Java Memory Management: When to Call the Garbage Collector and When to Call Your Therapist!
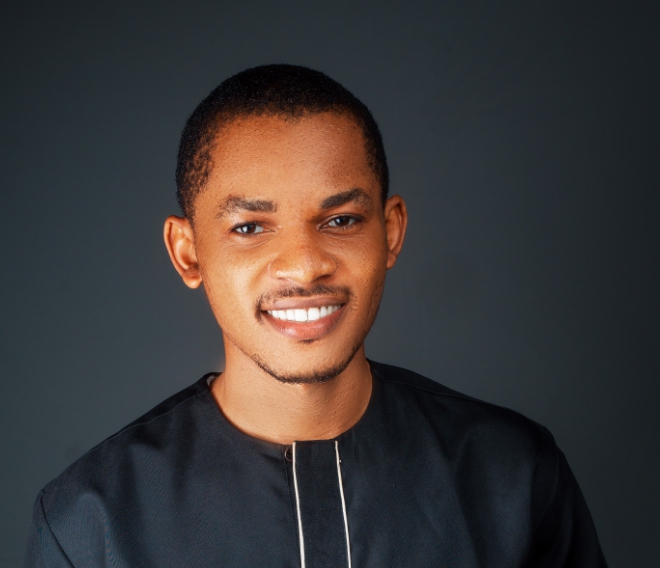
Hey there, Java dev! Have you ever been minding your own business, writing code, and then BAM! — out of nowhere, the dreaded “OutOfMemoryError” rears its ugly head? It’s like an uninvited guest who shows up right when you’re about to enjoy a smooth code session. Memory management in Java can feel like this invisible frenemy; you don’t notice it… until it decides to wreak havoc. But don’t worry! Mastering memory management will let you take control of the chaos and keep your apps running like a well-oiled machine. In this article, we’ll dive into Java’s memory principles, share some top-notch optimization techniques, and show you what happens when memory isn’t managed properly (spoiler: it’s not pretty).
Understanding Memory in Java
First up, let's uncover the secrets of Java's memory magic. Java’s memory is like a busy office with two main departments: the Heap and the Stack.
The Heap is where objects live, happily storing all the stuff you tell Java to remember. The Garbage Collector (GC) is the office janitor who’s always tidying up by removing objects that have overstayed their welcome.
The Stack is like the post-it note section, handling method calls and local variables. Whenever you call a method, a new “stack frame” post-it pops up, holding important notes until the method finishes. Then, off it goes!
Java’s GC does a solid job keeping things tidy most of the time, but it can only do so much. If memory isn’t optimized, you might end up with excessive GC cycles or worse — memory leaks that slow down your app faster than you can say OutOfMemoryError
.
The Perils of Poor Memory Management
When memory is mismanaged, it’s like leaving the office fridge full of expired takeout. Eventually, it stinks up the place and someone’s got to deal with it. In Java, this can mean memory leaks, sluggish performance, or the dreaded OutOfMemoryError. Let’s steer clear of the funk with these five memory-saving hacks!
Memory Optimization Techniques in Java
1. Use StringBuilder for String Manipulation
Strings in Java are as stubborn as your old, single-serve coffee maker — they won’t change. Every time you modify a String, Java creates a brand new one. Multiply this by a loop, and you’ve got a heap full of leftovers.
To save memory, try using StringBuilder
instead:
javaCopy codeStringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("Number: ").append(i).append("\n");
}
String result = sb.toString();
Using StringBuilder
lets you reuse the same object, cutting down on needless memory clutter. It’s like drinking from a refillable mug instead of using 1000 paper cups!
2. Avoid Creating Unnecessary Objects
Creating objects like they’re going out of style? Java’s Heap isn’t a bargain store — it’ll eventually get crowded! Instead of creating a new object each time in a loop, reuse an existing one where you can.
Instead of doing this:
javaCopy codefor (int i = 0; i < 1000; i++) {
Point p = new Point(0, 0);
// Do something with p
}
Do this:
javaCopy codePoint p = new Point(0, 0);
for (int i = 0; i < 1000; i++) {
p.setLocation(0, 0);
// Do something with p
}
Less clutter, less GC cleanup. Think of it as reusing your old grocery bags instead of filling up with new ones each time.
3. Use Weak References for Caching
Want to save memory without manually clearing out your cache? Enter the WeakReference
— your app’s “use it or lose it” storage. Weak references allow objects to be GC’ed when memory is tight, keeping your app from hoarding data it doesn’t need.
javaCopy codeimport java.lang.ref.WeakReference;
Map<String, WeakReference<ExpensiveObject>> cache = new HashMap<>();
cache.put("key1", new WeakReference<>(new ExpensiveObject()));
With weak references, your cache will keep important data close but will gladly hand it over if memory is in demand. It’s like a fridge that magically throws out leftovers before they spoil!
4. Use Primitive Data Types Instead of Wrappers
If your app crunches numbers like a calculator on steroids, using wrapper classes (Integer
, Double
, etc.) could drain memory faster than you can say int
. Switch to primitive types for lighter, faster data handling.
For example, use int[]
instead of Integer[]
whenever possible. Less bloat, more power.
5. Optimize Collection Sizes with initialCapacity
Collections like ArrayList
and HashMap
will grow dynamically, but each resize requires extra memory. Give them an initialCapacity
if you know how big they’ll get. It’s like ordering the large pizza when you know everyone’s starving.
javaCopy codeList<String> list = new ArrayList<>(100); // Enough space for 100 elements
Setting an initial capacity means less time spent resizing — and more time spent doing actual work.
Conclusion
Memory management in Java can be a balancing act, but it doesn’t have to be a mystery. By wielding, reusing objects, and using memory-savvy techniques like weak references, your app can stay lean and quick. Remember, just because the GC is watching doesn’t mean you can’t lend it a helping hand.
Happy coding! And next time memory issues arise, you’ll be ready to handle them like a pro (or at least give the GC a little less to stress about).
Subscribe to my newsletter
Read articles from Chinemeze ThankGod directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
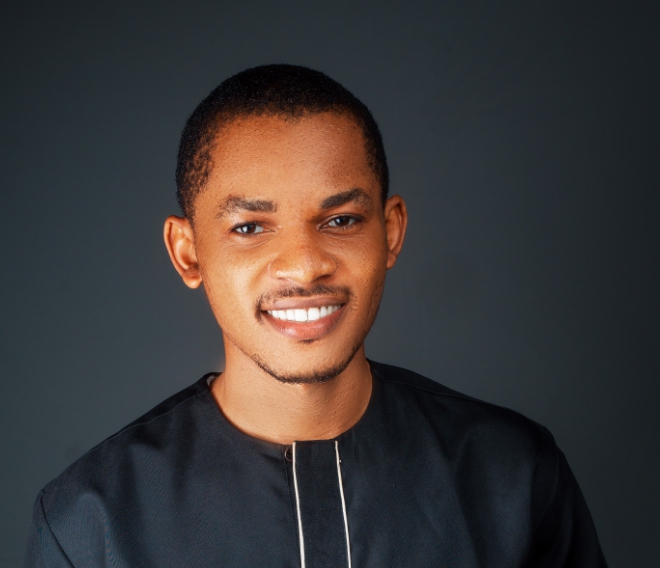
Chinemeze ThankGod
Chinemeze ThankGod
Software Engineer | DevOps Engineer | Problem Solver | Mentor | Systems Designer | Tech Enthusiast With 5 years of experience in software development, I specialize in creating robust Java applications and solving complex problems. I have a passion for teaching and mentoring, having guided many aspiring tech professionals on their journey. In addition to coding, I enjoy writing technical articles, indulging in music, and tackling challenging math problems. Always eager to learn and share knowledge, I thrive on innovation and creativity in the tech world.