Pull-to-Refresh in iOS 18

1 min read
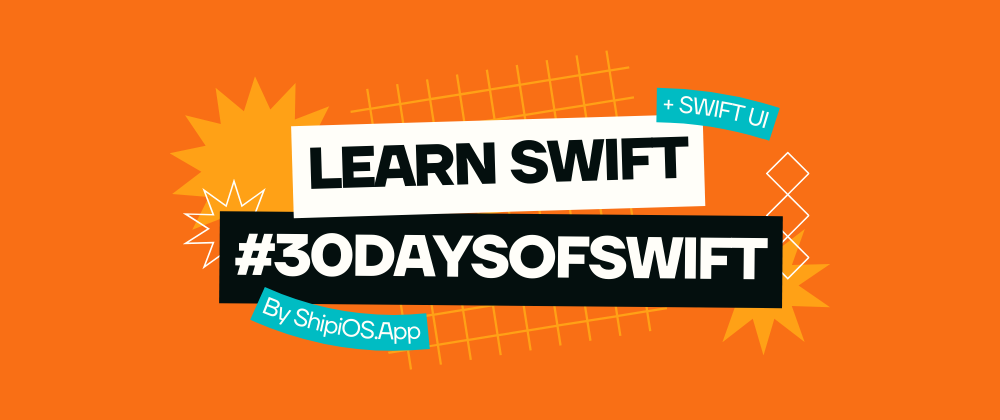
Day 20: Adding a Pull-to-Refresh Feature to Your Lists or Views ๐
Let's implement the pull-to-refresh feature in SwiftUI, a common gesture that enhances user experience by allowing users to refresh content easily.
Implementing Pull-to-Refresh
To add a pull-to-refresh feature, we can use the .refreshable
modifier available in SwiftUI.
Code Example
import SwiftUI
struct ContentView: View {
@State private var items = ["Item 1", "Item 2", "Item 3"] // Sample data
@State private var isLoading = false // State to manage loading state
var body: some View {
NavigationView {
List(items, id: \.self) { item in
Text(item) // Display items in the list
}
.navigationTitle("Pull to Refresh")
.refreshable {
await loadData() // Call the async function to load data
}
}
}
func loadData() async {
isLoading = true // Set loading state
// Simulate network delay
try? await Task.sleep(nanoseconds: 2 * 1_000_000_000) // Wait for 2 seconds
// Update items with new data
items.append("Item \(items.count + 1)") // Add a new item for demonstration
isLoading = false // Reset loading state
}
}
@main
struct PullToRefreshApp: App {
var body: some Scene {
WindowGroup {
ContentView() // Main content view
}
}
}
The full series is available on my profile and the components can also be found at shipios.app/components.
Happy Coding! ๐
0
Subscribe to my newsletter
Read articles from Vaibhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vaibhav
Vaibhav
From product vision to pixel-perfect execution, I'm a seasoned Product Manager turned iOS App Developer, leveraging Swift and SwiftUI to craft intuitive, user-centric experiences.