Control the Flow: Making Decisions in JavaScript!
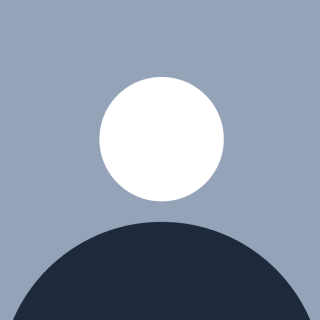
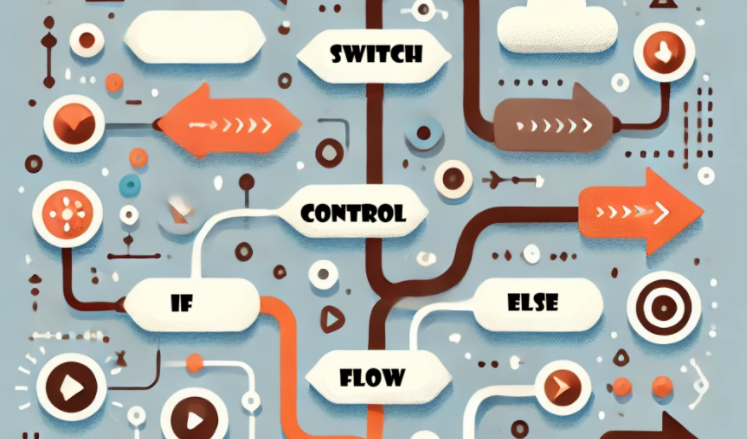
Imagine you’re the hero of an epic quest. You’ve just entered an enchanted forest filled with choices at every turn—fight or flee, left or right, friend or foe. The path you choose determines the outcome of your journey. In programming, the decisions you make are like those in your adventure: control flow lets you direct the story of your program based on conditions, guiding the execution of your code like a game.
JavaScript’s control flow helps you decide what happens next in your code, allowing it to make choices, repeat actions, or skip some entirely, just as you would decide in your adventure. Ready? Let’s start with how your choices determine the path ahead.
Your First Quest: Understanding Control Flow
Control flow is the order in which statements are executed in a program. Without control flow, a program would simply run from the top to the bottom, executing every single line without any decision-making. But what happens when you need to make decisions?
In your adventure game, imagine you encounter a fork in the road:
Go left, and you might find treasure.
Go right, and there could be danger.
In JavaScript, control flow helps you make these decisions. It checks conditions and takes different paths based on what it finds.
Let’s break down the two key tools for decision-making: if-else and switch statements.
The Fork in the Road: Conditional Statements
The Basic If-Else Structure
When your hero reaches the fork in the road, how do they decide which way to go? You might ask a question like, "Is the path to the left clear?" If it is, they’ll go left; otherwise, they’ll go right. In JavaScript, this logic is captured with if-else statements.
Basic If-Else Syntax:
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
Let’s bring this to life with an example:
Code Snippet: If-Else Example
let path = "clear";
if (path === "clear") {
console.log("You take the left path toward the treasure!");
} else {
console.log("The left path is blocked, so you head right!");
}
Here, the code checks if the condition path === "clear"
is true. If it is, you take the left path. If not, you are forced to go right.
Decision-Making Flowchart
To visualize this, let’s create a flowchart that outlines the decision-making process in your quest:
[ Encounter Fork in the Road ]
|
V
[ Is the left path clear? ]
/ \
Yes No
| \
[ Take left ] [ Take right ]
| |
[ Find treasure! ] [ Face danger! ]
This flowchart illustrates how the program checks the condition and makes a decision based on whether the path is clear.
Going Deeper: Else If for Multiple Conditions
Sometimes, there are more than two choices—what if there’s also a hidden third option? Let’s extend our example to include multiple conditions with else if.
Code Snippet: Else If Structure
let path = "overgrown";
if (path === "clear") {
console.log("You take the left path toward the treasure!");
} else if (path === "overgrown") {
console.log("You cut through the bushes on the middle path!");
} else {
console.log("The left path is blocked, so you head right!");
}
Here, if the first condition (the path being clear) is false, we check another condition (the path being overgrown). This allows for multiple possible outcomes, much like discovering hidden paths in your adventure.
The Bigger Picture: Switch Statements
Now imagine the adventure grows more complex. You might find yourself facing more than two or three options at once. In JavaScript, switch statements handle scenarios where you want to check multiple possibilities against the same expression.
Think of a switch statement like a branching decision tree. Instead of checking one condition at a time, it compares a single value against multiple cases and runs the block of code that matches.
Code Snippet: Using Switch
Let’s revisit our adventure. This time, instead of checking individual conditions, the switch statement compares your path against several predefined options:
let path = "cave";
switch (path) {
case "forest":
console.log("You venture deep into the forest, finding hidden treasures.");
break;
case "mountain":
console.log("You climb the mountain and face fierce winds.");
break;
case "cave":
console.log("You explore a dark cave and discover an ancient artifact.");
break;
default:
console.log("You wander aimlessly with no destination.");
}
This switch structure allows you to easily add multiple choices without needing multiple else if statements. If none of the cases match, the default
case runs, representing an unexpected outcome—just like stumbling upon an unknown area in your adventure.
Multi-Path Adventure with Switch Statements
[ Choose Your Path ]
|
V
[ Check Path ]
/ | \
Forest Mountain Cave
| | |
[ Find treasure ] [ Face winds ] [ Discover artifact ]
\ | /
[ Continue journey ]
The switch statement elegantly handles multiple paths, simplifying the decision-making logic in scenarios with numerous possible outcomes.
Challenge Time! - Create Your Own Adventure Quiz
Now that you’ve learned how to guide your hero through decisions using control flow, it’s time for you to build your own adventure! For this challenge, you'll create a simple, text-based adventure game using if-else and switch statements to guide the player through different scenarios.
Here’s the structure to help you get started:
Step 1: Set up the game with a scenario where the player must make decisions.
Step 2: Use if-else or switch statements to handle the player's choices.
Step 3: Provide feedback based on the decisions they make.
Example Structure:
let weapon = prompt("You encounter a wild beast! Choose your weapon: sword, bow, or magic.");
if (weapon === "sword") {
console.log("You bravely fight the beast with your sword and win!");
} else if (weapon === "bow") {
console.log("You shoot an arrow from a distance and defeat the beast!");
} else if (weapon === "magic") {
console.log("You cast a powerful spell and vanquish the beast!");
} else {
console.log("Without a proper weapon, you flee the scene.");
}
let path = prompt("You come to a fork in the road. Do you go left or right?");
switch (path) {
case "left":
console.log("You find a peaceful village.");
break;
case "right":
console.log("You are ambushed by bandits!");
break;
default:
console.log("You wander off the path and get lost.");
}
Challenge Explanation:
This simple adventure quiz combines both if-else and switch statements to create a branching narrative. Depending on the player’s input, different outcomes occur. You can expand the adventure with more decisions and deeper storylines as you improve your control flow skills.
Expanding Your Adventure: Adding Complexity
Let’s take it up a notch. Add more layers to the adventure by incorporating nested conditions and logical operators. For instance, imagine a scenario where the player must have both a key and a torch to open a hidden door. This requires a more complex conditional check using the && operator.
Example with Logical Operators:
let hasKey = true;
let hasTorch = false;
if (hasKey && hasTorch) {
console.log("You open the hidden door and enter the secret chamber!");
} else if (hasKey && !hasTorch) {
console.log("You have the key, but it's too dark to find the door.");
} else {
console.log("You can't open the door without the key.");
}
This approach introduces more dynamic outcomes, making your game feel more engaging, where players must meet certain conditions to advance.
The Power of Decisions in Programming
Just like in an adventure game, making decisions in JavaScript defines the flow and outcome of your program. Control flow is the mechanism that allows you to shape your code, giving it flexibility and direction. Whether it's guiding your hero through treacherous forests, deciding when to fight or flee, or determining which path to take, control flow in JavaScript helps you create dynamic, interactive experiences.
By mastering control flow structures like if-else and switch statements, you gain the ability to make your programs responsive and adaptable. This foundational skill will empower you to tackle more complex challenges in your coding journey.
Now that you’ve mastered control flow, it's time to explore another vital part of your coding adventure: loops! Just as you might face repeated challenges in your journey, loops allow you to repeat actions in your code until you achieve a desired result.
In the next article, we’ll dive into loops—the structures that let you run the same block of code multiple times, iterating through data and automating repetitive tasks. Get ready for the next exciting chapter in your JavaScript journey!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by