🚀 Day 12 of #30DaysOfJavaScript: Exploring Async and Await in JavaScript
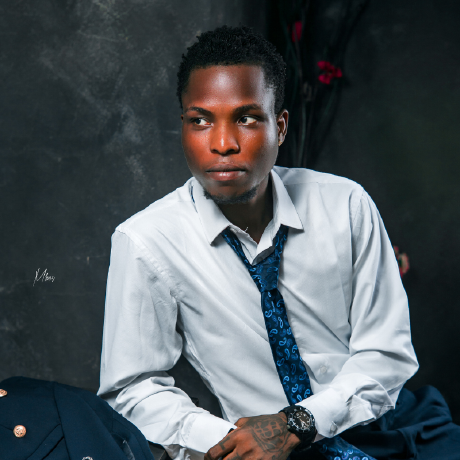
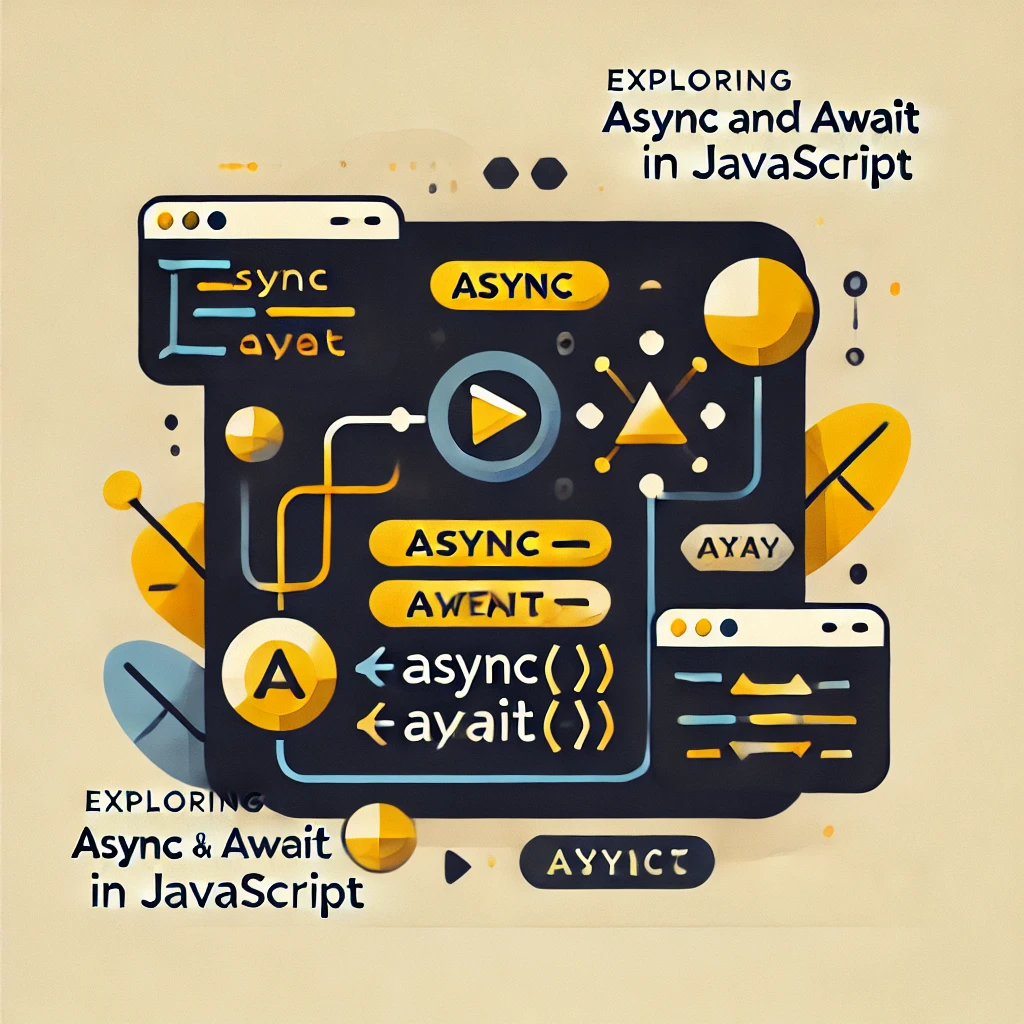
Today, we dove into the world of async and await—a key part of handling asynchronous code in JavaScript. If you're wondering what asynchronous code is and why it's so crucial, let's break it down.
🌐 What is Asynchronous Code?
Asynchronous code allows JavaScript to start tasks that take time, like fetching data from a server, without waiting for them to finish. Instead, it “sets them aside” and continues with other tasks. This is crucial for keeping apps responsive, especially when dealing with network requests or large calculations.
Without an async code, your program would freeze up every time it had to wait, frustrating users.
🚀 Enter Async and Await
Async and await are newer keywords in JavaScript, introduced to simplify working with asynchronous operations. They make async code look more like “normal” synchronous code, which is easier to read and understand.
💡 Getting Started with Async and Await
Async Functions: By adding
async
before a function, JavaScript knows that it will contain asynchronous code.async function getData() { // async code here }
Await: Inside an async function,
await
pauses the function until the awaited task is completed. This allows us to directly work with the final result without chaining.then()
calls.async function getData() { let response = await fetch('https://api.example.com/data'); let data = await response.json(); console.log(data); }
🔍 Why Use Async and Await?
Async/await simplifies how we handle async tasks, making it easier to read and maintain code. It also reduces “callback hell” and makes error handling more straightforward with try...catch
blocks.
🌟 Practical Example with Async and Await
Let’s see how to fetch data using async/await:
async function fetchUser() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/users/1');
const user = await response.json();
console.log('User:', user);
} catch (error) {
console.error("Error fetching data:", error);
}
}
fetchUser();
In this example:
await
pauses execution untilfetch()
completes.If an error occurs, it’s caught in the
catch
block, keeping the code cleaner and easier to debug.
🚀 Wrapping Up
Today, async/await unlocked a new level of readability for our async JavaScript code. Try experimenting with async/await in your projects, and see how it can simplify async programming!
Stay tuned for tomorrow, where we’ll dive into the world of JSON and explore how to handle, parse, and work with data in JavaScript!
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
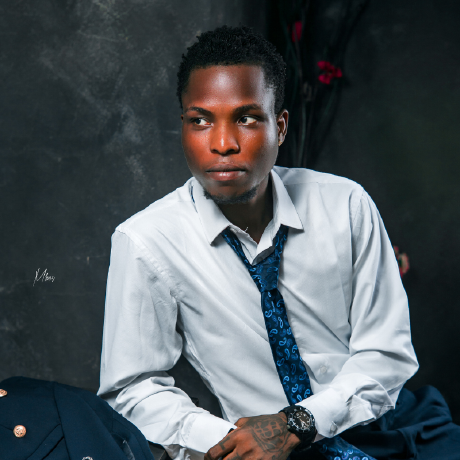
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com