Creating an e-commerce platform with Laravel
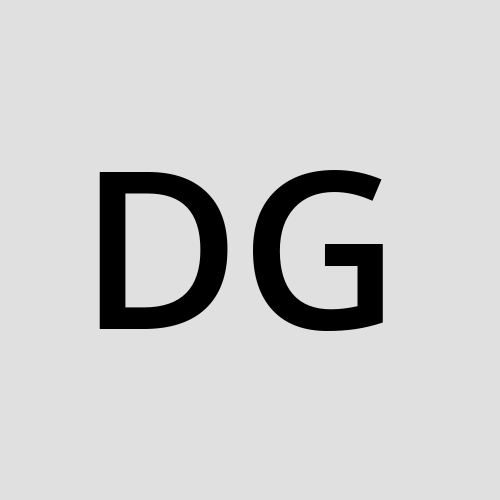
Creating an e-commerce platform with Laravel involves several key steps. Here's a high-level outline to guide you through the process, from setting up the initial project to implementing essential e-commerce features.
1. Setting Up the Laravel Project
Install Laravel: Start by installing Laravel using Composer.
composer create-project laravel/laravel ecommerce-platform
Configure Environment: Set up your
.env
file with the necessary configurations:Database credentials
Email service provider for notifications and order confirmations
Stripe or PayPal API keys for payment processing
2. Setting Up the Database and Models
Database Design: Define tables for
users
,products
,categories
,orders
,order_items
,payments
, etc.Migrations: Create migrations for each table.
php artisan make:migration create_products_table php artisan make:migration create_orders_table
Models and Relationships:
Create models for each main entity: Product, Order, User, Cart, etc.
Define relationships in the models:
// Product.php public function category() { return $this->belongsTo(Category::class); } // Order.php public function user() { return $this->belongsTo(User::class); } public function items() { return $this->hasMany(OrderItem::class); }
3. Authentication and User Management
Authentication: Use Laravel Breeze, Jetstream, or Laravel UI to scaffold authentication features.
composer require laravel/breeze php artisan breeze:install php artisan migrate
Roles and Permissions: Set up roles (e.g., customer, admin) using Laravel's built-in authorization features or a package like
spatie/laravel-permission
.
4. Building the Product and Category Management System
Admin Panel: Create a backend admin dashboard where admins can:
Add, edit, delete products
Organize products by category
Product Model and Controller:
php artisan make:model Product -mcr
Image Uploads: Implement a file upload feature for product images using Laravel’s
Storage
facade.
5. Shopping Cart Implementation
Session-Based Cart: Create a shopping cart system that stores items in the session.
Add/Update/Remove Items: Define routes and methods for adding, updating, and removing items from the cart.
Cart Model: Optional, you can create a
Cart
model and persist cart data in the database if you want to save user carts across sessions.
6. Checkout and Order Processing
Order Form: Create a checkout page where users enter their shipping and payment details.
Order and Order Items Models: Define an
Order
model and associatedOrderItem
model.Order Confirmation: After successful payment, save the order details in the database and send an email confirmation to the user.
7. Payment Integration
Stripe or PayPal: Integrate a payment gateway like Stripe or PayPal.
Install the Stripe package:
composer require stripe/stripe-php
Create routes and controllers to handle payment processing.
Payment Controller: Implement logic to create a payment intent and complete the payment.
Order Status: Update the order status to "completed" after successful payment.
8. Order Management and User Dashboard
Order Tracking: Allow users to view their past orders and track current orders.
Admin Order Management: Create an order management dashboard for admins to view, manage, and update orders.
Notifications: Set up notifications for order confirmation, shipment updates, etc.
9. Product Search and Filtering
Search Feature: Implement a search bar to allow users to search for products by name, description, or category.
Filtering: Add filters for categories, price range, and product attributes.
Sorting: Enable sorting options for price, popularity, or newest products.
10. Frontend Design
Laravel Blade Templates: Use Blade templating for the frontend.
UI Framework: Consider using Tailwind CSS, Bootstrap, or custom CSS to style your e-commerce platform.
Product Pages: Create detailed product pages with images, descriptions, reviews, and an “Add to Cart” button.
11. Testing and Deployment
Testing: Use PHPUnit and Laravel’s built-in testing features to test key functionalities.
Deployment: Deploy the application using services like Laravel Forge, DigitalOcean, or AWS.
12. Advanced Features (Optional)
Discounts and Coupons: Implement a system for discounts and coupon codes.
Product Reviews and Ratings: Allow users to leave reviews and rate products.
Wishlist: Enable users to add products to a wishlist.
Inventory Management: Set up automatic inventory tracking and notifications for low stock.
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
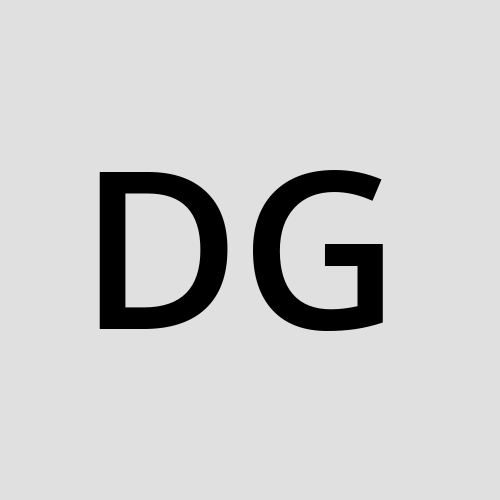
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.