Loop the Loop: Iterating Through Data!
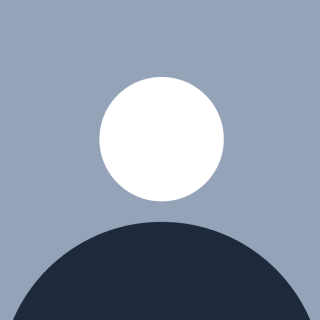
Table of contents
- The Basics of Loops
- For Loops — Your First Spin Around
- Visualizing a For Loop
- While Loops — Spin Until You Say Stop
- While Loop on the Ferris Wheel
- Do-While Loops — Always Spin At Least Once
- Break and Continue: Exiting and Skipping Loops
- Riding the Loops in JavaScript
- Advanced Spins: Introducing For-In and For-Of Loops
- The For-In Loop — Inspecting Your Environment
- The For-Of Loop — Riding the Data Ferris Wheel
- Break and Continue in For-Of and For-In
- Looping with Arrays and Objects — The Magic of Iteration
- Challenge Time — Create a Countdown Timer
- Mastering Looping in JavaScript
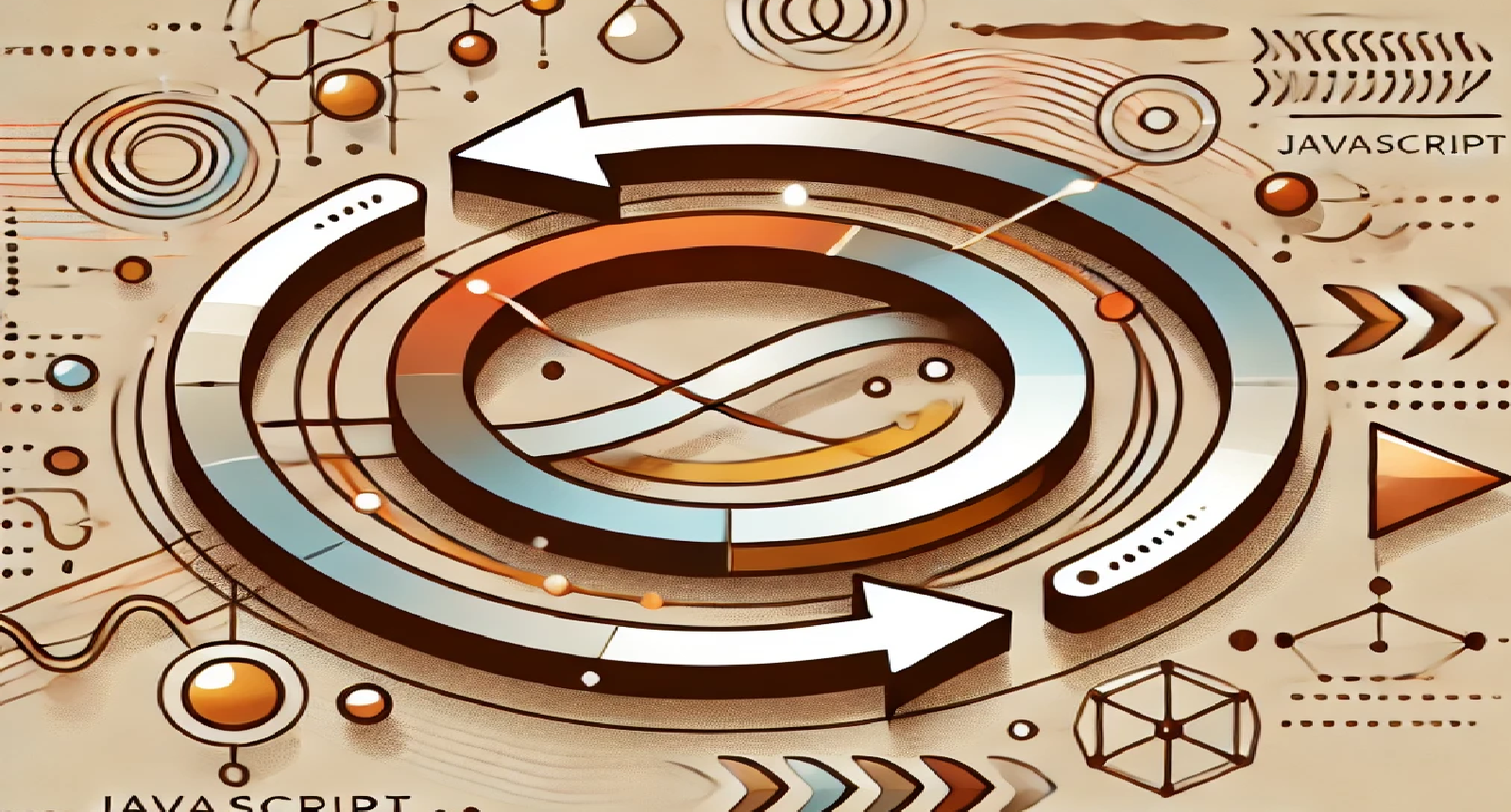
Imagine you’re on a Ferris wheel. The ride goes around, and each time you reach the top, you start the loop over again. In programming, loops are like this Ferris wheel: they allow you to repeat a set of actions until you decide to stop. Whether you’re iterating through a list of tasks, cycling through an array of data, or repeating a sequence of operations, loops are essential tools in JavaScript that keep your code moving.
In this article, we’ll explore the magic of loops—from basic structures to advanced techniques. By the time we’re done, you’ll know how to make your code loop like a well-oiled Ferris wheel.
The Basics of Loops
Loops are used to repeatedly execute a block of code as long as a specific condition is met. JavaScript offers several loop structures, each suited to different scenarios. The most common types of loops include:
For Loop
While Loop
Do-While Loop
But that’s just the start. Along with these, you’ll learn about other loop constructs such as for-in, for-of, and how to control your loops with break and continue statements.
Let’s start by taking our first ride on the Ferris wheel: the for loop.
For Loops — Your First Spin Around
A for loop is like the ticket to your Ferris wheel ride: you know how many times you’ll go around before you get off. For loops are great when you know the number of iterations in advance, such as when looping through an array of known size.
Basic Syntax:
for (initialization; condition; increment) {
// Code to execute each time the loop runs
}
Initialization: This is where you set your starting point (e.g.,
let i = 0
).Condition: As long as this condition is true, the loop keeps running (e.g.,
i < 10
).Increment: After each loop cycle, the value of
i
is updated (e.g.,i++
).
Simple For Loop
Let’s say you’re riding the Ferris wheel and you want to print out each ride number as you loop around:
javascriptCopy codefor (let ride = 1; ride <= 5; ride++) {
console.log("You’re on ride number " + ride);
}
Explanation:
The loop starts at
ride = 1
.The condition checks whether
ride <= 5
. As long as this is true, the loop continues.After each iteration, the value of
ride
increases by 1 (ride++
).
Output:
You’re on ride number 1
You’re on ride number 2
You’re on ride number 3
You’re on ride number 4
You’re on ride number 5
The loop terminates once ride
exceeds 5. You’ve completed your 5 laps on the Ferris wheel!
Visualizing a For Loop
Here’s how a for loop works in a visual format, just like tracking your position on the Ferris wheel:
[ Start Loop ]
|
[ Initialize (ride = 1) ]
|
[ Check Condition (ride <= 5) ]
| \
Yes No
| \
[ Run Code ] [ End Loop ]
|
[ Increment ride (ride++) ]
|
[ Repeat ]
This loop continues until the condition ride <= 5
becomes false, and then the loop stops.
While Loops — Spin Until You Say Stop
While loops are a bit different from for loops. You don’t always know how many times you’ll spin on this Ferris wheel; you just keep going as long as a condition remains true.
Basic Syntax:
while (condition) {
// Code to execute while the condition is true
}
While loops are perfect when you don’t know how many iterations are needed in advance. For example, maybe you’ll keep riding the Ferris wheel until you’re ready to get off.
While Loop Example
Let’s simulate a Ferris wheel ride where you keep going until you feel dizzy:
let dizzy = false;
let ride = 0;
while (!dizzy) {
ride++;
console.log("You’re on ride number " + ride);
if (ride === 3) {
dizzy = true; // You get dizzy after 3 rides
}
}
Explanation:
The loop continues until
dizzy
becomestrue
.After 3 rides, the player gets dizzy and exits the loop.
Output:
You’re on ride number 1
You’re on ride number 2
You’re on ride number 3
While Loop on the Ferris Wheel
Here’s how a while loop works, like continuously riding the Ferris wheel until you signal to stop:
[ Start Loop ]
|
[ Check Condition (!dizzy) ]
| \
Yes No
| |
[ Run Code ] [ End Loop ]
|
[ Repeat ]
In this scenario, the loop keeps going until the condition !dizzy
becomes false, allowing the loop to break.
Do-While Loops — Always Spin At Least Once
Sometimes, you have no choice but to take one spin on the Ferris wheel, whether you like it or not. That’s where the do-while loop comes in: it guarantees the loop will run at least once, even if the condition is false.
Basic Syntax:
do {
// Code to execute
} while (condition);
The do-while loop checks the condition after running the code block, ensuring at least one iteration.
Do-While Loop Example
Let’s say you’re forced to take at least one ride on the Ferris wheel, even if you don’t want to:
let forcedToRide = false;
let ride = 0;
do {
ride++;
console.log("You’re on ride number " + ride);
} while (forcedToRide);
Explanation:
Even though
forcedToRide
is false, the loop still executes once because the condition is checked after the code runs.After the first ride, the loop terminates because the condition is false.
Output:
You’re on ride number 1
Break and Continue: Exiting and Skipping Loops
Loops are powerful, but sometimes you need more control. Maybe you want to exit the Ferris wheel early (using break
), or maybe you want to skip a ride (using continue
).
Break Statement: Exiting the Loop
If you decide you’ve had enough fun, you can exit the loop entirely with the break
statement.
for (let ride = 1; ride <= 5; ride++) {
if (ride === 3) {
console.log("You’ve had enough fun, exiting the ride.");
break; // Exit the loop
}
console.log("You’re on ride number " + ride);
}
Output:
You’re on ride number 1
You’re on ride number 2
You’ve had enough fun, exiting the ride.
Continue Statement: Skipping an Iteration
Maybe there’s one ride you’d prefer to skip. The continue
statement allows you to skip the current iteration and move on to the next.
for (let ride = 1; ride <= 5; ride++) {
if (ride === 3) {
console.log("You decide to skip ride number 3.");
continue; // Skip this iteration
}
console.log("You’re on ride number " + ride);
}
Output:
You’re on ride number 1
You’re on ride number 2
You decide to skip ride number 3.
You’re on ride number 4
You’re on ride number 5
Riding the Loops in JavaScript
In this first part of our journey through loops, you’ve learned the basics of for loops, while loops, and do-while loops. You’ve also gained control over your loops with break and continue, just like deciding when to stay on or get off the Ferris wheel.
Now, we’ll explore more advanced looping techniques, including the for-in and for-of loops, which let you iterate over objects and arrays in new ways.
Advanced Spins: Introducing For-In and For-Of Loops
Now that you’re familiar with the basics of looping through data using for, while, and do-while loops, it’s time to explore more advanced loops that will make your life easier when dealing with objects and arrays.
Unlike the traditional for
loop that requires you to manage an index manually, for-in and for-of loops allow you to iterate over the properties or values of objects and arrays seamlessly. Think of these loops as automated Ferris wheels where you don’t need to keep track of how many spins you’ve made—the loop takes care of it.
The For-In Loop — Inspecting Your Environment
The for-in loop is perfect for when you want to loop through the properties of an object. Imagine it as inspecting every part of a Ferris wheel before starting the ride—you want to check every seat, control panel, and light to ensure everything is in place.
Basic Syntax:
for (let property in object) {
// Code to execute for each property
}
For-in loops allow you to loop over an object’s enumerable properties. It gives you access to each property name (or key), one at a time.
Code Snippet: Using For-In with Objects
Let’s imagine you’re in charge of inspecting a Ferris wheel’s control system. You want to loop through every part of the system and check its status:
let ferrisWheel = {
seats: 20,
speed: "medium",
lightsOn: true
};
for (let part in ferrisWheel) {
console.log(part + ": " + ferrisWheel[part]);
}
Explanation:
The
for-in
loop iterates over each property (seats
,speed
,lightsOn
) in theferrisWheel
object.It prints the property name (key) along with its corresponding value.
Output:
seats: 20
speed: medium
lightsOn: true
The For-Of Loop — Riding the Data Ferris Wheel
While for-in is great for objects, the for-of loop is ideal when you want to loop over iterable objects like arrays, strings, or sets. It allows you to directly access each element in a sequence—like taking a ride on the Ferris wheel and focusing on each view as it comes around.
Basic Syntax:
for (let element of iterable) {
// Code to execute for each element
}
For-of loops let you iterate over the values directly, unlike for-in
, which gives you keys or property names.
Using For-Of with Arrays
Imagine you’re listing the passengers taking a ride on the Ferris wheel:
let passengers = ["Alice", "Bob", "Charlie"];
for (let passenger of passengers) {
console.log(passenger + " is riding the Ferris wheel.");
}
Explanation:
The
for-of
loop iterates over thepassengers
array.It directly accesses each element (the names of the passengers) and prints a message for each.
Output:
Alice is riding the Ferris wheel.
Bob is riding the Ferris wheel.
Charlie is riding the Ferris wheel.
Break and Continue in For-Of and For-In
The break and continue statements that you learned in Part 1 can also be used within for-in and for-of loops. Let’s explore how they can be applied to skip or exit these loops.
Skipping an Entry with Continue
What if you wanted to skip a certain property or value in a loop? For instance, you may want to skip over any seat that’s out of order.
let seats = ["Seat 1", "Seat 2", "Broken Seat", "Seat 4"];
for (let seat of seats) {
if (seat === "Broken Seat") {
console.log(seat + " is out of order, skipping...");
continue; // Skip the broken seat
}
console.log(seat + " is ready for passengers.");
}
Output:
Seat 1 is ready for passengers.
Seat 2 is ready for passengers.
Broken Seat is out of order, skipping...
Seat 4 is ready for passengers.
In this example, the continue
statement allows us to skip over "Broken Seat" and move on to the next iteration.
Looping with Arrays and Objects — The Magic of Iteration
Now that we’ve seen the basics of looping with objects and arrays, let’s dig a bit deeper into how these loops can help you iterate through more complex data structures.
Nested Loops with Arrays of Objects
Often, you’ll find yourself working with more complex data structures, such as an array of objects. In such cases, you can use nested loops to iterate through each item in the array and its properties.
Let’s say you’re managing a series of Ferris wheel rides, and each ride has details about the passengers on board. You want to loop through each ride and print out the names of the passengers.
Code Snippet: Nested For-Of Loop Example
let rides = [
{ rideNumber: 1, passengers: ["Alice", "Bob"] },
{ rideNumber: 2, passengers: ["Charlie", "David"] }
];
for (let ride of rides) {
console.log("Ride " + ride.rideNumber + ":");
for (let passenger of ride.passengers) {
console.log(" - " + passenger);
}
}
Output:
codeRide 1:
- Alice
- Bob
Ride 2:
- Charlie
- David
Here, we use a nested for-of loop to iterate over each ride, and then another loop to access each passenger on that ride.
Challenge Time — Create a Countdown Timer
Let’s put what we’ve learned into practice with a challenge. Your task is to create a simple countdown timer using a while loop.
Challenge 1 : Countdown Timer
Scenario: You’re about to launch a Ferris wheel ride, but you need to count down from 5 before the ride starts.
Challenge Code:
let countdown = 5;
while (countdown > 0) {
console.log("Starting in " + countdown + "...");
countdown--;
}
console.log("The ride has started!");
Explanation:
The
while
loop runs as long ascountdown
is greater than 0.Each time the loop runs, the value of
countdown
decreases by 1.Once the countdown reaches 0, the loop exits, and the ride begins.
Challenge 2: Skip Every Third Ride
Scenario: You’re in charge of rides, and every third ride needs to be skipped for maintenance. Write a loop that skips over every third ride.
Challenge Code:
let totalRides = 10;
for (let ride = 1; ride <= totalRides; ride++) {
if (ride % 3 === 0) {
console.log("Ride " + ride + " is skipped for maintenance.");
continue; // Skip every third ride
}
console.log("Ride " + ride + " is operational.");
}
Challenge 3: You have a list of rides, each with a duration in minutes. Write a loop to find the longest ride duration.
Challenge 4: You’re preparing a game, and you need to reverse a string (such as the name of a player). Write a loop to reverse the string.
Mastering Looping in JavaScript
Congratulations! You’ve now mastered the fundamentals and advanced concepts of loops in JavaScript. From for, while, and do-while loops to more specialized tools like for-in and for-of, you’ve learned how to iterate through data efficiently.
By controlling loops with break and continue, and nesting loops for more complex scenarios, you’ve gained a deeper understanding of how loops keep your programs running smoothly—just like the continuous turning of a Ferris wheel.
In the next article, we’ll dive into collections—exploring arrays, maps, sets, and more. You’ll learn how to store and manipulate data in powerful ways to build even more interactive and dynamic programs.
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by