Demystifying Neural Networks: A Beginner's Guide to Building Your First Model

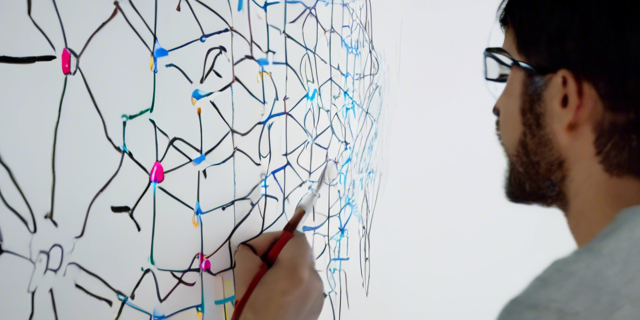
Neural networks have become a cornerstone of modern artificial intelligence, powering applications ranging from image recognition to natural language processing. If you're an aspiring AI enthusiast, this guide will help you understand neural networks and guide you through building your first model using TensorFlow.
What is a Neural Network?
A neural network is a computational model inspired by the way biological neural networks in the human brain work. It consists of layers of interconnected nodes (neurons), where each connection has an associated weight. Neural networks learn from data through a process called training, where they adjust these weights to minimize the error in their predictions.
Key Components of a Neural Network
Neurons: The basic units of a neural network that process input data and generate output.
Layers:
Input Layer: The first layer that receives the input data.
Hidden Layers: Intermediate layers that perform transformations on the input data.
Output Layer: The final layer that produces the output.
Activation Functions: Functions applied to the output of each neuron, introducing non-linearity. Common activation functions include ReLU (Rectified Linear Unit), sigmoid, and softmax.
Loss Function: A function that measures how well the neural network's predictions match the actual target values. Common loss functions include mean squared error for regression tasks and categorical cross-entropy for classification tasks.
Optimizer: An algorithm that adjusts the weights of the network to minimize the loss function. Popular optimizers include Stochastic Gradient Descent (SGD), Adam, and RMSprop.
Step-by-Step Guide to Building Your First Neural Network
Step 1: Set Up Your Environment
To start building your neural network, you'll need to install Python and the necessary libraries. Use the following command to install TensorFlow:
pip install tensorflow
Step 2: Import Required Libraries
Start by importing the necessary libraries:
import tensorflow as tf
from tensorflow.keras import layers, models
import numpy as np
import matplotlib.pyplot as plt
Step 3: Prepare the Dataset
For this example, we’ll use the famous MNIST dataset, which consists of handwritten digits (0-9). TensorFlow provides easy access to this dataset:
# Load the MNIST dataset
(train_images, train_labels), (test_images, test_labels) = tf.keras.datasets.mnist.load_data()
# Normalize the images to a range of 0 to 1
train_images = train_images.astype('float32') / 255
test_images = test_images.astype('float32') / 255
# Reshape the images to include a channel dimension (28x28 pixels with 1 color channel)
train_images = np.expand_dims(train_images, axis=-1)
test_images = np.expand_dims(test_images, axis=-1)
Step 4: Build the Neural Network Model
Next, create a simple feedforward neural network model using Keras:
# Build the model
model = models.Sequential([
layers.Flatten(input_shape=(28, 28, 1)), # Flatten the input
layers.Dense(128, activation='relu'), # Hidden layer with 128 neurons
layers.Dense(10, activation='softmax') # Output layer with 10 neurons for classification
])
Step 5: Compile the Model
Before training the model, you need to compile it by specifying the loss function, optimizer, and metrics:
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy', # Suitable for integer labels
metrics=['accuracy'])
Step 6: Train the Model
Now, train the model using the training dataset:
history = model.fit(train_images, train_labels, epochs=5, batch_size=32, validation_split=0.2)
Step 7: Evaluate the Model
After training, evaluate the model's performance on the test dataset:
test_loss, test_acc = model.evaluate(test_images, test_labels)
print(f'Test accuracy: {test_acc:.4f}')
Step 8: Visualize Training Progress
To understand how well your model has learned, visualize the training and validation accuracy:
# Plot training & validation accuracy and loss values
plt.figure(figsize=(12, 5))
# Accuracy
plt.subplot(1, 2, 1)
plt.plot(history.history['accuracy'], label='Training Accuracy')
plt.plot(history.history['val_accuracy'], label='Validation Accuracy')
plt.title('Model Accuracy')
plt.ylabel('Accuracy')
plt.xlabel('Epoch')
plt.legend()
# Loss
plt.subplot(1, 2, 2)
plt.plot(history.history['loss'], label='Training Loss')
plt.plot(history.history['val_loss'], label='Validation Loss')
plt.title('Model Loss')
plt.ylabel('Loss')
plt.xlabel('Epoch')
plt.legend()
plt.show()
Practical Example: Making Predictions
Now that your model is trained, you can use it to make predictions on new data:
# Make predictions on the test set
predictions = model.predict(test_images)
# Display the first test image, its predicted label, and the true label
plt.imshow(test_images[0].reshape(28, 28), cmap='gray')
plt.title(f'Predicted: {np.argmax(predictions[0])}, True: {test_labels[0]}')
plt.axis('off')
plt.show()
Conclusion
Congratulations! You’ve built your first neural network using TensorFlow. By following these steps, you now have a foundational understanding of how neural networks work and how to implement them in Python. As you continue your journey in AI and machine learning, you can experiment with more complex architectures, datasets, and techniques.
Next Steps
Explore Different Architectures: Try using convolutional neural networks (CNNs) for image-related tasks.
Tune Hyperparameters: Experiment with different learning rates, batch sizes, and optimizers to improve performance.
Dive Deeper into Transfer Learning: Learn how to leverage pre-trained models for tasks with limited data.
Embrace the learning process, and happy coding!
Subscribe to my newsletter
Read articles from Temitope Ologunbaba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Temitope Ologunbaba
Temitope Ologunbaba
I am Temitope Ologunbaba, a software engineer with 5 years of experience specializing in developing innovative solutions and enhancing user experiences built over 30 apps on both playstore and app store, and 2 models currently being used by the companies in app for giving their user better experience. Proficient in Python, Java and Dart(Flutter). As an enthusiast of machine learning and artificial intelligence, I am passionate about leveraging technology to derive insights and improve decision-making processes. My expertise allows me to understand user needs and preferences deeply, facilitating effective collaboration with cross-functional teams to deliver diverse perspectives on projects. I am committed to continuous learning and applying my skills to drive impactful solutions in the tech industry.