Building a Remote Code Collaboration Tool: A Comprehensive Guide
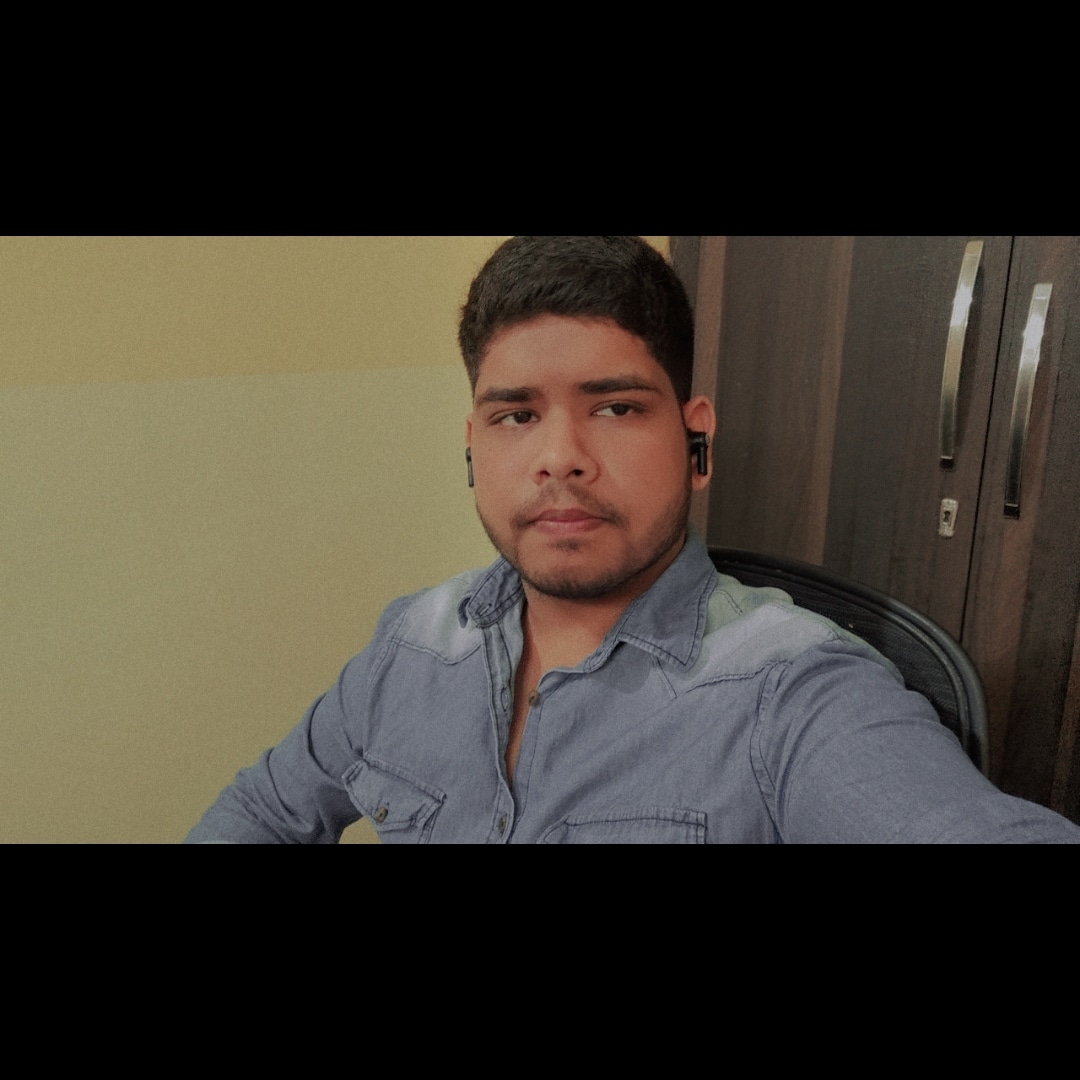
Introduction
In today’s fast-paced software development environment, collaboration is key to productivity. I embarked on creating a Remote Code Collaboration Tool that allows multiple users to code together in real-time. This article provides a detailed walkthrough of the technologies used, the challenges faced, and the key features of the application.
Technologies Used
Frontend
React: For building a responsive user interface.
CodeMirror: A versatile text editor that supports syntax highlighting for various programming languages.
React Router: For seamless navigation between components.
Socket.IO: To enable real-time communication between users.
Backend
Node.js: For setting up the server.
Express: A minimal web framework for building the API.
Socket.IO: For managing real-time interactions.
Judge0 API: For executing code in various programming languages and returning the output.
Project Structure
The project is structured with a clear separation between the frontend and backend components. Here's a brief overview of the directory structure:
/remote-code-collaboration-tool
├── /client # Frontend code
└── /server # Backend code
Key Features
Real-Time Collaboration: Users can join a room and edit code simultaneously, making collaboration effortless.
Syntax Highlighting: CodeMirror provides syntax highlighting for various languages, enhancing the coding experience.
Code Execution: Users can run their code snippets directly from the editor and see the results instantly.
User Management: Each user can join using a unique Room ID and a chosen username, ensuring a personalized experience.
Member Notifications: The application provides notifications when users join or leave the room, keeping everyone informed.
Implementation Details
Setting Up the Frontend
Here’s a snippet of the code for the Home
component, which allows users to enter the Room ID and their username:
const Home = () => {
const navigate = useNavigate();
const [roomid, setroomid] = useState("");
const [username, setusername] = useState("");
const roomidchange = (e) => {
setroomid(e.target.value);
};
const usernamechange = (e) => {
setusername(e.target.value);
};
const joinroom = (event) => {
if (!roomid || !username) {
toast.error("Both fields are required.");
return;
}
navigate(`/editor/${roomid}`, { state: { username, roomid } });
toast.success("Entered the room");
};
// ... (other code)
};
Handling Real-Time Communication
To facilitate real-time interactions, I utilized Socket.IO for managing user connections. Here’s a snippet from the backend server setup:
const io = require("socket.io")(server);
io.on("connection", (socket) => {
console.log(`User connected: ${socket.id}`);
socket.on("join-room", (roomid, username) => {
socket.join(roomid);
io.to(roomid).emit("user-connected", username);
});
socket.on("disconnect", () => {
console.log(`User disconnected: ${socket.id}`);
});
});
Error Handling and Challenges
While developing the application, I encountered several challenges:
Real-Time Data Sync: Ensuring that all users see the same version of the code required careful handling of Socket.IO events.
Code Execution Errors: Integrating the Judge0 API sometimes led to errors, especially with unsupported languages or syntax issues. I implemented error handling to provide users with meaningful feedback.
UI Responsiveness: Making sure the UI was responsive and user-friendly required extensive testing and adjustments.
Conclusion
The Remote Code Collaboration Tool is designed to enhance coding collaboration among users, making it easier to work on projects together, especially in remote settings. The application is fully functional, but I plan to improve it further with additional features and enhancements.
You can find the source code for this project on my GitHub repository. I will be deploying the application soon, making it accessible to users for collaborative coding experiences.
Thank you for reading! If you have any questions or feedback, feel free to reach out.
Subscribe to my newsletter
Read articles from Arham Iqbal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
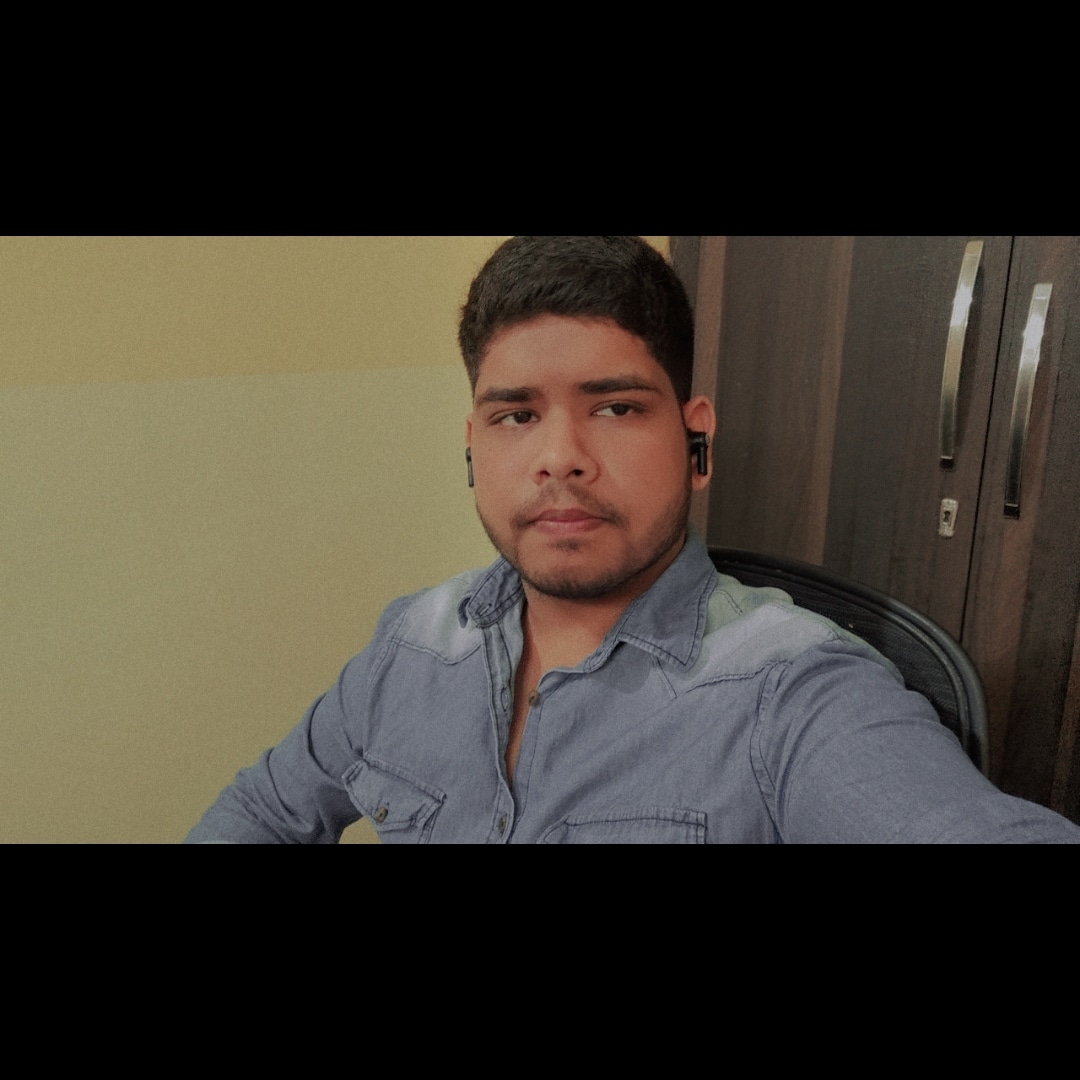
Arham Iqbal
Arham Iqbal
Fueled by a passion for both DevOps and full-stack development, I specialize in transforming complex workflows and optimizing cloud infrastructures while building dynamic web applications. My expertise in DevOps includes automating pipelines and leveraging cutting-edge cloud technologies to drive efficiency and scalability. As a dedicated full-stack MERN developer, I excel in crafting robust applications using MongoDB, Express.js, React.js, and Node.js. Combining my skills in modern DevOps practices with my full-stack development capabilities, I strive to deliver innovative, scalable solutions that push the boundaries of technology. Eager to connect and explore exciting opportunities to advance in the tech world.