Introduction to DNNS

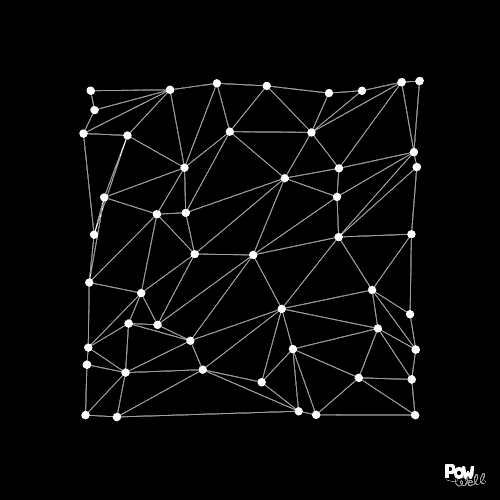
Artificial Neural Networks (ANNs): ANNs are computational models inspired by the human brain. They consist of interconnected nodes or 'neurons' that process information and make decisions. ANNs are used in various applications, from image recognition to natural language processing.
Deep Neural Networks (DNNs): DNNs are a type of ANN with multiple layers between the input and output layers. These additional layers allow DNNs to model complex patterns in data.
Building Blocks of Neural Networks
Neurons and Layers: Neurons are the basic units of a neural network, organized into layers. The input layer receives data, hidden layers process it, and the output layer produces the result.
Activation Functions: These functions determine the output of a neuron. Common activation functions include ReLU, sigmoid, and tanh.
Setting Up Your Environment
To start building neural networks, you'll need Python and libraries like TensorFlow or Keras. Here's how to set up your environment:
!pip install tensorflow
!pip install numpy
!pip install matplotlib
Building an ANN with TensorFlow/Keras
Here's a simple example of building an ANN using TensorFlow/Keras:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Define the model
model = Sequential([
Dense(64, activation='relu', input_shape=(input_size,)),
Dense(32, activation='relu'),
Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(x_train, y_train, epochs=15, batch_size=32, validation_data=(x_test, y_test))
# Evaluate the model
loss, accuracy = model.evaluate(x_test, y_test)
print("Loss:", loss)
print("Accuracy:", accuracy)
Practical Applications
Image Recognition: Convolutional Neural Networks (CNNs), a type of ANN, are widely used for tasks like object detection and facial recognition.
Natural Language Processing (NLP): ANNs are used in NLP tasks such as sentiment analysis and chatbots.
Financial Forecasting: ANNs can predict stock prices and detect fraud by learning from historical data.
Resources for Further Learning
Deep Learning with TensorFlow/Keras Guide: A comprehensive guide with code snippets for various neural network architectures.
ANN in Python from Scratch: A detailed guide to building ANNs in Python, perfect for beginners.
Conclusion
Neural networks are powerful tools for solving complex problems. By understanding their structure and functionality, you can apply them to a wide range of applications. Keep experimenting and exploring to deepen your understanding and skills in this exciting field.
Subscribe to my newsletter
Read articles from Sujit Nirmal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sujit Nirmal
Sujit Nirmal
๐ Hi there! I'm Sujit Nirmal, a AI /ML Developer with a passion for creating intelligent, seamless ML applications. With a strong foundation in both machine learning and Deep Learning I thrive at the intersection of data and technology.