Introduction to SQL Language: SQL Syntax and Command Structure
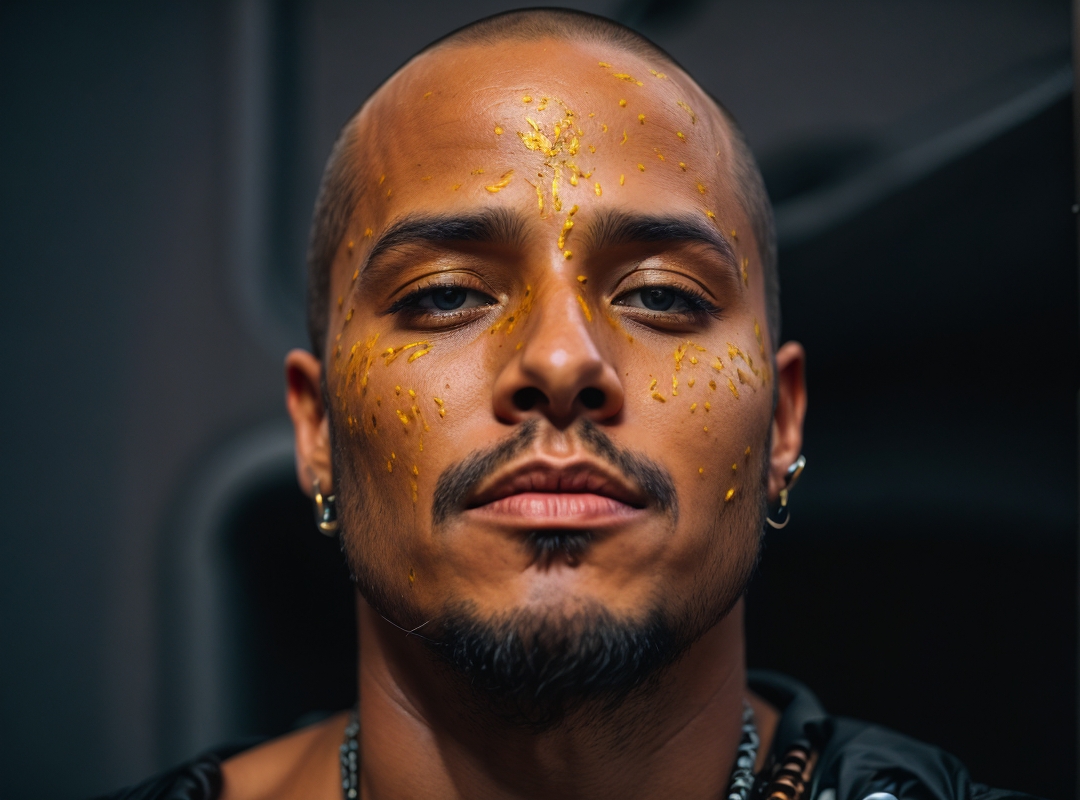
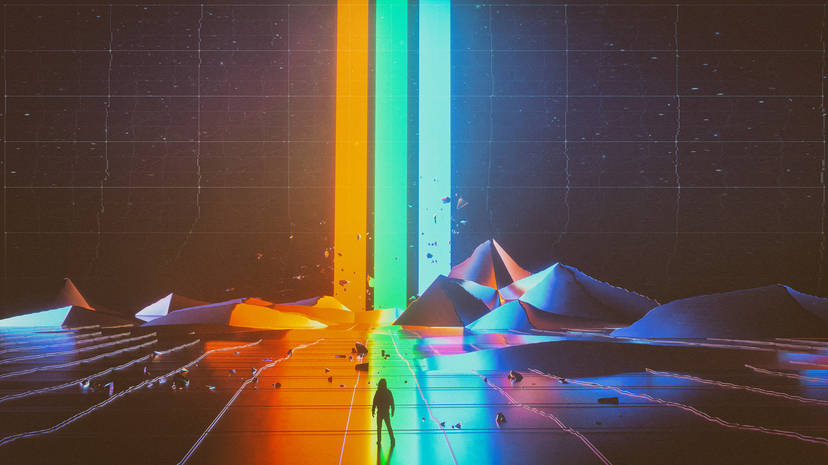
SQL, as a declarative language, is designed with an intuitive syntax that resembles natural language. This design philosophy allows users to express their data management needs succinctly and clearly. In this section, we will delve deeper into the structural elements of SQL statements, the types of literals, keywords, identifiers, and the use of comments for better documentation.
SQL Statement Structure
An SQL statement typically begins with a verb that signifies the action you want to perform, such as SELECT
, INSERT
, UPDATE
, or DELETE
. This command is followed by clauses that define the operation's scope and parameters.
Example of an SQL Statement
Here’s a breakdown of a simple SQL statement:
SELECT
first_name
FROM
employees
WHERE
YEAR(hire_date) = 2000;
This statement can be read as "Get the first names of employees who were hired in 2000." The components include:
SELECT Clause: Specifies the columns that you want to retrieve, in this case,
first_name
.FROM Clause: Indicates the table from which to retrieve the data, here
employees
.WHERE Clause: (Optional) Sets conditions to filter the results. In this statement, it filters for employees hired in the year 2000.
Mandatory and Optional Clauses
Mandatory Clauses: The
SELECT
andFROM
clauses are essential for any SQL query.Optional Clauses: Additional clauses, such as
WHERE
,GROUP BY
, orORDER BY
, enhance the query's functionality but are not strictly required.
SQL Syntax Explained
SQL's simplicity makes it accessible to various users, from non-technical stakeholders to seasoned developers. Unlike imperative programming languages (e.g., PHP, Java, C++), which require you to explain how to perform tasks, SQL focuses on what you want to achieve. This declarative nature allows for straightforward query writing, suited for generating ad-hoc reports and queries.
SQL Commands
Each SQL command typically ends with a semicolon (;
), which serves as a delimiter indicating the conclusion of a command. Here are examples of different SQL commands:
SELECT
first_name, last_name
FROM
employees;
DELETE FROM employees
WHERE
hire_date < '1990-01-01';
In the above examples:
The first command retrieves first and last names from the
employees
table.The second command deletes records of employees hired before January 1, 1990.
SQL Tokens
SQL statements consist of tokens, which are the fundamental building blocks. Tokens can be categorized into:
Literals: Explicit values in SQL commands, such as string, numeric, or binary literals.
Keywords: Reserved words with special meanings, such as
SELECT
,INSERT
, andDROP
.Identifiers: The names of database objects, including tables and columns.
Types of Literals
Literals in SQL are values that remain constant throughout the execution of the query. They can be divided into three main categories:
String Literals: Enclosed in single quotes, they represent text values.
'John' '1990-01-01' 'string example'
Numeric Literals: Represent numbers, which can be integers or decimals.
200 -- An integer -5 -- A negative integer 6.022 -- A decimal number
Binary Literals: Used to represent binary values in hexadecimal format.
x'01' x'0f0ff'
It's important to note that SQL is case-sensitive regarding string literals, meaning 'John' and 'JOHN' would be treated as different values. However, SQL keywords and identifiers are case-insensitive.
SQL Keywords and Identifiers
Keywords: Reserved words that cannot be used as identifiers for tables, columns, or other database objects. Common keywords include
SELECT
,INSERT
,UPDATE
,DELETE
,FROM
, etc.Identifiers: Names used to refer to database objects such as tables, columns, and indexes. SQL treats the identifiers as case-insensitive, so:
SELECT * FROM employees; SELECT * FROM EMPLOYEES;
In both cases, the commands are equivalent.
Coding Conventions
To enhance readability, it's a common practice to write SQL keywords in uppercase and identifiers in lowercase:
SELECT employee_id, salary
FROM employees
WHERE salary < 3000;
Comments in SQL
Comments in SQL allow developers to annotate their queries and document their logic. They are ignored by the SQL parser during execution.
Single-Line Comments
Single-line comments are initiated by two consecutive hyphens--
. Everything following the --
on that line will be treated as a comment.
Example of a Single-Line Comment:
SELECT
employee_id, salary
FROM
employees
WHERE
salary < 3000; -- This retrieves employees with a salary less than 3000
Multi-Line Comments
For comments spanning multiple lines, you can use the C-style notation, which starts with /*
and ends with */
. This is particularly useful for longer comment blocks or documentation.
Example of a Multi-Line Comment:
/*
This query updates the salary of employees whose
current salary is less than 3000, increasing it by 5%.
*/
UPDATE employees
SET
salary = salary * 1.05
WHERE
salary < 3000;
Using comments effectively in your SQL code can make a significant difference in code maintainability, especially when collaborating with others or revisiting your code after some time.
Conclusion
In this tutorial, we introduced the fundamental aspects of SQL syntax and structure, demonstrating how SQL enables users to express their data management requirements in an intuitive manner. We covered key elements including the structure of SQL statements, the role of mandatory and optional clauses, the types of SQL commands, and the importance of using literals, keywords, identifiers, and comments.
By understanding these concepts, you’ll be better equipped to write SQL statements that are not only functional but also easy to read and maintain. SQL's declarative nature allows for efficient querying and data manipulation, making it an invaluable skill for developers, analysts, and anyone working with data.
Subscribe to my newsletter
Read articles from Younis Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
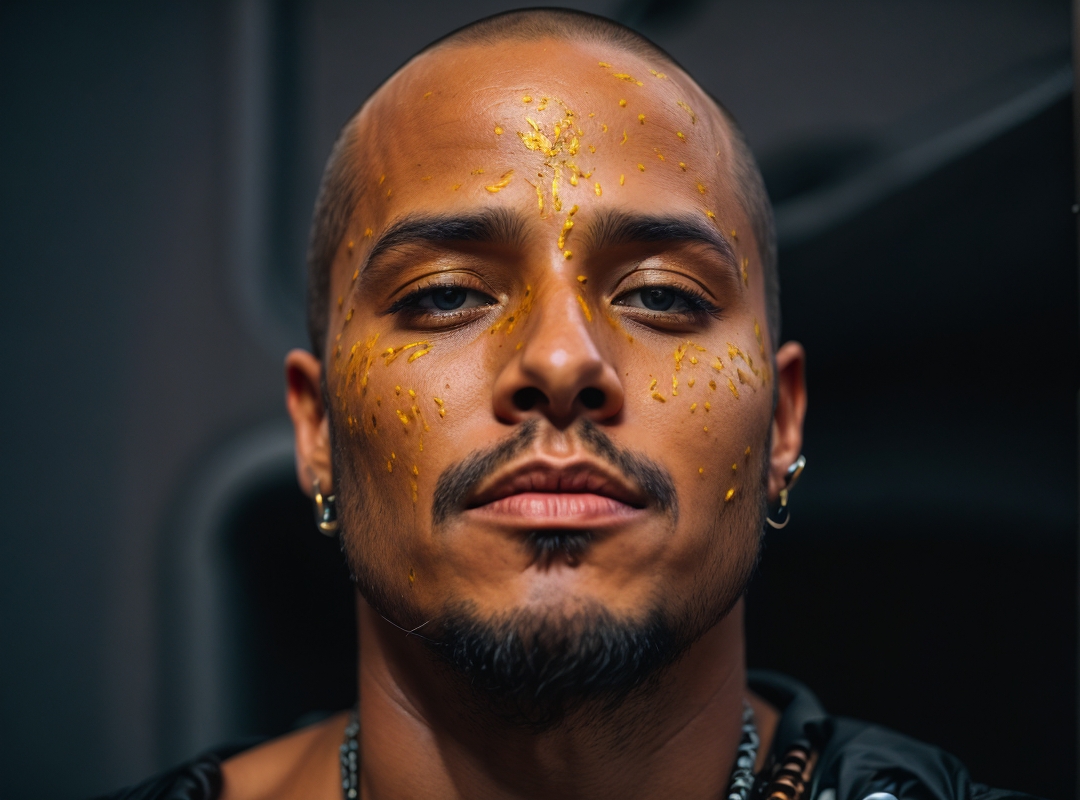
Younis Ahmed
Younis Ahmed
Hey there! 👋 Developer by day, Graphic Designer by night, and Software Engineer Student in between. 🌟 Join me on this journey as I explore the world of coding, design, and everything tech-related. 🖥️✨ #DeveloperLife #GraphicDesignPassion #TechJourney