Demystifying PHP Traits: Your Comprehensive Guide to Code Reusability

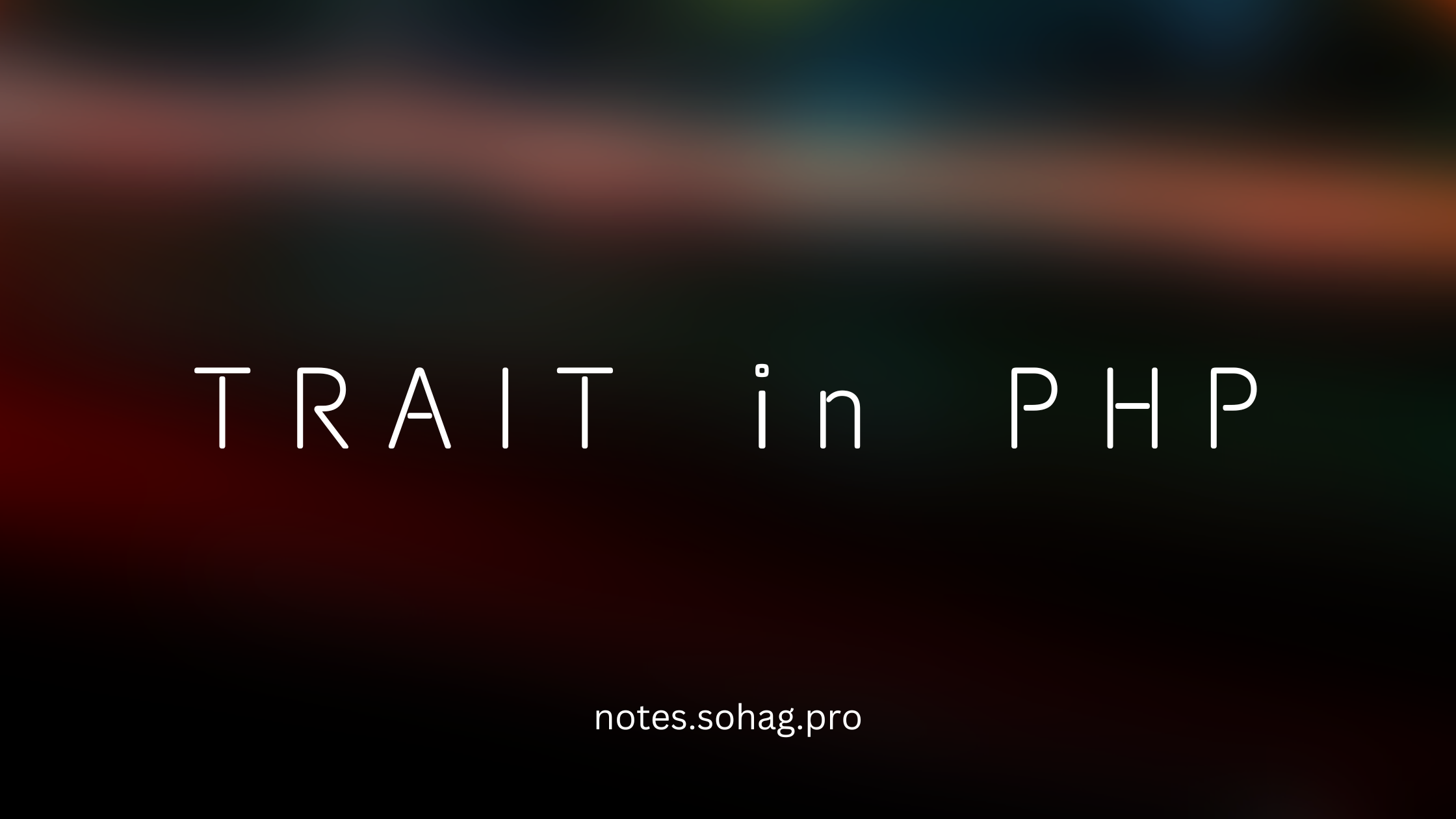
PHP Traits
What are Traits in PHP?
Imagine you're building a house, and instead of constructing everything from scratch, you could simply pick up pre-made modules and attach them wherever you need. In PHP, Traits are exactly like these modular building blocks for your code!
A trait is a mechanism for code reuse in single inheritance languages like PHP. It allows you to share methods across different classes without using traditional inheritance. Think of traits as a way to "copy-paste" functionality into your classes, but with much more elegance and control.
Why Do We Need Traits?
PHP follows single inheritance, meaning a class can only extend one parent class. But what if you want to share similar methods across multiple, unrelated classes? This is where traits come to the rescue!
Real-World Analogy: Swiss Army Knife of Coding
Consider a Swiss Army knife. It has multiple tools (like a blade, scissors, screwdriver) that can be used in different contexts. Traits work similarly – they're versatile code components you can "attach" to various classes.
Basic Trait Syntax
trait LoggerTrait {
public function log($message) {
echo date('Y-m-d H:i:s') . ": $message\n";
}
}
class User {
use LoggerTrait; // Importing the trait
public function register() {
$this->log("New user registered"); // Using trait method
}
}
Advanced Trait Features
1. Multiple Trait Usage
trait DatabaseTrait {
public function save() {
// Database save logic
}
}
trait ValidationTrait {
public function validate() {
// Validation logic
}
}
class Product {
use DatabaseTrait, ValidationTrait;
public function create() {
$this->validate();
$this->save();
}
}
2. Conflict Resolution
When traits have methods with the same name, PHP provides mechanisms to resolve conflicts:
trait FirstTrait {
public function sayHello() {
echo "Hello from First Trait";
}
}
trait SecondTrait {
public function sayHello() {
echo "Hello from Second Trait";
}
}
class Greeter {
use FirstTrait, SecondTrait {
FirstTrait::sayHello insteadof SecondTrait; // Use FirstTrait's method
SecondTrait::sayHello as greet; // Alias the conflicting method
}
}
Practical Real-Life Examples
1. E-commerce Logging Trait
trait OrderLoggingTrait {
public function logOrderCreation($orderId) {
// Log order details to file/database
file_put_contents('order_log.txt',
"Order $orderId created at " . date('Y-m-d H:i:s') . "\n",
FILE_APPEND
);
}
}
class Order {
use OrderLoggingTrait;
public function create() {
// Order creation logic
$orderId = uniqid();
$this->logOrderCreation($orderId);
}
}
2. User Permission Trait
trait PermissionTrait {
protected $userRoles = ['admin', 'editor', 'viewer'];
public function hasPermission($requiredRole) {
return in_array($this->role, $this->userRoles);
}
}
class UserAccount {
use PermissionTrait;
protected $role;
public function __construct($role) {
$this->role = $role;
}
}
When to Use Traits
✅ Sharing common methods across unrelated classes ✅ Avoiding deep inheritance hierarchies ✅ Adding utility functions to multiple classes ❌ Not a replacement for proper object-oriented design
Performance Considerations
Traits are resolved at compile-time, so they have minimal runtime performance overhead. They're a clean way to share code without the complexity of multiple inheritance.
Best Practices
Keep traits focused and with a single responsibility
Avoid creating massive traits with numerous unrelated methods
Use type hinting and proper method visibility
Consider composition over trait usage for complex scenarios
Common Pitfalls to Avoid
Don't overuse traits
Maintain clear, readable code
Be mindful of method name conflicts
Remember that traits can't be instantiated directly
Conclusion
Traits in PHP are powerful tools for code reuse, offering flexibility beyond traditional inheritance. They allow you to write more modular, maintainable code by letting you share methods across different classes effortlessly.
Start small, experiment, and you'll soon see how traits can simplify your PHP development process!
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author