Object-Oriented Programming in PHP: A Beginner's Journey

Table of contents
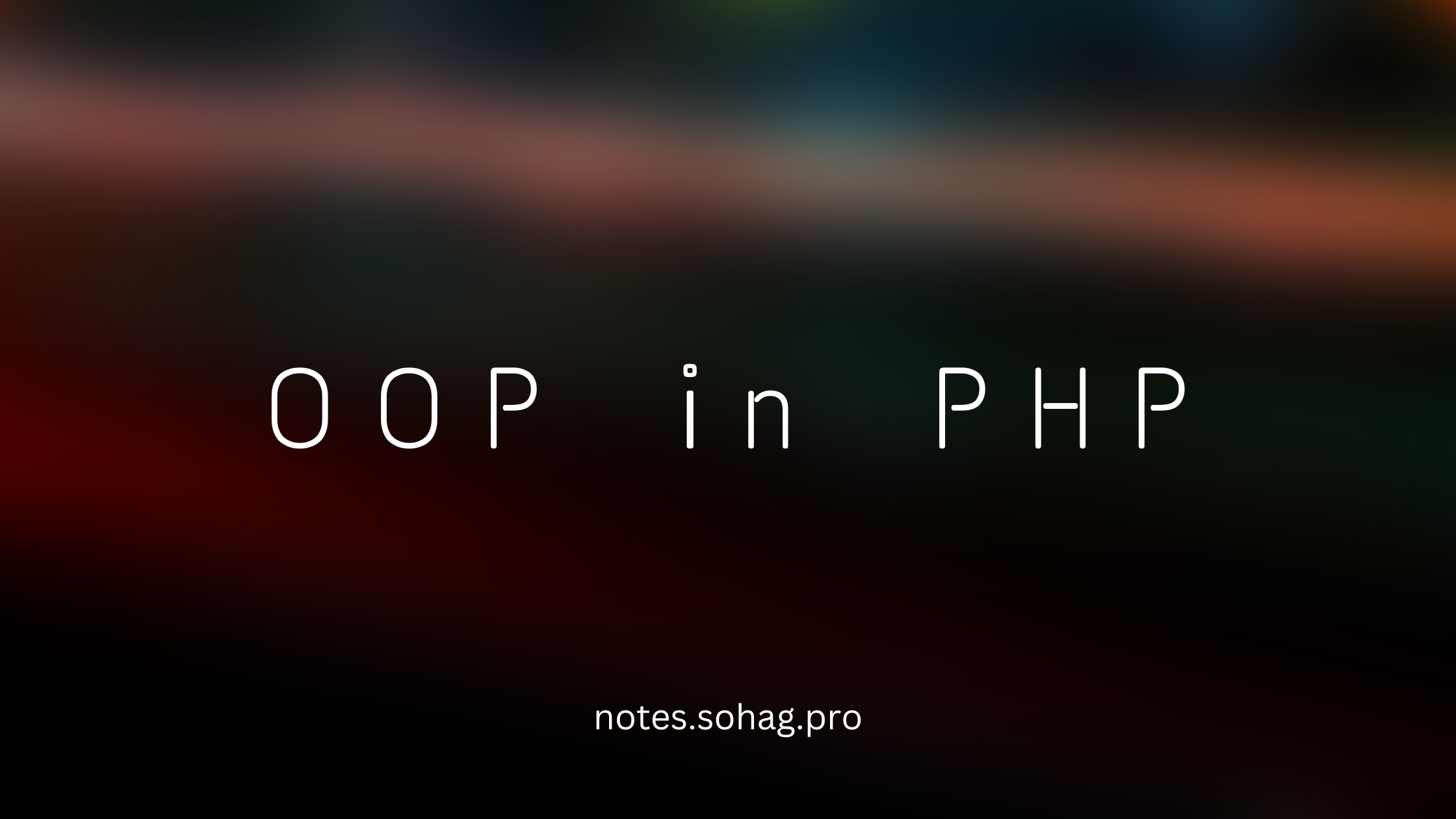
Object-Oriented Programming in PHP
Introduction
Imagine you're organizing a kitchen. Object-Oriented Programming (OOP) is a lot like creating a well-organized cooking space where everything has its place and purpose. In PHP, OOP helps us write cleaner, more organized, and more efficient code by treating our programming elements like real-world objects.
1. Classes and Objects: Your Kitchen Blueprint Analogy
What is a Class?
A class is like a blueprint or a recipe card. It defines the structure, properties, and behaviors that objects will have. Think of it as a detailed plan for creating something.
What is an Object?
An object is a specific instance of a class - it's like an actual kitchen tool created from that blueprint. If a class is a recipe card for a cake, an object is the actual cake you bake.
Let's break this down with a practical example:
// This is a class - a blueprint for creating refrigerators
class Refrigerator {
// Properties (characteristics of the refrigerator)
private $brand;
private $temperature;
private $items = [];
// Constructor - sets up the initial state when creating a new refrigerator
public function __construct($brand) {
$this->brand = $brand;
$this->temperature = 4; // Default temperature in Celsius
}
// Methods (actions a refrigerator can do)
public function addItem($item) {
$this->items[] = $item;
return "Added {$item} to the {$this->brand} refrigerator";
}
public function getItems() {
return $this->items;
}
public function getBrand() {
return $this->brand;
}
}
// Creating objects (actual refrigerators) from the class
$homeRefrigerator = new Refrigerator("Samsung");
$officeRefrigerator = new Refrigerator("LG");
// Using the objects
echo $homeRefrigerator->addItem("Milk");
echo $officeRefrigerator->addItem("Lunch Box");
// Each object is separate and can have different contents
print_r($homeRefrigerator->getItems());
print_r($officeRefrigerator->getItems());
Key Differences Between Class and Object:
Class:
A template or blueprint
Defines properties and methods
Does not consume memory until an object is created
Like an architectural plan for a house
Object:
A specific instance of a class
Has actual values for properties
Consumes memory
Like an actual house built from the architectural plan
Real-Life Analogy
Class = Car Model Design
Defines what a specific car model looks like
Specifies features like number of doors, engine type, etc.
Object = Actual Cars
Specific cars created from that design
Each with its own color, license plate, current mileage
Multiple cars can be created from the same class
Multiple Objects, Same Class
class Car {
private $color;
private $mileage = 0;
public function __construct($color) {
$this->color = $color;
}
public function drive($distance) {
$this->mileage += $distance;
return "Drove {$distance} miles";
}
public function getMileage() {
return $this->mileage;
}
}
// Creating multiple objects from the same class
$redCar = new Car("Red");
$blueCar = new Car("Blue");
$redCar->drive(100);
$blueCar->drive(50);
echo "Red Car Mileage: " . $redCar->getMileage(); // 100
echo "Blue Car Mileage: " . $blueCar->getMileage(); // 50
2. Encapsulation: Protecting Your Kitchen Secrets
Encapsulation is like having a locked pantry. You control who can access and modify your ingredients.
class CoffeeMaker {
// Private property - can't be accessed directly from outside
private $waterLevel;
// Public method to safely interact with private property
public function fillWater($amount) {
if ($amount > 0 && $amount <= 1000) {
$this->waterLevel = $amount;
}
}
public function brew() {
if ($this->waterLevel >= 250) {
return "Coffee is brewing!";
}
return "Not enough water";
}
}
3. Inheritance: The Family Recipe Book
Inheritance is like passing down family recipes. A child class inherits properties and methods from a parent class.
class Vehicle {
protected $wheels;
public function move() {
return "Moving forward";
}
}
class Car extends Vehicle {
public function __construct() {
$this->wheels = 4;
}
public function honk() {
return "Beep beep!";
}
}
$myCar = new Car();
echo $myCar->move(); // Inherited method
echo $myCar->honk(); // Car's own method
4. Polymorphism: The Multi-Talented Kitchen Gadget
Polymorphism is like a Swiss Army knife that can perform different actions based on how you use it.
interface Cookable {
public function prepare();
}
class Pizza implements Cookable {
public function prepare() {
return "Kneading dough, adding toppings";
}
}
class Salad implements Cookable {
public function prepare() {
return "Chopping vegetables, mixing dressing";
}
}
function cookItem(Cookable $item) {
echo $item->prepare();
}
$pizza = new Pizza();
$salad = new Salad();
cookItem($pizza); // Different implementations
cookItem($salad);
5. Abstraction: Simplifying Complex Processes
Abstraction is like using a coffee machine. You don't need to know how it works internally, just how to use it.
abstract class SmartDevice {
abstract public function turnOn();
abstract public function turnOff();
public function restart() {
$this->turnOff();
$this->turnOn();
}
}
class Smartphone extends SmartDevice {
public function turnOn() {
return "Smartphone booting up";
}
public function turnOff() {
return "Smartphone shutting down";
}
}
Additional Pro Tips for OOP in PHP
Understanding Object Relationships
Composition: Objects can contain other objects
Dependency Injection: Pass objects as parameters to create flexible code
class Engine {
public function start() {
return "Engine started";
}
}
class Car {
private $engine;
public function __construct(Engine $engine) {
$this->engine = $engine;
}
public function startCar() {
return $this->engine->start();
}
}
Conclusion
OOP in PHP is like being a master chef in a well-organized kitchen. By understanding these principles, you can write code that's:
Organized
Reusable
Easy to maintain
Scalable
Final Takeaways:
Always think of objects as real-world entities
Keep your classes focused and simple
Use inheritance and polymorphism wisely
Protect your data with encapsulation
Start small and gradually build complexity
Learning Path:
Understand basic class and object concepts
Practice creating simple classes
Explore each OOP principle
Build small projects to reinforce learning
Happy Coding! ๐๐ฉโ๐ป๐จโ๐ป
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author