Solving CS50P - Problem Set 5
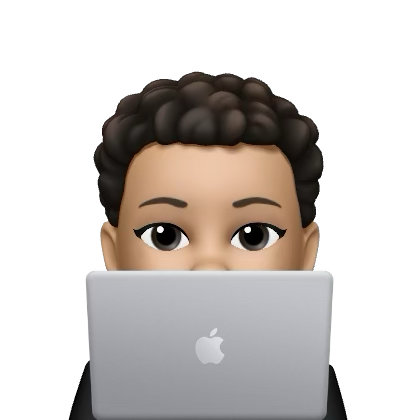
3 min read
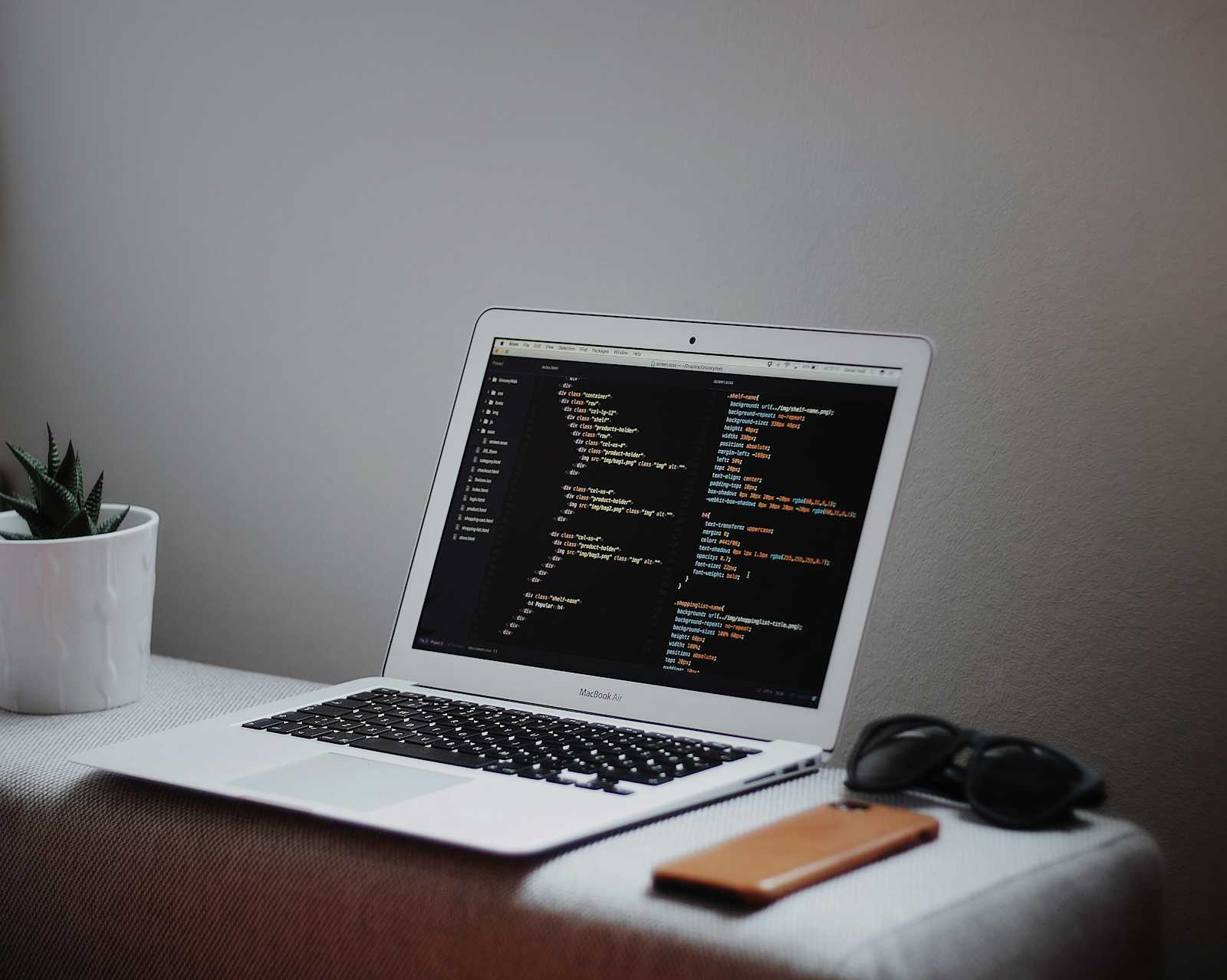
In this Problem set we revisited some of our older problems and wrote test code to check that the code is working as expected. With the help of the pytest library we were able to test our code in the terminal, by calling pytest (filename). Pytest is a useful tool that helps you test the integrity of your code.
ℹ
Disclaimer: The following code solutions are for educational purposes only and are not intended to be used or submitted as your own. Cheating is a violation of the Academic Honesty of the course. All problem sets presented are owned by Harvard University.
Testing my twttr
from twttr import shorten
def main():
test_uppercase()
test_lowercase()
test_numeric()
test_no_replacement()
test_punctuation()
test_printing_uppercase()
def test_uppercase():
for v in ["A", "E", "I", "O", "U"]:
assert shorten(v) == ""
def test_lowercase():
for v in ["a", "e", "i", "o", "u"]:
assert shorten(v) == ""
def test_numeric():
assert shorten("50") == "50"
def test_no_replacement():
for v in ["A", "E", "I", "O", "U"]:
assert shorten(v) != v
def test_punctuation():
for p in ["!", ".", "?", ","]:
assert shorten(p) == p
def test_printing_uppercase():
assert shorten("hi") == "h"
if __name__ == "__main__":
main()
Back to the Bank
from bank import value
def main():
test_hello()
test_h()
test_other()
test_case()
def test_hello():
assert value("hello") == 0
def test_h():
assert value("h") == 20
assert value("howdy") == 20
def test_other():
assert value("greetings") == 100
assert value("salut") == 100
def test_case():
assert value("Hello") == 0
assert value("H") == 20
assert value("Greetings") == 100
if __name__ == "__main__":
main()
Re-requesting a Vanity Plate
from plates import is_valid
def main():
test_length()
test_beginning_alphabetical()
test_zero_placement()
test_number_placement()
test_puncuation()
def test_length():
assert is_valid("GOODBYE") == False
assert is_valid("B") == False
assert is_valid("AB") == True
assert is_valid("ABCDEF") == True
def test_beginning_alphabetical():
assert is_valid("NO") == True
assert is_valid("CS50") == True
assert is_valid("123456") == False
assert is_valid("A123") == False
def test_zero_placement():
assert is_valid("ABC07") == False
assert is_valid("AC70") == True
def test_number_placement():
assert is_valid("CS50P") == False
assert is_valid("ABC7D") == False
def test_punctuation():
assert is_valid("PI3.14") == False
assert is_valid("PI3!14") == False
assert is_valid("PI3 14") == False
if __name__ == "__main__":
main()
Refueling
from fuel import convert
from fuel import gauge
import pytest
def main():
test_full()
test_empty()
test_regular()
test_str()
test_larger_numerator()
test_zero_denonominator()
def test_full():
assert gauge(99) == "F"
assert gauge(100) == "F"
def test_empty():
assert gauge(1) == "E"
assert gauge(0.9) == "E"
def test_regular():
assert gauge(50) == "50%"
assert gauge(25) == "25%"
assert gauge(12) == "12%"
def test_convert():
assert convert("1/2") == 50
assert convert("9/10") == 90
assert convert("3/4") == 75
assert convert("0/100") == 0
def test_str():
with pytest.raises(ValueError):
convert("cat/dog")
def test_larger_numerator():
with pytest.raises(ValueError):
convert("9/2")
def test_zero_denonominator():
with pytest.raises(ZeroDivisionError):
convert("1/0")
if __name__ == "__main__":
main()
0
Subscribe to my newsletter
Read articles from Karabo Molefi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
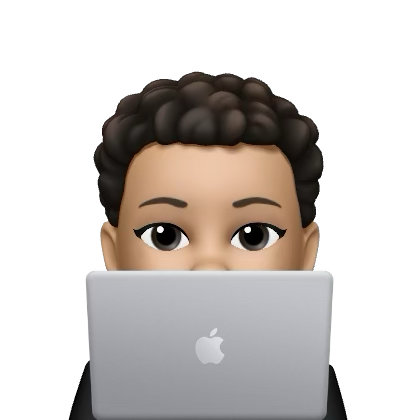