ConfigMap in K8s simplified
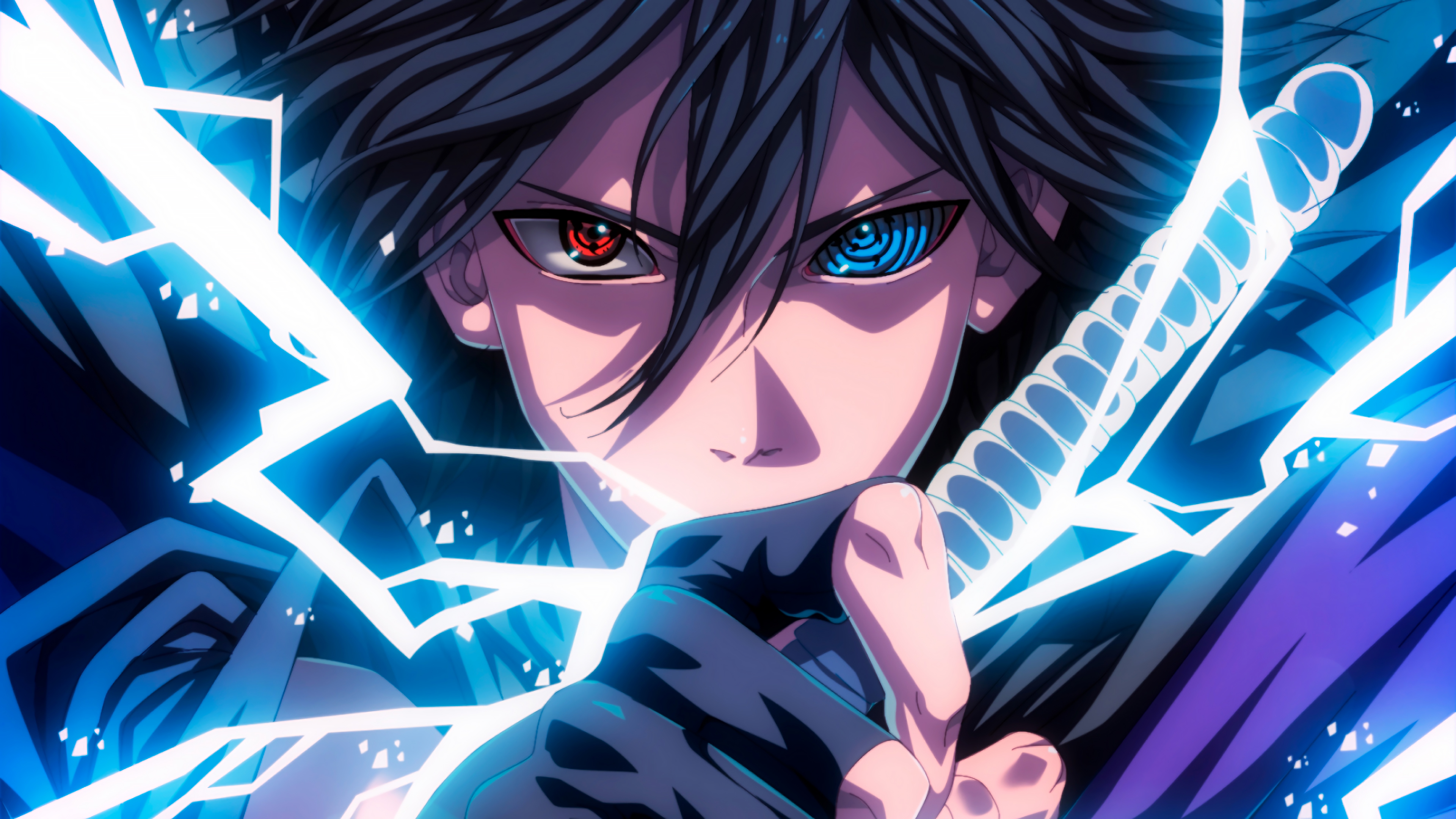
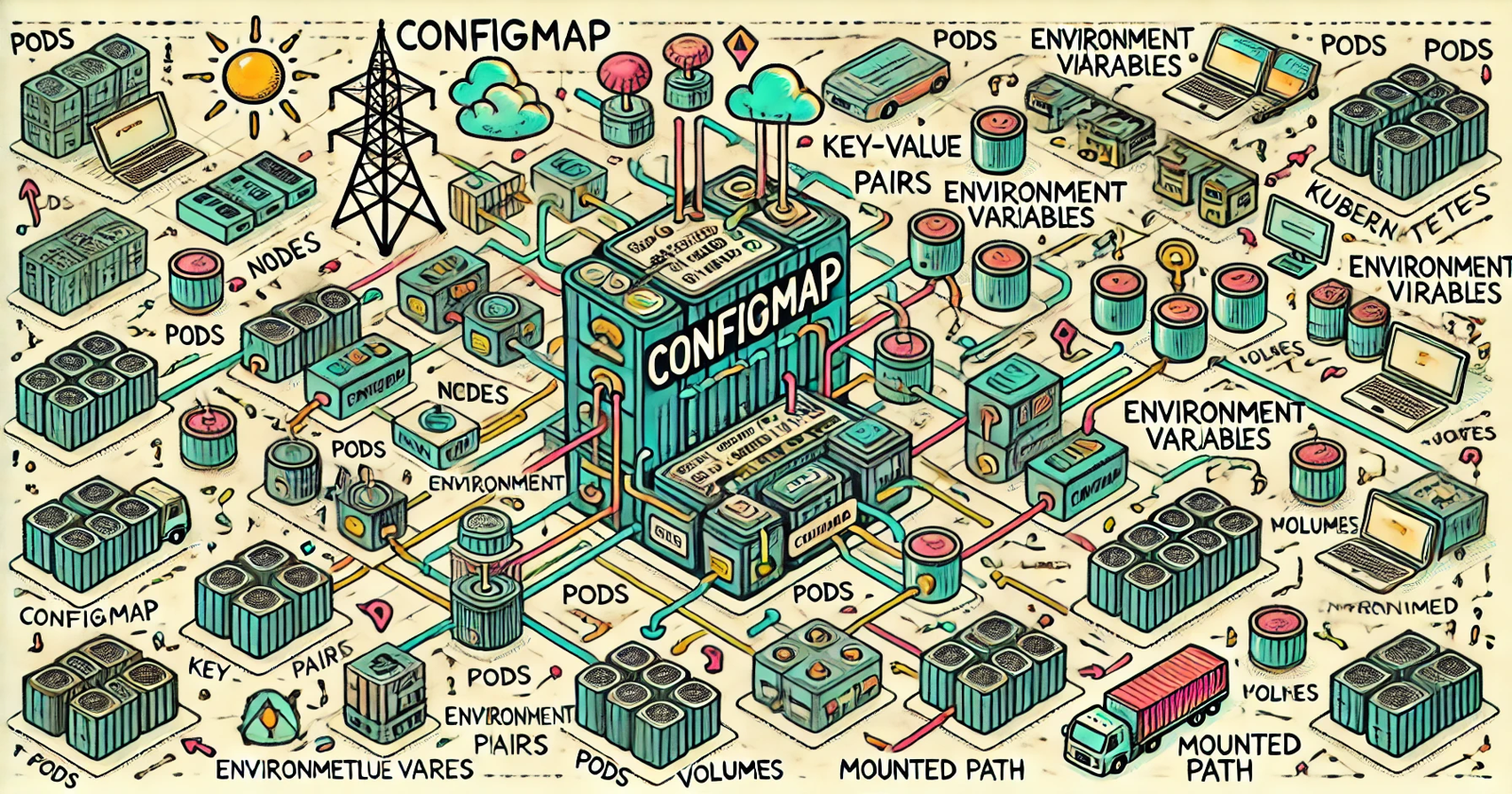
In Kubernetes, a ConfigMap
is a resource used to store configuration data in key-value pairs. This configuration data can then be used in your applications or Kubernetes components without embedding the configuration directly in the container images. ConfigMaps
provide flexibility in managing application settings, making them an essential tool for deploying applications across environments with varying configuration requirements.
Why Do We Need ConfigMap?
Separation of Configuration and Code:
ConfigMap
allows developers to keep their configuration separate from application code, adhering to the twelve-factor app principle.Flexibility Across Environments: Different environments (development, testing, production) often need different configuration values, such as database URLs or API keys.
ConfigMap
allows dynamic changes without needing to rebuild images.Centralized Configuration Management: Configurations for different applications can be centrally managed, updated, and retrieved, enhancing manageability.
Reusability: Multiple pods or services can access the same
ConfigMap
, making configurations easy to reuse across components.
Creating ConfigMap: Imperative and Declarative Methods
1. Imperative Creation
The imperative way of creating ConfigMap
is quick and efficient for testing or one-off tasks, where the configurations are set directly using kubectl
commands.
Example: Creating ConfigMap Using Key-Value Pairs in Command
kubectl create configmap example-config --from-literal=key1=value1 --from-literal=key2=value2
This creates a ConfigMap
named example-config
with two key-value pairs.
Example: Creating ConfigMap from File
You can also create a ConfigMap
from a file. For instance, if you have a configuration file config.txt
:
codekey1=value1
key2=value2
Then, you can create a ConfigMap
from this file:
kubectl create configmap example-config --from-file=config.txt
2. Declarative Creation
In the declarative approach, you define the ConfigMap
in a YAML file and apply it with kubectl
. This method is ideal for configurations under version control, such as in CI/CD pipelines or production environments.
Example: ConfigMap YAML File
Create a YAML file named configmap.yaml
:
apiVersion: v1
kind: ConfigMap
metadata:
name: example-config
data:
key1: value1
key2: value2
Apply this file with kubectl
:
kubectl apply -f configmap.yaml
Accessing ConfigMap in Pods
You can access ConfigMap
data in your pods in various ways:
As Environment Variables: This method makes configuration values directly accessible in your application code as environment variables.
Example:
apiVersion: v1 kind: Pod metadata: name: configmap-env-pod spec: containers: - name: app-container image: nginx envFrom: - configMapRef: name: example-config
Here, all key-value pairs in
example-config
will be available as environment variables in theapp-container
.As Volume: Mounting a
ConfigMap
as a volume allows applications to access configurations as files.Example:
apiVersion: v1 kind: Pod metadata: name: configmap-volume-pod spec: containers: - name: app-container image: nginx volumeMounts: - name: config-volume mountPath: /etc/config volumes: - name: config-volume configMap: name: example-config
In this setup, each key in
example-config
will be represented as a file under/etc/config
, with the file content as the corresponding value.Using
kubectl
to View ConfigMap: You can view the contents of aConfigMap
using:kubectl describe configmap example-config
or
kubectl get configmap example-config -o yaml
Updating a ConfigMap
Updating a ConfigMap
can be done through kubectl
if created imperatively, or by reapplying the YAML file if created declaratively.
Example: Updating Imperatively
kubectl create configmap example-config --from-literal=key3=value3 --dry-run=client -o yaml | kubectl apply -f -
This command adds key3
to example-config
.
Example Use Case
Suppose you have an application that needs different logging levels in various environments. You can create a ConfigMap
with the logging level setting and use it across environments.
apiVersion: v1
kind: ConfigMap
metadata:
name: app-config
data:
LOG_LEVEL: "DEBUG"
In the application pod definition, you reference this ConfigMap
to set the logging level as an environment variable:
apiVersion: v1
kind: Pod
metadata:
name: app-pod
spec:
containers:
- name: app-container
image: myapp:latest
envFrom:
- configMapRef:
name: app-config
Best Practices with ConfigMap
Do Not Store Sensitive Data:
ConfigMap
is not secure and should not be used for sensitive information like passwords or API keys. For sensitive data, use KubernetesSecrets
.Version Your Configurations: Store
ConfigMap
YAML files in version control to maintain a history of configuration changes.Use Environment-Specific ConfigMaps: Create different
ConfigMap
s for each environment (e.g.,dev
,test
,prod
) to avoid unintentional configuration overlap.Update Pods on ConfigMap Change: Changing a
ConfigMap
won’t automatically update the pods using it. Consider rolling updates or restarting pods to pick up configuration changes.
Summary
ConfigMap is a key-value store for non-sensitive configuration data in Kubernetes.
You can create
ConfigMap
imperatively (kubectl create configmap
) or declaratively (using YAML files).ConfigMap
can be accessed by pods as environment variables or as mounted volumes.Best practices include using
Secrets
for sensitive data and managingConfigMap
s in version control.
Using ConfigMap
effectively can significantly improve the maintainability and scalability of Kubernetes applications, especially in dynamic, multi-environment deployments.
Subscribe to my newsletter
Read articles from Syed Mahmood Ali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
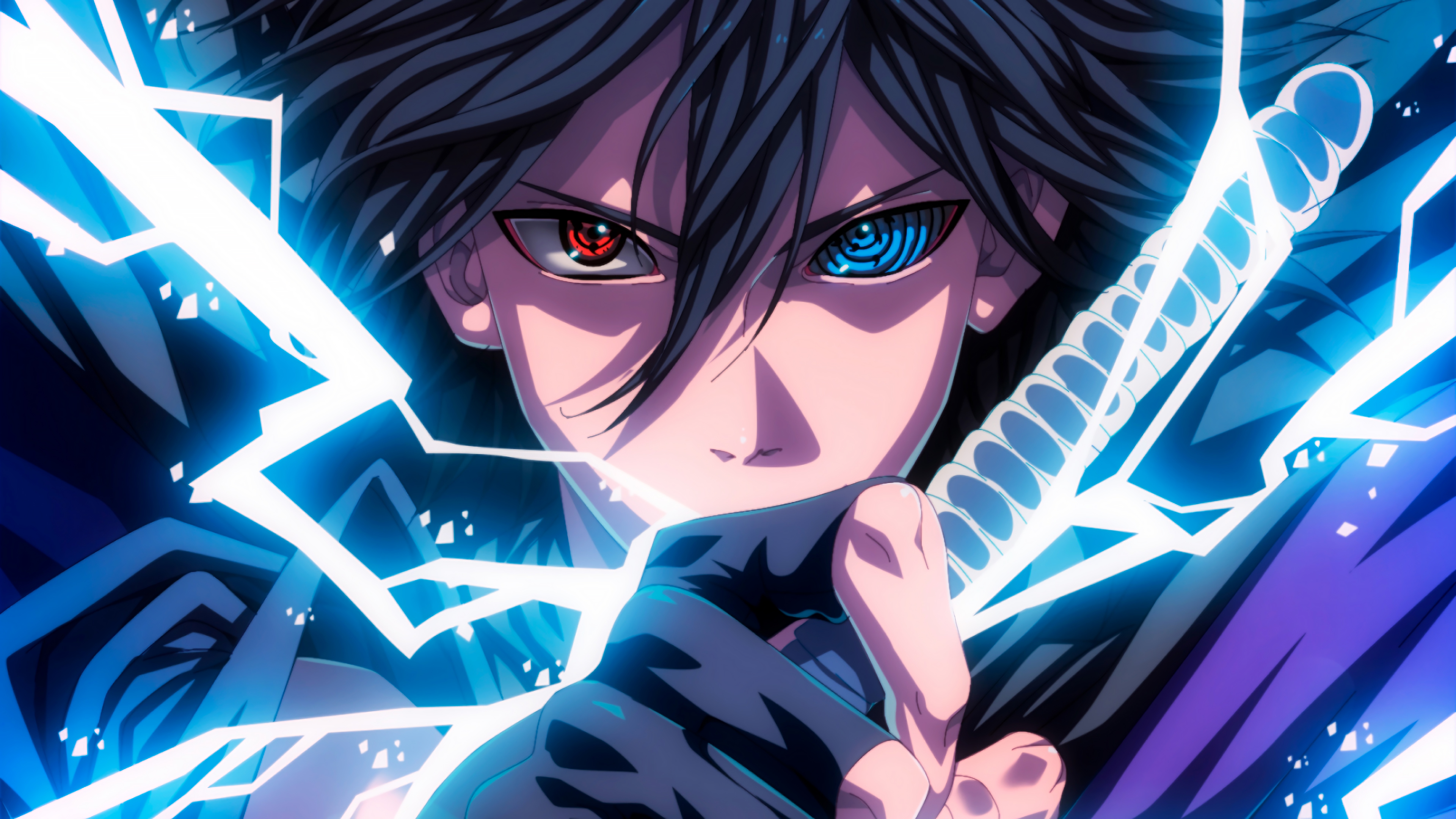