Day 11 Mastering🖥️ Error Handling in Shell Scripting🎯
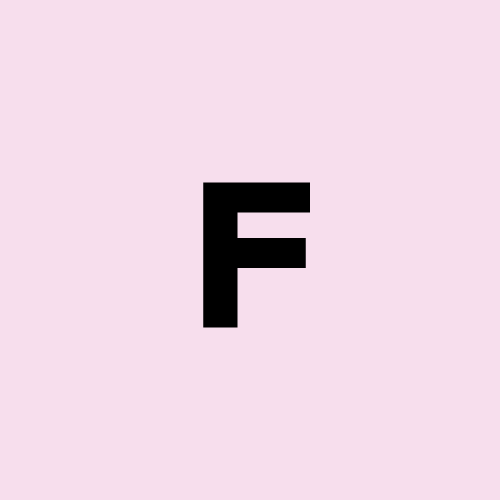
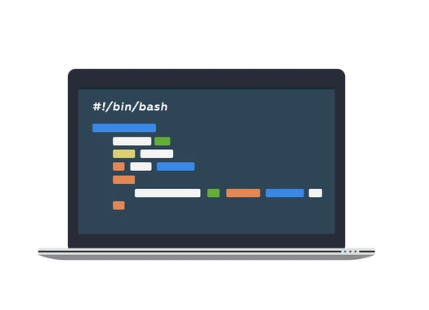
Error handling in shell scripting is essential for building resilient scripts that can handle unexpected situations gracefully
Task 1: Checking Exit Status
Objective: Write a script that tries to create a directory and checks if the command was successful. If it fails, print an error message.
#!/bin/bash
# Task 1: Checking Exit Status
# Attempt to create a directory
mkdir /tmp/mydir
# Check if the command was successful
if [ $? -ne 0 ]; then
echo "Failed to create directory /tmp/mydir"
else
echo "Directory /tmp/mydir created successfully"
fi
Task 2: Using If Statements for Error Checking
Objective: Extend the script to include more commands (e.g., creating a file inside the directory). Use if
statements to check if each command is successful.
#!/bin/bash
# Task 2: Using if Statements for Error Checking
# Define the directory and file names
dir_name="/tmp/mydir"
file_name="$dir_name/myfile.txt"
# Attempt to create a directory
if mkdir "$dir_name"; then
echo "Directory $dir_name created successfully."
else
echo "Failed to create directory $dir_name."
exit 1
fi
# Attempt to create a file inside the directory
if touch "$file_name"; then
echo "File $file_name created successfully."
else
echo "Failed to create file $file_name."
exit 1
fi
Task 3: Using Trap for Cleanup
Objective: Write a script that creates a temporary file and sets a trap to delete the file if the script exits unexpectedly.
#!/bin/bash
# Task 3: Using trap for Cleanup
# Create a temporary file
tempfile=$(mktemp)
# Set a trap to delete the file if the script exits unexpectedly
trap "rm -f $tempfile" EXIT
# Write to the temporary file
echo "This is a temporary file." > "$tempfile"
# Display the contents of the temporary file
cat "$tempfile"
# Simulate an error (you can comment this line to see the cleanup)
exit 1
Task 4: Redirecting Errors
Objective: Write a script that tries to read a non-existent file and redirects the error message to a file called error.log
.
#!/bin/bash
# Task 4: Redirecting Errors
# Attempt to read a non-existent file and redirect error to error.log
cat non_existent_file.txt 2> error.log
# Inform the user
echo "Attempted to read non_existent_file.txt. Check error.log for details."
Task 5: Creating Custom Error Messages
Objective: Modify one of the previous scripts to include custom error messages that provide more context about what went wrong.
#!/bin/bash
# Task 5: Creating Custom Error Messages
# Attempt to create a directory
dir_name="/tmp/mydir"
if mkdir "$dir_name"; then
echo "Directory $dir_name created successfully."
else
echo "Error: Directory $dir_name could not be created. Check if you have the necessary permissions."
exit 1
fi
# Attempt to create a file inside the directory
file_name="$dir_name/myfile.txt"
if touch "$file_name"; then
echo "File $file_name created successfully."
else
echo "Error: File $file_name could not be created. Check if the directory exists."
exit 1
fi
Running the Scripts
Save each script to a separate file, for example,
task1.sh
,task2.sh
, etc.Make each script executable:
chmod +x task1.sh
Run the scripts:
./task1.sh
Summary of Error Handling Techniques Used
Exit Status Checking: After each command, we check the exit status using
$?
.If Statements for Error Checking: Using
if
statements to check for success or failure of commands and handle errors accordingly.Using
trap
for Cleanup: Setting up atrap
command to clean up temporary files when the script exits.Redirecting Errors: Redirecting error messages to a file using
2>
.Creating Custom Error Messages: Providing meaningful error messages to help with debugging.
By mastering these error-handling techniques, you’ll build a solid foundation for writing reliable, resilient shell scripts. These practices empower you to handle unexpected scenarios gracefully, improve script stability, and create maintainable code ready for production environments.
Subscribe to my newsletter
Read articles from Fauzeya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
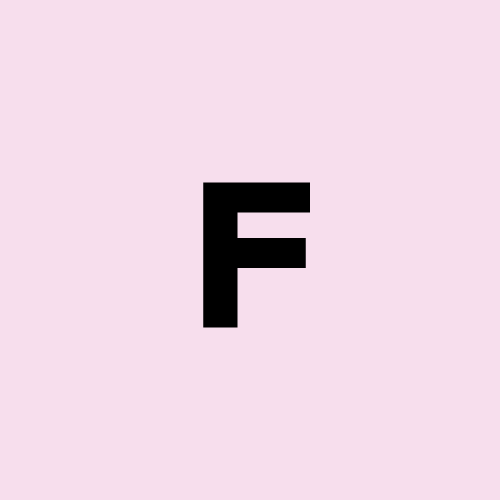
Fauzeya
Fauzeya
Hi there! I'm Fauzeya 👩💻, a passionate DevOps Engineer with a background in Computer Science Engineering🎓. I’m committed to enhancing security🔒, efficiency⚙️, and effectiveness in software development and deployment processes. With extensive knowledge in cloud computing☁️, containerization📦, and automation🤖, I aim to stay updated with the latest tools and methodologies in the DevOps field. Currently, I’m on a journey to deepen my understanding of DevOps I enjoy sharing my learning experiences and insights through my blog, 📝where I cover topics related to DevOps practices, tutorials, and challenges. I believe in continuous growth and learning and am excited to connect with fellow tech enthusiasts and professionals🤝. Let’s embark on this journey together!🚀