Understanding Object-Oriented Programming in Ballerina
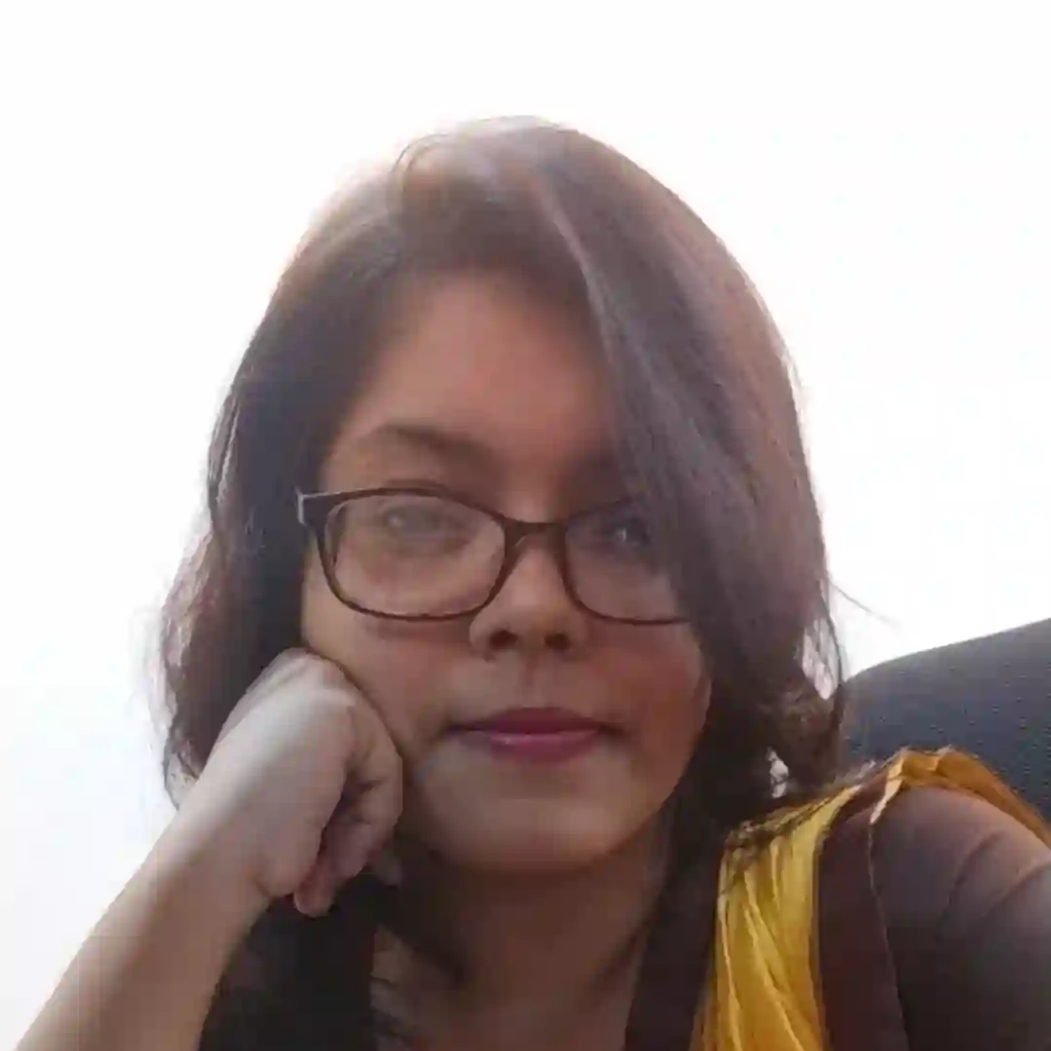
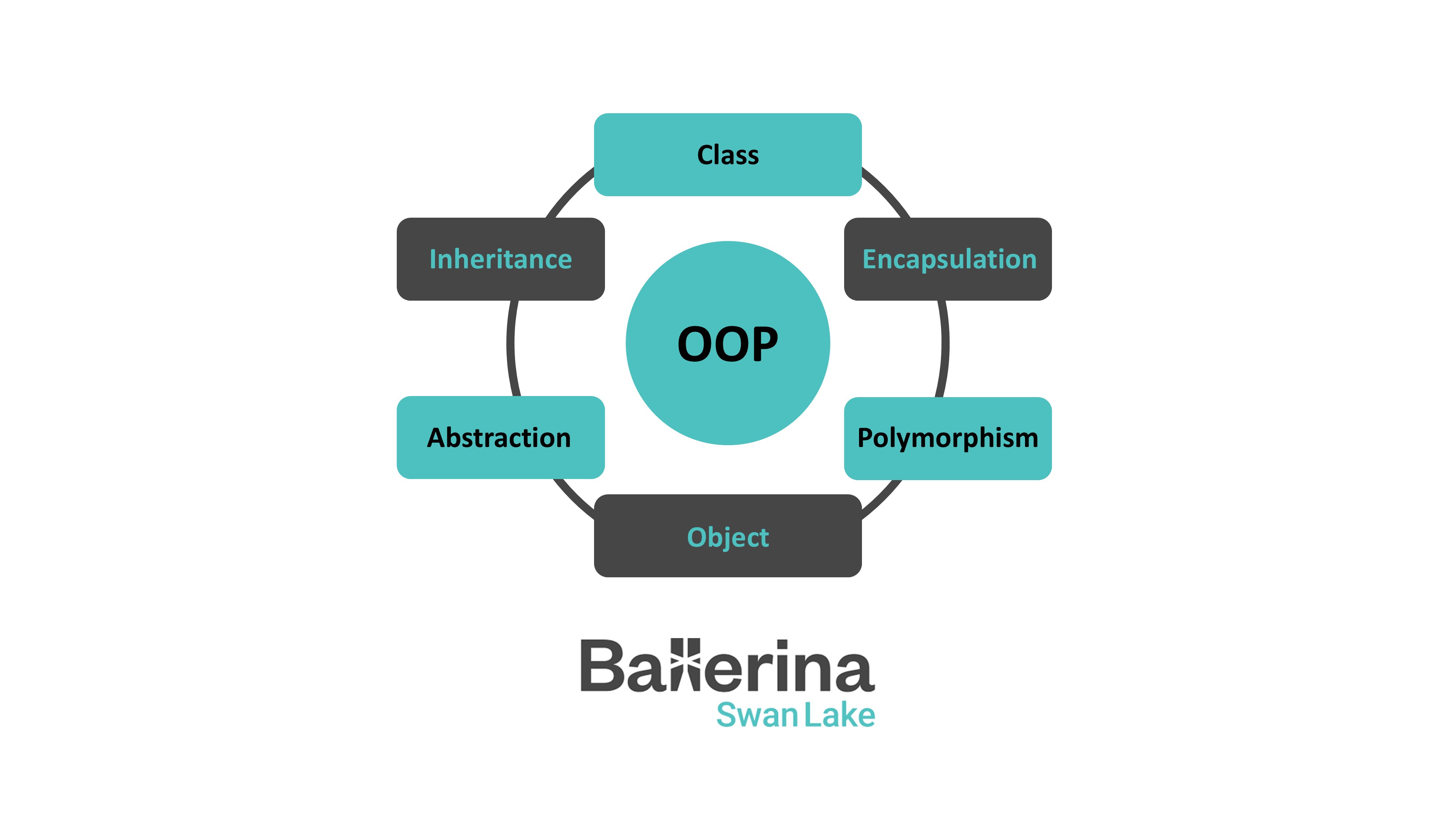
Introduction to OOP:
Object-Oriented Programming (OOP) is a way of programming that organizes code into "objects" with data and actions. The main goal of OOP is to link data and the functions that work on it, so only those specific functions can access the data, keeping it protected from other parts of the code. Ballerina, a modern programming language designed for cloud-native applications, supports OOP concepts, making it easier for developers to manage complex systems.
Key Concepts of OOP in Ballerina:
Class: In Ballerina, a class is a blueprint for creating objects. It encapsulates data and methods that operate on that object. Here’s how you can define a simple class:
import ballerina/io;
class Person {
string name;
int age;
// Constructor
public function init(string name, int age) {
self.name = name;
self.age = age;
}
// Method to introduce the person
public function introduce() {
io:println("Hello, my name is ", self.name, " and I am ", self.age, " years old.");
}
};
Object: It is a basic unit of Object-Oriented Programming and represents the real-life entities. An Object is an instance of a Class. Defining a class doesn’t allocate memory, but when the class is instantiated (an object is created), memory is then allocated.
// Creating an object of the Person class
Person person1 = new("Alice", 30);
person1.introduce();
Inheritance: Inheritance is a key concept in Object-Oriented Programming, allowing a class to inherit properties and features from another class. Ballerina supports inheritance, allowing you to create new classes based on existing ones.
// Derived class
class Student {
*Person;
int studentID;
// Constructor
public function init(string name, int age, int studentID) {
// Call the parent constructor
self.name = name;
self.age = age;
self.studentID = studentID;
}
// Overriding the introduce method
public function introduce() {
io:println("I am a student with ID: ", self.studentID, " and my name is ", self.name);
}
};
You can create a Student object that inherits properties and methods from the Person class:
// Creating an object of the Student class
Student student1 = new("Bob", 20, 12345);
student1.introduce();
Encapsulation: Encapsulation is the mechanism that binds together code and the data it manipulates. It restricts access to some components. You can use access modifiers to control visibility:
class Account {
private int balance = 0;
public function deposit(int amount) {
self.balance += amount;
}
public function getBalance() returns int {
return self.balance;
}
};
Abstraction: Abstraction in Object-Oriented Programming is the concept of hiding complex details and showing only the essential features of an object. The derived class must implement the abstract function of the abstract class.
// Abstract class
class Animal {
public function activity() {
}
};
// Derived class
class Dog {
*Animal;
public function activity() {
io:println("Running");
}
};
Polymorphism: Polymorphism means "many forms." Simply put, it refers to the ability for a message to be presented in different ways. Ballerina supports method overriding to achieve polymorphism.
// Overriding the introduce method
public function introduce() {
io:println("I am a student with ID: ", self.studentID, " and my name is ", self.name);
}
Putting It All Together:
Here’s a complete example that demonstrates OOP concepts in Ballerina:
import ballerina/io;
class Person {
string name;
int age;
// Constructor
public function init(string name, int age) {
self.name = name;
self.age = age;
}
// Method to introduce the person
public function introduce() {
io:println("Hello, my name is ", self.name, " and I am ", self.age, " years old.");
}
};
// Derived class
class Student {
*Person;
int studentID;
// Constructor
public function init(string name, int age, int studentID) {
// Call the parent constructor
self.name = name;
self.age = age;
self.studentID = studentID;
}
// Overriding the introduce method
public function introduce() {
io:println("I am a student with ID: ", self.studentID, " and my name is ", self.name);
}
};
class Account {
private int balance = 0;
public function deposit(int amount) {
self.balance += amount;
}
public function getBalance() returns int {
return self.balance;
}
};
// Abstract class
class Animal {
public function activity() {
}
};
// Derived class
class Dog {
*Animal;
public function activity() {
io:println("Running");
}
};
// Main function
public function main() {
// Creating an object of the Person class
Person person1 = new ("Alice", 30);
person1.introduce();
// Creating an object of the Student class
Student student1 = new ("Bob", 20, 12345);
student1.introduce(); // Output: I am a student with ID: 12345 and my name is Bob.
}
Conclusion:
Ballerina provides a powerful way to implement OOP principles. By using classes, objects, inheritance, encapsulation, and polymorphism, you can build strong cloud-native applications, improving the structure and functionality of your microservices or APIs.
Subscribe to my newsletter
Read articles from Faria Karim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
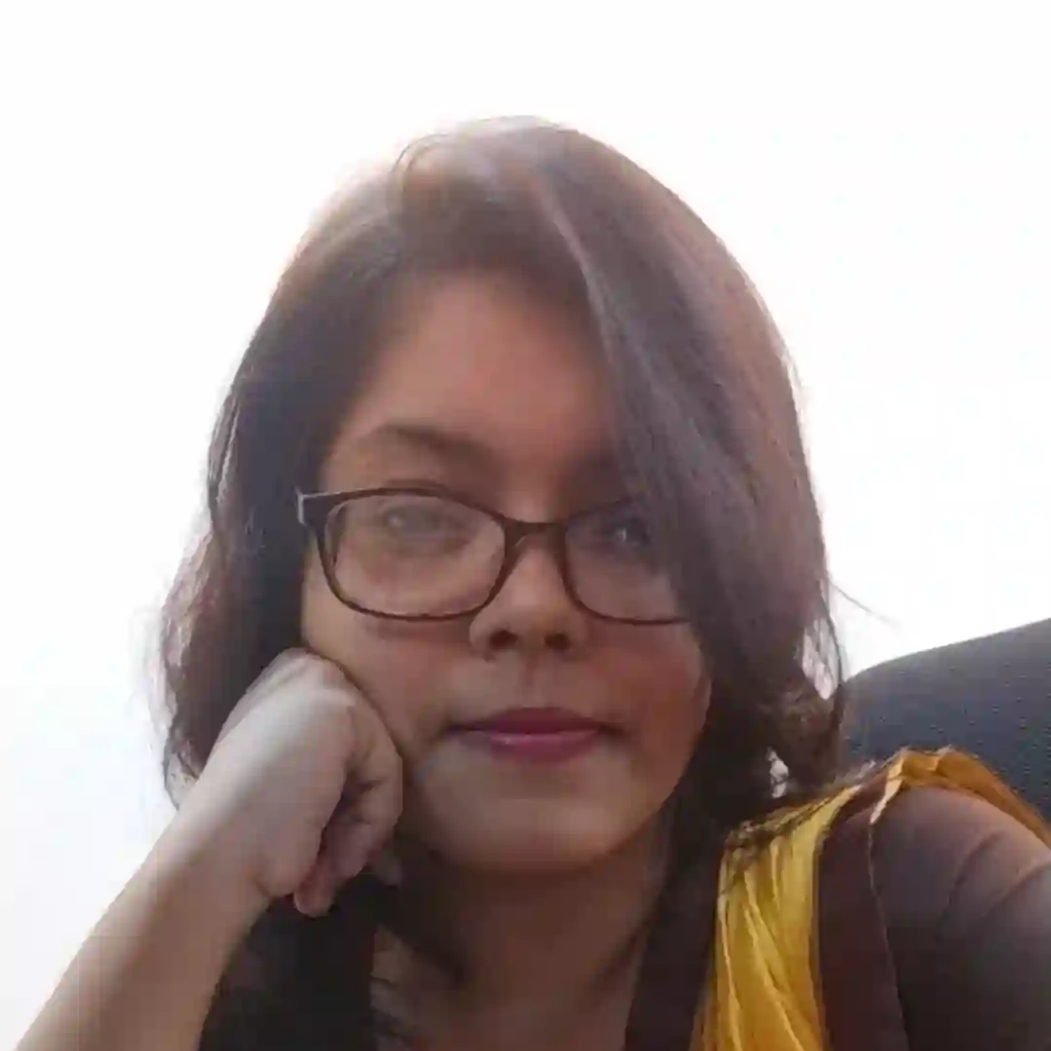
Faria Karim
Faria Karim
Currently, I am working as an "Associate Software Engineer" at "Kaz Software". I have completed my graduation from North South University in the year 2020 with the highest distinction. I always love to explore new ideas. I am a curious and self-motivated person. So, I always try to keep learning and share my ideas with other people. I enjoy problem-solving a lot. I have solved around 100+ problems in various online judges and I am also a three-star coder at Hackerrank. Moreover, I have participated in the ACM ICPC two times. I have completed the off-sight round of ACM ICPC of 2018. I have some basic knowledge of c/c++, Java, Javascript, Php, and Python. I have done several projects using these programming languages. I have hands-on experience on various backend frameworks like Node.js, Django, Flask, and Laravel. I have also used React.js as the front-end library for a few of my projects. Currently, I am more into React.js. For web-based projects, I have worked on both MySQL and NoSQL (MongoDB) as the database system. Machine learning is my another matter of interest. I have research experience in the fuzzy system. I have done a few projects using Tensorflow, Keras, CNN, and LSTM. Recently I have grown my interest in game development. I have built two games. One is using construct 2 and the another one is in Unity 3D. Side by side, I am also trying to figure out the things on ionic. In my free time, I mostly like to surf the internet, watch movies, and also love to do animations and illustrations using Powerpoint.