Boost Your C# 'For Loop' Abilities: Essential Exercises for HNDIT Students

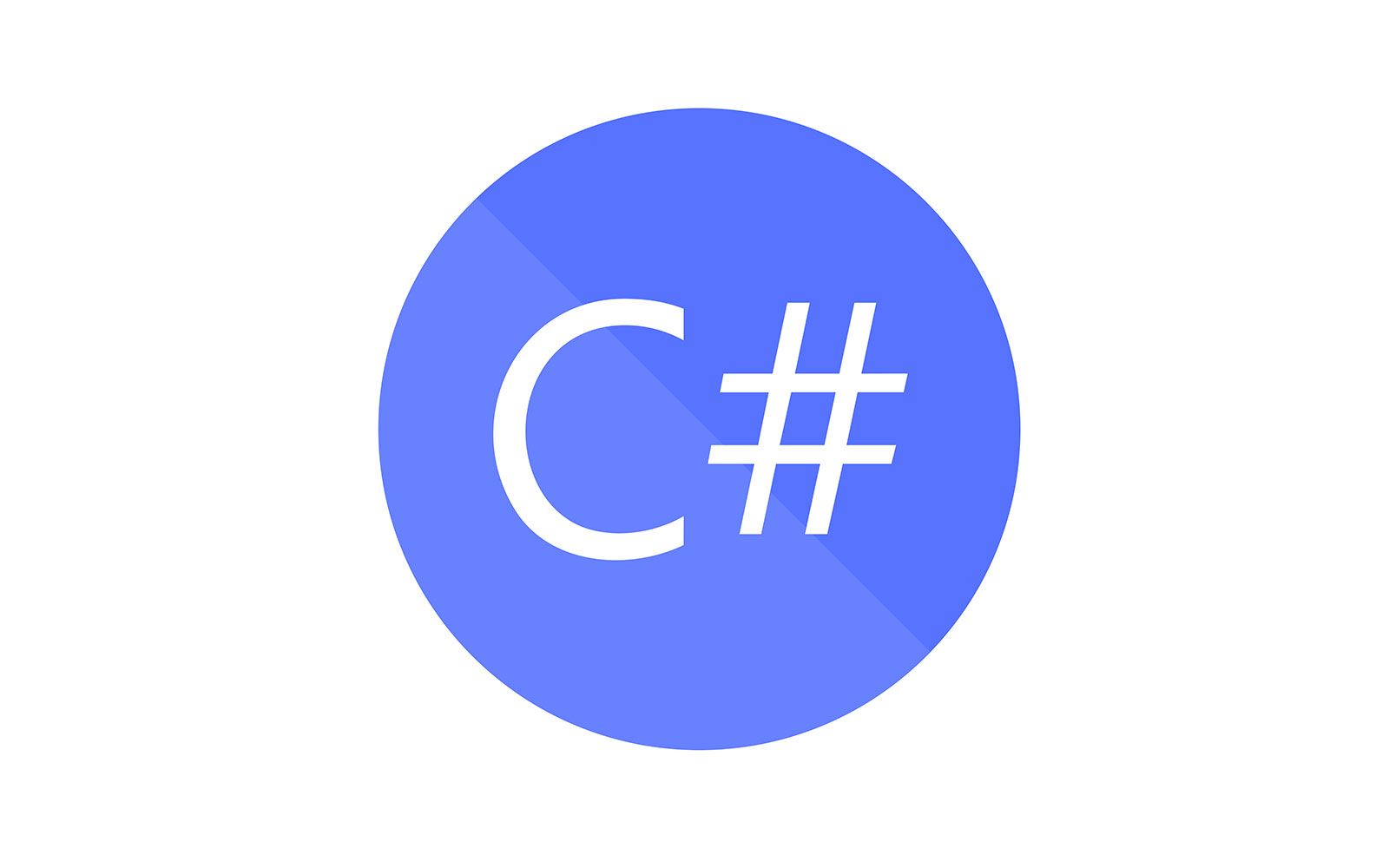
Here are some basic for loop exercises in C# . These exercises stick to simple counting and looping tasks that help students practice the structure of for
loops.
Exercise 1: Print Numbers from 1 to 10
Objective: Introduce the structure of a for
loop.
// Print numbers from 1 to 10
for (int i = 1; i <= 10; i++)
{
Console.WriteLine(i);
}
Exercise 2: Print Even Numbers from 2 to 20
Objective: Understand how to control the loop step size.
// Print even numbers from 2 to 20
for (int i = 2; i <= 20; i += 2)
{
Console.WriteLine(i);
}
Exercise 3: Print Odd Numbers from 1 to 19
Objective: Practice using a loop to print specific numbers (odd).
// Print odd numbers from 1 to 19
for (int i = 1; i < 20; i += 2)
{
Console.WriteLine(i);
}
Exercise 4: Print Multiplication Table of a Given Number
Objective: Use a for
loop to display a multiplication table.
// Print multiplication table of 5
int number = 5;
for (int i = 1; i <= 10; i++)
{
Console.WriteLine(number + " x " + i + " = " + (number * i));
}
Exercise 5: Calculate the Sum of Numbers from 1 to N
Objective: Use a for
loop to calculate a sum up to a given number, N
.
// Calculate and print the sum of numbers from 1 to N
int N = 10; // You can change N to any positive integer
int total = 0;
for (int i = 1; i <= N; i++)
{
total += i;
}
Console.WriteLine("Sum: " + total);
Exercise 6: Count Down from 10 to 1
Objective: Use a decrementing for
loop.
// Print numbers from 10 down to 1
for (int i = 10; i >= 1; i--)
{
Console.WriteLine(i);
}
Exercise 7: Print Powers of 2 up to 1024
Objective: Use a for
loop to demonstrate exponential growth by printing powers of 2.
// Print powers of 2 up to 1024
for (int i = 1; i <= 1024; i *= 2)
{
Console.WriteLine(i);
}
Exercise 8: Calculate the Factorial of a Given Number
Objective: Use a for
loop to calculate factorial (multiplying a series of numbers).
// Calculate and print the factorial of a given number, N
int N = 5; // Change N to any positive integer
int factorial = 1;
for (int i = 1; i <= N; i++)
{
factorial *= i;
}
Console.WriteLine("Factorial of " + N + " is " + factorial);
Here’s a for loop exercise in C# to print a simple triangle pattern. This exercise will help students practice using nested loops to create patterns.
Exercise 9: Create a Triangle Pattern
Objective: Use nested for
loops to print a triangle pattern with stars (*
).
// Create a triangle pattern
int rows = 5; // Number of rows in the triangle
for (int i = 1; i <= rows; i++)
{
for (int j = 1; j <= i; j++)
{
Console.Write("*");
}
Console.WriteLine(); // Move to the next line after each row
}
Expected Output:
*
**
***
****
*****
Explanation
The outer loop (
for
loop withi
) controls the number of rows.The inner loop (
for
loop withj
) controls the number of*
characters printed on each row, increasing with each iteration of the outer loop.
Exercise 10: Right-Aligned Triangle
Objective: Print a right-aligned triangle using spaces and stars.
int rows = 5;
for (int i = 1; i <= rows; i++)
{
for (int j = 1; j <= rows - i; j++)
{
Console.Write(" ");
}
for (int k = 1; k <= i; k++)
{
Console.Write("*");
}
Console.WriteLine();
}
Expected Output:
*
**
***
****
*****
Exercise 11: Inverted Left-Aligned Triangle
Objective: Print an inverted left-aligned triangle.
int rows = 5;
for (int i = rows; i >= 1; i--)
{
for (int j = 1; j <= i; j++)
{
Console.Write("*");
}
Console.WriteLine();
}
Expected Output:
*****
****
***
**
*
Exercise 12: Inverted Right-Aligned Triangle
Objective: Print an inverted right-aligned triangle.
int rows = 5;
for (int i = rows; i >= 1; i--)
{
for (int j = 1; j <= rows - i; j++)
{
Console.Write(" ");
}
for (int k = 1; k <= i; k++)
{
Console.Write("*");
}
Console.WriteLine();
}
Expected Output:
*****
****
***
**
*
Exercise 13: Symmetrical Pyramid
Objective: Print a symmetrical pyramid.
int rows = 5;
for (int i = 1; i <= rows; i++)
{
for (int j = 1; j <= rows - i; j++)
{
Console.Write(" ");
}
for (int k = 1; k <= (2 * i - 1); k++)
{
Console.Write("*");
}
Console.WriteLine();
}
Expected Output:
*
***
*****
*******
*********
Exercise 14: Inverted Symmetrical Pyramid
Objective: Print an inverted symmetrical pyramid.
int rows = 5;
for (int i = rows; i >= 1; i--)
{
for (int j = 1; j <= rows - i; j++)
{
Console.Write(" ");
}
for (int k = 1; k <= (2 * i - 1); k++)
{
Console.Write("*");
}
Console.WriteLine();
}
Expected Output:
*********
*******
*****
***
*
Subscribe to my newsletter
Read articles from Arzath Areeff directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arzath Areeff
Arzath Areeff
I co-founded digizen.lk to promote online safety and critical thinking. Currently, I’m developing an AI app to fight misinformation. As Founder and CEO of ideaGeek.net, I help turn startup dreams into reality, and I share tech insights and travel stories on my YouTube channels, TechNomad and Rz Omar.