Ultimate Guide to JavaScript Utility Functions
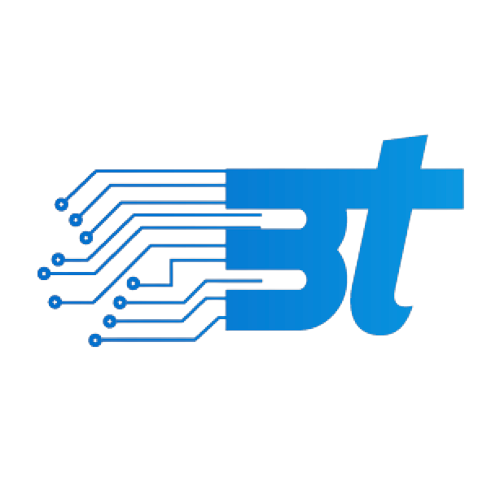
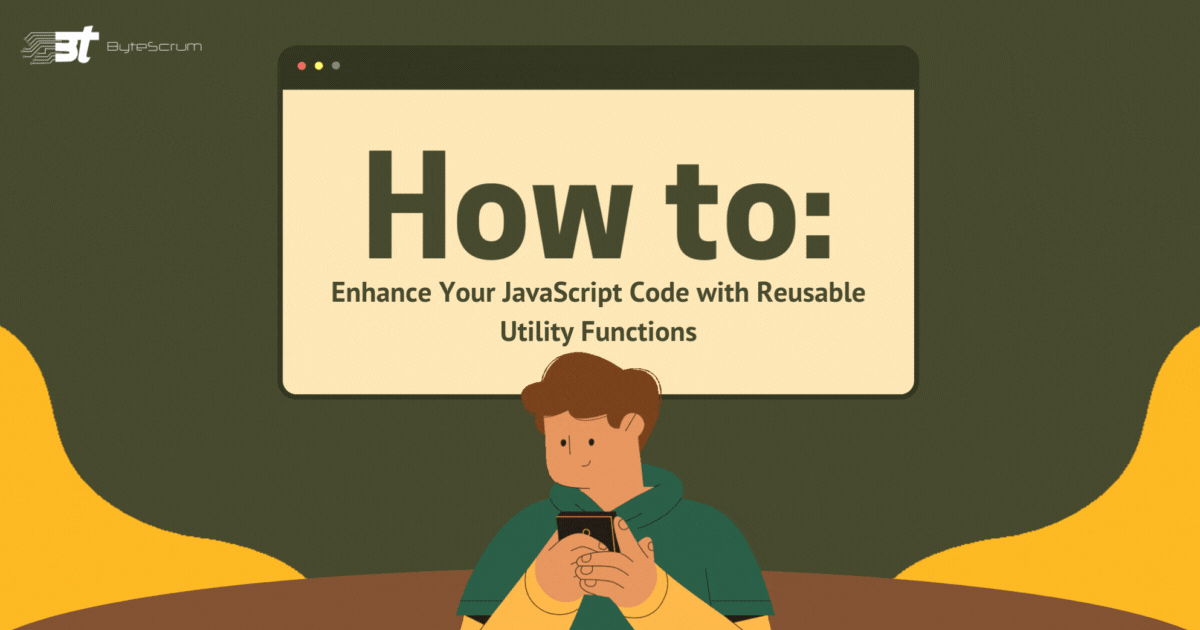
JavaScript utility functions, commonly known as utils, are reusable helper functions that simplify and optimize code. They abstract out common operations into smaller, easy-to-maintain pieces of logic, enhancing both readability and reusability. In this blog, weβll dive into:
What utility functions are
Why you need them
Common utility libraries
Essential custom utility functions with examples
Tips on organizing utils in a project
π What are Utility Functions?
Utility functions are reusable snippets of code designed to perform a specific task. Think of them as small, isolated tools that prevent you from rewriting the same code over and over again. For example, converting strings to camelCase
, deep cloning objects, or formatting dates.
These functions serve two main purposes:
Code Reusability: No need to write the same logic in multiple places.
Code Readability: Utils make your main code more readable by offloading complex logic to helper functions.
β‘ Why Use Utility Functions?
Without utility functions, your project can quickly become unmanageable. A well-structured utils module ensures:
Cleaner Code: You write once and reuse throughout your app.
Maintainability: Changes only need to happen in the utility module.
Avoid Errors: Tested utils can reduce bugs caused by code repetition.
Performance Optimizations: Utils can encapsulate more efficient algorithms.
π₯ Popular Utility Libraries in JavaScript
Many libraries offer prebuilt utility functions. Some popular ones are:
Lodash: A utility library delivering consistency, performance, and extras.
Underscore.js: Similar to Lodash but smaller.
Ramda: Functional utilities focusing on immutability and composability.
Moment.js / Day.js: Date-time utilities for parsing, formatting, and manipulation.
uuid: For generating unique IDs.
However, for many projects, you might need to write custom utility functions tailored to your specific needs.
π οΈ Custom JavaScript Utility Functions with Code Examples
Below are some essential utility functions categorized by their use case, along with code examples.
1. String Utilities
1.1. capitalize()
Capitalizes the first letter of a string.
function capitalize(str) {
if (typeof str !== 'string') return '';
return str.charAt(0).toUpperCase() + str.slice(1);
}
console.log(capitalize('hello')); // "Hello"
1.2. camelCase()
Converts a string to camelCase.
function camelCase(str) {
return str
.toLowerCase()
.replace(/[-_ ]+(\w)/g, (_, char) => char.toUpperCase());
}
console.log(camelCase('hello_world')); // "helloWorld"
2. Array Utilities
2.1. unique()
Removes duplicate elements from an array.
function unique(arr) {
return [...new Set(arr)];
}
console.log(unique([1, 2, 2, 3, 4, 4])); // [1, 2, 3, 4]
2.2. chunk()
Splits an array into smaller chunks.
function chunk(arr, size) {
const result = [];
for (let i = 0; i < arr.length; i += size) {
result.push(arr.slice(i, i + size));
}
return result;
}
console.log(chunk([1, 2, 3, 4, 5, 6], 2)); // [[1, 2], [3, 4], [5, 6]]
3. Object Utilities
3.1. deepClone()
Performs a deep clone of an object.
function deepClone(obj) {
return JSON.parse(JSON.stringify(obj));
}
const original = { a: 1, b: { c: 2 } };
const clone = deepClone(original);
console.log(clone); // { a: 1, b: { c: 2 } }
3.2. mergeObjects()
Merges two objects deeply.
function mergeObjects(obj1, obj2) {
return { ...obj1, ...obj2 };
}
console.log(mergeObjects({ a: 1 }, { b: 2 })); // { a: 1, b: 2 }
4. Date Utilities
4.1. formatDate()
Formats a date to YYYY-MM-DD
.
function formatDate(date) {
return date.toISOString().split('T')[0];
}
console.log(formatDate(new Date())); // "2024-10-30"
4.2. timeAgo()
Converts a date to a "time ago" format.
function timeAgo(date) {
const seconds = Math.floor((new Date() - date) / 1000);
const intervals = {
year: 31536000,
month: 2592000,
week: 604800,
day: 86400,
hour: 3600,
minute: 60,
};
for (const [unit, value] of Object.entries(intervals)) {
const interval = Math.floor(seconds / value);
if (interval > 1) return `${interval} ${unit}s ago`;
}
return 'Just now';
}
console.log(timeAgo(new Date(Date.now() - 60000))); // "1 minute ago"
5. Number Utilities
5.1. randomInt()
Generates a random integer between two values.
function randomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
console.log(randomInt(1, 10)); // Random integer between 1 and 10
5.2. formatCurrency()
Formats a number as currency.
function formatCurrency(num, locale = 'en-US', currency = 'USD') {
return new Intl.NumberFormat(locale, { style: 'currency', currency }).format(num);
}
console.log(formatCurrency(1234.56)); // "$1,234.56"
6. Utility for Promises and Async Operations
6.1. sleep()
Delays the execution for a given amount of time.
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function demo() {
console.log('Waiting...');
await sleep(2000);
console.log('Done!');
}
demo(); // Logs "Waiting..." then "Done!" after 2 seconds
6.2. retry()
Retries an async function multiple times if it fails.
async function retry(fn, retries = 3) {
let lastError;
for (let i = 0; i < retries; i++) {
try {
return await fn();
} catch (error) {
lastError = error;
}
}
throw lastError;
}
async function fetchData() {
// Simulate API call
throw new Error('Network Error');
}
retry(fetchData).catch(console.error); // "Network Error"
π How to Organize Utility Functions in Your Project?
Itβs essential to keep your utility functions well-organized. Here are a few tips:
Group by Type: Separate utils by type:
stringUtils.js
,arrayUtils.js
, etc.Use a
utils
Folder: Create autils/
folder and keep your helpers inside.Export Functions: Export individual utils and create an index to simplify imports.
Example folder structure:
/utils
βββ stringUtils.js
βββ arrayUtils.js
βββ dateUtils.js
βββ index.js
In index.js
:
export * from './stringUtils';
export * from './arrayUtils';
export * from './dateUtils';
Conclusion
Take the examples from this guide, start building your own utility library, and see how it transforms your projects!
Happy coding! π
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
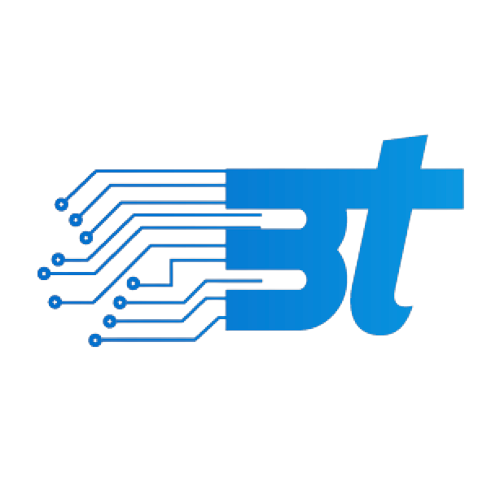
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.