Understanding AWS Config: Ensuring Resource Compliance in AWS
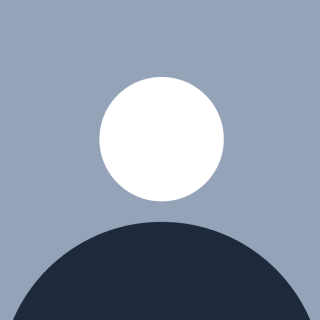
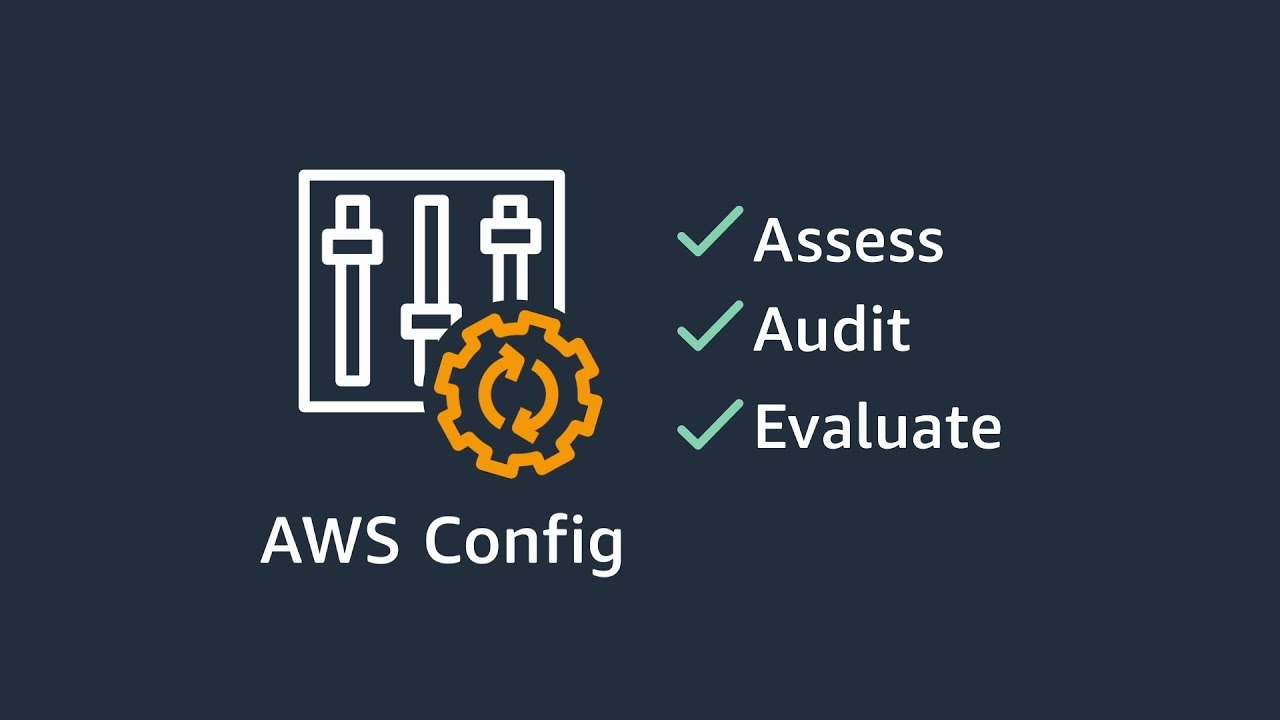
AWS Config is a powerful service that helps organizations maintain compliance and security standards across their AWS resources. In this comprehensive guide, we'll explore how AWS Config works, its practical implementation, and how to create custom compliance rules using Lambda functions.
What is AWS Config?
AWS Config is a service that enables you to assess, audit, and evaluate the configurations of your AWS resources. It helps ensure that your resources comply with organizational rules and regulations. This is particularly crucial for:
Government projects
Banking sector applications
Financial institutions
Organizations with strict security requirements
Why Use AWS Config?
Organizations often have specific compliance requirements for their cloud resources. For example:
All S3 buckets must have lifecycle management enabled
EC2 instances must have detailed monitoring enabled
All resources must have specific tags
Public access must be disabled for certain resources
Manually tracking compliance across hundreds or thousands of resources is impractical. AWS Config automates this process by continuously monitoring and evaluating resource configurations.
Practical Implementation: A Real-World Example
Let's walk through implementing a custom compliance rule that checks if EC2 instances have detailed monitoring enabled.
Step 1: Creating a Custom Rule
Navigate to AWS Config in the console
Click on "Rules" → "Add rule"
Choose "Create custom Lambda rule"
Configure the rule:
Provide a name and description
Specify the Lambda function ARN
Choose trigger type (configuration changes or periodic)
Select resources to monitor (EC2 instances in our case)
Step 2: Implementing the Lambda Function
Here's a Python Lambda function that checks EC2 instance monitoring status:
import boto3
import json
def lambda_handler(event, context):
# Initialize boto3 client
ec2_client = boto3.client('ec2')
# Default compliance status
compliance_status = 'COMPLIANT'
try:
# Extract instance ID from the AWS Config event
configuration_item = json.loads(event['invokingEvent'])['configurationItem']
instance_id = configuration_item['resourceId']
# Get instance details
response = ec2_client.describe_instances(InstanceIds=[instance_id])
instance = response['Reservations'][0]['Instances'][0]
# Check monitoring state
if instance['Monitoring']['State'] != 'enabled':
compliance_status = 'NON_COMPLIANT'
# Prepare evaluation response
evaluation = {
'ComplianceResourceType': 'AWS::EC2::Instance',
'ComplianceResourceId': instance_id,
'ComplianceType': compliance_status,
'OrderingTimestamp': configuration_item['configurationItemCaptureTime']
}
# Update compliance status in AWS Config
config = boto3.client('config')
config.put_evaluations(
Evaluations=[evaluation],
ResultToken=event['resultToken']
)
except Exception as e:
print(f"Error: {str(e)}")
raise e
return {
'statusCode': 200,
'body': json.dumps('Evaluation complete')
}
Step 3: Required IAM Permissions
The Lambda function needs specific permissions to interact with AWS services. Create an IAM role with these policies:
CloudWatchFullAccess
EC2FullAccess
AWSConfigRole
CloudTrailFullAccess
Note: For production environments, you should follow the principle of least privilege and restrict these permissions to only what's necessary.
How It Works
When an EC2 instance is created or modified, AWS Config detects the change
It triggers the Lambda function, passing the instance details
The Lambda function:
Retrieves the instance ID from the event
Checks if detailed monitoring is enabled
Updates the compliance status in AWS Config
AWS Config dashboard shows compliant and non-compliant resources
Best Practices
Start with AWS Managed Rules: AWS provides many pre-built rules. Use these before creating custom rules.
Custom Rules for Specific Needs: Create custom rules only for organization-specific requirements.
Proper Error Handling: Implement robust error handling in Lambda functions.
Least Privilege Access: Grant minimum required permissions to Lambda functions.
Regular Monitoring: Regularly review compliance reports and address non-compliant resources.
Extending the Implementation
You can extend this pattern to monitor other AWS resources and compliance requirements:
S3 bucket configurations
RDS instance settings
IAM policy compliance
Security group rules
Resource tagging standards
Conclusion
AWS Config is a vital tool for maintaining security and compliance in AWS environments. By automating compliance checking, organizations can ensure their resources consistently meet required standards and quickly identify and remediate any deviations.
Remember to customize the compliance rules based on your organization's specific requirements and regulatory needs. Regular monitoring and updates to these rules ensure your AWS infrastructure remains secure and compliant.
Tags: AWS, DevOps, Security, Compliance, Lambda, Python, Cloud Computing
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by