A Comprehensive Guide to React State Management
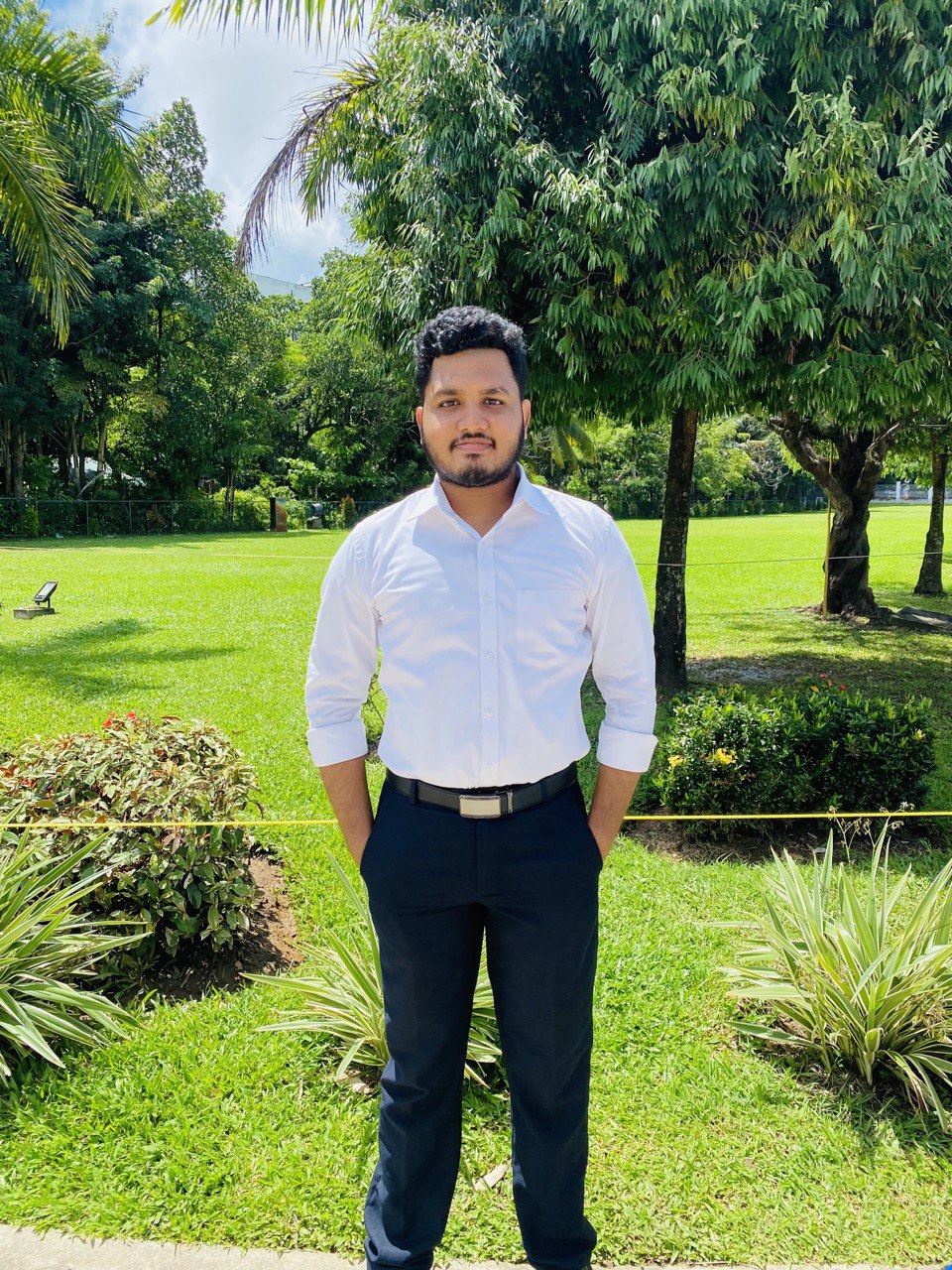
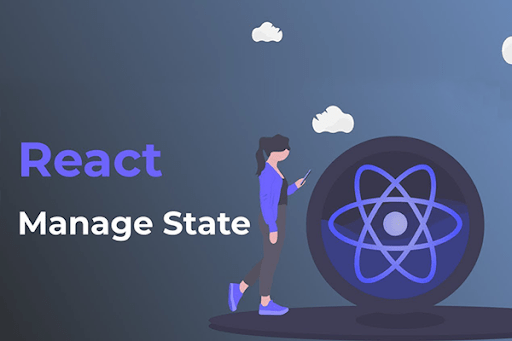
What is State in React?
In React, "state" refers to the data that a component manages and renders. A component’s state can hold any data related to the UI, such as form input values, user preferences, or API responses. React manages state as an object, and updating the state triggers a re-render of the component, reflecting any UI changes.
Types of State in React Applications
Managing state well requires understanding its types and scope. The main types of state in React applications include:
Local State: Data managed within a single component using
useState
oruseReducer
.Global State: State shared across multiple components, often through context or libraries like Redux.
Server State: Data fetched from an external server, requiring synchronization between the server and UI. Tools like
React Query
this can help manage server state.URL State: Data stored in the URL, such as query parameters, used for pagination, search filters, or navigation.
Built-in State Management Options
React provides several hooks for managing state natively:
1. useState
The useState
hook is a core method for managing local state in functional components. Here’s an example of a simple counter using useState
:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
2. useReducer
For more complex state logic, useReducer
is ideal. It’s similar to useState
but allows for managing state through actions, reducing state updates to functions. Here’s a simple example:
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
}
3. useContext
React's Context API allows sharing state across components without prop drilling. However, Context API alone may not be enough for managing complex state or for performance-sensitive applications.
import React, { createContext, useState, useContext } from 'react';
const ThemeContext = createContext();
function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
}
function App() {
const { theme, setTheme } = useContext(ThemeContext);
return (
<div className={theme}>
<button onClick={() => setTheme(theme === 'light' ? 'dark' : 'light')}>
Toggle Theme
</button>
</div>
);
}
Global State Management Libraries
While React's built-in state management tools are suitable for small to medium projects, larger applications often require more robust solutions. Below are some popular libraries to manage global state effectively.
1. Redux
Redux is one of the most widely used libraries for managing global state. It introduces a predictable, centralized store where state is immutable, and updates happen only through dispatched actions and reducers.
Pros: Predictable, excellent developer tools, community support.
Cons: Boilerplate-heavy, especially for simple applications.
Here’s a basic example with Redux Toolkit:
// store.js
import { configureStore, createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: (state) => state + 1,
decrement: (state) => state - 1,
},
});
export const { increment, decrement } = counterSlice.actions;
const store = configureStore({
reducer: {
counter: counterSlice.reducer,
},
});
export default store;
2. Zustand
Zustand is a lightweight and flexible library that simplifies state management. It uses a hook-based approach, making it intuitive to use.
Pros: Minimalistic, flexible, no boilerplate.
Cons: Limited for very complex applications.
Example:
import create from 'zustand';
const useStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
function Counter() {
const { count, increment, decrement } = useStore();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
3. Recoil
Recoil is a state management library from Facebook that integrates seamlessly with React. It introduces "atoms" for state and "selectors" for derived state.
Pros: Built for React, great for complex dependency management.
Cons: Limited community compared to Redux.
import { atom, selector, useRecoilState, useRecoilValue } from 'recoil';
const countState = atom({
key: 'countState',
default: 0,
});
const doubleCountState = selector({
key: 'doubleCountState',
get: ({ get }) => get(countState) * 2,
});
function Counter() {
const [count, setCount] = useRecoilState(countState);
const doubleCount = useRecoilValue(doubleCountState);
return (
<div>
<p>Count: {count}</p>
<p>Double Count: {doubleCount}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
}
Conclusion
React offers a variety of tools for state management. The best choice depends on your app’s complexity, size, and requirements. For smaller applications, native hooks like useState
and useContext
are often enough. For more complex needs, libraries like Redux, Zustand, and Recoil provide powerful solutions to efficiently manage global state.
Whichever approach you choose, understanding the core principles of state management will help you build more efficient and scalable React applications. Happy coding!
Subscribe to my newsletter
Read articles from Sasika Chandila directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
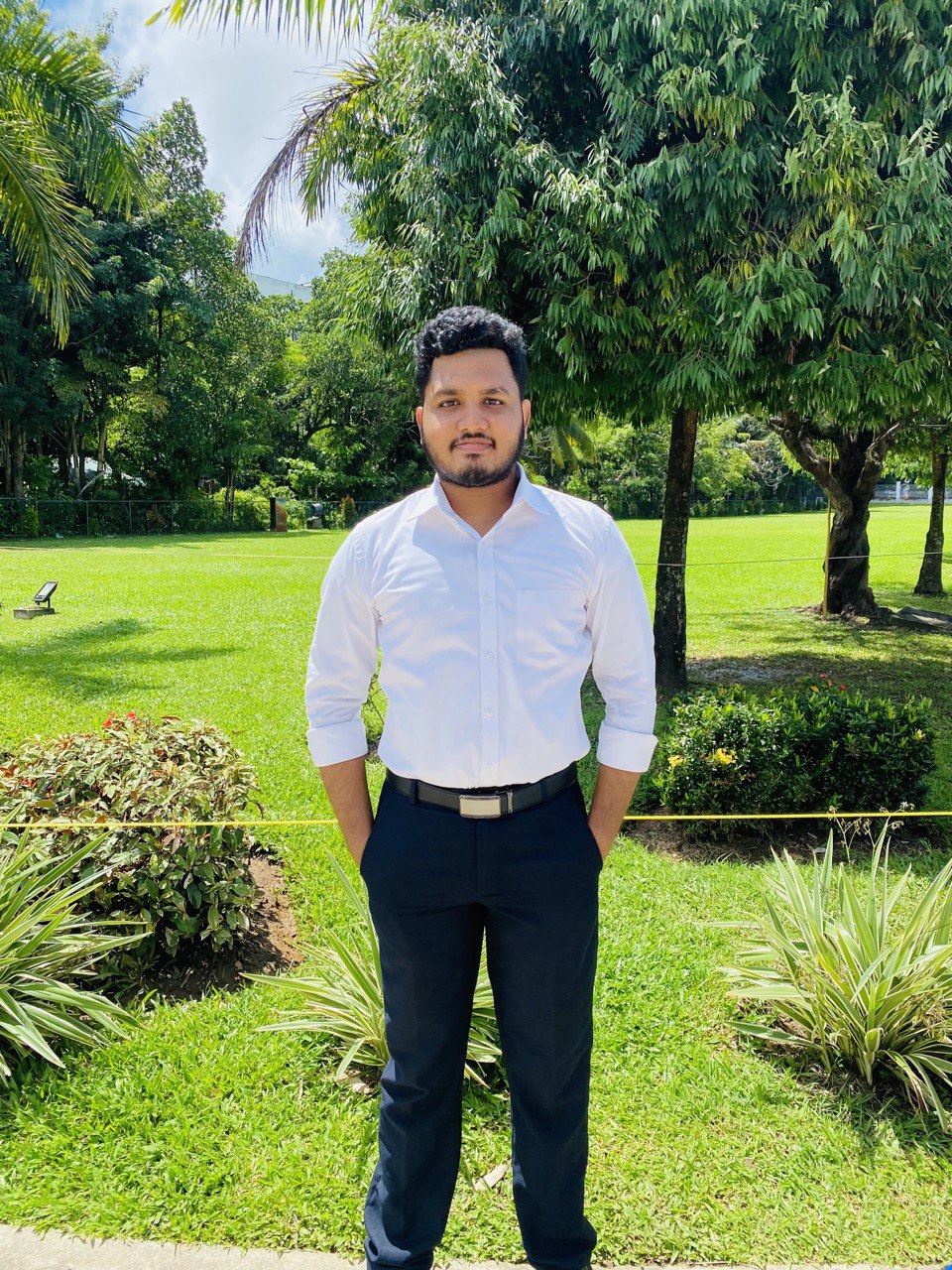
Sasika Chandila
Sasika Chandila
Aspiring Undergraduate Software Engineer | Full Stack Developer | Java Enthusiast 🚀 Greetings! 👋 I'm Sasika Chandila, an ambitious Software Engineering undergraduate with a passion for crafting innovative solutions and enhancing user experiences. 🌐 🛠️ Tech Stack: Java: Leveraging the power of Java to build robust backend systems. React & Flutter: Creating dynamic and responsive user interfaces that captivate and engage. C# & Kotlin: Proficient in developing versatile applications for various platforms. MongoDB & SQL: Building scalable and efficient databases to drive seamless data management. HTML & CSS: Crafting visually appealing and user-friendly web applications. 🌟 Highlights Problem Solver: I thrive on challenges and enjoy solving complex problems with elegant solutions. Collaborative Team Player: Experienced in working within interdisciplinary teams to achieve common goals. Continuous Learner: Committed to staying at the forefront of technological advancements through ongoing learning and professional development. 🎓 Education: Currently pursuing a degree in Software Engineering at NIBM. 🔗 Connect with Me: Let's connect and explore opportunities to collaborate, share insights, and contribute to the ever-evolving world of technology. Open to exciting projects, internships, and networking opportunities! #SoftwareEngineering #FullStackDeveloper #TechInnovation #OpenToOpportunities