The DRY Principle in PHP: Writing Cleaner, More Efficient Code

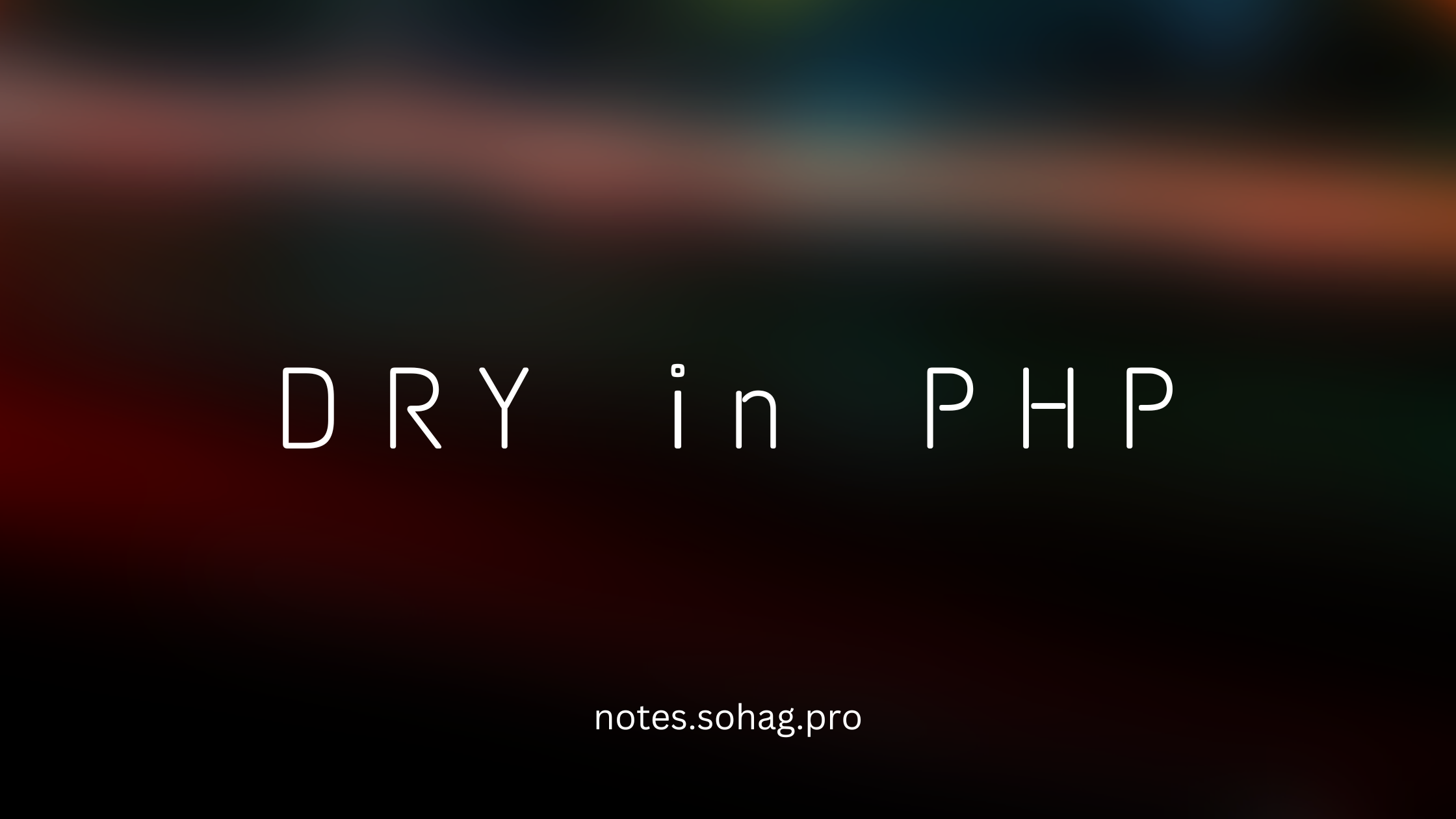
[“DRY” => “Don't Repeat Yourself”]
What is the DRY Principle?
DRY stands for "Don't Repeat Yourself" - a fundamental principle of software development that aims to reduce repetition in code. Think of it like a cooking recipe: if you find yourself doing the same steps over and over, it's time to create a reusable method that does the work for you.
Real-Life Analogy: The Coffee Shop Workflow
Imagine you're managing a coffee shop. Every time a customer orders a drink, you:
Greet the customer
Take their order
Process the payment
Prepare the drink
Serve the drink
Instead of manually going through these steps each time, you'd create a standard process (or in programming terms, a method) that handles these repeated tasks efficiently.
Why DRY Matters in PHP
Before DRY: Repetitive Code
<?php
// Calculating total price for different order types
function calculatePizzaTotal($pizzaPrice, $quantity) {
$tax = 0.1;
$total = $pizzaPrice * $quantity;
$totalWithTax = $total * (1 + $tax);
return $totalWithTax;
}
function calculateBurgerTotal($burgerPrice, $quantity) {
$tax = 0.1;
$total = $burgerPrice * $quantity;
$totalWithTax = $total * (1 + $tax);
return $totalWithTax;
}
function calculateSaladTotal($saladPrice, $quantity) {
$tax = 0.1;
$total = $saladPrice * $quantity;
$totalWithTax = $total * (1 + $tax);
return $totalWithTax;
}
After DRY: Efficient and Reusable Code
<?php
class PriceCalculator {
private const TAX_RATE = 0.1;
public function calculateTotal($itemPrice, $quantity) {
$total = $itemPrice * $quantity;
return $total * (1 + self::TAX_RATE);
}
}
// Usage
$calculator = new PriceCalculator();
$pizzaTotal = $calculator->calculateTotal(10, 2);
$burgerTotal = $calculator->calculateTotal(8, 3);
Key DRY Principles in PHP
1. Create Reusable Functions
<?php
// Before DRY
function sendEmailToAdmin($message) {
$to = 'admin@example.com';
mail($to, 'System Notification', $message);
}
function sendEmailToSupport($message) {
$to = 'support@example.com';
mail($to, 'System Notification', $message);
}
// After DRY
function sendEmail($to, $message) {
mail($to, 'System Notification', $message);
}
sendEmail('admin@example.com', 'Admin message');
sendEmail('support@example.com', 'Support message');
2. Use Inheritance and Traits
<?php
trait Loggable {
public function log($message) {
file_put_contents('app.log', $message . PHP_EOL, FILE_APPEND);
}
}
class UserService {
use Loggable;
public function createUser($userData) {
// User creation logic
$this->log('User created: ' . $userData['username']);
}
}
class ProductService {
use Loggable;
public function addProduct($productData) {
// Product addition logic
$this->log('Product added: ' . $productData['name']);
}
}
3. Configuration and Constants
<?php
class AppConfig {
public const DATABASE_HOST = 'localhost';
public const DATABASE_USER = 'root';
public const DATABASE_PASS = 'password';
public static function getDatabaseConnection() {
return new PDO(
'mysql:host=' . self::DATABASE_HOST,
self::DATABASE_USER,
self::DATABASE_PASS
);
}
}
Common DRY Pitfalls to Avoid
Over-Abstraction: Don't create complex methods for simple tasks
Premature Optimization: Write readable code first, then optimize
Copy-Paste Coding: Always refactor repeated code
Benefits of DRY Principle
Reduced Maintenance: Less code means fewer places to fix bugs
Improved Readability: Clean, concise code is easier to understand
Faster Development: Reusable components speed up coding
Lower Cognitive Load: Less mental effort to manage code
Conclusion
The DRY principle is like a secret weapon in a programmer's toolkit. It's not about being lazy, but about being smart. By reducing repetition, you create more maintainable, efficient, and elegant code.
Quick Tips for PHP Developers
Always look for patterns in your code
Refactor regularly
Use PHP's object-oriented features
Embrace code reusability
Happy coding! 🚀👨💻
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author