ViewComponent vs Phlex vs Partials: A Comprehensive Comparison in Ruby on Rails

Table of contents
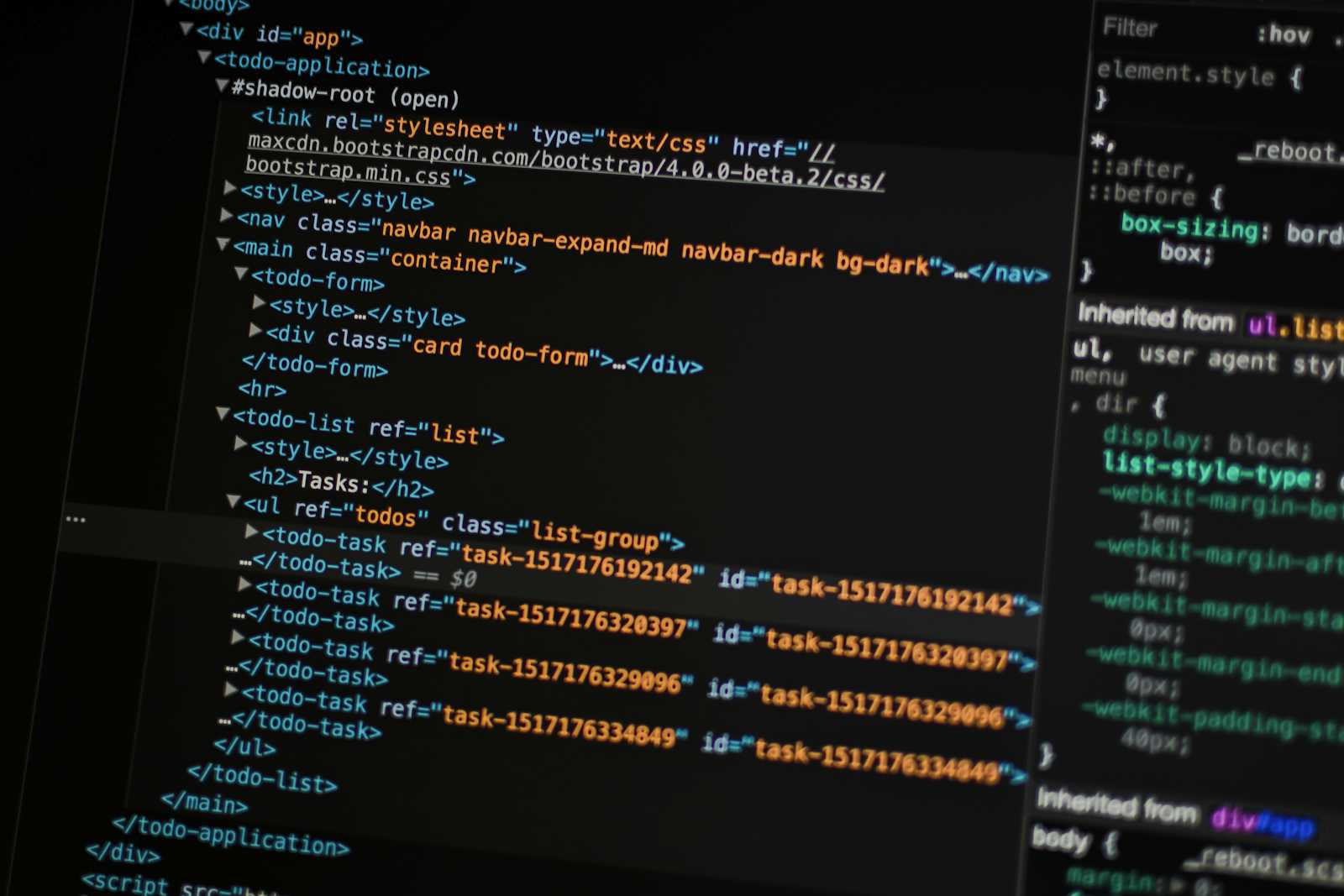
In the world of Ruby on Rails, developers are often faced with the task of managing the presentation layer of their applications.
This is where the choice of view management strategy becomes crucial. Three popular approaches are ViewComponent, Phlex, and traditional partials.
Each of these methods offers unique advantages and trade-offs, and the decision to use one over the others depends on the specific requirements of the project.
In this article, we will dive deep into the world of ViewComponent, Phlex, and partials, comparing and contrasting their features, use cases, and implementation details.
By the end, you'll have a comprehensive understanding of the strengths and weaknesses of each approach, allowing you to make an informed decision on the best fit for your Ruby on Rails application.
ViewComponent
ViewComponent is a Ruby on Rails library that provides a component-based approach to building user interfaces. It was developed by GitHub and has gained popularity in the Ruby on Rails community due to its flexibility and scalability.
What is ViewComponent?
ViewComponent is a way of encapsulating the presentation logic of your application into reusable, testable, and composable components. These components are represented as Ruby classes that inherit from ViewComponent::Base
and are responsible for rendering the corresponding HTML. This approach allows developers to keep their view logic organized, maintainable, and testable.
Here's a simple example of a ViewComponent:
# app/components/card_component.rb
class CardComponent < ViewComponent::Base
def initialize(title:, content:)
@title = title
@content = content
end
def render?
@title.present? && @content.present?
end
end
In this example, the CardComponent
is responsible for rendering a card with a title and content. The render?
method is used to conditionally render the component based on the presence of the title
and content
attributes.
To use this component in your views, you can simply call it like this:
<!-- app/views/pages/home.html.erb -->
<%= render(CardComponent.new(title: "Welcome", content: "This is a card.")) %>
Benefits of ViewComponent
Separation of Concerns: ViewComponent helps to separate the presentation logic from the rest of your application, making your codebase more organized and maintainable.
Testability: ViewComponent components are regular Ruby classes, which means they can be easily tested in isolation using standard testing frameworks like RSpec or Minitest.
Reusability: ViewComponent components can be reused across your application, reducing duplication and promoting consistency in your user interface.
Flexibility: ViewComponent allows you to pass dynamic data to your components, making them more versatile and adaptable to different use cases.
Debugging: ViewComponent's clear separation of concerns and testability make it easier to debug issues related to the presentation layer of your application.
Limitations of ViewComponent
Learning Curve: ViewComponent introduces a new way of thinking about view management in Ruby on Rails, which may require some time for developers to fully understand and adopt.
Boilerplate: Creating a new ViewComponent can involve more boilerplate code compared to traditional partials, as each component is a separate Ruby class.
Performance: While ViewComponent aims to be performant, there may be cases where the overhead of creating and rendering a component can impact the overall performance of your application.
Integration with Existing Codebase: Adopting ViewComponent in an existing Ruby on Rails application may require significant refactoring of the existing view layer, which can be time-consuming and disruptive.
Perspective from Basecamp
It's worth noting that the team at Basecamp have shared their perspective on ViewComponents. In a July 2023 post on the Ruby on Rails discussion forum, David Heinemeier Hansson (DHH), a core member of the Ruby on Rails team, stated:
We do not use ViewComponents at 37signals, and have no plans to do so. Glad to see that they're working for some people, but in my opinion, you're usually better off with regular partial templates the majority of the time.
Hansson went on to explain that Basecamp generally prefers to use presenter objects to encapsulate domain logic in their views, rather than calling #render
directly from these objects. This provides an alternative perspective on view management approaches in Ruby on Rails.
Phlex
Phlex is another library for building component-based user interfaces in Ruby on Rails. It is designed to be a lightweight alternative to ViewComponent.
What is Phlex?
Phlex is a Ruby library that allows you to define your view components as Ruby classes, similar to ViewComponent. However, Phlex takes a more minimal approach, focusing on simplicity and ease of use.
Here's an example of a Phlex component:
# app/components/card_component.rb
class CardComponent < Phlex::Component
def initialize(title:, content:)
@title = title
@content = content
end
def template
div class: "card" do
h2 class: "card-title", @title
p class: "card-content", @content
end
end
end
In this example, the CardComponent
is defined using the Phlex DSL, which allows you to declaratively define the HTML structure of the component.
To use this component in your views, you can call it like this:
<!-- app/views/pages/home.html.erb -->
<%= render(CardComponent.new(title: "Welcome", content: "This is a card.")) %>
Benefits of Phlex
Simplicity: Phlex takes a more minimalist approach compared to ViewComponent, with a simpler API and less boilerplate code.
Ease of Use: Phlex's DSL-based approach to defining components can be more intuitive and easier to learn for some developers.
Performance: Phlex aims to be more performant than ViewComponent, as it uses a more lightweight approach to rendering components.
Flexibility: Phlex allows you to customize the rendering process of your components, giving you more control over the output.
Limitations of Phlex
Lack of Maturity: Phlex is a newer library compared to ViewComponent, which means it may have fewer features, plugins, and community support.
Limited Tooling: Phlex may not have the same level of tooling and integration with other Ruby on Rails libraries as ViewComponent.
Testability: While Phlex components can be tested, the testing story may not be as well-developed as with ViewComponent.
Integration with Existing Codebase: Adopting Phlex in an existing Ruby on Rails application may require significant refactoring, similar to the challenges with ViewComponent.
Partials
Partials (ERB, etc.) are a traditional way of managing the presentation layer in Ruby on Rails applications. They are essentially small, reusable HTML templates that can be included in other views.
What are Partials?
Partials are Ruby on Rails' built-in mechanism for organizing and reusing view code. They are defined in the app/views/
directory and can be included in other views using the render
helper.
Here's an example of a partial:
<!-- app/views/shared/_card.html.erb -->
<div class="card">
<h2 class="card-title"><%= title %></h2>
<p class="card-content"><%= content %></p>
</div>
To use this partial in a view, you can call it like this:
<!-- app/views/pages/home.html.erb -->
<%= render "shared/card", title: "Welcome", content: "This is a card." %>
Benefits of Partials
Simplicity: Partials are a well-established and straightforward way of managing view code in Ruby on Rails, which can make them easier to understand and adopt for some developers.
Familiarity: Partials are a built-in feature of Ruby on Rails, which means they have a long history of use and widespread adoption in the Ruby on Rails community.
Integration: Partials seamlessly integrate with the rest of the Ruby on Rails ecosystem, including features like layout inheritance and view helpers.
Limitations of Partials
Lack of Encapsulation: Partials do not provide the same level of encapsulation and separation of concerns as ViewComponent or Phlex, as the presentation logic is still closely tied to the rest of the application.
Testability: It can be more difficult to test partials in isolation compared to ViewComponent or Phlex components, as they are more tightly coupled to the rest of the application.
Reusability: While partials can be reused across views, they may not be as easily composable or adaptable to different use cases as ViewComponent or Phlex components.
Debugging: Debugging issues related to the presentation layer can be more challenging with partials, as the separation of concerns is not as clear as with ViewComponent or Phlex.
Comparison and Use Cases
Now that we've explored the individual characteristics of ViewComponent, Phlex, and partials, let's compare them and discuss their respective use cases.
Separation of Concerns
ViewComponent and Phlex both provide a more robust separation of concerns between the presentation logic and the rest of the application, as they encapsulate the view code into reusable components.
This can lead to a more organized and maintainable codebase, especially as the application grows in complexity.
Partials, on the other hand, are more tightly coupled to the rest of the application, as the presentation logic is still mixed with the rest of the view code.
Testability
ViewComponent and Phlex components can be more easily tested in isolation, as they are represented as regular Ruby classes. This can make it easier to write comprehensive, unit-level tests for the presentation layer of your application.
Partials, being more tightly coupled to the rest of the application, can be more challenging to test in isolation, as they often depend on other view-related code and helpers.
Reusability and Composability
ViewComponent and Phlex components are designed to be highly reusable and composable, as they encapsulate a specific piece of UI functionality. This can make it easier to build complex user interfaces by combining smaller, modular components.
Partials can also be reused across views, but they may not be as easily composable as ViewComponent or Phlex components.
Performance
Phlex aims to be more performant than ViewComponent, as it uses a more lightweight approach to rendering components. However, the performance difference may not be significant in many real-world applications.
Partials, being a built-in feature of Ruby on Rails, are generally well-optimized and can provide good performance in most cases.
Learning Curve and Adoption
Partials are a long-established and well-understood feature of Ruby on Rails, which can make them easier to learn and adopt, especially for developers who are new to the framework.
ViewComponent and Phlex, being third-party libraries, may have a steeper learning curve, as they introduce a new way of thinking about view management in Ruby on Rails. However, the benefits they provide may outweigh the initial investment for more complex applications.
Code Example Comparison
Let's consider a simple use case where we need to display a list of products with their name, price, and an "Add to Cart" button.
We'll implement this using each of the three approaches: ViewComponent, Phlex, and Partials.
ViewComponent
# app/components/product_list_component.rb
class ProductListComponent < ViewComponent::Base
def initialize(products:)
@products = products
end
def render
content_tag :ul, class: "product-list" do
@products.map do |product|
content_tag :li, class: "product-item" do
render(ProductComponent.new(product: product))
end
end.join.html_safe
end
end
end
# app/components/product_component.rb
class ProductComponent < ViewComponent::Base
def initialize(product:)
@product = product
end
def render
content_tag :div, class: "product-card" do
safe_join([
content_tag(:h3, @product.name),
content_tag(:p, number_to_currency(@product.price)),
button_tag("Add to Cart", class: "add-to-cart-btn")
])
end
end
end
Phlex
# app/components/product_list_component.rb
class ProductListComponent < Phlex::Component
def initialize(products:)
@products = products
end
def template
ul class: "product-list" do
@products.each do |product|
li class: "product-item" do
render(ProductComponent.new(product: product))
end
end
end
end
end
# app/components/product_component.rb
class ProductComponent < Phlex::Component
def initialize(product:)
@product = product
end
def template
div class: "product-card" do
h3 @product.name
p number_to_currency(@product.price)
button "Add to Cart", class: "add-to-cart-btn"
end
end
end
Partials
<!-- app/views/shared/_product_list.html.erb -->
<ul class="product-list">
<% products.each do |product| %>
<li class="product-item">
<%= render "shared/product_card", product: product %>
</li>
<% end %>
</ul>
<!-- app/views/shared/_product_card.html.erb -->
<div class="product-card">
<h3><%= product.name %></h3>
<p><%= number_to_currency(product.price) %></p>
<button class="add-to-cart-btn">Add to Cart</button>
</div>
In this example, we have a ProductListComponent
that renders a list of products, and a ProductComponent
(or partial) that renders the individual product card.
The key differences are:
ViewComponent and Phlex: The presentation logic is encapsulated in reusable components, making it easier to test and maintain.
Partials: The presentation logic is spread across two partials, which are more tightly coupled to the rest of the application.
Phlex: The Phlex version uses a more declarative, DSL-style syntax for defining the component structure.
Choosing the right approach will depend on the specific requirements of your project, as well as the preferences and expertise of your development team.
Use Cases
ViewComponent: Recommended for large, complex Ruby on Rails applications that require a highly organized and maintainable presentation layer. The additional structure and testability of ViewComponent can be particularly beneficial in these scenarios.
Phlex: Suitable for Ruby on Rails applications that prioritize simplicity and developer productivity, or for projects with specific performance requirements. Phlex's lightweight approach can be a good fit for smaller to medium-sized applications.
Partials: Appropriate for simpler Ruby on Rails applications or for projects where the presentation layer is not a major concern. Partials can be a good choice for quickly building out basic user interfaces or for projects with limited resources.
Conclusion
In the world of Ruby on Rails, developers have several options when it comes to managing the presentation layer of their applications.
ViewComponent, Phlex, and partials each offer unique advantages and trade-offs, and the choice between them will depend on the specific requirements and constraints of your project.
ViewComponent provides a robust, component-based approach to view management, with a focus on separation of concerns, testability, and reusability.
Phlex offers a more lightweight and simplified alternative, prioritizing ease of use and performance.
Partials, being a built-in feature of Ruby on Rails, are a straightforward and familiar option, but may not offer the same level of encapsulation and testability as the other two approaches.
By understanding the strengths and weaknesses of each approach, you can make an informed decision on the best fit for your Ruby on Rails application.
Regardless of the choice, the key is to select the view management strategy that aligns best with the needs of your project and the preferences of your development team.
Subscribe to my newsletter
Read articles from BestWeb Ventures directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

BestWeb Ventures
BestWeb Ventures
From cutting-edge web and app development, using Ruby on Rails, since 2005 to data-driven digital marketing strategies, we build, operate, and scale your MVP (Minimum Viable Product) for sustainable growth in today's competitive landscape.