Introducing Validator Forge - Simplified Form Validation for Flutter
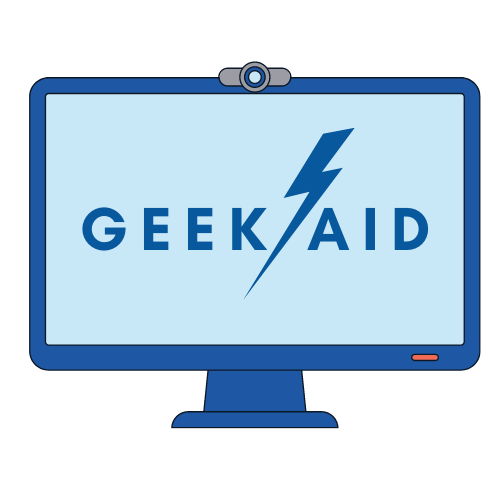
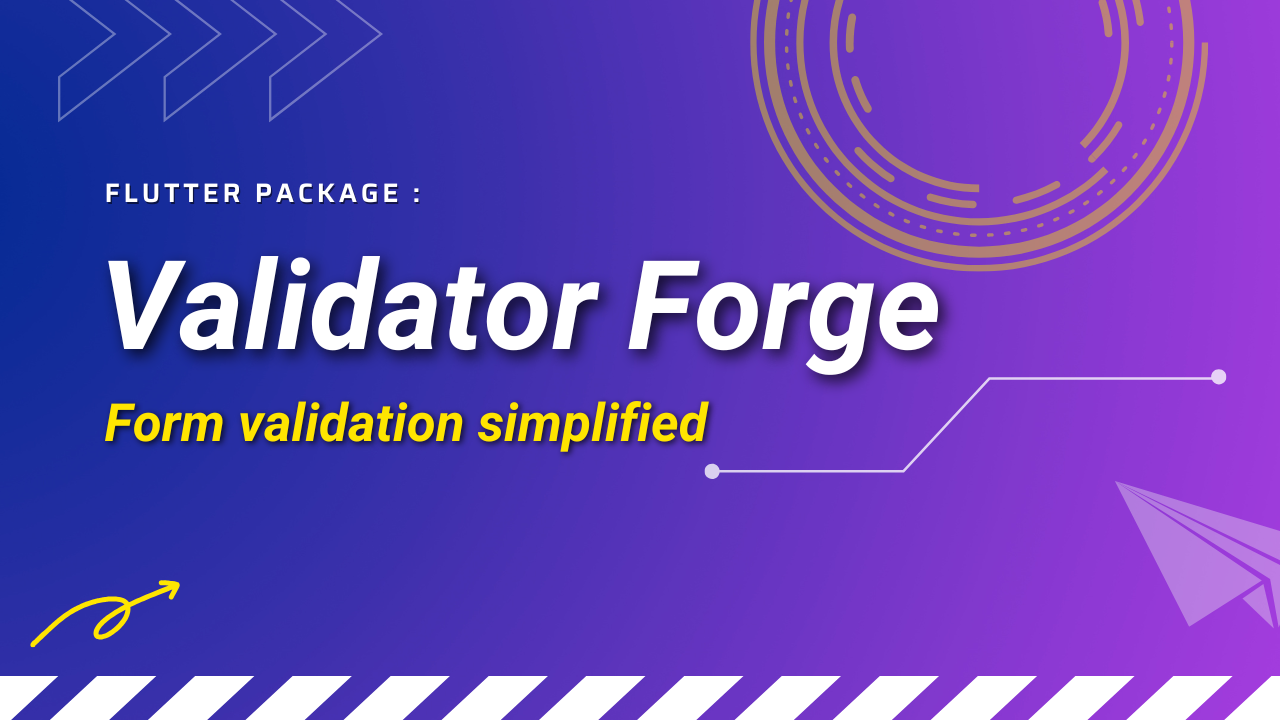
Form validation is essential for ensuring data accuracy and improving the user experience in tech product development. As a Flutter developer, I often use the built-in form features and the reactive_forms package. While reactive_forms has great validation tools, some of its other features are lacking. This led me to create Validator Forge, a lightweight and easy-to-use package that simplifies form validation in Flutter apps.
Why Validator Forge?
Writing the same validation logic over and over can be tiring. For example:
validator: (value) {
if (value == null || value.isEmpty) {
return "Required";
}
return null;
}
With Validator Forge, you can avoid repetitive code, allowing you to focus more on building great apps.
Key Features of Validator Forge
Simple and Efficient
Validator Forge has an easy-to-use API for common validations. Whether you need to validate required fields, emails, phone numbers, URLs, or custom patterns, you can do it quickly using the built-in validators.Comprehensive Validators
The package includes validators for:Required Fields: Make sure users don’t leave important fields empty.
Email Validation: Ensures email addresses are correctly formatted.
Phone Number Validation: Verifies 10-digit phone numbers.
URL Validation: Checks for valid URLs.
Custom Patterns: Use your regular expressions for custom validations.
Numeric Validation: Validates numbers with minimum and maximum values.
Length Validation: Controls the minimum and maximum length of input.
Custom Error Messages
You can easily customize error messages, giving users clear feedback on what needs fixing.Easy to Integrate
Adding Validator Forge to your Flutter project is simple. Just include it in yourpubspec.yaml
file like this:
yaml
Copy code
dependencies:
validator_forge: ^1.0.0
Example Usage
Here’s an example of how to use Validator Forge in a Flutter app:
dart
Copy code
validator: (String? value) {
final amount = num.tryParse(value ?? '');
final error = validatorBuilder([
() => Validators.requiredValidator(value),
() => Validators.minimum(amount, min: 5000),
() => Validators.maximum(amount, max: 5000000),
]);
return error;
}
Open Source Contribution
Validator Forge is open source! I welcome contributions, suggestions, and feedback from other developers. Together, we can make this package even better.
Conclusion
If you're looking for a simple and effective way to manage form validations in Flutter, try Validator Forge! It’s designed to make your work easier and help you build better apps.
You can find the package on pub.dev and contribute on GitHub.
I appreciate your support and happy coding!
Subscribe to my newsletter
Read articles from Geek Aid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
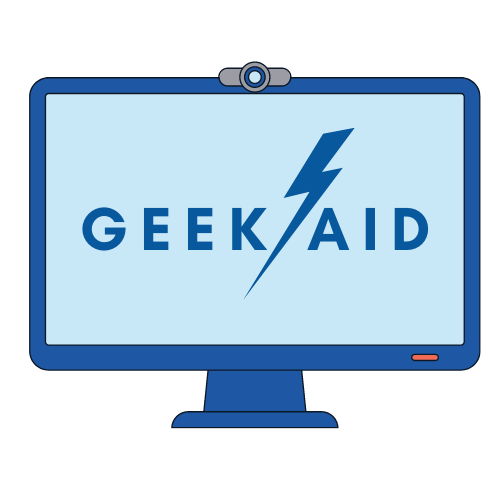