The KISS Principle in PHP: Simplicity is Your Superpower

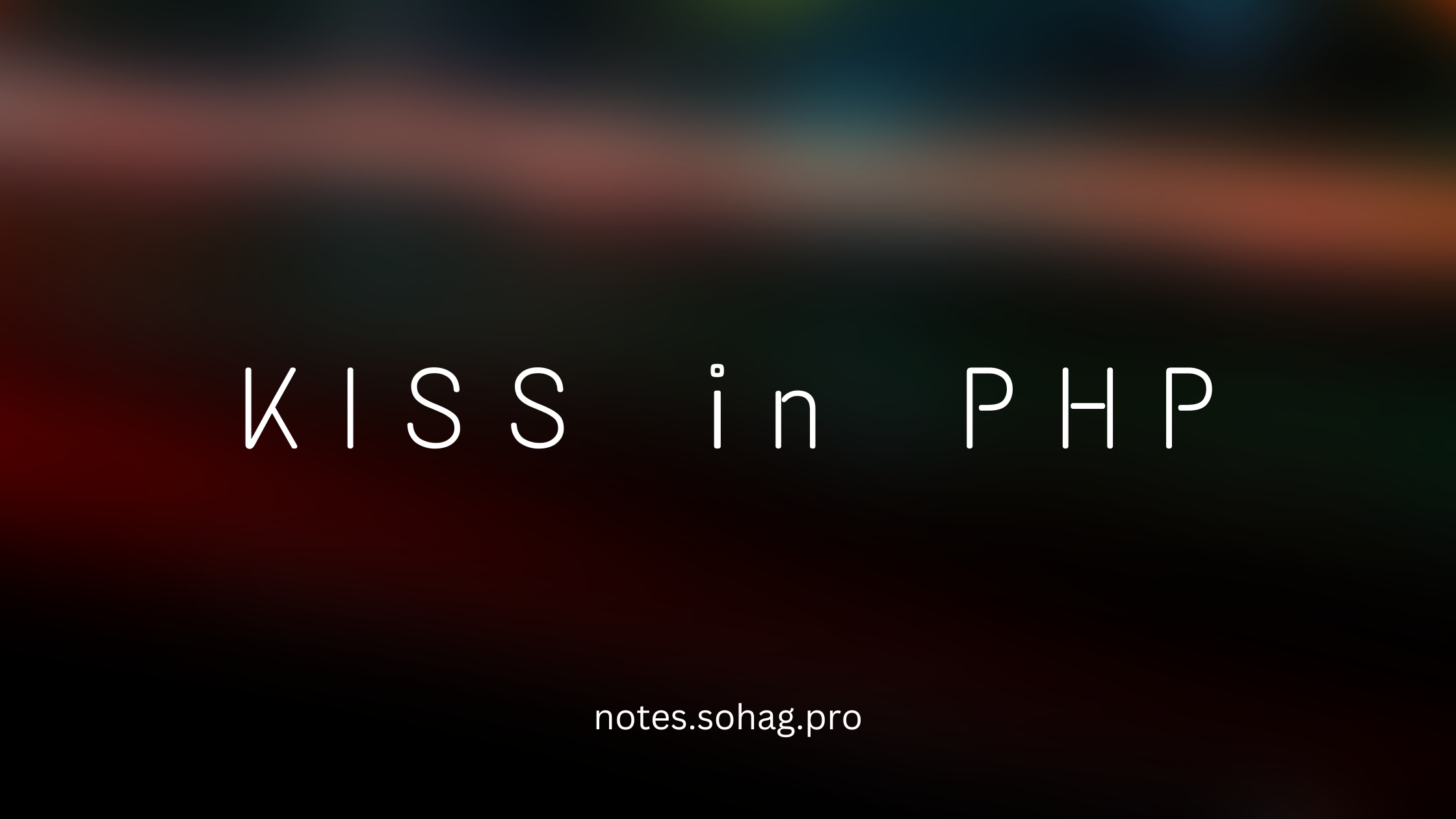
[“KISS” => “Keep It Simple, Stupid”]
Introduction: What is the KISS Principle?
KISS stands for "Keep It Simple, Stupid" - a principle that is as powerful as it is straightforward. In the world of programming, and especially in PHP, complexity is often the enemy of good code. The KISS principle advocates for creating code that is easy to read, understand, and maintain.
Imagine you're building a house. Would you prefer a complex architectural marvel with hidden passages and intricate mechanisms, or a well-designed, functional home that meets all your needs? Programming is no different. Simple code is like a well-planned house - it's efficient, reliable, and easy to live in.
Why Simplicity Matters in PHP
In PHP development, simplicity isn't just a preference - it's a necessity. Complex code leads to:
Increased debugging time
Higher probability of bugs
Difficulty in collaboration
Reduced code maintainability
Real-Life Examples of KISS in Action
Example 1: User Authentication
❌ Complex Approach:
function authenticateUser($credentials) {
$complexPasswordValidation = function($password) {
$specialCharRegex = '/^(?=.*[!@#$%^&*(),.?":{}|<>])(?=.*[A-Z])(?=.*[a-z])(?=.*[0-9]).{12,}$/';
$databaseBlacklistedPasswords = ['password123', 'admin1234'];
$complexHistoricalCheck = function($password) {
// Extremely complex password history check
return false;
};
return preg_match($specialCharRegex, $password) &&
!in_array($password, $databaseBlacklistedPasswords) &&
!$complexHistoricalCheck($password);
};
// Overly complicated authentication logic
return $complexPasswordValidation($credentials['password']);
}
✅ KISS Approach:
function authenticateUser($credentials) {
// Simple, clear password validation
return strlen($credentials['password']) >= 8 &&
!empty($credentials['username']);
}
Example 2: Calculating Discount
Consider an e-commerce scenario where you want to apply discounts:
❌ Overly Complex Discount Calculation:
function calculateDiscount($price, $customerType, $season, $loyaltyPoints, $specialPromoCode) {
$discountMatrix = [
'vip' => [
'summer' => function($price, $loyaltyPoints, $promoCode) {
// Extremely complex discount calculation
return $price * 0.5;
},
'winter' => function($price, $loyaltyPoints, $promoCode) {
// Another complex calculation
return $price * 0.4;
}
],
// More complex nested conditions...
];
// Convoluted logic to determine final discount
return $discountMatrix[$customerType][$season]($price, $loyaltyPoints, $specialPromoCode);
}
✅ KISS Approach:
function calculateDiscount($price, $discountPercentage) {
return $price * (1 - $discountPercentage);
}
// Simple usage
$finalPrice = calculateDiscount(100, 0.2); // 20% discount
Practical Tips for Embracing KISS in PHP
Write Clear, Readable Code
Use descriptive variable names
Keep functions small and focused
Avoid nested conditionals
Simplify Logic
Break complex problems into smaller, manageable parts
Use built-in PHP functions instead of reinventing the wheel
Refactor Regularly
Continuously review and simplify your code
Remove unnecessary complexity
Common KISS Antipatterns to Avoid
Overengineering solutions
Premature optimization
Creating unnecessarily abstract code
Writing clever code instead of clear code
The Zen of Simplicity
Remember, great code is not about being the most clever or complex. It's about being clear, maintainable, and solving the problem at hand efficiently.
"Simplicity is the ultimate sophistication." - Leonardo da Vinci
Conclusion
The KISS principle in PHP is about writing code that speaks for itself. It's about creating solutions that are immediately understandable, easy to maintain, and a joy to work with.
Next time you're coding, ask yourself: "Can I make this simpler?"
Happy coding! 🚀👨💻
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author