Understanding closure in JavaScript.
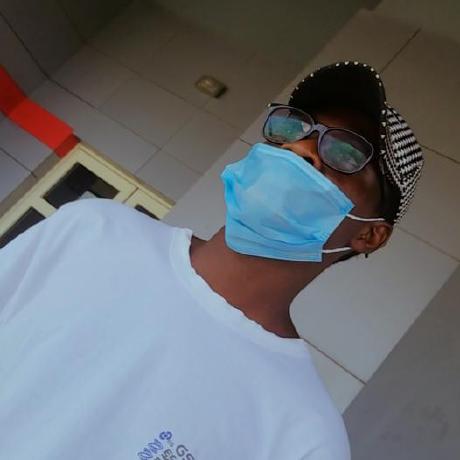
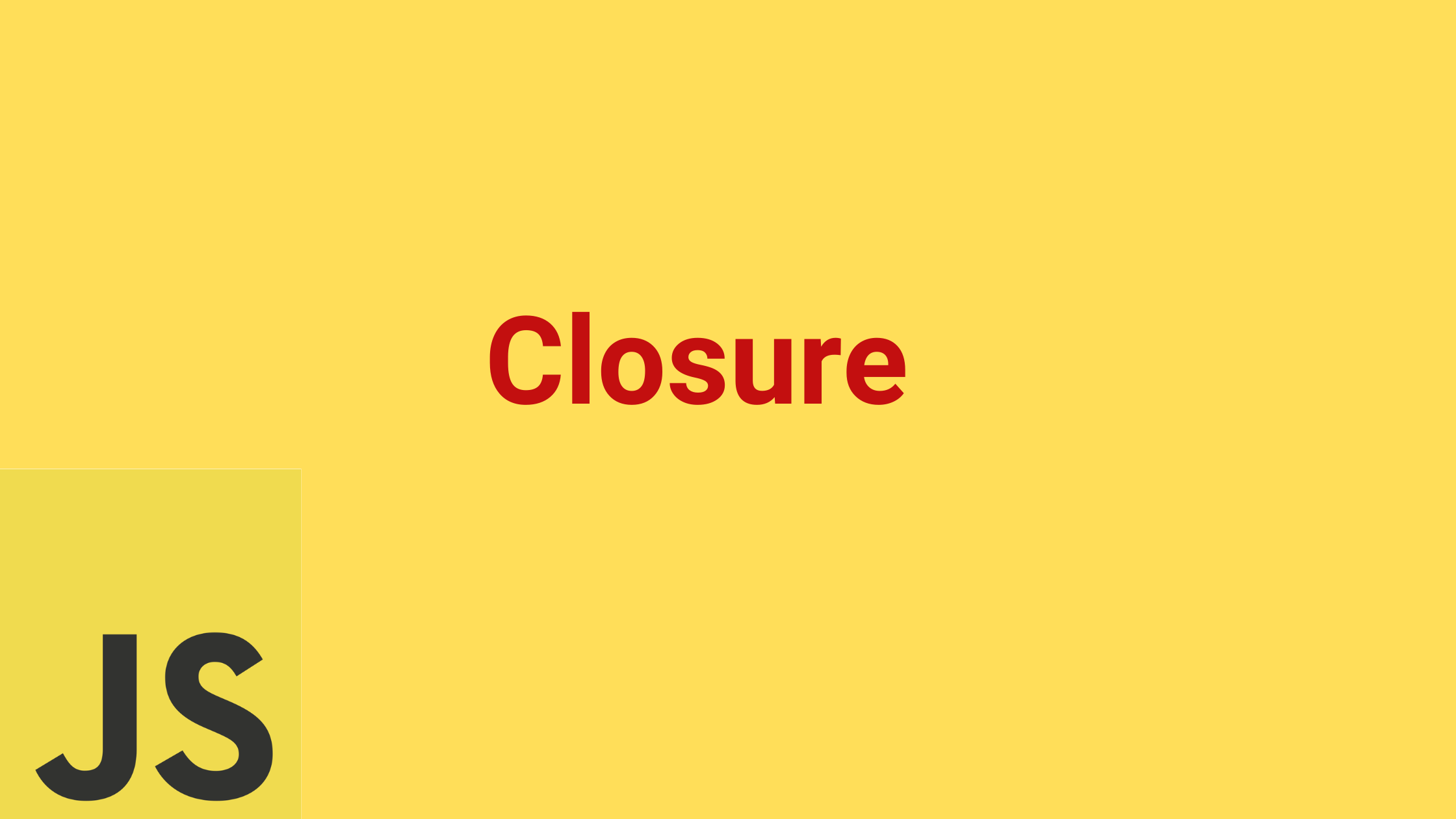
If you’ve spent any time in JavaScript, you’ve probably run into the word closure and thought, “What is this magic?” Closures can feel like an advanced topic, but once you get them, they make so much sense and open up a lot of possibilities in your code. Let’s break closures down, so they become a tool you can reach for with confidence.
What’s the Deal with Closures?
In a nutshell, a closure is created when a function returns another function, and that inner function has access to variables in the outer function even after the outer function has finished running. It’s like the inner function is carrying a little “backpack” of variables with it.
Example of closure.
To get a feel for closures, let’s build a counter function. Here’s the plan: we want a function that keeps track of a count
variable and lets us increment it each time we call it. Here’s how we’d set that up in code:
function createCounter() {
let count = 0; // This variable lives inside createCounter
function incrementCounter() { // This function can access 'count'
count++;
return count;
}
return incrementCounter;
}
const increment = createCounter();
// Calls createCounter, but returns incrementCounter
console.log(increment()); // Output: 1
console.log(increment()); // Output: 2
console.log(increment()); // Output: 3
So, what’s happening here? Each time we call increment()
, it goes back to that count
variable, even though createCounter
finished running a while ago. Thanks to closures, increment
has “closed over” the count
variable and keeps it alive.
The explaniation below,
Calling
createCounter()
: When we callcreateCounter()
, JavaScript sets up a new Execution context (a space in memory) and a variable environment for it. Thecount
variable is created in this environment with an initial value of 0.incrementCounter
is defined withincreateCounter
, so it can accesscount
.Returning
incrementCounter
: OncecreateCounter
finishes, instead of throwing away its environment, JavaScript holds onto it becauseincrementCounter
—the function we returned—still needs it. So,incrementCounter
has a “backpack” containingcount
.Using
increment()
: Now, each time we callincrement()
, JavaScript looks inside that “backpack” to get the currentcount
value. Every time we callincrement()
, it increments the savedcount
value by 1, even thoughcreateCounter
finished executing a while back.
Imagine that createCounter
is like a hiker packing a “backpack” before a long trip. Once the hiker (the outer function) finishes their setup, they can leave, but their backpack (the closure) goes along with the items they packed. Whenever the hiker (in this case, the incrementCounter
function) needs something from their backpack, it’s still there, ready to use.
Why Closures Are So Useful
Closures are JavaScript’s way of allowing functions to keep track of variables without having to use global variables. They’re the secret sauce behind a lot of popular JavaScript patterns, like creating private variables or functions that can “remember” information.
Summary: Why Closures Make JavaScript Click
Closures make JavaScript’s function scope powerful. They allow:
Functions to remember variables even after their parent functions finish running.
Private data management, lets us track information without cluttering up global variables.
So next time you’re writing a function inside a function, remember closures they’re just a way for inner functions to keep a “backpack” of variables around for later use. Give closures a try, and watch them make your code more powerful and flexible!
I hope you find this helpful and cheers to my first post🎉, I would love your feedback so I can and grow along the way. Thank you for reading.
Subscribe to my newsletter
Read articles from Oyeyemi Wole Toheeb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
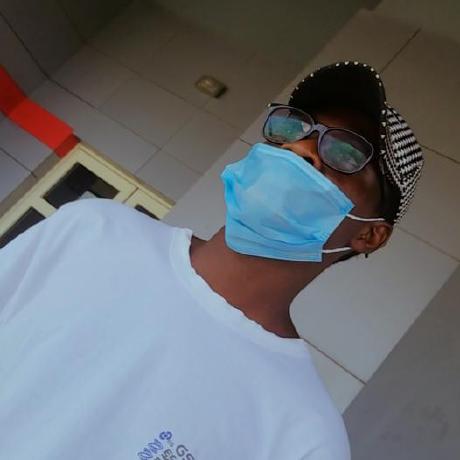