🚀 Day 11 of #30DaysOfJavaScript: Understanding Promises in JavaScript
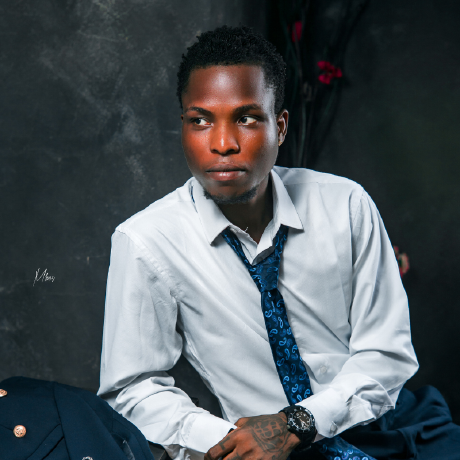
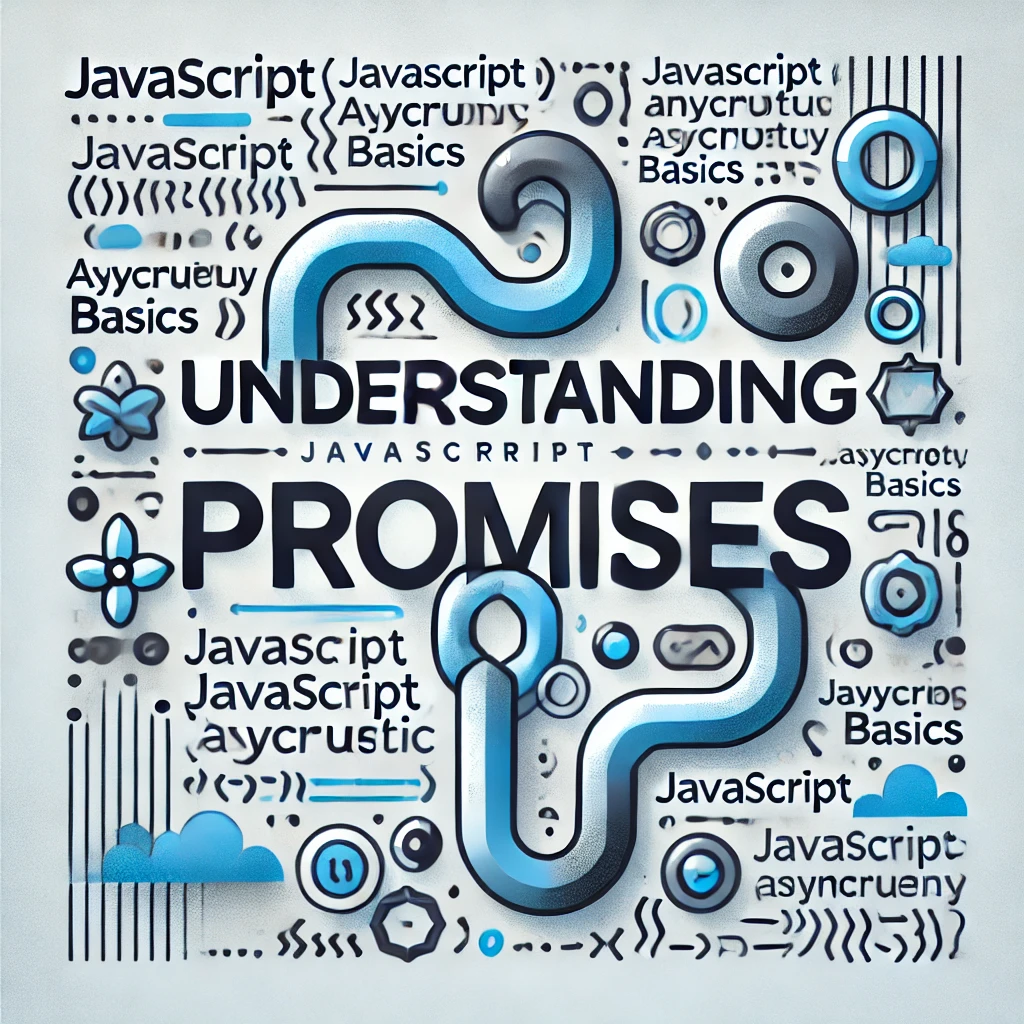
Welcome to Day 11! Today, we’re diving into a fundamental concept in JavaScript’s approach to handling asynchronous operations: Promises. If you’ve ever been stumped by complex asynchronous code, understanding promises will be your gateway to more readable, efficient, and manageable JavaScript!
🌟 What Are Promises?
In JavaScript, a promise is an object that represents the eventual completion (or failure) of an asynchronous operation. Think of it as a placeholder for a value we don’t yet have but will eventually. Promises allow us to handle operations that take time, like fetching data from an API or performing heavy calculations, without blocking the rest of the code.
A promise can be in one of three states:
Pending: The operation has not yet been completed in the initial state.
Fulfilled: The operation was successful, and a value is available.
Rejected: Operation failed, and an error reason is available.
💡 Why Use Promises?
Promises help to avoid “callback hell,” where nested callbacks make code difficult to read and maintain. With promises, we get a cleaner syntax that’s easier to manage, chain, and debug. They’re crucial for async tasks like network requests, timers, or reading files.
🔍 How Promises Work: A Simple Example
Let's start with a basic example:
let myPromise = new Promise((resolve, reject) => {
let success = true;
setTimeout(() => {
if (success) {
resolve("Promise fulfilled!");
} else {
reject("Promise rejected!");
}
}, 2000);
});
myPromise
.then(result => {
console.log(result); // Output: "Promise fulfilled!"
})
.catch(error => {
console.error(error); // Output: "Promise rejected!"
});
Here’s what’s happening:
We create a new
Promise
and pass it a function with two arguments:resolve
andreject
.If the operation succeeds, we call
resolve()
with the value we want.If the operation fails, we call
reject()
with an error message.
📋 Practical Use Case: Fetching Data
Promises shine when we need to fetch data, such as from an API. Here’s an example using the fetch()
function, which returns a promise:
fetch('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
if (!response.ok) {
throw new Error("Network response was not ok");
}
return response.json();
})
.then(data => {
console.log(data); // Output: Post data as JSON object
})
.catch(error => {
console.error("There was a problem with the fetch operation:", error);
});
In this example:
fetch()
initiates a request and returns a promise.The
then()
block handles the successful response.If there’s an error (e.g., the server is unreachable), the
catch()
block handles it.
🌐 Promise Chaining
You can chain multiple .then()
calls to sequence-dependent asynchronous operations:
fetch('https://jsonplaceholder.typicode.com/users/1')
.then(response => response.json())
.then(user => {
console.log("User found:", user);
return fetch(`https://jsonplaceholder.typicode.com/posts?userId=${user.id}`);
})
.then(response => response.json())
.then(posts => {
console.log("User's posts:", posts);
})
.catch(error => {
console.error("Error:", error);
});
This example fetches a user and then uses their ID to fetch their posts. Each
.then()
handles the result of the previous operation, creating a logical flow of actions.
✅ Error Handling with .catch()
Promises simplify error handling. Instead of handling errors at every step, we can use .catch()
at the end of the chain to catch any errors from any preceding step:
fetch('https://jsonplaceholder.typicode.com/invalid-endpoint')
.then(response => response.json())
.catch(error => {
console.error("Caught error:", error);
});
🛠️ Practical Tips for Working with Promises
Always handle rejections: Unhandled promise rejections can lead to bugs and unpredictable behavior.
Keep promises in scope: Chained
.then()
calls work well when each promise depends on the previous one.Use
Promise.all()
for multiple promises: If you have multiple asynchronous tasks that can run concurrently,Promise.all()
lets you wait for all of them to complete.
Example with Promise.all()
:
const fetchUser = fetch('https://jsonplaceholder.typicode.com/users/1');
const fetchPosts = fetch('https://jsonplaceholder.typicode.com/posts?userId=1');
Promise.all([fetchUser, fetchPosts])
.then(responses => Promise.all(responses.map(response => response.json())))
.then(data => {
console.log("User:", data[0]);
console.log("Posts:", data[1]);
})
.catch(error => {
console.error("Error with fetching data:", error);
});
🚀 Wrapping Up
Promises are powerful tools for handling asynchronous tasks in JavaScript, making your code cleaner and more manageable. Mastering promises not only enhances your JavaScript skills but also prepares you for understanding async/await, which builds on promises for even more readable code.
📹 Additional Video Resources
To further clarify JavaScript promises, here are some helpful videos. They offer quick, in-depth explanations of promises, with practical examples for both beginners and advanced users.
JavaScript Promises in 10 Minutes by Web Dev Simplified
This 10-minute tutorial explains the essentials of promises, from basic concepts to practical uses.Understanding JavaScript Promises: A Beginner's Guide
A step-by-step breakdown for newcomers, ideal for anyone looking to start working with promises.JavaScript Promises Crash Course by Academind
This crash course dives deeper, covering error handling, chaining, and real-world examples.
Stay tuned for tomorrow’s lesson as we explore async/await and see how it complements promises to make async programming even smoother. Happy coding!
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
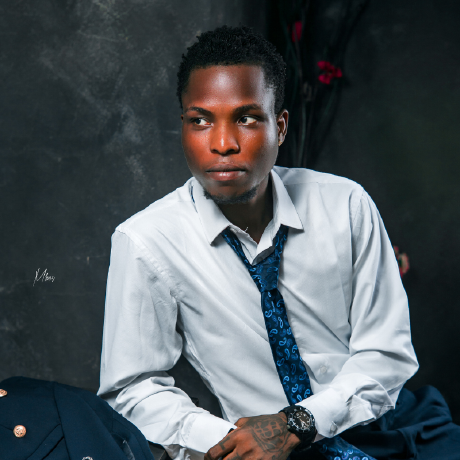
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com