How Hugging Face tools make traditional setups obsolete, enabling shorter code?
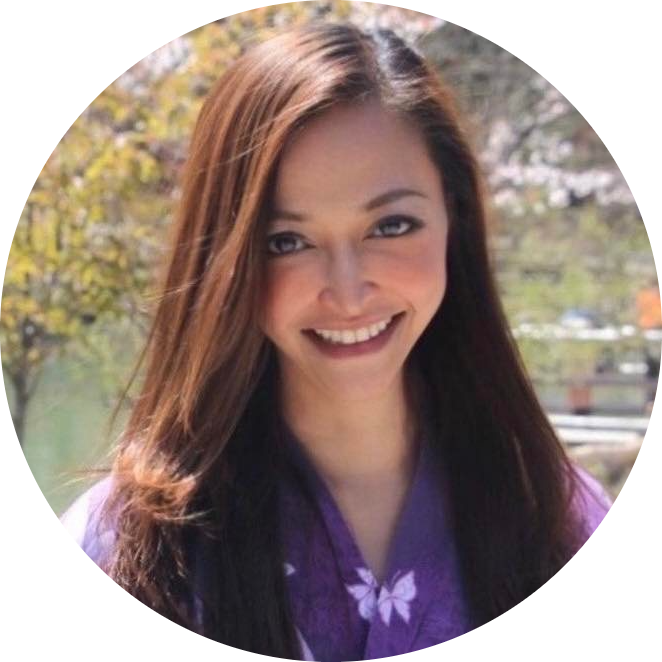
Table of contents
- Hugging Face Library Overview
- 1. Transformers Library: Unified Model Access
- 2. Tokenizers Library: High-Speed Tokenization
- 3. Datasets: Ready-to-Use Data for NLP Tasks
- 4. Trainer API: Simplified Model Training
- 5. PEFT: Efficient Fine-Tuning for Large Models
- 6. Spaces & Gradio: Interactive Model Demos Made Easy
- 7. AutoModel and Pipeline API: Reducing Setup Complexity
- Conclusion
As the landscape of Natural Language Processing (NLP) and machine learning continues to grow, Hugging Face has emerged as a go-to platform, offering a unified ecosystem that greatly simplifies model training, fine-tuning, and deployment. Before Hugging Face, workflows often involved piecing together various libraries and tools, which could be time-consuming and inefficient. Here, we’ll walk through Hugging Face’s major components, showcasing how they replace older libraries and streamline complex tasks.
Let’s start with a quick summary table, followed by a deeper dive into each component and its advantages.
Hugging Face Library Overview
Hugging Face Component | Replaces | Benefits |
Transformers Library | TensorFlow, PyTorch (manual) | Pre-trained models with easy loading and fine-tuning |
Tokenizers Library | NLTK, spaCy | Fast tokenization, optimized for transformer models |
Datasets | TensorFlow Datasets, manual scripts | Standardized, easily accessible data for NLP tasks |
Trainer API | Custom training loops (PyTorch) | Simplified training, evaluation, and multi-GPU support |
PEFT | Full model fine-tuning | Efficient memory and compute use for large models |
Accelerate | Custom distributed training setups | Multi-GPU/TPU support, device management |
Hugging Face Hub | Private model repositories | Centralized, versioned model sharing and access |
Model Card | Ad-hoc model documentation | Standardized, detailed guidance for model use |
Hub Client | Custom scripts for automation | Automated model management with a few lines of code |
Spaces | Custom server setups for demos | Easy, hosted interactive ML app deployment |
Gradio | Jupyter widgets, Flask for UIs | Quick, user-friendly UIs for ML demos |
AutoModel / AutoTokenizer | Manual model and tokenizer setup | Automatic configuration, supports model switching |
Pipeline API | Custom code for common NLP tasks | One-line solutions for tasks like text generation |
1. Transformers Library: Unified Model Access
Before Hugging Face, models were implemented manually in TensorFlow or PyTorch, requiring extensive setup. Hugging Face's Transformers Library simplifies this with thousands of pre-trained models for text, vision, and audio tasks, making model loading and fine-tuning easier.
Example: Sentiment Analysis
from transformers import pipeline
classifier = pipeline("sentiment-analysis")
result = classifier("I love using the Transformers library!")
print("Sentiment:", result)
2. Tokenizers Library: High-Speed Tokenization
Tokenization was traditionally handled by libraries like NLTK or spaCy. Hugging Face’s Tokenizers Library optimizes this process with fast, transformer-specific tokenizers that handle large datasets efficiently.
Example: Tokenizing Text for BERT
from transformers import BertTokenizer
tokenizer = BertTokenizer.from_pretrained("bert-base-uncased")
tokens = tokenizer.tokenize("Hello, Hugging Face!")
input_ids = tokenizer.encode("Hello, Hugging Face!", add_special_tokens=True)
print("Tokens:", tokens)
print("Token IDs:", input_ids)
3. Datasets: Ready-to-Use Data for NLP Tasks
Before Hugging Face, loading and preprocessing data often required TensorFlow Datasets or custom scripts. Hugging Face’s Datasets Library offers standardized datasets, making it easier to get started with high-quality data.
Example: IMDb Sentiment Dataset
from datasets import load_dataset
dataset = load_dataset("imdb")
print("Sample:", dataset['train'][0])
4. Trainer API: Simplified Model Training
Custom training loops in PyTorch were once the norm. The Trainer API automates training, evaluation, and model saving, with built-in support for metrics and multi-GPU training.
Example: Fine-Tuning a Sentiment Analysis Model
from transformers import Trainer, TrainingArguments, AutoModelForSequenceClassification, AutoTokenizer
from datasets import load_dataset
dataset = load_dataset("imdb")
tokenizer = AutoTokenizer.from_pretrained("distilbert-base-uncased")
model = AutoModelForSequenceClassification.from_pretrained("distilbert-base-uncased")
training_args = TrainingArguments(output_dir="./results", num_train_epochs=3, per_device_train_batch_size=8)
trainer = Trainer(model=model, args=training_args, train_dataset=dataset["train"])
trainer.train()
5. PEFT: Efficient Fine-Tuning for Large Models
Large model fine-tuning used to require significant compute resources. PEFT (Parameter Efficient Fine-Tuning) reduces memory usage by updating only a subset of parameters, making fine-tuning feasible on limited hardware.
Example: Fine-Tuning GPT-2 with LoRA
from transformers import AutoModelForCausalLM, AutoTokenizer
from peft import get_peft_model, LoraConfig, TaskType
model_name = "gpt2"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForCausalLM.from_pretrained(model_name)
lora_config = LoraConfig(task_type=TaskType.CAUSAL_LM, r=8, lora_alpha=32, lora_dropout=0.1)
peft_model = get_peft_model(model, lora_config)
inputs = tokenizer("PEFT is efficient.", return_tensors="pt")
outputs = peft_model(**inputs, labels=inputs["input_ids"])
print("Loss:", outputs.loss.item())
6. Spaces & Gradio: Interactive Model Demos Made Easy
Building interactive demos previously required custom server setups. Hugging Face Spaces and Gradio offer fast, hosted deployment for ML applications, letting users easily share models through web UIs.
Example: Sentiment Analysis Demo with Gradio
import gradio as gr
from transformers import pipeline
sentiment_pipeline = pipeline("sentiment-analysis")
def analyze_sentiment(text):
return sentiment_pipeline(text)[0]["label"]
demo = gr.Interface(fn=analyze_sentiment, inputs="text", outputs="text")
demo.launch()
7. AutoModel and Pipeline API: Reducing Setup Complexity
Before Hugging Face, setting up a model and tokenizer required model-specific knowledge. The AutoModel and Pipeline API reduce setup complexity by automatically selecting models and handling tokenization, making NLP tasks accessible with minimal code.
Example: Question Answering with Pipeline API
from transformers import pipeline
qa_pipeline = pipeline("question-answering")
result = qa_pipeline({
"question": "What is Transformers library?",
"context": "Transformers is a library by Hugging Face."
})
print("Answer:", result['answer'])
Conclusion
By consolidating model access, tokenization, training, and deployment in one ecosystem, Hugging Face offers a powerful alternative to traditional libraries. Each component is designed to be compatible, flexible, and scalable, allowing machine learning practitioners to focus on innovation rather than setup. Whether you’re training a model, deploying an app, or simply exploring NLP, Hugging Face tools simplify workflows and improve productivity.
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
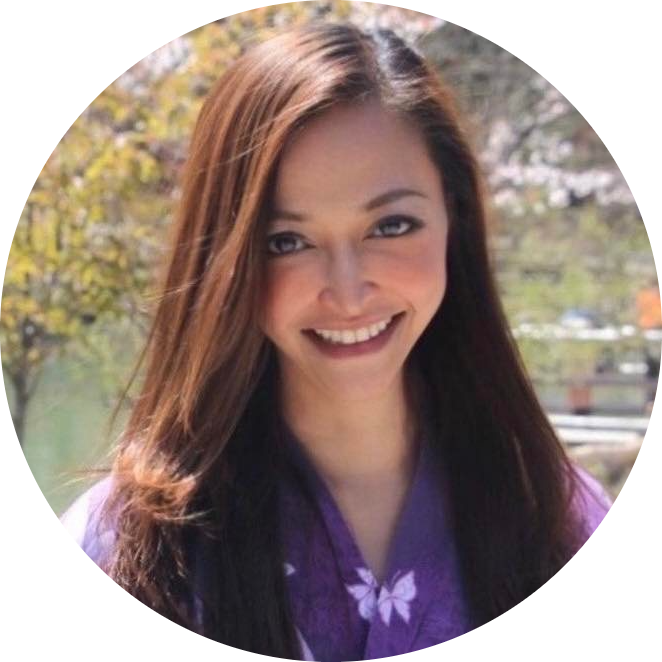