Babel is a popular JavaScript compiler

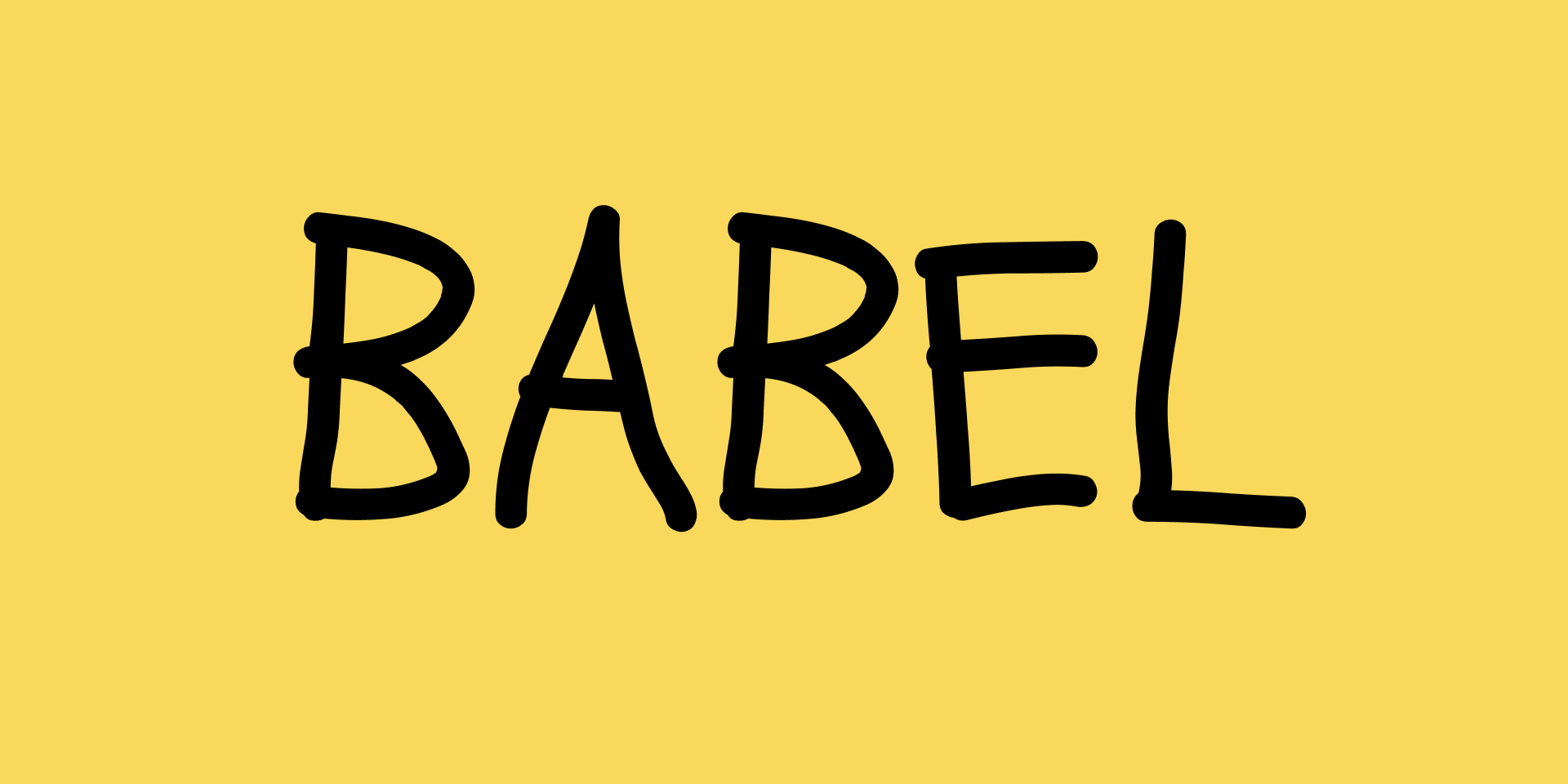
Babel is a popular JavaScript compiler used to transform modern JavaScript (ES6+ and beyond) into versions compatible with older browsers or environments that don’t support certain language features. It allows developers to write the latest JavaScript features without worrying about backward compatibility.
Key Features of Babel:
Transpiling ES6+ to ES5: Babel converts modern JavaScript code (like const, let, arrow functions, classes, etc.) into older versions (usually ES5) that older browsers (like Internet Explorer) can understand.
Polyfills: Babel can automatically include polyfills for new JavaScript features (e.g., Promise, Map, Set), making it easier to use newer APIs even in environments that don’t support them natively.
Plugins: Babel’s functionality is extended through plugins. Plugins are responsible for transforming specific syntax, such as arrow functions or destructuring.
- Example plugins include: - @babel/plugin-transform-arrow-functions: Converts ES6 arrow functions into regular functions. - @babel/plugin-transform-classes: Converts ES6 classes into constructor functions.
Presets: A preset is a collection of Babel plugins that enable specific transformations. For example:
- @babel/preset-env: This is the most commonly used preset that automatically determines which JavaScript features to transpile based on your target environment (e.g., browsers or Node.js).
Code Transformations: Babel can transform new language features like async/await, modules, and even JSX (used in React) into equivalent ES5 code.
Custom Configuration: Babel is highly configurable through a .babelrc file or a babel.config.js file. You can specify which plugins and presets to use, or configure Babel based on the environment (e.g., different setups for production vs. development).
Basic Babel Configuration Example (.babelrc):
- A .babelrc file is where Babel’s configuration resides. Here’s a simple example:
{
"presets": ["@babel/preset-env"]
}
This configuration uses the @babel/preset-env preset, which determines the necessary transformations based on the environment you want to support.
How Babel Works:
Parse: Babel first parses the JavaScript code into an abstract syntax tree (AST).
Transform: It applies transformations (based on plugins) to the AST.
Generate: It generates new JavaScript code from the transformed AST.
Example of Babel in Action
Suppose you have modern JavaScript code like this:
const greet = (name) => {
console.log(`Hello, ${name}!`);
};
If you configure Babel to target older browsers, this might be transpiled to:
var greet = function(name) {
console.log("Hello, " + name + "!");
};
Common Babel Use Cases:
Compatibility for Older Browsers: Babel is frequently used in projects to ensure compatibility with browsers that don’t support the latest JavaScript features.
React (JSX Transformation): Babel can transform JSX, the XML-like syntax used in React, into regular JavaScript. To do this, you’d use the @babel/preset-react preset:
{ "presets": ["@babel/preset-env", "@babel/preset-react"] }
Using ES6+ Features in Node.js: For projects running on Node.js that need to support older Node versions, Babel can ensure new language features are transpiled to work with those environments.
Setup in a Project:
Install Babel: To use Babel in your project, you would first need to install Babel and the necessary presets or plugins via npm: npm install —save-dev @babel/core @babel/preset-env
Add Configuration: In your .babelrc or babel.config.js:
{ "presets": ["@babel/preset-env"] }
Using Babel CLI: If you want to transpile a file manually using Babel, you can use the Babel CLI:
npx babel src --out-dir lib
Integrating Babel with Webpack or Rollup: When used in a larger project, Babel is often integrated with Webpack or Rollup. Here’s an example of using Babel with Webpack:
- webpack.config.js:
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: __dirname + '/dist'
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
}
]
}
};
In this setup, Webpack uses the babel-loader to process JavaScript files using Babel.
Common Plugins and Presets:
@babel/preset-env: The most popular preset that allows you to transpile ES6+ into compatible versions based on your target environment.
@babel/plugin-transform-runtime: Reduces code duplication in Babel’s output, especially for polyfills.
@babel/preset-react: Allows Babel to understand and transpile JSX.
@babel/plugin-proposal-class-properties: Enables support for class properties, a newer feature in JavaScript.
Advantages of Babel:
Cross-Browser Compatibility: It ensures that your modern JavaScript code can run in older environments, making it crucial for web development.
Supports New JavaScript Syntax: Allows developers to use the latest language features without waiting for all browsers to catch up.
Extensible with Plugins: Babel can be extended with a variety of plugins to handle specific transformations, like JSX for React or TypeScript.
Conclusion:
Babel is an essential tool for modern JavaScript development, helping developers write cutting-edge JavaScript without worrying about compatibility issues. It’s often integrated with other build tools like Webpack or Rollup to create optimized and backward-compatible JavaScript bundles.
Subscribe to my newsletter
Read articles from kietHT directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

kietHT
kietHT
I am a developer who is highly interested in TypeScript. My tech stack has been full-stack TS such as Angular, React with TypeScript and NodeJS.