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
depends_on:
- db
- redis
db:
image: postgres:13
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
redis:
image: redis:alpine
Let’s break down each part:
web: This service is built from the current directory (where your Dockerfile lives). It also exposes port 5000 on the host machine.
db: This service uses a ready-made Postgres image and defines environment variables for the username and password.
redis: This service is based on a lightweight Redis image.
In this setup, Docker Compose knows that the web service depends on db and redis, so it ensures they’re up and running first.
Step 3: Start Everything with a Single Command
Now for the fun part! With Docker Compose, you can spin up all three containers with just one command:
docker-compose up
This will:
Build the images if they aren’t already built,
Spin up the containers, and
Link them together so they can communicate.
Want to tear it down? Just run:
docker-compose down
And everything stops. No more manual stopping of each container.
Best Practices for Docker Compose
Use .env Files
To keep secrets like passwords out of your compose file, put them in a .env file and refer to them in your docker-compose.yml. For instance:POSTGRES_USER=user
POSTGRES_PASSWORD=password
Then, reference them in your docker-compose.yml file:
environment:
POSTGRES_USER: ${POSTGRES_USER}
POSTGRES_PASSWORD: ${POSTGRES_PASSWORD}Avoid Hardcoding Values
Use variables whenever possible, especially for things like ports, paths, and environment-specific settings.Take Advantage of ‘depends_on’
If your web service needs the database and cache to be running first, use depends_on. But remember, it only controls the startup order, not the readiness of each container.Scale Services Easily
Imagine your app’s getting popular, and you need more instances of the web server. You can scale with one simple command:docker-compose up --scale web=3
Now you have three instances of the web service running!
Clean Up with Volumes
Use Docker volumes to persist data for services like databases. Otherwise, your data is gone every time you bring down the containers.
Final Thoughts
Docker Compose is a game-changer for managing multi-container applications. While Dockerfile is essential for defining each container’s image, Docker Compose brings everything together. It simplifies setting up, managing, and scaling your application.
So, next time you’re starting a multi-service project, remember: a Dockerfile builds your containers, and Docker Compose runs them together. With these tools in your belt, you’re ready to tackle any project that comes your way! Happy Dockerizing!
Subscribe to my newsletter
Read articles from Chetan Mohanrao Mohod directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chetan Mohanrao Mohod
Chetan Mohanrao Mohod
DevOps Engineer focused on automating workflows, optimizing infrastructure, and building scalable efficient solutions.