Getting Started with Selenium WebDriver Automation in Node.js

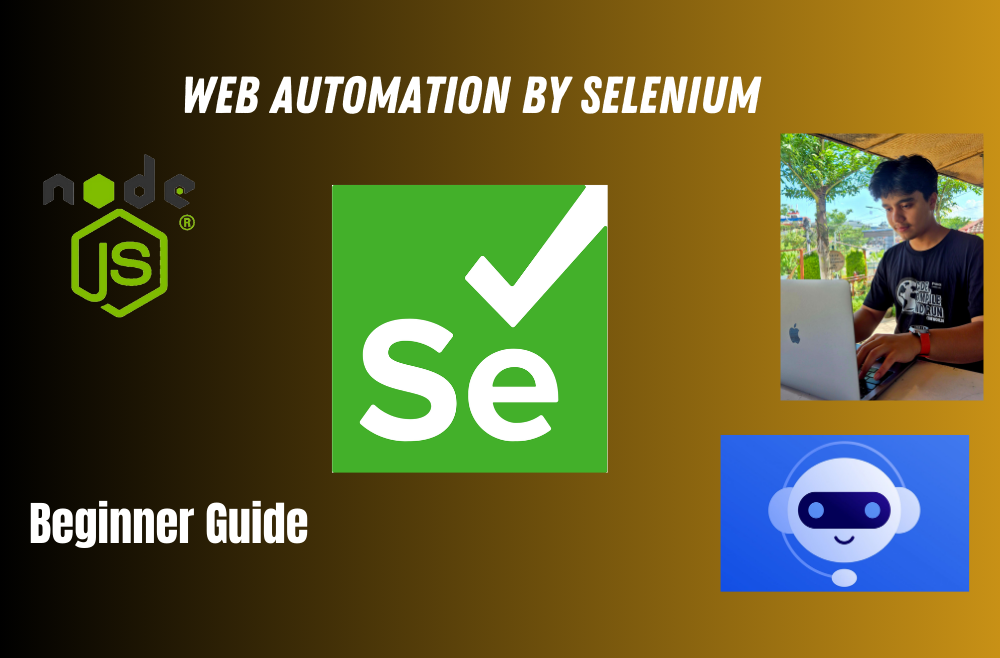
Selenium WebDriver is a powerful tool for automating web browser interactions, and when combined with Node.js, it provides a robust framework for web automation. In this guide, we'll walk through setting up and using Selenium WebDriver with Node.js to create automated browser tests.
Prerequisites
Before we begin, make sure you have:
Node.js installed on your system
A code editor (like VS Code)
Chrome browser installed
Basic knowledge of JavaScript
Setting Up Your Project
First, create a new directory for your project and initialize it:
mkdir selenium-automation
cd selenium-automation
npm init -y
Install the required dependencies:
npm install selenium-webdriver chromedriver
Writing Your First Automation Script
Here's a complete example that demonstrates how to automate a Google search:
const { Builder, By, Key, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
async function runAutomation() {
// Create a new Chrome driver instance
const driver = await new Builder()
.forBrowser('chrome')
.build();
try {
// Navigate to Google
await driver.get('https://www.google.com');
// Find the search box and type a query
const searchBox = await driver.findElement(By.name('q'));
await searchBox.sendKeys('Selenium WebDriver', Key.RETURN);
// Wait for search results to load
await driver.wait(until.elementLocated(By.css('h3')), 5000);
// Get and print the title of the first result
const firstResult = await driver.findElement(By.css('h3'));
console.log('First result:', await firstResult.getText());
} finally {
// Always close the browser
await driver.quit();
}
}
runAutomation().catch(console.error);
Key Concepts Explained
1) WebDriver Setup
The Builder()
class creates a new WebDriver instance. You can configure various options like browser type, window size, and more.
2. Locating Elements
Selenium provides multiple ways to locate elements:
By.id
()
: Find element by IDBy.name
()
: Find element by name attributeBy.css()
: Find element using CSS selectorsBy.xpath()
: Find element using XPathBy.className()
: Find element by class name
3. Waiting Strategies
Always implement proper waits in your automation:
// Explicit wait
await driver.wait(until.elementLocated(By.id('myElement')), 5000);
// Implicit wait
await driver.manage().setTimeouts({ implicit: 5000 });
4. Common Actions
// Click an element
await element.click();
// Type text
await element.sendKeys('Hello World');
// Clear input field
await element.clear();
// Get text content
const text = await element.getText();
// Check if element is displayed
const isVisible = await element.isDisplayed();
Best Practices
Always Use Try-Finally Blocks Ensure your browser sessions are properly closed even if errors occur.
Implement Proper Waits Don't rely on fixed timeouts with
setTimeout
. Use explicit or implicit waits.Use Page Object Model Organize your code by separating page elements and actions into classes
class LoginPage {
constructor(driver) {
this.driver = driver;
this.usernameInput = By.id('username');
this.passwordInput = By.id('password');
this.loginButton = By.css('button[type="submit"]');
}
async login(username, password) {
await this.driver.findElement(this.usernameInput).sendKeys(username);
await this.driver.findElement(this.passwordInput).sendKeys(password);
await this.driver.findElement(this.loginButton).click();
}
}
Handling Different Browsers
While Chrome is popular, you might need to support multiple browsers:
const firefox = require('selenium-webdriver/firefox');
const edge = require('selenium-webdriver/edge');
// Firefox setup
const firefoxDriver = new Builder()
.forBrowser('firefox')
.build();
// Edge setup
const edgeDriver = new Builder()
.forBrowser('MicrosoftEdge')
.build();
Error Handling
Implement robust error handling in your automation scripts:
try {
await driver.get('https://example.com');
await driver.findElement(By.id('non-existent'));
} catch (error) {
if (error.name === 'NoSuchElementError') {
console.error('Element not found:', error.message);
} else {
console.error('Unexpected error:', error);
}
} finally {
await driver.quit();
}
Conclusion
Selenium WebDriver with Node.js provides a powerful platform for web automation. Whether you're writing test scripts or automating repetitive tasks, following these patterns and best practices will help you create maintainable and reliable automation scripts.
Remember to always handle errors properly, implement appropriate waits, and structure your code well. As your automation suite grows, consider implementing a test framework like Mocha or Jest for better organization and reporting.
Happy automating!
Subscribe to my newsletter
Read articles from Pranish Poudel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranish Poudel
Pranish Poudel
Full Stack Developer | React | Next | Node | Express | AWS