The React Lifecycle: Understanding How Pre-Built Tools Can Save Time When Implementing Methods, Hooks and Interactions
The React lifecycle defines how a component behaves at different stages of development— specifically when a code is created, modified, updated, and eventually removed from the Document Object Model (DOM).
By understanding the React lifecycle, developers can customize their JavaScript to better handle complex behaviors within the user interface (UI), and tailor their apps with greater flexibility. It also allows them to create and debug UI test cases before going live to the public.
To achieve this, developers will use different lifecycle methods to manage specific functions within class components during different phases of the component lifecycle. They’ll use hooks, on the other hand, to manage and execute function components for similar lifecycle management and state management capabilities.
And by understanding how class components and hooks interact with function components, developers will be in a much better position to control how their app reacts to changes and performs over time.
Understanding React lifecycle methods
The React lifecycle is defined by how effectively a component moves through each of the four primary phases of the React lifecycle—from the onset of its creation to the ultimate destruction of each component in the UI.
The React lifecycle has four different phases, often categorized as:
Initialization
The initialization phase of the React lifecycle is where the component is constructed and set up for the very first time. The initial state of the component is defined, and its props are assigned before mounting can take place. Class components are most often defined using this.state, while function components are assigned a useState. This phase isn't always necessary, and therefore, some consider it an optional phase within the React lifecycle.
- 💡If initialization is considered unnecessary, there's no need to include a constructor. This method initializes the state of the component and binds event-handler methods within the component, itself, usually before rendering.
Mounting
Mounting is when the life of a component actually begins. This phase handles setup tasks like API calls or initial state configuration. And because a component can only be created once, this is often called the “initial render.
Once a new component has been created, it can be inserted into the DOM during the mounting phase using each of the four key lifecycle methods:
constructor static getDerivedStateProps render() componentDidMount()
💡Methods, such as the componentDidMount, allow you to add side effects like sending network requests or updating the component's state within the UI. If you’re not careful with these methods, however, you may be faced with unnecessary re-renders that can negatively impact the performance of the UI.
Updating
Should a state or prop be changed, components will automatically be updated, and re-rendering will occur. These changes often take place due to a user-related event and are triggered when either the props or state is updated. Typing, clicking, and dragging the mouse are considered user events, and each action will trigger certain components to update.
💡Developers can optimize component behavior and improve the performance of the UI by minimizing unnecessary re-renders and managing their resources more efficiently.
Common methods include:
shouldComponentUpdate() getSnapshotBeforeUpdate() componentDidUpdate() render() getDerivedStateFromProps setState() Function
This phase can occur multiple times throughout the React lifecycle and can come in very handy when new data is available.
Unmounting
To invoke the last phase within a component's lifecycle, the componentWillUnmount() method will call the component to finally be unmounted and removed from the DOM. It’s also used to clean up resources, such as event listeners that have been put into place or network requests that may have been placed, as well as the function of any useEffect() hooks that may still exist within the code.
Lifecycle Methods are gradually being replaced by hooks.
Lifecycle methods are gradually being replaced by hooks, however. Hooks provide similar capabilities to lifecycle methods but are exclusive to function components, whereas class components rely on lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount to manage their behavior throughout the React lifecycle.
Hooks, for example, will allow function components to handle lifecycle events, like useEffect() for componentDidMount or componentWillUnmount. They were introduced to address limitations in class components, making it easier for developers to write and maintain cleaner, reusable code.
They create cleaner and more reusable code.
Hooks eliminate the need for complex class syntax by developers, making code easier to read and maintain. Hooks also let developers separate concerns by placing related code together. For instance, useEffect() can handle side effects that would otherwise be scattered across multiple lifecycle methods.
They’re more functional and modular than most lifecycle methods.
Hooks allow function components to use both states and side effects, which, in turn, allow developers to take a more functional programming approach toward avoiding confusing “this” bindings in classes and rely, instead, on simple, flexible functions.
Functional components using hooks tend to be more reusable and adaptable, thereby allowing organizations to scale their applications and reduce the complexity of maintaining larger codebases — especially for small startups with limited manpower. This functional design also opens the door to more effective testing and debugging practices.
Performance is both optimized and improved.
Hooks like useMemo() and useCallback() help developers by preventing unnecessary re-renders and optimize performance, especially in complex UIs, by reducing unnecessary calculations and preventing redundant function creation.
This means developers can build faster applications with fewer resources. And better performance directly impacts both the user experience and resource utilization, leading to cost savings and potentially higher customer satisfaction overall.
Custom hooks mean simplified component logic.
Custom hooks let developers reuse logic across different components without duplicating code, which is more challenging with class components. This promotes code reuse and improves maintainability. They also allow developers to isolate and reuse side effect logic across multiple components — something not as easily achieved with lifecycle methods in class components.
By reusing hooks, developers experience a reduced need for rewriting complex logic, not only saving time in both development and bug fixing, but achieving a more efficient use of developer resources and getting more done on a smaller budget.
How lifecycle methods and hooks interact to manage the flow of React components
Both lifecycle methods and hooks should be used together for smoother React lifecycle management. Both approaches manage side effects and allow for fine-grained control over how components interact with the DOM and update over time. Hooks, however, offer a more modern, flexible alternative to the lifecycle methods. The flow of components and their behaviors are best defined and optimized as they transition between each phase of the React lifecycle process.
By understanding how each phase of the React lifecycle interacts with hooks during the development of function components and UI behavior, developers can more effectively optimize class components, manage clean-up, and improve resource utilization.
Hooks in action
In today’s React environment, hooks like useEffect() are used to manage lifecycle events. They are associated with behavior and require rendering to function. In fact, they simplify lifecycle management by executing additional effects after the initial render or when specific dependencies change, providing developers with a more straightforward path toward handling these interactions—especially in function components that do not have access to class-based lifecycle methods.
While hooks align with functional programming concepts, they are used to create a simpler, more efficient code. Early, we addressed how hooks like useMemo and useCallback help minimize redundant re-renders, improving both performance and memory usage in complex applications.
An example of this is when useEffect() is used to run code upon first rendering of a component (similar to componentDidMount) or when it is removed (similar to componentWillUnmount) altogether. Similarly, memoization techniques (like React.memo and useMemo) can reduce unnecessary renders, which are crucial for optimizing performance in complex applications.
During updates, Concurrent Mode, an experimental feature in React (using features like useTransition), allows developers to manage asynchronous updates more efficiently and with fewer errors in the process. By deferring non-urgent updates, asynchronous interactions will feel more responsive and user-friendly without the compromise of other processes.
Error handling during lifecycle development
A key mechanism for implementing error boundaries is the componentDidCatch() lifecycle method. React 16 introduced a powerful concept known as error boundaries, which is capable of handling even the most sophisticated errors within React applications and components during the various phases of rendering, lifecycle methods, and constructors. This can be used to catch and log errors and can be quite valuable when managing unexpected issues in the React lifecycle.
Specifically, error boundaries are designed to catch and manage errors that occur during the rendering process, within lifecycle methods, and even within the constructors of components.
By implementing componentDidCatch(), developers gain the ability to:
Log Errors: Capture and record error details for later analysis and debugging purposes. This can be invaluable for identifying and resolving issues that may not be immediately apparent.
Display Fallback UI: Prevent the entire application from crashing by rendering a user-friendly fallback UI within the error boundary. This fallback UI can inform users about the error in a controlled manner and potentially offer options for recovery.
Prevent Cascading Errors: Isolate the problematic component and prevent the error from propagating to other parts of the application. This helps to maintain the overall stability and functionality of the application.
Incorporating error boundaries into React applications is highly recommended practice for this reason — especially when managing unexpected issues and improving the overall user experience.
It’s worth noting that hooks do not currently support error boundaries, and only class components can use componentDidCatch(). Although they can wrap around function components, error boundaries are only available in class components. There are workarounds, however, when using the ErrorBoundary component to wrap function components, if and when necessary for the developer to do so.
Simplify complex React component life cycles with pre-built tools.
Pre-built tools can save developers thousands of clock hours by simplifying the development process on major projects and applications. In fact, they tend to optimize lifecycle management processes, allowing developers the freedom to focus on more of the dynamic features they build, rather than the headaches of managing each component manually.
Through pre-built components, developers can get a better grip on their lifecycle management for faster development and more optimized platform optimization, especially when working with a large number of components, projects, and applications—and still be able to implement custom logic using JavaScript to manage complex UI behaviors as necessary.
Why is this important?
It’s important for developers to understand the React lifecycle when effectively managing component behavior and optimizing the user interface (UI) for performance. By leveraging lifecycle methods and hooks, developers can control how components are initialized, mounted, updated, and unmounted.
By utilizing pre-built tools, they can further simplify the development process and ultimately focus on building a portfolio of glitch-free and dynamic features their customers will love and reputable tools their organizations will not only profit from but lose fewer resources when creating.
Subscribe to my newsletter
Read articles from Jessica N. Abraham | writer. designer. publicist. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
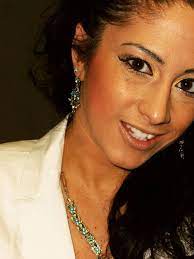
Jessica N. Abraham | writer. designer. publicist.
Jessica N. Abraham | writer. designer. publicist.
ABOUT JESSICA N. ABRAHAM: Jessica N. Abraham is a writer, designer and publicist, who has spent almost 20 years dedicated to the worlds of Social Branding, Public Relations and Digital Marketing Strategy. As a writer, she specializes in Business, Technology and the Jobs Industry and has written for a number of online platforms on global news, technology and thought leadership, often writing about topics, relating to the corporate arena, connected technologies, electrification, team-building and globalization. Jessica has written for sites like Benzinga, Benzinga Money, NewsBreak, The Muse, TMC Net, CBS Local, PeopleKeys, TalentZoo, Digital Pivot, Yahoo! Contributor, The Associated Content, RecipeStation, AXS, Examiner and more! Her work has also been featured on Yahoo! Finance, TD Ameritrade, Etrade, Robinhood, Interactive Brokers, Tradestation, Questrade, JP Morgan Chase, Webull, Business Insider, MarketInsider, MSN Money, MarketWatch, Smartnews, Gate.io, Axi, Fidelity, Trading View, Investor Place, FX Street, Seeking Alpha and the Stansberry Terminal.