Docker for DevOps Engineers
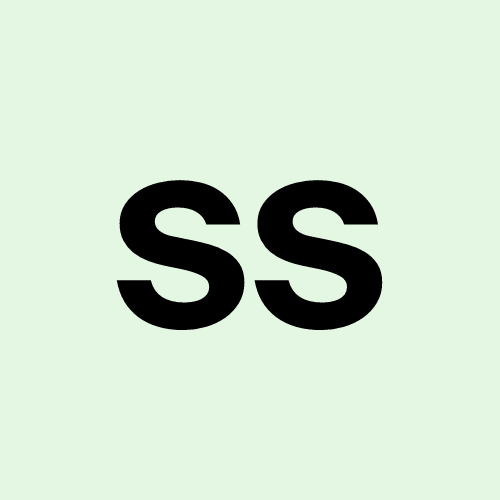
Table of contents
- What is Docker?
- Essential Docker Commands
- Pulling Docker Images with docker pull
- Listing Images with docker images
- Starting a New Container with docker run
- Listing Containers with docker ps
- Entering a Running Container with docker exec
- Inspecting a Container or Image with docker inspect
- Listing Port Mappings with docker port
- Monitoring Resource Usage with docker stats
- Viewing Processes in a Container with docker top
- Saving Docker Images with docker save
- Loading Docker Images with docker load
- Removing Images with docker rmi
- Stopping Containers with docker stop
- Starting Containers with docker start
- Restarting Containers with docker restart
- Killing Containers with docker kill
- Removing Containers with docker rm
- Cleaning Up Unused Resources with docker system prune
- Conclusion
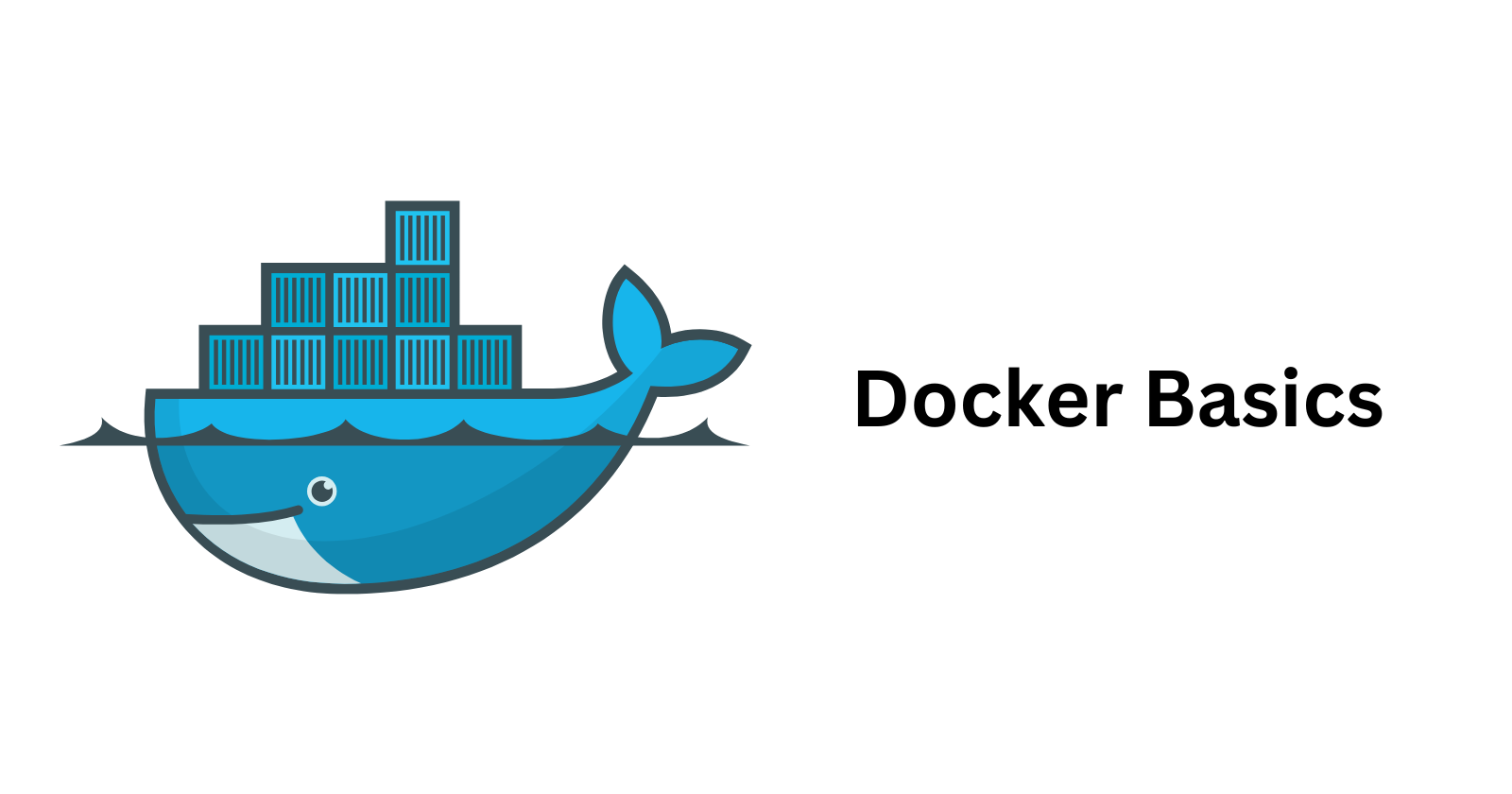
Hello, DevOps enthusiasts! Today, we’re diving into Docker, one of the essential tools for containerizing applications and streamlining deployments. Docker allows you to create isolated environments, known as containers, which makes building, testing, and deploying applications smoother, faster, and more consistent.
This blog will introduce you to Docker, why it’s valuable for DevOps, and guide you through some fundamental Docker commands to manage containers and images.
What is Docker?
Docker is a platform that packages applications into containers: self-contained units that include everything required to run the application: libraries, system tools, code, and runtime. Think of Docker as a consistent environment across different systems, where your code runs as expected, whether in development, testing, or production.
Why Docker?
Consistency: Docker ensures your code runs the same way everywhere by isolating it in containers.
Scalability: You can scale your applications horizontally with ease.
Portability: Containers can run on any system with Docker installed, making deployment straightforward.
Efficiency: Containers are lightweight and use fewer resources than traditional virtual machines.
Essential Docker Commands
Let's jump into some practical tasks to solidify your understanding of Docker. The following commands cover container management, resource monitoring, and handling Docker images.
Pulling Docker Images with docker pull
The docker pull
command is used to download images from Docker Hub (or other configured registries) to your local system.
Example:
docker pull nginx
Explanation: This command fetches the latest nginx image from Docker Hub and stores it on your local machine. It’s useful when you need a specific image to work with or when starting a new project.
Sample Output:
latest: Pulling from library/nginx
...
Status: Downloaded newer image for nginx:latest
Listing Images with docker images
The docker images
command displays all images stored locally on your system, along with details like the repository, tag, and size.
Example:
docker images
Explanation: This command is useful for managing local images, especially when you want to see what images you have downloaded or built.
Sample Output:
REPOSITORY TAG IMAGE ID CREATED SIZE
java-app latest 7a1a83dd9da4 10 days ago 326MB
mysql latest be960704dfac 2 weeks ago 602MB
nginx latest 3b25b682ea82 4 weeks ago 192MB
Starting a New Container with docker run
The docker run
command is used to start a new container from an image. It fetches the image if it’s not present locally.
Example:
docker run hello-world
Explanation: This command downloads the hello-world
image and starts a container. It’s a quick way to verify that Docker is correctly installed and functioning.
Sample Output:
Hello from Docker!
This message shows that your installation appears to be working correctly.
Listing Containers with docker ps
The docker ps
command lists all currently running containers. Adding -a
shows all containers, both running and stopped.
Example:
docker ps # Lists only running containers
docker ps -a # Lists all containers, including stopped ones
Explanation: This command is essential for checking the status of containers, along with their IDs, image names, uptime, and ports.
Sample Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
ee5d95fadbbf nginx:latest "/docker-entrypoint.…" 10 days ago Up 10 days 0.0.0.0:80->80/tcp, :::80->80/tcp gracious_galileo
Entering a Running Container with docker exec
The docker exec
command lets you run commands directly within a running container. Use it to start an interactive session.
Example:
docker exec -it <container-id> /bin/bash
Explanation: This command opens a shell inside the container, letting you troubleshoot, configure, or inspect files in the container directly.
Sample Output:
root@1a2b3c4d5e6f:/#
Inspecting a Container or Image with docker inspect
The docker inspect
command retrieves detailed information about a specific container or image, providing details such as configuration, networking, and volume data.
Example:
docker inspect hello-world
Explanation: This command returns detailed information about the hello-world
image or any container you specify, useful for checking environment variables, network settings, and mount paths.
Sample Output (truncated):
[
{
"Id": "sha256:hello-world",
"Config": {
"Hostname": "hello-world",
"Env": null,
"ExposedPorts": null,
...
},
"NetworkSettings": {
...
}
}
]
Listing Port Mappings with docker port
Docker allows you to map container ports to host ports, making applications accessible from outside the container. The docker port
command displays the port mappings for a specific container.
Example:
docker run -d -p 8080:80 nginx
docker port <container-id>
Explanation: This command starts an Nginx server, mapping the container’s port 80 to host port 8080. Using docker port <container-id>
shows these mappings, helping you verify how the container is accessible from the host.
Sample Output:
80/tcp -> 0.0.0.0:8080
Monitoring Resource Usage with docker stats
docker stats
provides real-time resource usage statistics for running containers, showing CPU, memory, and network usage.
Example:
docker stats
Explanation: Running docker stats
displays a dashboard of resource usage for all running containers, making it easy to monitor performance and resource allocation.
Sample Output:
CONTAINER ID NAME CPU % MEM USAGE / LIMIT NET I/O BLOCK I/O PIDS
a1b2c3d4e5 nginx 0.08% 3.5MiB / 500MiB 2.1kB / 3.1kB 0B / 0B 2
Viewing Processes in a Container with docker top
The docker top
command shows all processes running inside a specific container.
Example:
docker run -d nginx
docker top <container-id>
Explanation: This command gives you a view of the processes inside the container, similar to the top
command on Linux, helping you check which processes are active within a container.
Sample Output:
UID PID PPID C STIME TTY TIME CMD
root 23217 23196 0 Oct20 ? 00:00:00 nginx: master process nginx -g daemon off;
message+ 23262 23217 0 Oct20 ? 00:00:00 nginx: worker process
Saving Docker Images with docker save
To transfer or archive a Docker image, you can save it as a tar file. The docker save
command packages an image and its layers into a tar archive, useful for sharing images offline or moving them between systems.
Example:
docker save -o nginx_backup.tar nginx
Explanation: This command saves the nginx
image into a file named nginx_backup.tar
, which can then be shared or stored as a backup.
Loading Docker Images with docker load
After saving an image as a tar file, you can load it onto any Docker system with the docker load
command.
Example:
docker load -i nginx_backup.tar
Explanation: This command loads the nginx_backup.tar
image into the Docker registry on your system, making it available for creating containers. You can use this command on any system, making image transfer hassle-free.
Sample Output:
Loaded image: nginx:latest
Removing Images with docker rmi
Use docker rmi
to delete unused or unnecessary images from your system to free up space.
Example:
docker rmi <image-id>
Explanation: This command removes the specified image by ID, so only keep images you actively need. If a container is using the image, you’ll need to stop and remove it first.
Stopping Containers with docker stop
The docker stop
command gracefully stops a running container by sending a SIGTERM signal, allowing processes within the container to shut down properly.
Example:
docker stop <container-id>
Explanation: This command stops the specified container without immediately killing it, giving it a chance to terminate processes gracefully.
Sample Output:
<container-id> stopped
Starting Containers with docker start
docker start <container-id>
Explanation: This command starts a container that was previously stopped, resuming its processes exactly where they left off.
Restarting Containers with docker restart
The docker restart
command combines stop
and start
in one command, making it easy to restart a container in one step.
Example:
docker restart <container-id>
Explanation: Restarting a container is often needed when updating configurations or applying new code changes without creating a new container.
Killing Containers with docker kill
If you need to stop a container immediately, you can use the docker kill
command. This command sends a SIGKILL signal to the container, terminating it abruptly.
Example:
docker kill <container-id>
Explanation: This command forces the specified container to stop immediately, which can be useful in cases where a container is unresponsive.
Sample Output:
<container-id> killed
Removing Containers with docker rm
To clean up your system, you can remove stopped containers using the docker rm
command.
Example:
docker rm <container-id>
Explanation: This command removes the specified container from your local Docker environment, freeing up resources. Be cautious, as this command permanently deletes the container.
Sample Output:
<container-id> removed
Cleaning Up Unused Resources with docker system prune
docker system prune
is a cleanup command that removes stopped containers, unused networks, dangling images, and build cache.
Example:
docker system prune
Explanation: Run this command to free up space, especially after testing or experimenting with multiple containers. Use with caution, as it permanently deletes unused resources.
Sample Output:
Deleted Containers:
1a2b3c4d5e6f
7g8h9i0j1k2l
Deleted Images:
sha256:abc123def456
sha256:789abc456def
Deleted Networks:
my_network
Total reclaimed space: 500MB
Conclusion
Docker is a powerful tool for DevOps engineers, providing an isolated environment for your applications and making deployments more reliable. Mastering Docker commands can help you automate deployment tasks, optimize resource usage, and easily manage containers and images.
Key Takeaways:
docker pull
fetches images from Docker Hub to your local system.docker images
– View downloaded images.Use
docker run
to start containers from images.docker ps
– List running containers.docker exec
– Run commands inside a container.docker inspect
provides detailed insights into containers and images.docker port
shows port mappings, making container access easy to manage.docker stats
helps you monitor container resource usage in real-time.docker top
gives visibility into processes within containers.docker save
anddocker load
allow you to export and import Docker images as tar files, simplifying image management.docker rmi
– Remove an image by ID.docker start/stop
– Start or stop containers.docker restart
– Restart a container.Use
docker kill
to immediately terminate unresponsive containers.Use
docker rm
to remove containers and free up resources.docker system prune
– Clean up unused resources.
With these commands in your toolkit, you’re set to explore Docker’s potential in DevOps, from container management to resource optimization. Keep experimenting with Docker, and you’ll quickly see why it’s a go-to tool in DevOps engineering!
Happy Docker-ing! 🐳
Subscribe to my newsletter
Read articles from Spoorti Shetty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
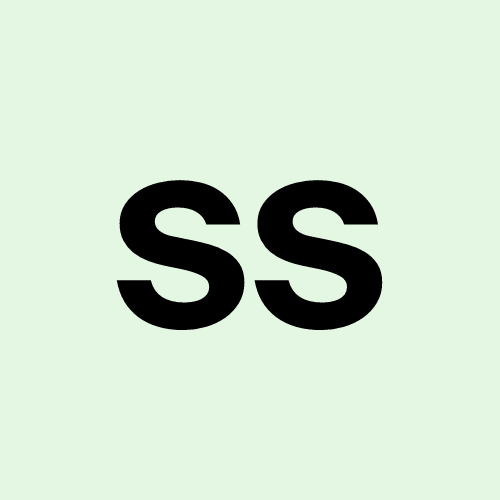