Understanding API Paradigms: A Deep Dive into GraphQL, REST, and gRPC
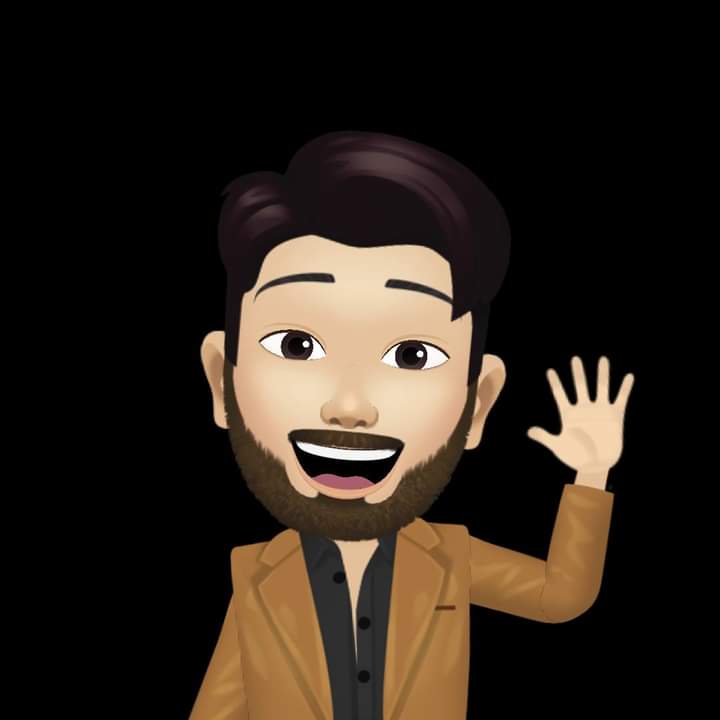
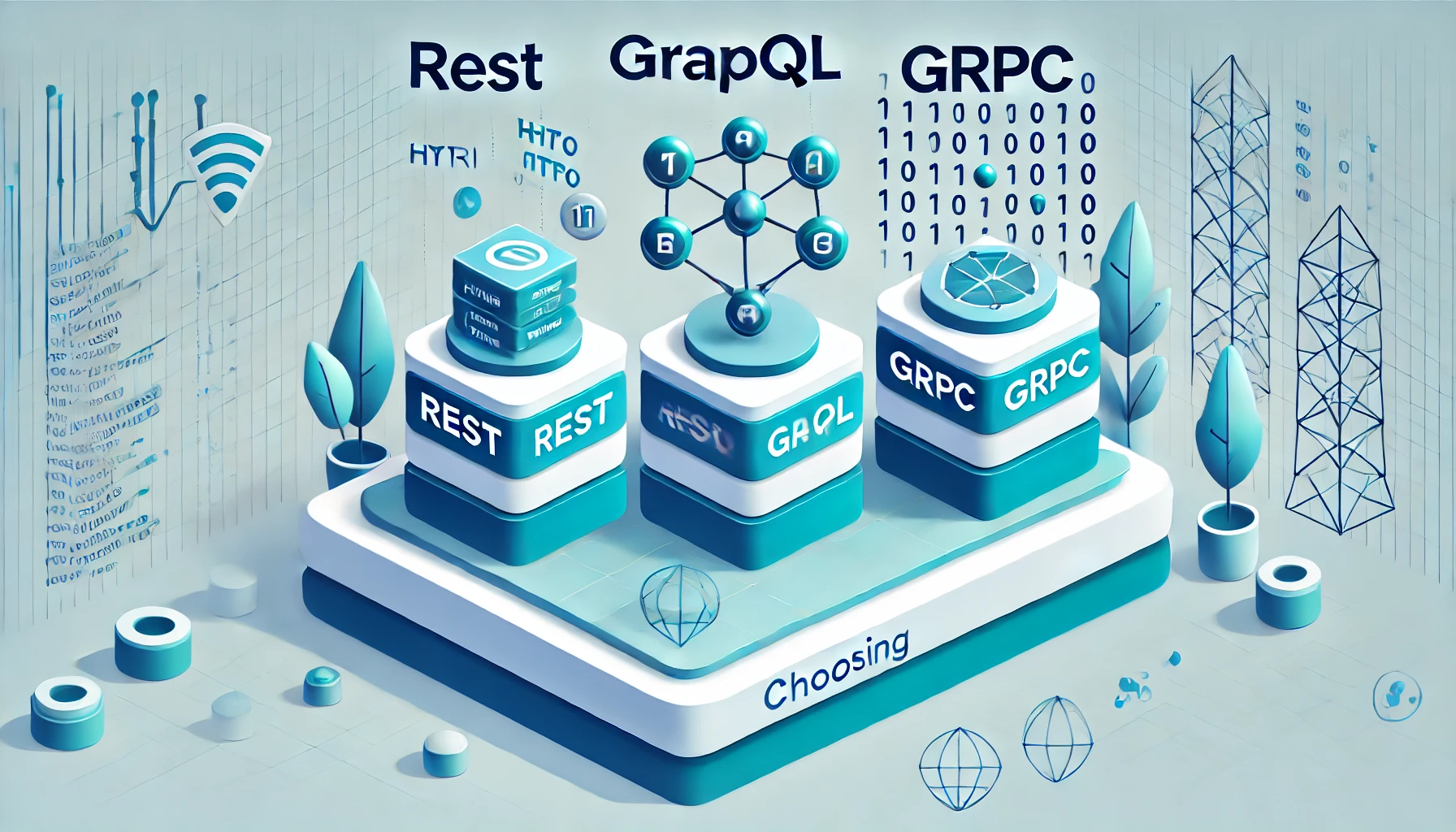
In today's software development landscape, APIs (Application Programming Interfaces) play a crucial role in enabling communication between different systems. Among the various types of APIs available, GraphQL, REST, and gRPC are three of the most popular. Each has its strengths and weaknesses, making them suitable for different use cases. In this post, we’ll explore each of these API paradigms, provide basic examples, and discuss when to choose one over the others.
What is REST API?
Overview
REST (Representational State Transfer) is an architectural style that uses standard HTTP methods to enable communication between clients and servers. RESTful APIs are stateless, meaning each request from a client contains all the information needed to process that request.
Characteristics
Resource-Oriented: REST treats data as resources that can be created, read, updated, and deleted (CRUD).
HTTP Methods: Common methods include GET (retrieve data), POST (create data), PUT (update data), and DELETE (remove data).
Statelessness: Each request is independent; the server does not store client context.
Cacheable: Responses can be cached to improve performance.
Example
Here’s a simple REST API for a resource called "books":
GET /books: Retrieve a list of books.
GET /books/1: Retrieve details of a specific book.
POST /books: Add a new book.
PUT /books/1: Update the book with ID 1.
DELETE /books/1: Remove the book with ID 1.
// Example Response for GET /books
[
{
"id": 1,
"title": "1984",
"author": "George Orwell"
},
{
"id": 2,
"title": "To Kill a Mockingbird",
"author": "Harper Lee"
}
]
What is GraphQL?
Overview
GraphQL is a query language for APIs and a runtime for executing those queries with your existing data. It allows clients to request exactly the data they need, making it more flexible than traditional REST APIs.
Characteristics
Client-Driven Queries: Clients specify the structure of the response, which minimizes over-fetching and under-fetching of data.
Single Endpoint: GraphQL APIs typically use a single endpoint for all requests.
Strongly Typed: GraphQL schemas define the types and relationships of the data, which enhances developer experience and reduces errors.
Example
Using the same "books" example, a GraphQL query might look like this:
{
books {
id
title
author
}
}
The response would be:
{
"data": {
"books": [
{
"id": 1,
"title": "1984",
"author": "George Orwell"
},
{
"id": 2,
"title": "To Kill a Mockingbird",
"author": "Harper Lee"
}
]
}
}
What is gRPC?
Overview
gRPC (gRPC Remote Procedure Calls) is a high-performance, open-source framework developed by Google. It uses HTTP/2 for transport and Protocol Buffers (protobuf) as its interface description language.
Characteristics
Bi-directional Streaming: gRPC supports streaming requests and responses, enabling real-time communication.
Strongly Typed Interfaces: Interfaces are defined using Protocol Buffers, ensuring type safety and clear contract between services.
Performance: gRPC is generally faster than REST due to its binary protocol and HTTP/2 features, such as multiplexing.
Example
Here’s a simple gRPC service definition for the "books" resource:
syntax = "proto3";
service BookService {
rpc GetBooks(Empty) returns (BookList);
}
message Empty {}
message Book {
int32 id = 1;
string title = 2;
string author = 3;
}
message BookList {
repeated Book books = 1;
}
When to Use Each
REST API
Use Cases:
When you need to interact with web browsers or clients that expect standard HTTP methods.
For simple CRUD operations where the resource structure is relatively stable.
When you require stateless interactions, such as public APIs or microservices.
GraphQL
Use Cases:
When you have complex data relationships and clients need flexibility in their queries.
When you want to minimize data transfer by allowing clients to specify exactly what data they need.
For applications where you expect frequent changes to the data structure, as GraphQL's schema can evolve more easily.
gRPC
Use Cases:
When performance and efficiency are critical, such as in microservices that require high throughput and low latency.
For real-time applications where bi-directional streaming is beneficial (e.g., chat applications, live data feeds).
When you have strong typing and versioning needs, as Protocol Buffers allow for structured contracts between services.
Comparison Table: REST vs. GraphQL vs. gRPC
To help you choose the best API for your needs, here’s a quick comparison of REST, GraphQL, and gRPC, highlighting their primary features, use cases, and best-fit scenarios.
Feature | REST | GraphQL | gRPC |
Data Structure | Resource-oriented with CRUD operations | Flexible, client-driven queries | Strongly-typed with Protocol Buffers |
Communication | HTTP/1.1 (text-based) | HTTP/1.1 (text-based) | HTTP/2 (binary) |
End-to-End Speed | Moderate | Moderate | Fast (efficient binary encoding) |
Response Customization | Fixed, all or nothing | Client-specified, tailored responses | Predefined, can’t be customized at runtime |
Caching | Built-in HTTP caching | Needs custom implementation | Needs custom implementation |
Best Use Cases | CRUD, web APIs, public APIs | Complex data needs, mobile/SPA apps | High-performance microservices, real-time needs |
Learning Curve | Easy, widely known | Moderate, requires schema knowledge | Steep, requires Protocol Buffers |
Feature Breakdown
Data Structure: REST is centered around CRUD operations with resources like “users” or “products,” each represented by a URL. In contrast, GraphQL allows clients to specify exactly what data they need, even spanning multiple resources, in a single query. gRPC uses Protocol Buffers, offering a structured, binary format that ensures efficient serialization and strong typing for inter-service communication.
Communication: REST and GraphQL typically use HTTP/1.1 with text-based payloads, making them broadly compatible with web technologies. gRPC, however, is designed for performance with HTTP/2, which supports binary transmission, compression, and multiplexing for higher throughput and lower latency.
End-to-End Speed: REST and GraphQL are generally efficient but can become slow when handling larger, complex requests. gRPC is the fastest due to its binary encoding and HTTP/2 capabilities, making it ideal for high-performance, low-latency scenarios.
Response Customization: REST APIs provide fixed response structures for each endpoint, meaning clients receive either all available data or none. GraphQL’s flexibility allows clients to request exactly the fields they need, which can reduce bandwidth usage. gRPC has predefined responses that, while fast, cannot be tailored dynamically at runtime.
Caching: REST integrates well with standard HTTP caching, simplifying performance optimization. GraphQL and gRPC, on the other hand, lack native caching and typically require custom implementation.
Best Use Cases
REST: Its simplicity and extensive support make REST ideal for basic CRUD operations, web and mobile applications, and publicly accessible APIs.
GraphQL: When applications need complex data retrieval, flexibility in response shape, or minimal data transfer (like single-page applications), GraphQL’s query language and schema make it an ideal choice.
gRPC: For microservices, high-throughput systems, and real-time applications that demand bi-directional communication (e.g., live data feeds, chat applications), gRPC offers optimal speed, type-safety, and performance.
Learning Curve
REST is straightforward and widely adopted, making it easy for most developers to start using immediately.
GraphQL has a moderate learning curve due to the need for schema design and understanding client-driven queries.
gRPC requires knowledge of Protocol Buffers and a familiarity with HTTP/2, making it the steepest learning curve but rewarding for performance-focused applications.
This table provides a clear overview to guide your choice of API based on the requirements and constraints of your project, enabling you to build efficient, scalable systems suited to your needs.
Conclusion
Choosing between GraphQL, REST, and gRPC depends on your specific use case, performance needs, and team expertise. REST APIs are widely adopted and easy to use, making them a great choice for simple applications. GraphQL offers flexibility and efficiency, making it suitable for complex, client-driven applications. gRPC excels in performance and real-time scenarios, ideal for microservices and high-volume data exchanges.
By understanding the strengths and limitations of each API type, you can make informed decisions that align with your project goals and technical requirements.
Subscribe to my newsletter
Read articles from JealousGx directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
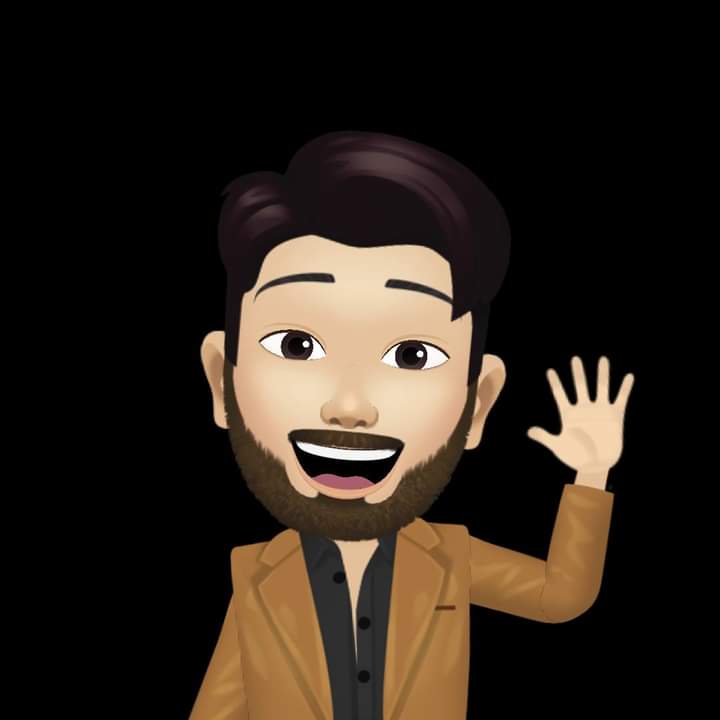
JealousGx
JealousGx
Hello, I'm a highly skilled full stack web developer with a rich background in creating and maintaining dynamic websites that drive online engagement and brand awareness. My expertise extends beyond WordPress to include a versatile skill set.