Understanding Sorting Algorithms: A Guide to Arranging Data Efficiently
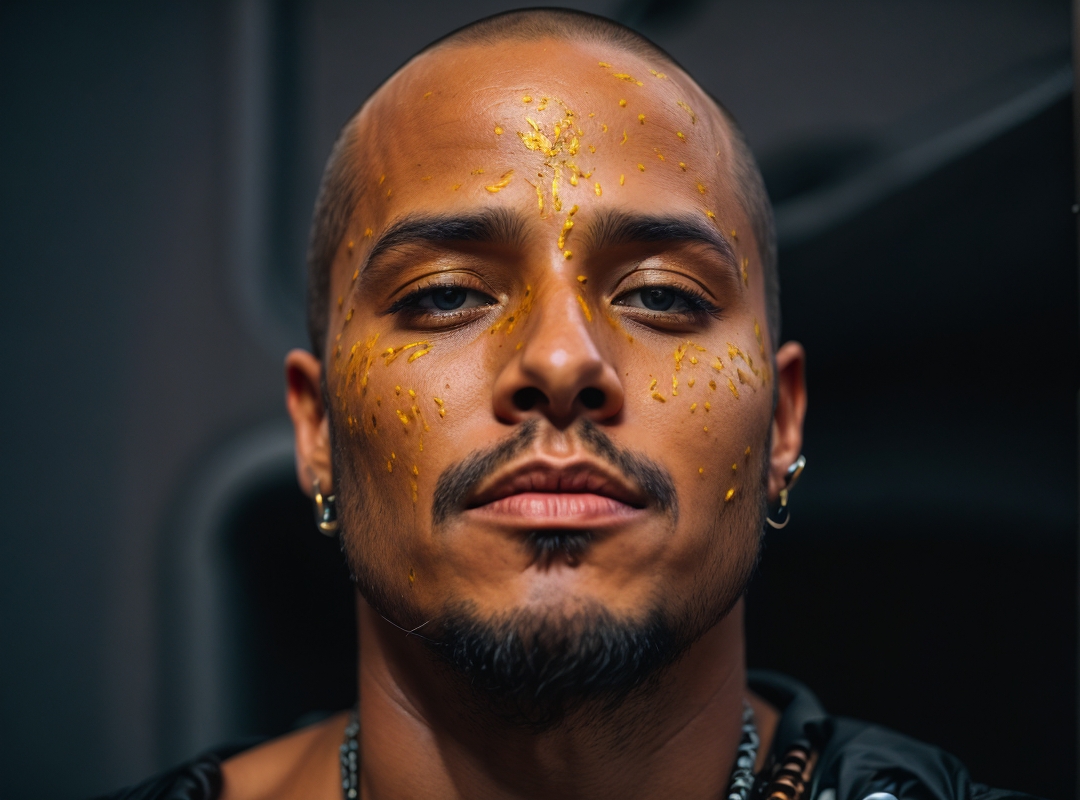
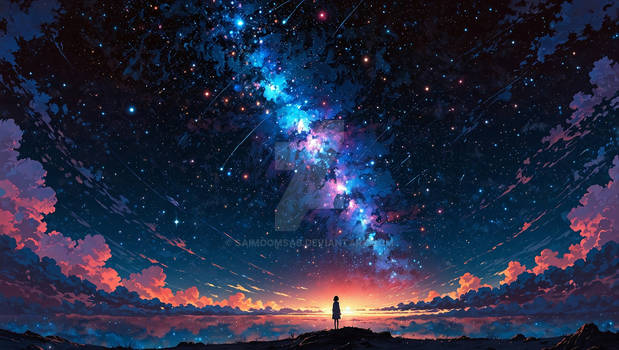
Sorting algorithms are fundamental tools in computer science and data processing. They allow us to rearrange elements in an array or list based on specified comparison criteria, enabling efficient searching, analyzing, and manipulating of data. In this blog post, we will explore what sorting algorithms are, why they are important, and examine some of the most commonly used sorting algorithms, alongside their advantages, disadvantages, and practical C language implementations.
What is a Sorting Algorithm?
A sorting algorithm is a method used to rearrange the elements of a list or array into a specific order, typically ascending or descending. These elements can be numbers, letters, or any other type of data that can be compared using a defined comparison operator. The comparison operator guides the algorithm in deciding the order of elements; for example, by checking if one number is less than, greater than, or equal to another.
Sorting is performed for various purposes in computing, such as:
Improving search efficiency: Sorted data can significantly reduce search times, especially when using binary search algorithms.
Data organization: Organized data is easier to read, analyze, and visualize.
Enhancing overall performance: Several algorithms and processes rely on sorted inputs to function optimally.
Commonly Used Sorting Algorithms
There are numerous sorting algorithms, each with its specific advantages and drawbacks. Here are some of the most popular ones, along with corresponding C code examples.
1. Bubble Sort
How it Works: This algorithm repeatedly steps through the array, compares adjacent elements, and swaps them if they are in the wrong order. This process continues until no swaps are needed, meaning the array is sorted.
Complexity:
Best Case: O(n)
Average Case: O(n^2)
Worst Case: O(n^2)
Advantages:
Simple to understand and implement.
Requires no additional storage space.
Disadvantages:
Inefficient on larger lists.
Performs many unnecessary comparisons.
C Implementation:
#include <stdio.h>
// Function to perform Bubble Sort
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr) / sizeof(arr[0]);
bubbleSort(arr, n);
printf("Sorted array using Bubble Sort: \n");
printArray(arr, n);
return 0;
}
2. Selection Sort
How it Works: The selection sort algorithm divides the input list into two parts: the sorted part and the unsorted part. It repeatedly selects the smallest (or largest) element from the unsorted part and swaps it with the leftmost unsorted element.
Complexity:
Best Case: O(n^2)
Average Case: O(n^2)
Worst Case: O(n^2)
Advantages:
Simple and easy to implement.
Works well for small datasets.
Disadvantages:
Not efficient for large lists.
Fewer practical applications compared to more advanced algorithms.
C Implementation:
#include <stdio.h>
// Function to perform Selection Sort
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
// Swap the found minimum element with the first element
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr) / sizeof(arr[0]);
selectionSort(arr, n);
printf("Sorted array using Selection Sort: \n");
printArray(arr, n);
return 0;
}
3. Insertion Sort
How it Works: This algorithm builds the sorted array one element at a time, resembling how one might sort playing cards. Starting from the second element, it compares the current element with the sorted part and places it in the correct position.
Complexity:
Best Case: O(n) (when the list is already sorted)
Average Case: O(n^2)
Worst Case: O(n^2)
Advantages:
Efficient for small datasets and nearly sorted lists.
In-place sorting with low overhead.
Disadvantages:
- Becomes inefficient for larger lists.
C Implementation:
#include <stdio.h>
// Function to perform Insertion Sort
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
// Move elements of arr[0..i-1] that are greater than key
// to one position ahead of their current position
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr) / sizeof(arr[0]);
insertionSort(arr, n);
printf("Sorted array using Insertion Sort: \n");
printArray(arr, n);
return 0;
}
4. Merge Sort
How it Works: Merge sort is a divide-and-conquer algorithm. It repeatedly divides the array into sublists until each sublist contains one element and then merges those sublists to produce new sorted sublists.
Complexity:
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Advantages:
Consistent O(n log n) time complexity regardless of input data.
Efficient for large datasets.
Disadvantages:
- Requires additional space proportional to the size of the data.
C Implementation:
#include <stdio.h>
// Merging function
void merge(int arr[], int left, int mid, int right) {
int i, j, k;
int n1 = mid - left + 1;
int n2 = right - mid;
int L[n1], R[n2];
for (i = 0; i < n1; i++)
L[i] = arr[left + i];
for (j = 0; j < n2; j++)
R[j] = arr[mid + 1 + j];
i = 0; // Initial index of first sub-array
j = 0; // Initial index of second sub-array
k = left; // Initial index of merged sub-array
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
// Function to perform Merge Sort
void mergeSort(int arr[], int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {38, 27, 43, 3, 9, 82
}
// Function to perform Heap Sort
void heapSort(int arr[], int n) {
// Build heap (rearrange array)
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
// One by one extract elements from heap
for (int i = n - 1; i >= 0; i--) {
swap(&arr[0], &arr[i]); // Move current root to end
heapify(arr, i, 0); // Call max heapify on the reduced heap
}
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {12, 11, 13, 5, 6, 7};
int n = sizeof(arr) / sizeof(arr[0]);
heapSort(arr, n);
printf("Sorted array using Heap Sort: \n");
printArray(arr, n);
return 0;
}
7. Counting Sort
How it Works: Counting sort works best when the range of input values (the difference between the maximum and minimum values) is known and relatively small. It counts the occurrences of each unique value in the input data and calculates the positions of each element in the sorted array.
Complexity:
Best Case: O(n + k) (where k is the range of the input data)
Average Case: O(n + k)
Worst Case: O(n + k)
Advantages:
Efficient for sorting integers or objects with a known range.
Performs better than O(n log n) comparison sorts under certain conditions.
Disadvantages:
Not suitable for sorting data with a large range of values or non-integer data types.
Requires extra space proportional to the range of input values.
C Implementation:
#include <stdio.h>
#include <stdlib.h>
// Function to perform Counting Sort
void countingSort(int arr[], int n) {
int output[n]; // Output array
int count[256] = {0}; // Count array for storing count of elements
// Store count of each number
for (int i = 0; i < n; i++)
count[arr[i]]++;
// Change count[i] so that count[i] now contains the actual position of this number in output[]
for (int i = 1; i <= 255; i++)
count[i] += count[i - 1];
// Build the output array
for (int i = n - 1; i >= 0; i--) {
output[count[arr[i]] - 1] = arr[i];
count[arr[i]]--;
}
// Copy the output array to arr[], so that arr[] now contains sorted numbers
for (int i = 0; i < n; i++)
arr[i] = output[i];
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {4, 2, 2, 8, 3, 3, 1};
int n = sizeof(arr) / sizeof(arr[0]);
countingSort(arr, n);
printf("Sorted array using Counting Sort: \n");
printArray(arr, n);
return 0;
}
8. Radix Sort
How it Works: Radix sort processes integer keys by individual digits, sorting input numbers by each digit from the least significant to the most significant. It relies on a stable sub-sort (commonly counting sort) to ensure that numbers with the same digit do not switch order.
Complexity:
Best Case: O(nk) (where k is the number of digits in the maximum number)
Average Case: O(nk)
Worst Case: O(nk)
Advantages:
Can sort numbers in linear time when the range of integers is not significantly large compared to the number of items.
Efficiently handles large datasets that have fixed-length keys.
Disadvantages:
Requires additional space equivalent to the number of keys being sorted.
Not suitable for general-purpose sorting and typically limited to numeric data.
C Implementation:
#include <stdio.h>
// Function to perform Counting Sort based on the digit represented by exp
void countingSortForRadix(int arr[], int n, int exp) {
int output[n]; // Output array
int count[10] = {0}; // Count array for storing count of occurrences of each digit
// Store count of occurrences of each digit in the current exponent
for (int i = 0; i < n; i++) {
count[(arr[i] / exp) % 10]++;
}
// Change count[i] so that count[i] contains the actual position of this digit in output[]
for (int i = 1; i < 10; i++) {
count[i] += count[i - 1];
}
// Build the output array
for (int i = n - 1; i >= 0; i--) {
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
// Copy the output array to arr[], so that arr[] now contains sorted numbers according to the current digit
for (int i = 0; i < n; i++) {
arr[i] = output[i];
}
}
// Function to perform Radix Sort
void radixSort(int arr[], int n) {
// Find the maximum number to know the number of digits
int max = arr[0];
for (int i = 1; i < n; i++) {
if (arr[i] > max)
max = arr[i];
}
// Apply counting sort for each digit
for (int exp = 1; max / exp > 0; exp *= 10) {
countingSortForRadix(arr, n, exp);
}
}
// Function to print the array
void printArray(int arr[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {170, 45, 75, 90, 802, 24, 2, 66};
int n = sizeof(arr) / sizeof(arr[0]);
radixSort(arr, n);
printf("Sorted array using Radix Sort: \n");
printArray(arr, n);
return 0;
}
Choosing the Right Sorting Algorithm
Selecting the appropriate sorting algorithm for a given situation depends on various factors, including:
Data Size: Simpler algorithms like bubble sort and insertion sort may be efficient for small datasets, but larger datasets often require more advanced algorithms.
Data Characteristics: If the data is nearly sorted, insertion sort or bubble sort may perform well. In contrast, random data may benefit more from quick sort or merge sort.
Memory Considerations: If memory usage is a critical concern, in-place algorithms (like quick sort and heap sort) are preferable.
Algorithm Complexity: Understanding the time complexity of each algorithm, according to the specific use case, is essential. If guaranteed performance is needed, algorithms like merge sort or heap sort may be chosen.
Conclusion
Sorting algorithms are a cornerstone of computer science that facilitate various data operations, from searching to data analysis. By understanding the mechanics, advantages, and trade-offs of different sorting methods—from simple to complex—you can make informed choices about which algorithm to implement for your specific needs.
Whether you are processing a small list of items or deploying large datasets in an enterprise system, recognizing the nuances of sorting algorithms will empower you to enhance performance and efficiency in your applications. Take time to experiment with different algorithms and consider their behaviors in various situations to become proficient in data handling.
Subscribe to my newsletter
Read articles from Younis Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
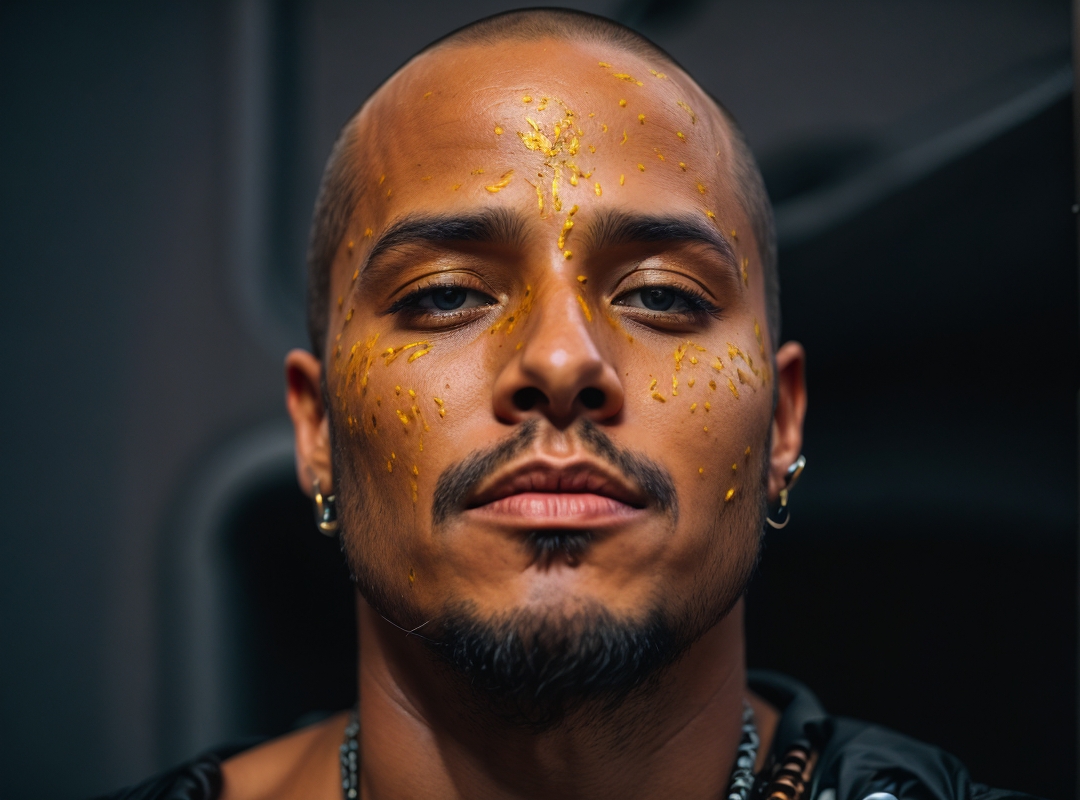
Younis Ahmed
Younis Ahmed
Hey there! 👋 Developer by day, Graphic Designer by night, and Software Engineer Student in between. 🌟 Join me on this journey as I explore the world of coding, design, and everything tech-related. 🖥️✨ #DeveloperLife #GraphicDesignPassion #TechJourney