YAGNI Principle in PHP: Keeping Your Code Lean and Mean

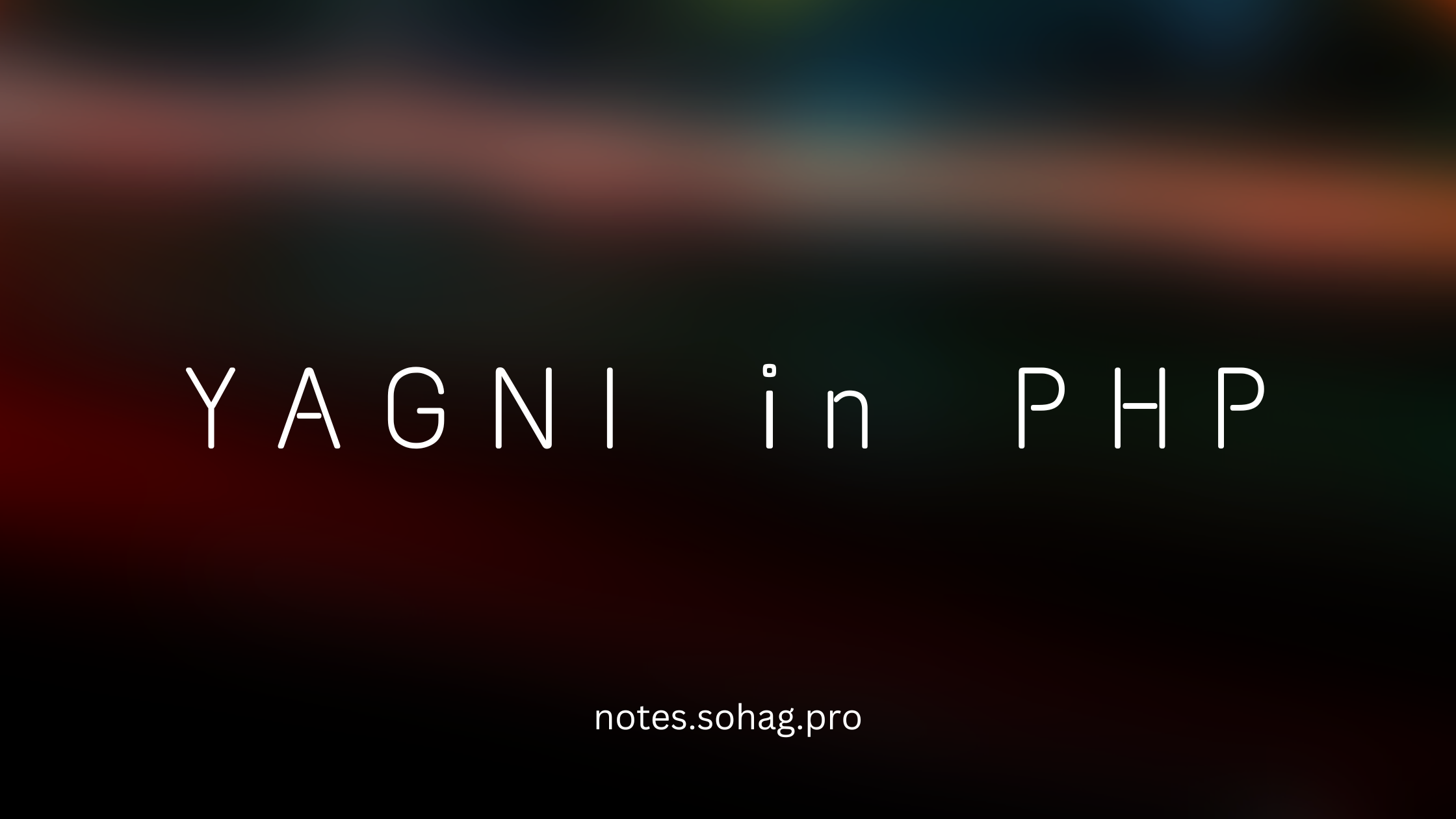
[“YAGNI” => “You Aren't Gonna Need It”]
What is YAGNI?
YAGNI stands for "You Aren't Gonna Need It" - a principle that sounds simple but can revolutionize the way you write code. Coined by extreme programming guru Ron Jeffries, YAGNI is all about avoiding unnecessary complexity by not adding functionality until it's absolutely necessary.
The Core Philosophy
Imagine you're packing for a weekend trip. Would you pack a winter coat, ski gear, and snow boots if you're going to a tropical beach? Of course not! Similarly, in programming, YAGNI advises against adding features or complexity that you might need in some hypothetical future scenario.
Why YAGNI Matters in PHP Development
Let's break down why this principle is crucial:
Reduces Unnecessary Complexity
Saves Development Time
Keeps Code Maintainable
Improves Code Readability
Real-World Examples
Bad Example: Over-Engineering a User Registration
class UserRegistration {
private $user;
private $emailValidator;
private $passwordStrengthChecker;
private $socialMediaIntegration;
private $advancedLoggingSystem;
private $futureFeatureFlags;
private $internationalizationSupport;
public function register(array $userData) {
// A method with dozens of potential future features
// Most of which aren't needed right now
}
}
Good Example: Focused, Minimal Implementation
class UserRegistration {
public function register(string $email, string $password) {
// Only what's immediately necessary
$this->validateEmail($email);
$this->hashPassword($password);
$this->saveUser($email, $password);
}
private function validateEmail(string $email) {
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
throw new InvalidArgumentException("Invalid email format");
}
}
private function hashPassword(string $password) {
return password_hash($password, PASSWORD_DEFAULT);
}
private function saveUser(string $email, string $password) {
// Basic user save logic
}
}
Relatable Analogies
The Restaurant Kitchen Analogy
Think of your code like a restaurant kitchen:
Bad Approach (Non-YAGNI): Buying every possible kitchen gadget before opening
Good Approach (YAGNI): Start with essential tools, add specialized equipment only when a specific need arises
The Backpack Packing Scenario
Non-YAGNI Coding:
Pack everything you might need
Carry a heavy, complex backpack
Waste energy managing unnecessary items
YAGNI Coding:
Pack only essential items
Keep your backpack light and manageable
Easily adapt as needs change
When to Apply YAGNI
During Initial Development
When Adding New Features
During Code Refactoring
Red Flags to Watch For
Excessive abstract classes
Complex inheritance hierarchies
Premature optimization
Anticipating hypothetical future requirements
Practical PHP Example
// Non-YAGNI Approach
class ReportGenerator {
public function generatePDFReport() { /* Complex PDF logic */ }
public function generateExcelReport() { /* Complex Excel logic */ }
public function generateWordReport() { /* Complex Word logic */ }
public function generateCSVReport() { /* Complex CSV logic */ }
}
// YAGNI Approach
class ReportGenerator {
public function generateReport(string $type, array $data) {
switch ($type) {
case 'pdf':
return $this->createPdfReport($data);
case 'csv':
return $this->createCsvReport($data);
default:
throw new InvalidArgumentException("Unsupported report type");
}
}
}
Common Misconceptions
YAGNI ≠ Never Plan Ahead
- It means don't implement until necessary
YAGNI ≠ Poor Architecture
- Maintain clean, flexible design
YAGNI ≠ Avoiding Good Practices
- Still follow solid software design principles
Conclusion
YAGNI is about writing intentional, focused code. It's not about being lazy, but about being smart. Add complexity only when it provides demonstrable value.
Pro Tips
Always ask: "Do I really need this right now?"
Embrace simplicity
Refactor when requirements change
Keep your code as lean as possible
Remember: Good code is not about how much you can add, but how little you need to solve the problem effectively.
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author