Immutable Data Structures in JavaScript: Unlocking Consistency and Performance

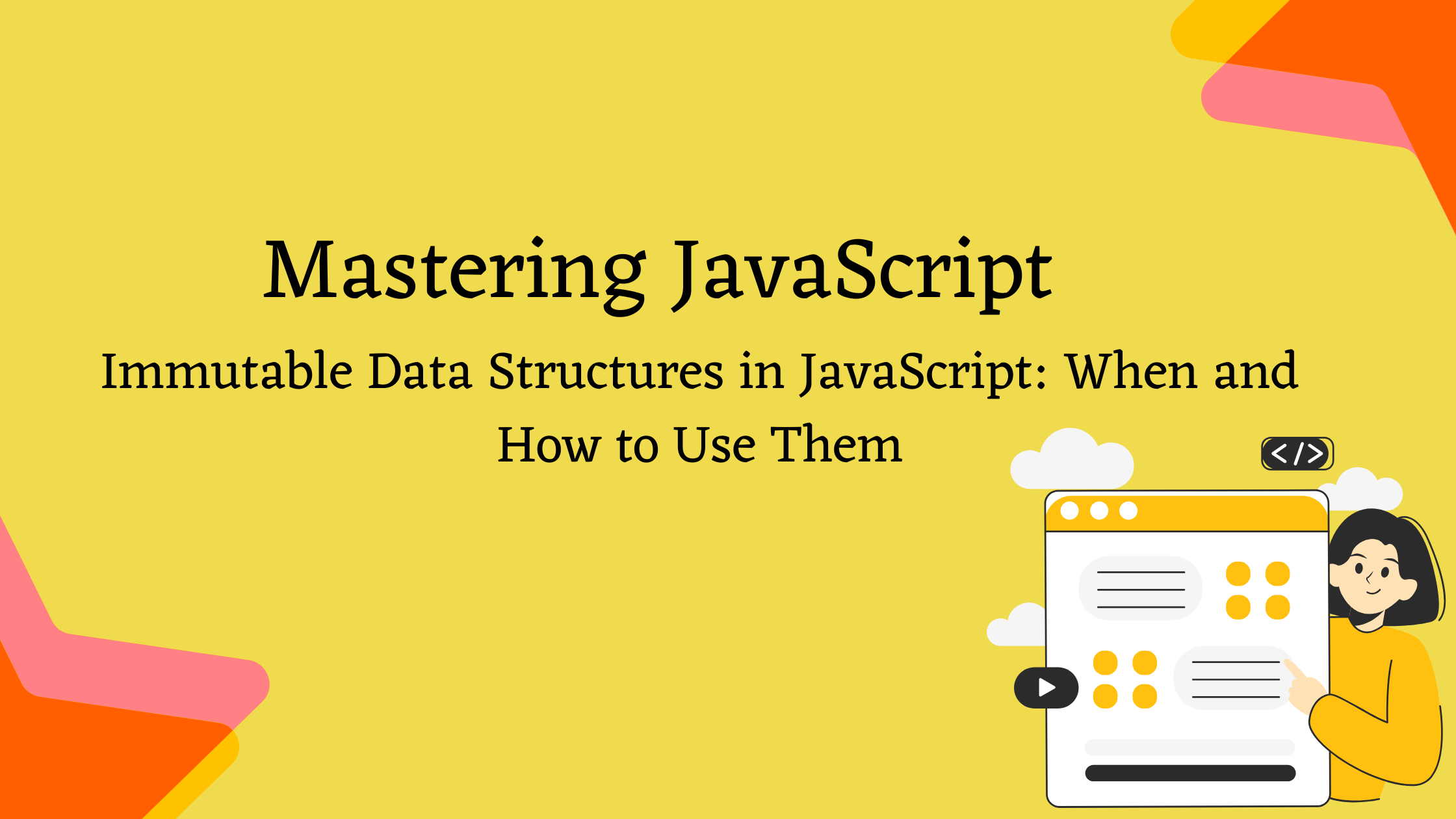
Introduction
In modern JavaScript development, especially in large-scale applications, data consistency and reliability are essential. One way to achieve this is by using immutable data structures, which prevent changes to data once it has been created. This means that instead of modifying an existing object or array, a new version of it is created every time a change is needed. This approach can lead to more predictable, maintainable, and bug-free code, especially in state management systems like React or Redux. But when and how should we use immutable data structures in JavaScript?
Let’s explore immutable data structures, their practical use cases, and the benefits and drawbacks of using them.
What Are Immutable Data Structures?
In JavaScript, most data types (like objects and arrays) are mutable by default. This means you can alter them after they’ve been created:
let user = { name: "John", age: 30 };
user.age = 31; // Mutating the object
In contrast, an immutable data structure doesn’t allow changes to the original object. If you want to "change" it, you create a new object with the updated value instead. JavaScript doesn’t natively support immutable data structures, but you can achieve immutability through patterns, libraries (such as Immutable.js), or simply by using techniques like Object.freeze()
.
For example:
const user = { name: "John", age: 30 };
const newUser = { ...user, age: 31 }; // Creating a new object with an updated age
Here, newUser
is a new object, while user
remains unchanged, demonstrating immutability.
Practical Use Cases for Immutable Data Structures
1. State Management in Front-End Frameworks
In frameworks like React and Redux, immutability is crucial for tracking state changes. Redux, for instance, follows a strict rule that the state should never be mutated directly, and every state update should produce a new state object. This makes it easier to debug and track state changes, and it improves performance through optimizations like shallow comparison (since a new reference signals that the data has changed).
Example in Redux:
const initialState = { count: 0 };
function reducer(state = initialState, action) {
switch (action.type) {
case "INCREMENT":
return { ...state, count: state.count + 1 }; // returning a new state object
default:
return state;
}
}
2. Concurrency and Multi-Threading
When dealing with concurrent operations or multi-threaded environments, immutability prevents race conditions or unpredictable behavior. Since data cannot be altered once created, different threads or asynchronous processes can access the same data safely without worrying about unwanted mutations.
3. Functional Programming
JavaScript supports functional programming principles, which often rely on immutability. In functional programming, functions should not have side effects, meaning they shouldn’t modify external variables. Using immutable data ensures functions return new data instead of changing the input, preserving pure functions and making code more predictable.
Benefits of Using Immutable Data Structures
1. Predictability and Debugging
Immutability ensures that data remains consistent throughout your application, which makes it easier to reason about. When state or data structures can’t be changed once created, you eliminate bugs that arise from unexpected mutations.
For instance, in mutable code, changes can happen unexpectedly:
const arr = [1, 2, 3];
const newArr = arr;
newArr.push(4); // This also changes `arr`, which may cause confusion.
Using immutability avoids such scenarios and ensures that each part of your application is working with data it expects.
2. Performance Optimizations
In frameworks like React, shallow comparisons are used to decide whether to re-render components. With immutability, the framework can quickly determine if something has changed just by comparing object references, making applications faster.
// With immutability
if (prevState !== currentState) {
// State has changed
}
This is more efficient than deeply comparing the properties of an object.
3. Concurrency and Parallelism
Immutable data structures are inherently thread-safe. When working with multiple threads or asynchronous code, you don’t have to worry about one thread or function changing data in a way that affects other parts of your application.
Drawbacks of Using Immutable Data Structures
While immutability has significant advantages, it’s not without its drawbacks:
1. Memory Overhead
Since every change to an object creates a new object, immutability can increase memory usage, especially if large or complex data structures are being duplicated frequently.
const original = { name: "Alice", age: 25 };
const updated = { ...original, age: 26 }; // Creates a new object
For large objects or data structures, this can become expensive in terms of memory.
2. Performance Costs
Creating new objects on every change can also lead to performance issues. In performance-critical applications, the cost of cloning large data structures repeatedly may outweigh the benefits of immutability.
3. Learning Curve
Developers accustomed to mutable data structures may initially find immutability difficult to work with. It requires adopting new patterns and practices, such as using spread operators, methods like map()
, filter()
, or third-party libraries to handle immutable data.
Conclusion
Immutable data structures bring significant benefits to JavaScript development, particularly in state management, concurrency, and functional programming. By preventing unwanted side effects and making data flow predictable, immutability leads to more robust and maintainable code. However, it’s important to consider the trade-offs, especially in terms of memory usage and performance. In large-scale production environments, immutability can enhance scalability and stability but should be implemented thoughtfully to avoid potential drawbacks.
In summary, while immutable data structures are not always necessary, they can be a powerful tool in your development toolbox when applied in the right scenarios.
Thank You!
Thank you for reading!
I hope you enjoyed this post. If you did, please share it with your network and stay tuned for more insights on software development. I'd love to connect with you on LinkedIn or have you follow my journey on HashNode for regular updates.
Happy Coding!
Darshit Anjaria
Subscribe to my newsletter
Read articles from Darshit Anjaria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshit Anjaria
Darshit Anjaria
An experienced professional with 5.5+ years in the industry, adept at collaborating effectively with developers across various domains to ensure timely and successful project deliveries. Proficient in Android/Flutter development and currently excelling as a backend developer specializing in Node.js. I bring a strong enthusiasm for learning new frameworks, paired with a quick-learning mindset and a passion for writing bug-free, optimized code. I am always ready to adapt to and learn cloud technologies, ensuring continuous growth and improvement. I actively contribute to communities by writing insightful articles on my blog and am seeking support from you all to create more valuable content and tutorials like this.