How to capture errors and exceptions with Sentry

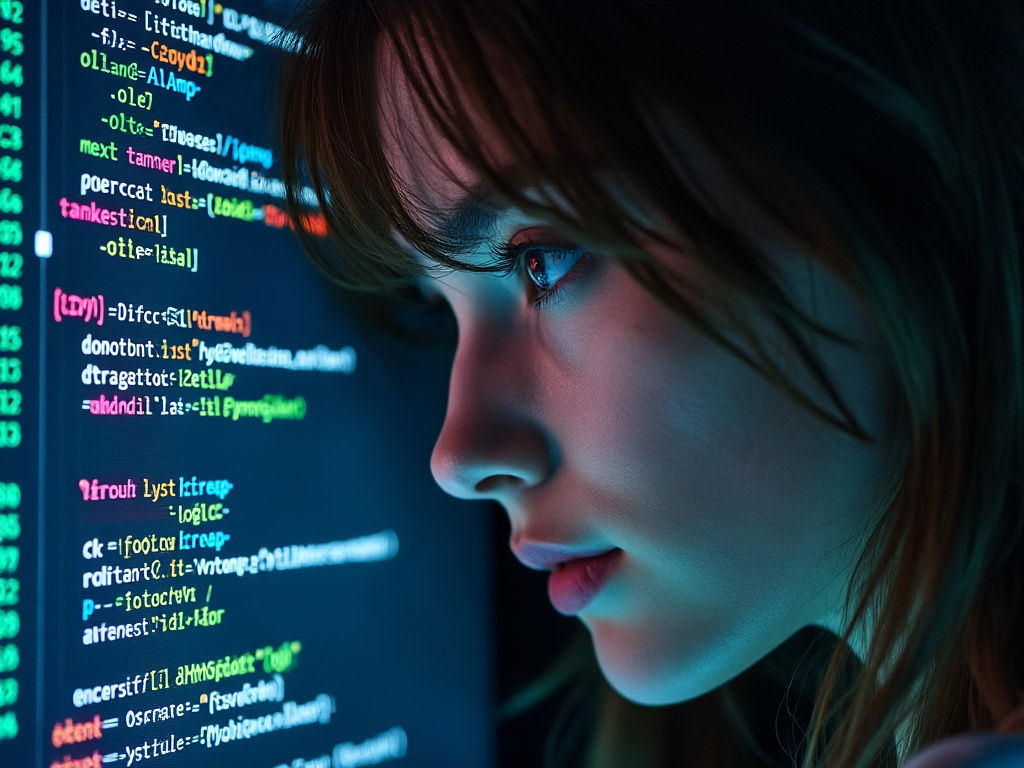
Keeping track of errors in production is essential to maintaining a healthy application. While console logs and error messages might help during development, you need a more robust solution for production environments. This is where Sentry comes in – a powerful error tracking service that helps you monitor and fix crashes in real time.
Why Sentry?
Real-time error tracking: Get instant notifications when errors occur
Detailed error context: Capture stack traces, user information, and environment data
Issue grouping: Similar errors are automatically grouped for easier debugging
Performance monitoring: Track application performance alongside errors
Cross-platform support: Works with multiple frameworks and languages
Getting Started
1. Installation
First, install the Sentry SDK in your JavaScript project:
# Using npm
npm install @sentry/browser
# Using yarn
yarn add @sentry/browser
2. Basic Configuration
Initialize Sentry as early as possible in your application:
import * as Sentry from "@sentry/browser";
Sentry.init({
dsn: "your-dsn-here",
// Set tracesSampleRate to 1.0 to capture 100% of transactions
tracesSampleRate: 1.0,
environment: process.env.NODE_ENV
});
3. Capturing Errors
Automatic Error Capturing
Once initialized, Sentry automatically captures unhandled exceptions and promise rejections:
// This error will be automatically captured
throw new Error("This is an example error");
Manual Error Capturing
For more control, you can manually capture errors:
try {
someRiskyOperation();
} catch (error) {
Sentry.captureException(error);
}
// Or capture a custom message
Sentry.captureMessage("Something went wrong", "error");
4. Adding Context
Make your error reports more useful by adding extra context:
// Add user context
Sentry.setUser({
id: "123",
email: "user@example.com",
username: "johnDoe"
});
// Add tags for better filtering
Sentry.setTag("page_locale", "de-at");
// Add extra context
Sentry.setExtra("character_count", 5);
5. Custom Error Breadcrumbs
Breadcrumbs help you understand what happened before an error:
Sentry.addBreadcrumb({
category: "auth",
message: "Attempting user login",
level: "info"
});
Best Practices
- Filter Sensitive Data
Sentry.init({
dsn: "your-dsn-here",
beforeSend(event) {
// Don't send passwords or personal info
if (event.request?.headers?.["Authorization"]) {
delete event.request.headers["Authorization"];
}
return event;
}
});
- Set Release Information
Sentry.init({
dsn: "your-dsn-here",
release: "my-app@1.0.0",
environment: process.env.NODE_ENV
});
- Configure Error Sampling
Sentry.init({
dsn: "your-dsn-here",
sampleRate: 0.5 // Only send 50% of errors
});
Framework-Specific Integration
React
import * as Sentry from "@sentry/react";
// Error boundary component
const FallbackComponent = () => <div>An error has occurred</div>;
export default Sentry.withErrorBoundary(YourComponent, {
fallback: FallbackComponent
});
Vue
import * as Sentry from "@sentry/vue";
Sentry.init({
Vue,
dsn: "your-dsn-here"
});
Conclusion
Implementing Sentry in your JavaScript application is a crucial step toward maintaining a reliable production environment. With its powerful features and easy integration, you can quickly start capturing and analyzing errors to improve your application's stability.
Remember to:
Initialize Sentry as early as possible in your application
Add relevant context to your error reports
Configure error sampling based on your needs
Filter out sensitive information
Use framework-specific integrations when available
By following these guidelines, you'll have a robust error tracking system that helps you identify and fix issues before they impact your users.
Subscribe to my newsletter
Read articles from Stefan Skorpen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
