Automating Application Deployment: A Complete CI/CD Pipeline with Jenkins, GitHub, Maven, SonarQube, Nexus, and Tomcat

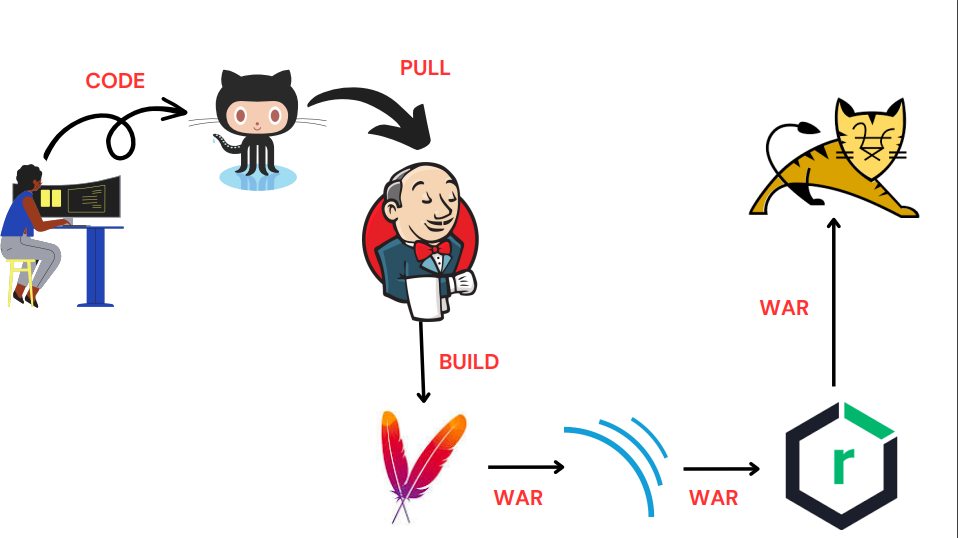
Deploying Application
In today’s fast-paced development environment, automation is essential to ensure quick releases with consistent quality. In this blog, I’ll walk you through the end-to-end CI/CD pipeline for deploying an application. We’ll cover code integration, building the application, ensuring code quality, managing artifacts, and deploying on Tomcat—all fully automated through Jenkins.
What Will We Cover?
Source Code Integration with GitHub
Build Automation using Maven
Code Quality Analysis with SonarQube
Artifact Management with Nexus
Application Deployment to Tomcat
Let's dive into the detailed workflow!
1️⃣ Source Code Management with GitHub
The process starts with version control. Developers push code to a GitHub repository that Jenkins fetches during the pipeline execution. GitHub enables effective collaboration while tracking code changes across branches.
Jenkins Pipeline Snippet:
groovyCopy codestage("Code") {
git "https://github.com/rohit23106/one.git"
}
2️⃣ Build Automation using Maven
In the next stage, Maven is used to build and package the application. Maven ensures all dependencies are in place and compiles the source code into a deployable WAR file.
Jenkins Pipeline Snippet:
groovyCopy codestage("Build") {
sh "mvn clean package"
}
Outcome:
- WAR file is created at
target/myweb-8.6.7.war
.
3️⃣ Code Quality Analysis with SonarQube
Automated code quality analysis is critical. We use SonarQube to analyze the codebase for bugs, vulnerabilities, and code smells, ensuring only high-quality code gets deployed.
Jenkins Pipeline Snippet:
groovyCopy codestage("CQA") {
withSonarQubeEnv(credentialsID: 'sonar-password') {
def mavenHome = tool name: "maven", type: "maven"
sh "${mavenHome}/bin/mvn sonar:sonar"
}
}
Result:
- SonarQube Dashboard: Displays quality metrics and issues, helping developers make improvements before release.
4️⃣ Artifact Management with Nexus Repository
Once the build is complete, the artifact (WAR) is uploaded to Nexus Repository Manager. Nexus helps manage versions of artifacts, making them available for future deployments.
Jenkins Pipeline Snippet:
groovyCopy codestage('Artifact') {
nexusArtifactUploader artifacts: [[artifactId: 'myweb',
classifier: '',
file: 'target/myweb-8.6.7.war',
type: 'war']],
credentialsId: 'nexus',
groupId: 'in.javahome',
nexusUrl: 'http://52.73.214.233:8081',
repository: 'rohitrepo',
version: '8.6.7'
}
5️⃣ Deployment on Tomcat Server
The final step involves deploying the packaged WAR file on a Tomcat server using Jenkins. Jenkins manages the deployment process, ensuring the latest build is deployed seamlessly.
Jenkins Pipeline Snippet:
groovyCopy codestage('Deploy') {
deploy adapters: [tomcat9(credentialsId: 'tomcat',
url: 'http://54.163.203.241:8080')],
contextPath: 'mydeployment',
war: 'target/*.war'
}
Result:
The application is now deployed and accessible through the configured Tomcat server URL.
🌟 Complete Jenkins Pipeline Script
Here is the full pipeline code for reference:
groovyCopy codenode {
stage("Code") {
git "https://github.com/rohit23106/one.git"
}
stage("Build") {
sh "mvn clean package"
}
stage("CQA") {
withSonarQubeEnv(credentialsID: 'sonar-password') {
def mavenHome = tool name: "maven", type: "maven"
sh "${mavenHome}/bin/mvn sonar:sonar"
}
}
stage('Artifact') {
nexusArtifactUploader artifacts: [[artifactId: 'myweb',
file: 'target/myweb-8.6.7.war',
type: 'war']],
credentialsId: 'nexus',
groupId: 'in.javahome',
nexusUrl: 'http://52.73.214.233:8081',
repository: 'rohitrepo',
version: '8.6.7'
}
stage('Deploy') {
deploy adapters: [tomcat9(credentialsId: 'tomcat',
url: 'http://54.163.203.241:8080')],
contextPath: 'mydeployment',
war: 'target/*.war'
}
}
Benefits of This CI/CD Pipeline Setup
Faster Releases: Automated builds and deployments reduce time to market.
Improved Code Quality: Continuous monitoring of code quality using SonarQube.
Centralized Artifact Management: Nexus ensures version control and easy access to artifacts.
Seamless Deployment: Tomcat integration enables smooth delivery of web applications.
Scalable & Repeatable: The same setup can be reused for different applications.
Conclusion
With this end-to-end CI/CD pipeline, we have achieved automated builds, testing, quality analysis, artifact management, and deployment. This setup enhances productivity, minimizes manual errors, and ensures the delivery of high-quality software.
I hope this article provides insights into setting up a modern CI/CD pipeline with Jenkins and other tools.
Happy deploying! 🎉
Tags:
#DevOps #CI/CD #Jenkins #GitHub #Maven #SonarQube #Nexus #Tomcat
Subscribe to my newsletter
Read articles from Majety Naga Venkata Rohit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
