Programming to Interface, Not to Implementation: A PHP Developer's Journey

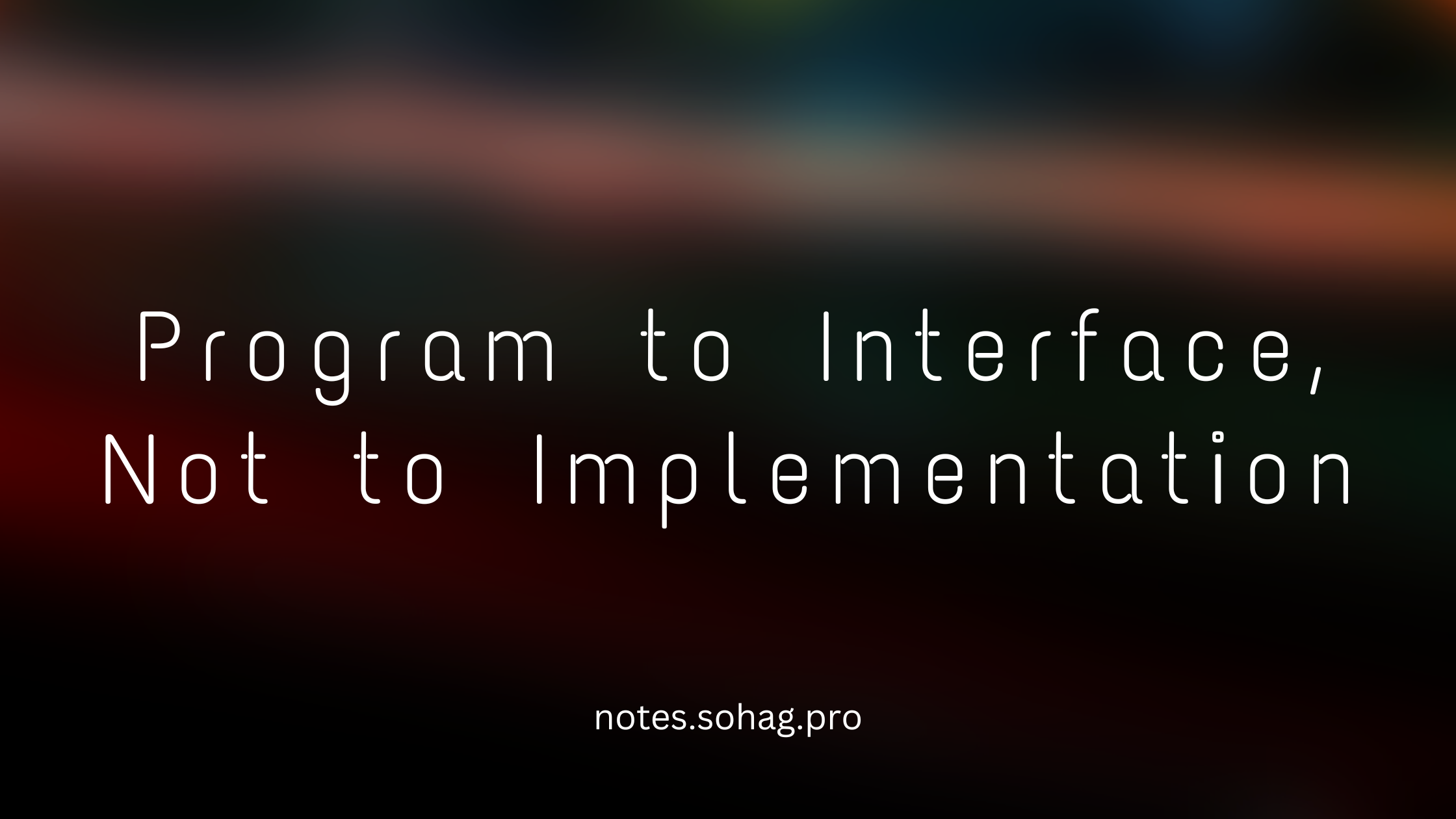
Program to Interface, Not to Implementation
Introduction
Imagine you're ordering food at a restaurant. You don't need to know how the chef prepares your meal, you just want a delicious dish served hot and on time. In the world of software development, the "Program to Interface, Not to Implementation" principle works exactly the same way!
What is the Interface Principle?
At its core, this principle suggests that you should depend on abstractions (interfaces) rather than concrete implementations. It's about creating flexible, maintainable code that can easily adapt to changes.
Breaking It Down Simply
Think of an interface like a contract. It promises certain behaviors without revealing exactly how those behaviors are achieved. Just like a restaurant menu promises a dish without detailing the exact cooking process.
Why Should You Care?
Benefits of Programming to Interface
Flexibility: Easily swap out implementations without breaking your code
Decoupling: Reduce dependencies between different parts of your application
Testability: Simplify unit testing by mocking interfaces
Scalability: Make your code more modular and extensible
Real-World Analogy: Transportation ๐
Let's use a transportation system as an example:
// Interface defines the contract for transportation
interface TransportInterface {
public function move(): string;
}
// Different implementations
class Car implements TransportInterface {
public function move(): string {
return "Driving on roads";
}
}
class Bicycle implements TransportInterface {
public function move(): string {
return "Pedaling through bike lanes";
}
}
class Train implements TransportInterface {
public function move(): string {
return "Traveling on railway tracks";
}
}
// Transport Manager depends on the interface, not specific implementations
class TransportManager {
private TransportInterface $vehicle;
public function __construct(TransportInterface $vehicle) {
$this->vehicle = $vehicle;
}
public function travel(): string {
return $this->vehicle->move();
}
}
// Usage
$carTrip = new TransportManager(new Car());
echo $carTrip->travel(); // Outputs: Driving on roads
$bikeTrip = new TransportManager(new Bicycle());
echo $bikeTrip->travel(); // Outputs: Pedaling through bike lanes
Another Practical Example: Notification Systems ๐ฑ
// Notification interface
interface NotificationInterface {
public function send(string $message): bool;
}
// Email implementation
class EmailNotification implements NotificationInterface {
public function send(string $message): bool {
// Email sending logic
return true;
}
}
// SMS implementation
class SMSNotification implements NotificationInterface {
public function send(string $message): bool {
// SMS sending logic
return true;
}
}
// Notification Service
class NotificationService {
private NotificationInterface $notifier;
public function __construct(NotificationInterface $notifier) {
$this->notifier = $notifier;
}
public function sendAlert(string $message): bool {
return $this->notifier->send($message);
}
}
// Easy to switch notification methods
$emailService = new NotificationService(new EmailNotification());
$emailService->sendAlert("System maintenance");
$smsService = new NotificationService(new SMSNotification());
$smsService->sendAlert("Urgent update");
Common Mistakes to Avoid
Tight Coupling: Don't create classes that are too dependent on specific implementations
Ignoring Interfaces: Always define clear contracts for your classes
Overcomplicating: Use interfaces where they add real value, not everywhere
When to Use Interfaces
When you expect multiple implementations
For strategies that might change
When you want to define a contract for behavior
To support dependency injection
Pro Tips ๐ก
Keep interfaces small and focused
Name interfaces clearly (e.g.,
NotificationInterface
, not justInterface
)Use type-hinting to enforce interface usage
Conclusion
Programming to interface is like being a flexible traveler. You're not tied to one specific mode of transportation but can adapt based on the situation. In coding, this means creating more robust, maintainable, and scalable applications.
Quick Recap
Depend on abstractions
Create clear contracts
Allow easy implementation swapping
Improve code flexibility
Happy coding, PHP developers! ๐๐จโ๐ป๐ฉโ๐ป
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author