Day 8 Of C:basic To Advanced: arrays And Their Operatons

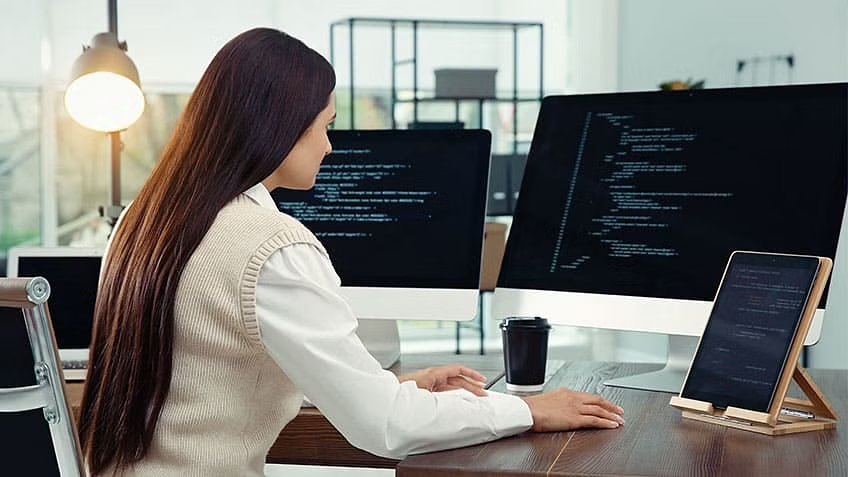
🚀 INTRODUCTION TO ARRAY
Array is basically the collection of similar data values in a single variable.
Arrays are introduced in square brackets[].
Arrays allows you to access a group of values together under a single name .
As when we define an array we have to tell the compiler that the maximum values the user can/should entered.
They store their data in a continuous memory location.
There are Types of Arrays:
Single Dimensional Array
Multidimensional Array
There are various Operations in Arrays in C:
Initilializing an Array
Accessing an Array
Defining an Array
Sorting of Array
Looping in Array
Multi Dimensional loop
🚀 Types of Arrays:
🔸SINGLE DIMENSIONAL ARRAY
Single Dimensional Array is basically a type of array that stores array in a single row form.
int numbers[5] = {1, 2, 3, 4, 5};
🔸MULTIDIMENSIONAL ARRAY
Multidimensional Array is the type of array where we can store the arrays in like Rows, Columns, etc.
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Also We can Initialize the Multidimensional Array in Another form
int numbers[3][4]={
{1,2,3,4,},
{5,6,7,8},
{9,10,11,12}
};
🚀 Operations of Array
🔸ACCESSING AN ARRAY
Each of the data items stored in an array can be accessed using various array operations.
While entering the array elements into the array the index starts from the 0 and goes upto size-1.
The array size should be declared in the square bracket next to the array name in the square bracket[].
int arr[5]={10,20,40,60,90};
/* Here the array size is 5 and elements are 5. 10 carries the index 0
and 90 carries the array index of 4*/
🔸INITIALIZING AN ARRAY
You can assign the initial values to the Arrays or take the user inputs to Enter the Values/elements of that arrays.
As also we can initiate the number of elements that should be present in that array. It can be user defined or already initiated.
If the elements in the given array is less than actual size of arrays(size-1 as first element has index 0) then the remaining elements are assigned as 0.
If the elements in the given array is more than the actal size of the arrays(index 0 to size-1) then the remaning elements are considered as the garbage elements and they are removed.
In C99 the new method was been using for initiating the elements into the arrays by enclosing a number in a pair of brackets,specific array elements can be initiated in any order.
e.g. float sample_data[500]={[2]=500..6,[1]=300.0,[0]=105.0};
#include<stdio.h>
int arr[5],int acc[5];
arr[5]={12,22,67,89,98,66};
for(int i=0;i<=5;i++)
printf("%d",arr[i]); //Limit exceeds and the element 66 goes into garbage element
acc[5]={2,3,7,9};
for(int j=0;j<=5;j++)
printf("%d",acc[j]); //Less elements are entered remaning elements are assigned value 0
🚀 ASSIGNMENTS /PROJECTS
- Addition of 1 D Matrix
// Online C compiler to run C program online
#include <stdio.h>
int sum(int a[],int n){
int sum=0,i;
for(i=0;i<n;i++)
sum=sum+a[i];
printf("The Sum of Elements is:%d\n",sum);
}
int main() {
// Write C code here
int a[10],i,n;
printf("Array SIze:\n");
scanf("%d",&n);
printf("ENter the %d Elements:\n",n);
for(i=0;i<n;i++){
scanf("%d",&a[i]);
} //displaying the array
for(i=0;i<n;i++)
printf("%d\t",a[i]);
sum(a,n);
return 0;
}
- Addition of Arrays and Finding the Transpose of the Arrays(With Matrix Form)
#include<stdio.h>
void display(int a[10][10],int m,int n)
{
int i,j;
for(i=0;i<m;i++){
for(j=0;j<n;j++)
printf("%4d",a[i][j]);
printf("\n");
}
}
void transpose(int a[10][10],int m,int n)
{
int i,j;
for(i=0;i<m;i++){
for(j=0;j<n;j++)
printf("%4d",a[j][i]);
printf("\n");
}
//}
int main()
{
int a[10][10],b[10][10],c[10][10],i,j,m,n;
printf("Enter the size of array:\n");
scanf("%d%d",&m,&n);
printf("Enter %d Elements for matrix'a':\n",m*n);
for(i=0;i<m;i++)
for(j=0;j<m;j++)
scanf("%d",&a[i][j]);
printf("Displaying Matrix 'a':\n");
display(a,m,n);
printf("Enter %d Elements for matrix'b':\n",m*n);
for(i=0;i<m;i++)
for(j=0;j<m;j++)
scanf("%d",&b[i][j]);
printf("Displaying Matrix 'b':\n");
display(b,m,n);
for(i=0;i<m;i++)
for(j=0;j<n;j++)
c[i][j]=a[i][j]+b[i][j];
printf("Displaying Matrix 'c':\n");
//printf("The Transpose of the Matrix is:\n");
//transpose(b,m,n);
display(c,m,n);
}
We will learn about further operations on arrays as we progress through the upcoming topics. ⏭️
Connect me on
Subscribe to my newsletter
Read articles from Soham Sandbhor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
