Creating an Accordion Component in React: A Step-by-Step Guide

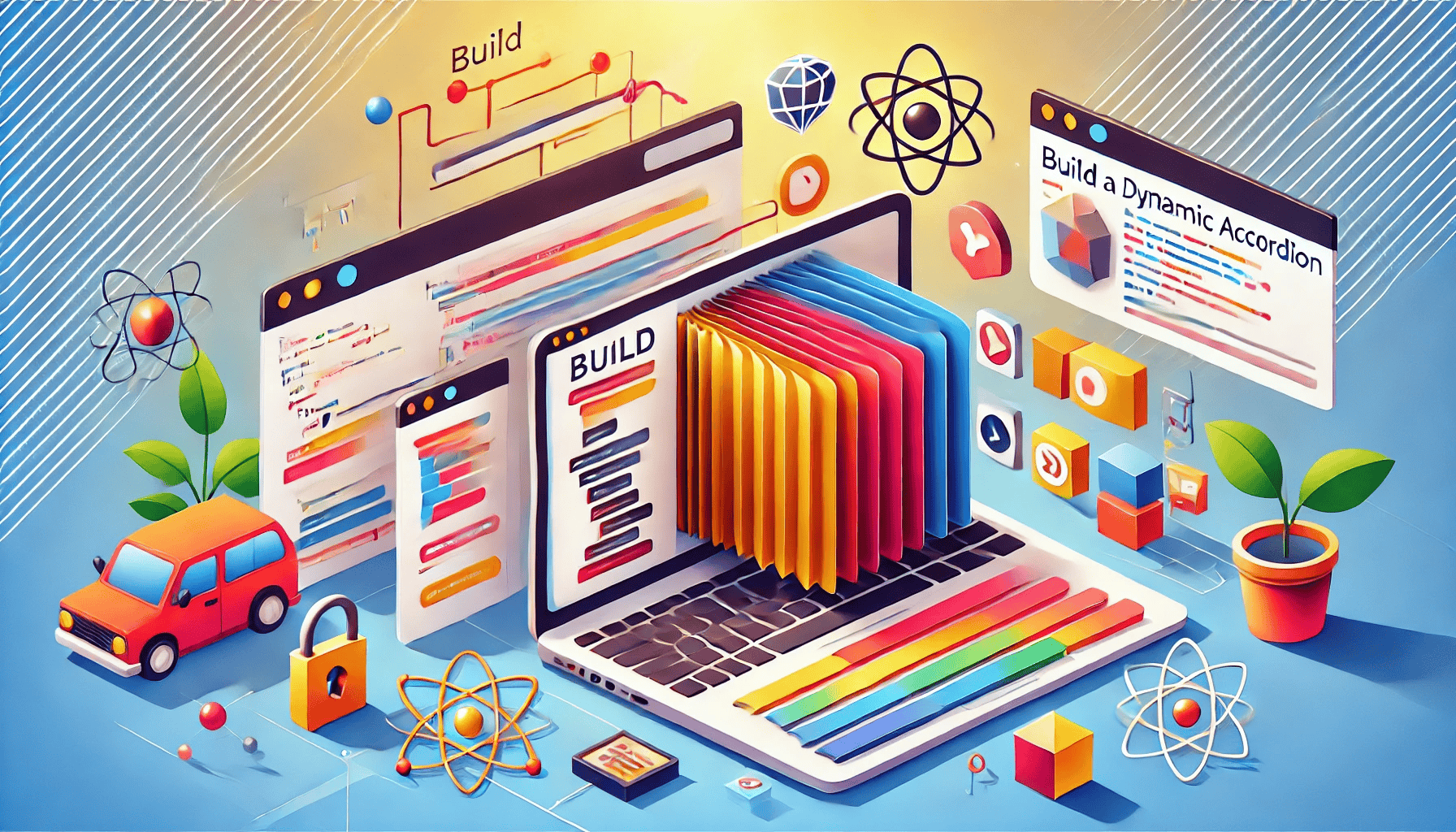
Hello, everyone! It's Amar Jondhalekar here, and today I'm excited to share with you how to build a dynamic accordion component using React. Accordions are a fantastic way to organize information in a compact space, making it easier for users to navigate and interact with your content. So, let’s dive right in!
What is an Accordion?
An accordion is a UI component that allows users to expand and collapse sections of content. This interaction helps manage large amounts of information, presenting it in a cleaner and more digestible format.
Why Use an Accordion?
Improves User Experience: By hiding and showing content as needed, you can create a cleaner interface.
Saves Space: It allows you to display a lot of information without overwhelming your users.
Easy Navigation: Users can quickly access the information they want without scrolling through long pages.
Getting Started
To create an accordion in React, we will use functional components and React's built-in hooks, specifically useState
. Here’s the complete code to get you started:
The Complete Code
import React, { useState } from 'react';
// Main Accordion Component
export default function Accordion() {
const [activeIndex, setActiveIndex] = useState(0); // Initialize active panel index
return (
<>
<h2>Almaty, Kazakhstan</h2>
<Panel
title="About"
isActive={activeIndex === 0}
onShow={() => setActiveIndex(0)}
>
With a population of about 2 million, Almaty is Kazakhstan's largest city. From 1929 to 1997, it was its capital city.
</Panel>
<Panel
title="Etymology"
isActive={activeIndex === 1}
onShow={() => setActiveIndex(1)}
>
The name comes from <span lang="kk-KZ">алма</span>, the Kazakh word for "apple" and is often translated as "full of apples."
</Panel>
</>
);
}
// Panel Component for each section of the Accordion
function Panel({ title, children, isActive, onShow }) {
return (
<section className="panel">
<h3>{title}</h3>
{isActive ? (
<p>{children}</p> // Show content when active
) : (
<button onClick={onShow}>Show</button> // Show button when inactive
)}
</section>
);
}
Explanation of the Code
Let’s break down the code to understand how it works.
Step 1: Setting Up State
In our Accordion
component, we initialize the state using useState
:
const [activeIndex, setActiveIndex] = useState(0);
activeIndex
: This state variable keeps track of which panel is currently expanded. By default, it is set to0
, meaning the first panel is open.
Step 2: Rendering the Panels
We render the Panel
components inside our Accordion
. Each panel has a title, a content section, and handlers to control its visibility:
<Panel
title="About"
isActive={activeIndex === 0} // Checks if this panel is active
onShow={() => setActiveIndex(0)} // Set this panel as active when clicked
>
{ /* Content here */ }
</Panel>
isActive
: This prop is a boolean that determines whether the panel should display its content. We compare the panel index toactiveIndex
.onShow
: This prop is a function that updatesactiveIndex
when the panel is clicked.
Step 3: Building the Panel Component
The Panel
component handles the display of each section:
function Panel({ title, children, isActive, onShow }) {
return (
<section className="panel">
<h3>{title}</h3>
{isActive ? (
<p>{children}</p> // Show content if active
) : (
<button onClick={onShow}>Show</button> // Show button if inactive
)}
</section>
);
}
title
: This prop displays the panel’s title.children
: This represents the content inside the panel, which will only be visible whenisActive
is true.
Customizing the Accordion
You can customize the accordion's appearance and behavior according to your application's needs. Here are a few ideas:
Styling: Use CSS to style the accordion for a more appealing look.
Animation: Consider adding smooth transitions to the opening and closing of panels.
Icons: Enhance usability by adding icons to indicate whether a panel is open or closed.
Conclusion
And that’s it! You now have a functional accordion component in React that you can easily integrate into your projects. This simple yet powerful UI element will help you present your information effectively.
Feel free to experiment with the code and make it your own. I hope you found this article helpful! If you have any questions or ideas, drop a comment below. Happy coding!
Subscribe to my newsletter
Read articles from Amar Jondhalekar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amar Jondhalekar
Amar Jondhalekar
👨💻 Amar Jondhalekar | Front End Web Developer at Cognizant | Content Creator | Founder at Campuslight With over 3 years of experience in web development, I specialize in HTML5, CSS3, JavaScript (ES6+), React.js, and Node.js. As a Full Stack Developer at Cognizant, I gained hands-on experience building dynamic, responsive applications focused on user-centric design and performance. I also founded Campuslight, where I create impactful digital solutions in the education sector, driven by a mission to make learning more accessible. Through my blogs, I share daily technical insights, coding tips, and career advice, aiming to inspire developers and impress recruiters alike. I’m dedicated to leveraging technology to create a more connected and accessible world. Let’s connect, collaborate, and make the web a better place for everyone!