Threading and Multiprocessing in Python: An In-Depth Guide

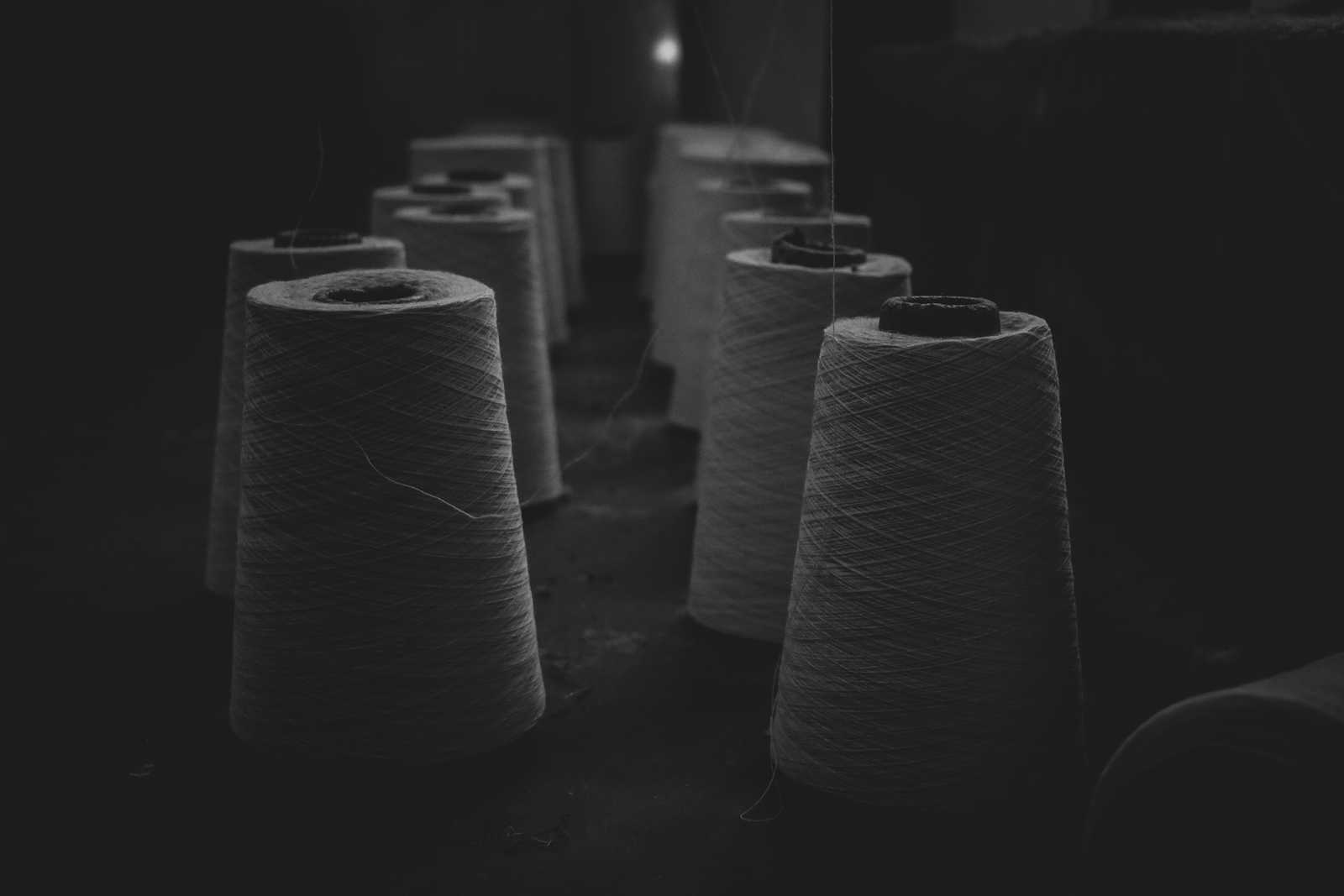
Introduction
Threading and multiprocessing are techniques to achieve concurrency in programs, allowing them to perform multiple tasks simultaneously. Understanding these concepts is crucial for optimizing program performance, especially in applications that require handling multiple operations at once, such as web servers, data processing tasks, and more.
Table of Contents
What is a Thread?
A thread is the smallest sequence of programmed instructions that can be managed independently by a scheduler. In the context of a process, threads share the same memory space and resources but execute independently.
Key Points:
Lightweight: Threads are lightweight compared to processes.
Shared Memory: Threads within the same process share memory and resources.
Concurrency: Threads allow a program to perform multiple operations simultaneously.
Threading Concepts
Processes vs Threads
Process: An instance of a program in execution. Processes have their own memory space.
Thread: A sequence of executable instructions within a process. Threads within the same process share memory.
Differences:
Memory Space:
Processes: Separate memory spaces.
Threads: Share memory space within the process.
Communication:
Processes: Communicate via inter-process communication (IPC) mechanisms.
Threads: Can communicate directly through shared variables.
Visual Representation:
+------------------+
| Process |
| +--------------+ |
| | Thread | |
| +--------------+ |
| +--------------+ |
| | Thread | |
| +--------------+ |
+------------------+
Global Interpreter Lock (GIL)
In Python, the Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecodes at once. This means that even in a multithreaded Python program, only one thread executes Python code at a time.
Implications:
CPU-bound Tasks: Multithreading may not provide performance benefits due to the GIL.
I/O-bound Tasks: Multithreading can improve performance as threads can run while waiting for I/O operations.
Multithreading in Python
Creating Threads
You can create threads in Python using the threading
module.
Example:
import threading
def print_numbers():
for i in range(1, 6):
print(f"Number: {i}")
# Create a thread
thread = threading.Thread(target=print_numbers)
# Start the thread
thread.start()
# Wait for the thread to complete
thread.join()
Thread Synchronization
When multiple threads access shared resources, synchronization is necessary to prevent data corruption.
- Lock: A mechanism to ensure that only one thread accesses a resource at a time.
Example using Lock:
import threading
counter = 0
lock = threading.Lock()
def increment_counter():
global counter
for _ in range(100000):
with lock:
counter += 1
threads = []
for _ in range(5):
thread = threading.Thread(target=increment_counter)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(f"Final Counter: {counter}")
Thread Communication
Event: Allows threads to communicate with each other using signaling.
Queue: Thread-safe FIFO implementation for passing data between threads.
Example using Queue:
import threading
import queue
def worker(q):
while True:
item = q.get()
if item is None:
break
print(f"Processing {item}")
q.task_done()
q = queue.Queue()
threads = []
# Start worker threads
for _ in range(3):
t = threading.Thread(target=worker, args=(q,))
t.start()
threads.append(t)
# Enqueue items
for item in range(10):
q.put(item)
# Block until all tasks are done
q.join()
# Stop workers
for _ in range(3):
q.put(None)
for t in threads:
t.join()
Multiprocessing in Python
Why Use Multiprocessing?
Due to the GIL, CPU-bound tasks do not benefit from multithreading in Python. Multiprocessing allows you to create separate processes, each with its own Python interpreter and memory space, bypassing the GIL.
Creating Processes
You can create processes using the multiprocessing
module.
Example:
import multiprocessing
def print_numbers():
for i in range(1, 6):
print(f"Number: {i}")
if __name__ == "__main__":
process = multiprocessing.Process(target=print_numbers)
process.start()
process.join()
Inter-process Communication
Processes have separate memory spaces, so you need special mechanisms to communicate between them.
Queue: Can be used for communication between processes.
Pipe: A two-way communication channel between processes.
Shared Memory: Using
Value
orArray
for shared data.
Example using Queue:
import multiprocessing
def worker(q):
while True:
item = q.get()
if item is None:
break
print(f"Processing {item}")
if __name__ == "__main__":
q = multiprocessing.Queue()
processes = []
# Start worker processes
for _ in range(3):
p = multiprocessing.Process(target=worker, args=(q,))
p.start()
processes.append(p)
# Enqueue items
for item in range(10):
q.put(item)
# Stop workers
for _ in range(3):
q.put(None)
for p in processes:
p.join()
Threading vs Multiprocessing
Aspect | Threading | Multiprocessing |
Memory Space | Shared among threads within the same process | Separate memory space for each process |
Communication | Easier (shared variables, data structures) | Requires IPC mechanisms (Queue, Pipe, etc.) |
GIL Limitation | Affected by GIL in Python (only one thread runs) | Bypasses GIL (multiple processes run) |
Overhead | Lower overhead (lightweight) | Higher overhead (heavier than threads) |
Use Cases | I/O-bound tasks | CPU-bound tasks |
Examples and Code
Multithreading Example
I/O-bound Task: Downloading Multiple URLs
import threading
import requests
import time
urls = [
'https://www.example.com',
'https://www.python.org',
'https://www.openai.com',
# Add more URLs as needed
]
def download_url(url):
response = requests.get(url)
print(f"Downloaded {url} with status {response.status_code}")
start_time = time.time()
threads = []
for url in urls:
thread = threading.Thread(target=download_url, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
end_time = time.time()
print(f"Downloaded {len(urls)} URLs in {end_time - start_time:.2f} seconds")
Explanation:
Objective: Download multiple URLs concurrently.
Benefit: Threads can perform I/O operations while others are waiting due to the GIL not being a bottleneck for I/O-bound tasks.
Multiprocessing Example
CPU-bound Task: Calculating Factorials
import multiprocessing
import math
import time
numbers = [50000, 60000, 70000, 80000]
def compute_factorial(n):
print(f"Computing factorial of {n}")
math.factorial(n)
print(f"Completed factorial of {n}")
if __name__ == "__main__":
start_time = time.time()
processes = []
for number in numbers:
process = multiprocessing.Process(target=compute_factorial, args=(number,))
processes.append(process)
process.start()
for process in processes:
process.join()
end_time = time.time()
print(f"Computed factorials in {end_time - start_time:.2f} seconds")
Explanation:
Objective: Compute factorials of large numbers concurrently.
Benefit: Multiprocessing utilizes multiple CPU cores, bypassing the GIL for CPU-bound tasks.
Conclusion
Threading and multiprocessing are powerful tools for achieving concurrency in Python. While threading is suitable for I/O-bound tasks due to the GIL, multiprocessing shines with CPU-bound tasks by utilizing multiple CPU cores. Understanding when and how to use each can greatly enhance the performance of your applications.
Quick Revision Notes
Thread: Smallest unit of execution within a process; threads share memory.
Process: An independent execution unit with its own memory space.
GIL: Global Interpreter Lock in Python that allows only one thread to execute at a time.
Multithreading:
Best for I/O-bound tasks.
Affected by GIL in CPU-bound tasks.
Uses the
threading
module.
Multiprocessing:
Ideal for CPU-bound tasks.
Bypasses the GIL.
Each process has its own Python interpreter and memory space.
Uses the
multiprocessing
module.
Thread Synchronization:
Use locks to prevent race conditions.
Lock
,RLock
,Semaphore
are synchronization primitives.
Inter-process Communication (IPC):
- Use
Queue
,Pipe
,Value
,Array
for sharing data between processes.
- Use
Remember: Choose multithreading for tasks that spend time waiting (like I/O operations) and multiprocessing for tasks that require heavy CPU computation.
Additional Resources:
Python Documentation:
Concurrency in Python:
Subscribe to my newsletter
Read articles from Sai Prasanna Maharana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
