Solving CS50P - Problem Set 7
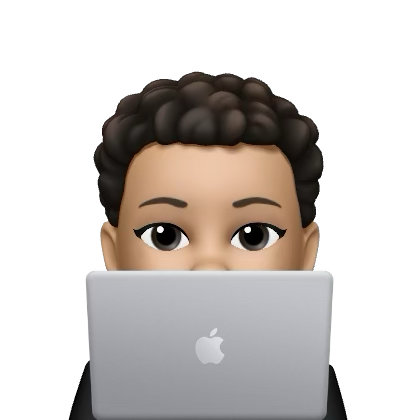
3 min read
In this problem set we explore the use of regexes (regular expressions) to examine patterns within our code. Regular expressions enable us to examine expressions in patterns, for a example that of an email address.
ℹ
Disclaimer: The following code solutions are for educational purposes only and are not intended to be used or submitted as your own. Cheating is a violation of the Academic Honesty of the course. All problem sets presented are owned by Harvard University.
NUMB3RS
import re
import sys
def main():
print(validate(input("IPv4 Address: ")))
def validate(ip):
if matches := re.search(r"^(\d+)\.(\d+)\.(\d+)\.(\d+)$", ip):
numbers = matches.groups()
for n in numbers:
if int(n) >=0 and int(n) <= 255:
continue
else:
return False
else:
return False
return True
if __name__ == "__main__":
main()
from numb3rs import validate
def main():
test_length()
test_period()
test_range()
def test_length():
assert validate("1.2.3.4") == True
assert validate("0.1.2.3") == True
assert validate("1.2.3.4.5") == False
def test_period():
assert validate("1.2.3.4.") == False
assert validate(".1.2.3.4.") == False
assert validate(".1.2.3.4") == False
def test_range():
assert validate("256.257.258.259") == False
assert validate("250.257.258.259") == False
assert validate("-256.257.258.259") == False
assert validate("-1.-2.-3.-4") == False
assert validate("255.200.100.0") == True
Watch on YouTube
import re
import sys
def main():
print(parse(input("HTML: ")))
def parse(s):
if matches := re.search(r"^<iframe\s?(?:width=\"\d+\")?\s?(?:height=\"\d+\")?\ssrc=\"https?://(?:www\.)?youtube.com/embed/(\w+)+\"\s?(?:title=\"Youtube video player\")?\s?(?:frameborder=\"\d+\")?\s?(?:allow=\"(?:(?:(?:\w|-)+);?\s?)+\")?\s?(?:allowfullscreen)?></iframe>$", s, re.IGNORECASE):
return f"https://youtu.be/{matches.group(1)}"
if __name__ == "__main__":
main()
Working 9 to 5
import re
import sys
def main():
print(convert(input("Hours: ")))
def convert(s):
matches = re.search(r"^(1[0-2]|[0-9]):[0-5][0-9]\s(AM|PM)\sto\s(1[0-2]|[0-9]):[0-5][0-9]\s(AM|PM)$", s) or \
re.search(r"^(1[0-2]|[0-9])\s(AM|PM)\sto\s(1[0-2]|[0-9])\s(AM|PM)$",s)
if matches:
if re.search(":", s):
return process1(s)
else:
return process2(s)
else:
raise ValueError
def process1(s):
new = []
times = s.split(" to ")
for time in times:
if re.search("AM", time):
time = time.replace(" AM","")
hours, min = time.split(":")
if int(hours) < 10:
hours = "0" + hours
elif int(hours) == 12:
hours = "00"
new.append(f"{hours}:{min}")
else:
time = time.replace(" PM","")
hours, min = time.split(":")
if int(hours) <= 10:
hours = 12 + int(hours)
hours = str(hours)
elif int(hours) == 12:
hours = "12"
new.append(f"{hours}:{min}")
return f"{new[0]} to {new[1]}"
def process2(s):
new = []
times = s.split(" to ")
for time in times:
if re.search("AM", time):
time = time.replace(" AM", "")
if int(time) < 10:
time = "0" + time + ":00"
elif int(time) == 12:
time = "00:00"
else:
time = time + ":00"
new.append(time)
else:
time = time.replace(" PM", "")
if int(time) != 12:
time = 12 + int(time)
time = str(time) + ":00"
else:
time = "12:00"
new.append(time)
return f"{new[0]} to {new[1]}"
if __name__ == "__main__":
main()
import pytest
from working import convert
def main():
test_convert()
test_omission()
test_range()
def test_convert():
assert convert("9 AM to 5 PM") == "09:00 to 17:00"
assert convert("9:00 AM to 5:00 PM") == "09:00 to 17:00"
assert convert("10 PM to 8 AM") == "22:00 to 08:00"
def test_omission():
try:
convert("9 AM 5 PM")
except ValueError:
pass
else:
assert False
def test_range():
try:
convert("13 AM to 19 PM")
except ValueError:
pass
else:
assert False
if __name__ == "__main__":
main()
Regular, um, Expressions
import re
import sys
def main():
print(count(input("Text: ")))
def count(s):
matches = re.findall(r"\bum\b", s, re.IGNORECASE)
return len(matches)
if __name__ == "__main__":
main()
from um import count
def main():
test_count()
test_word_bound()
test_case()
def test_count():
assert count("um") == 1
def test_word_bound():
assert count("yummy") == 0
assert count("umm mum") == 0
assert count("um mummy") == 1
def test_case():
assert count("UM") == 1
assert count("Um") == 1
if __name__ == "__main__":
main()
Response Validation
0
Subscribe to my newsletter
Read articles from Karabo Molefi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
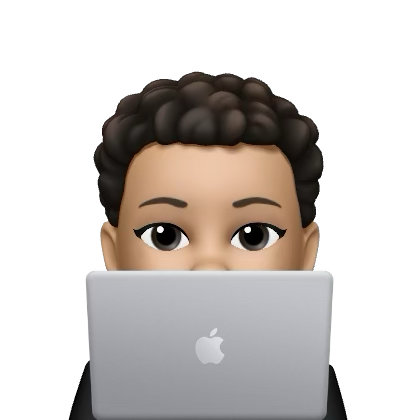