Effective API Rate Limiting and Throttling Techniques for High-Traffic Backends
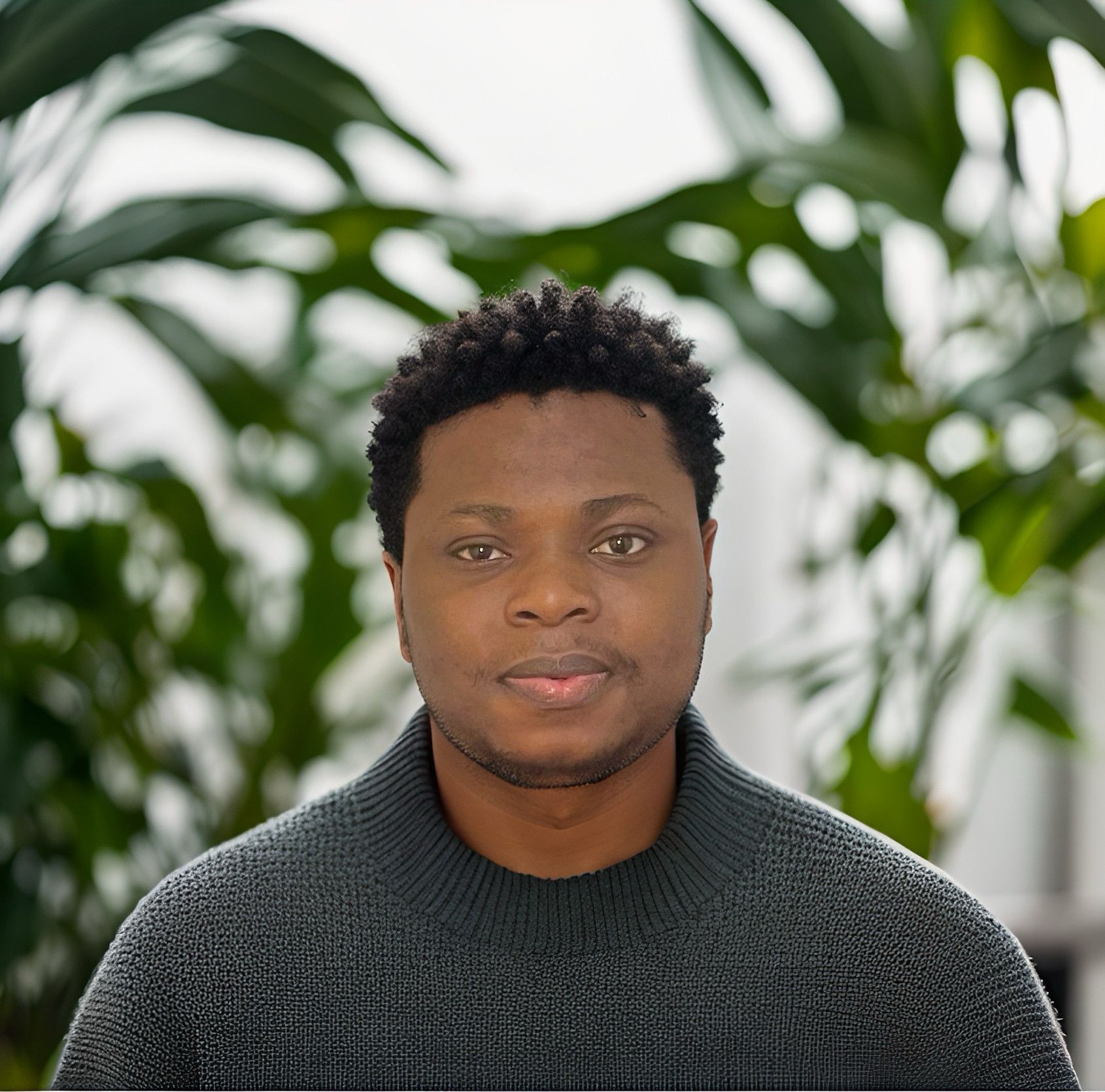
As backend services handle increasingly high traffic, protecting APIs from abuse and preventing resource overload becomes critical. Rate limiting and throttling are effective strategies to control the flow of requests, ensuring service stability and preventing unauthorized or excessive usage. This article explores various rate-limiting and throttling techniques, tools, and design patterns for handling high traffic, protecting backend resources, and managing different user access levels.
Why Rate Limiting and Throttling Matter
Rate limiting and throttling prevent excessive traffic from overloading backend servers. They help maintain API performance by restricting the number of requests a user, IP address, or API client can make within a specific time frame. Effective rate limiting strategies prevent:
API Abuse: Malicious actors and bots making unauthorized requests.
Service Overload: High traffic spikes, which can lead to downtimes.
Resource Management: Ensures fair use of backend resources for all users.
By using tools like NGINX, Redis, and API Gateways, you can implement these strategies in a flexible and scalable way to protect your services while providing a reliable user experience.
Key Rate Limiting and Throttling Techniques
Fixed Window Rate Limiting
Description: This approach limits requests within a fixed time interval, e.g., 100 requests per minute. It’s simple and commonly used but can be susceptible to bursts at the start and end of each window.
Example: Allow a maximum of 500 requests per hour from each IP address.
Sliding Window Rate Limiting
Description: Instead of a fixed interval, sliding windows calculate limits based on the current time and the number of requests within the last interval. This smooths out burst patterns.
Example: If a client has made 300 requests in the last 30 minutes, they may only make 200 more requests for the hour.
Token Bucket Algorithm
Description: This algorithm assigns tokens for each request, allowing up to a certain number within a set period. If the bucket has tokens available, the request proceeds. Otherwise, it is rejected or delayed until tokens are replenished.
Example: Allow 10 requests per second, replenished over time for burst traffic handling.
Leaky Bucket Algorithm
Description: The leaky bucket approach allows requests to pass at a fixed rate. Excess requests are queued or dropped, ensuring a constant rate rather than permitting bursts.
Example: A maximum of 5 requests per second, with any excess queued for later processing.
Tools for Implementing Rate Limiting and Throttling
1. Using NGINX for Rate Limiting
NGINX is a popular web server and reverse proxy that offers in-built rate-limiting features, making it ideal for controlling request flow at the edge level before they reach your backend.
Configuration Example:
http {
limit_req_zone $binary_remote_addr zone=api_zone:10m rate=10r/s;
server {
location /api/ {
limit_req zone=api_zone burst=20 nodelay;
proxy_pass http://backend;
}
}
}
In this example:
The
limit_req_zone
directive sets up a rate limit of 10 requests per second.burst=20
allows a burst of 20 requests at once, after which NGINX enforces the rate limit.nodelay
sends burst requests without delay until the burst limit is reached.
2. Using Redis for Distributed Rate Limiting
Redis is a highly performant, in-memory data store that can be used to implement distributed rate limiting, ideal for microservices or distributed environments.
How It Works:
Each time a request is made, a key (e.g.,
user:123:requests
) is incremented with an expiration set for the rate limit window.If the key exceeds the allowed rate, the request is rejected; otherwise, it proceeds.
Implementation Example:
const redis = require("redis");
const client = redis.createClient();
async function rateLimit(userId) {
const requests = await client.incr(userId);
if (requests === 1) {
await client.expire(userId, 60); // Set 1-minute expiration
}
if (requests > 100) {
throw new Error("Rate limit exceeded");
}
}
This example limits a user to 100 requests per minute. Redis is scalable, making it suitable for distributed applications where rate limiting must be enforced across multiple nodes.
3. Using API Gateway for Advanced Rate Limiting
API Gateways (such as AWS API Gateway or Kong) provide integrated rate-limiting features, ideal for handling complex access control policies and user quotas.
Example on AWS API Gateway:
- AWS API Gateway allows configuring rate limits and burst quotas per user or API key, with additional customization via usage plans.
How to Set Up:
Create a Usage Plan in API Gateway.
Attach API keys to users and configure their rate and burst limits under the usage plan.
API Gateway enforces these limits automatically, reducing load on your backend.
Rate Limiting Design Patterns
Tiered Rate Limiting
For applications with different user tiers (e.g., free, premium), set distinct rate limits for each tier. For example:
Free Tier: 100 requests per minute.
Premium Tier: 1000 requests per minute.
This approach aligns resource usage with user type, ensuring premium users receive prioritized access while controlling costs for free-tier users.
Geo-Based Rate Limiting
Limiting requests based on geographic location can help prevent abuse from certain regions without affecting overall service performance. Use geo-based rate limiting with tools like NGINX’s GeoIP module or API Gateway for specific countries or regions.
Monitoring and Managing Rate Limits
Monitoring rate limits is essential for maintaining the effectiveness of your throttling strategies. Use monitoring tools to analyze traffic patterns and adjust your rate-limiting thresholds based on real-world usage.
Metrics to Track
Request Rate: Track requests per second/minute for each endpoint.
Throttle Counts: Count how often rate limits are reached to identify overuse.
Latency: Monitor response times to ensure rate limiting doesn’t slow down requests.
Error Rates: Watch for increased error rates, which could indicate rate limiting issues.
Tooling for Monitoring
Prometheus and Grafana: Use Prometheus to scrape metrics from your backend and Grafana to visualize traffic, rate-limiting events, and latency.
DataDog: Track API usage and set alerts for when rate limits are frequently hit, which could indicate abusive patterns.
CloudWatch (for AWS): Monitor throttling metrics and set up automated responses if limits are exceeded frequently.
Conclusion
Implementing effective rate limiting and throttling strategies can safeguard your backend against abuse, prevent downtime, and provide a consistent experience for users across different traffic conditions. Using tools like NGINX, Redis, and API Gateways provides a scalable, flexible way to enforce rate limits, manage quotas for user tiers, and monitor traffic patterns. By adopting a layered approach that combines these tools and monitoring solutions, you’ll be well-equipped to handle high traffic and maintain your service’s performance.
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
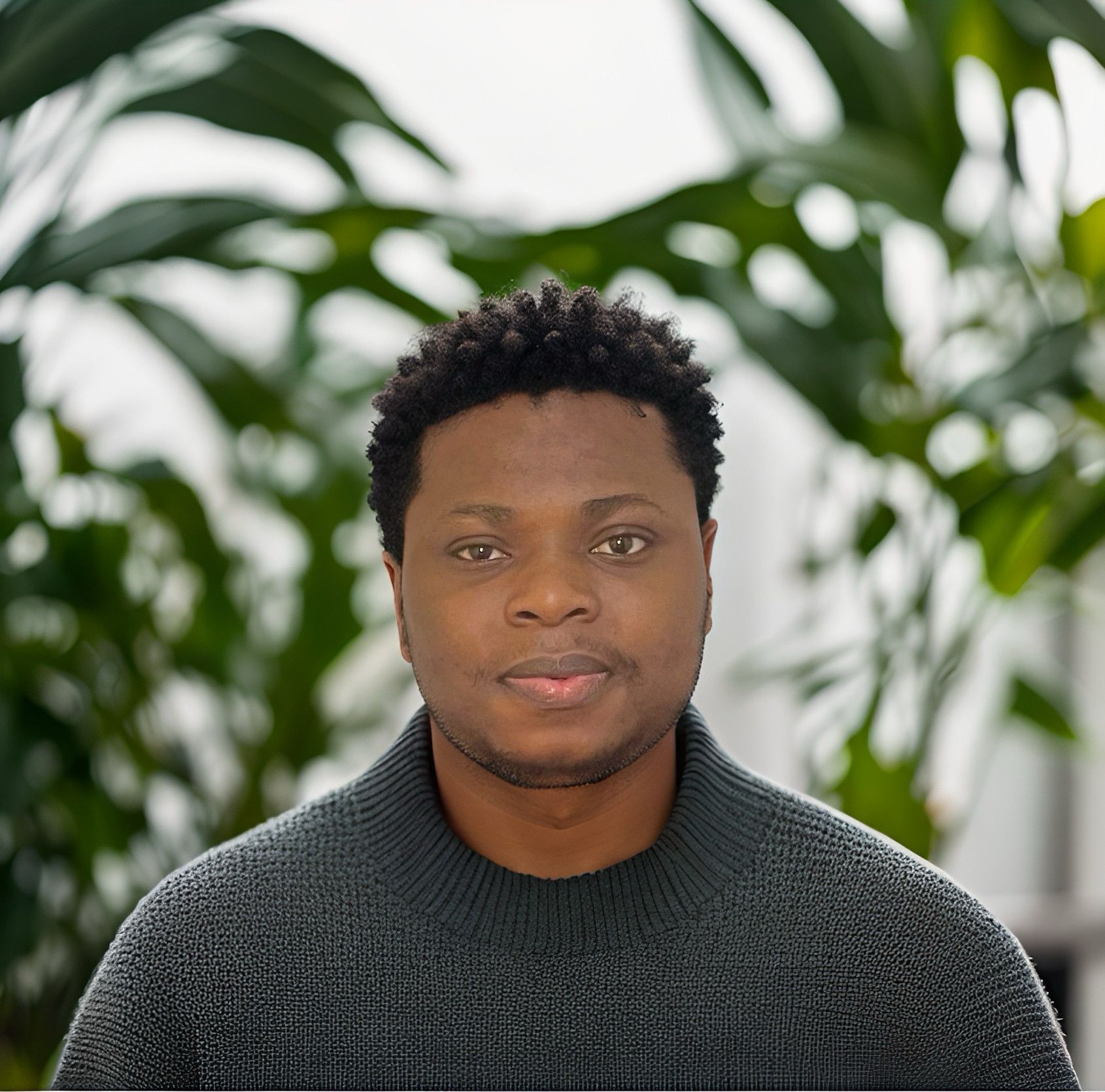