CI/CD Pipelines for Database Changes: Safe Schema Migrations and Rollbacks
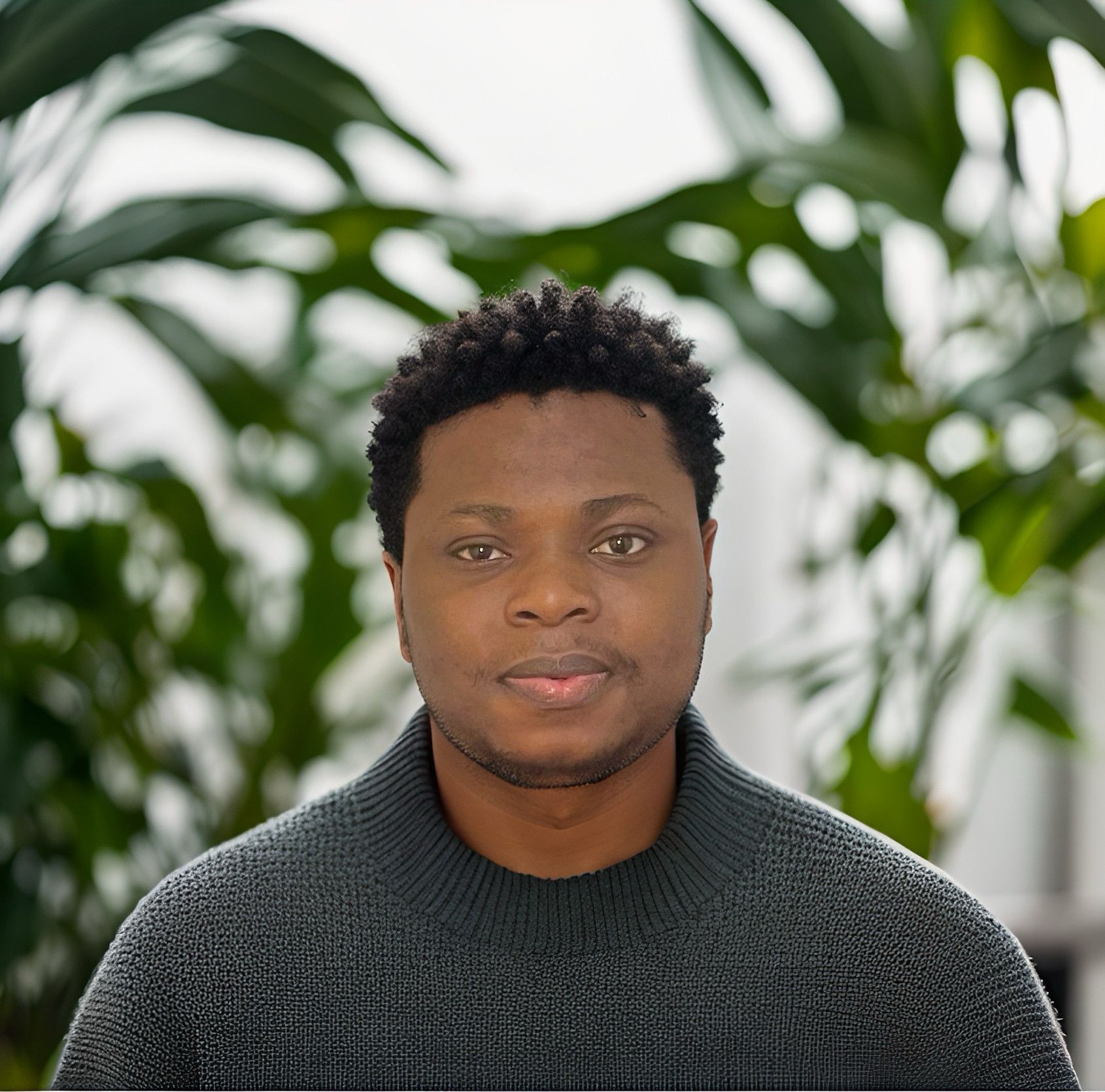
Table of contents
- The Need for CI/CD in Database Schema Changes
- Setting Up a Database CI/CD Pipeline
- 1. Planning and Testing Schema Changes
- 2. Using Migration Tools for Version Control
- 3. Validating Changes with Pre-Deployment Checks
- 4. Deploying Schema Changes Automatically
- 5. Automating Rollbacks for Fast Recovery
- Best Practices for Safe Schema Migrations
- Wrapping Up
Modern applications are highly data-dependent, so managing database schema changes safely is critical. Unlike code changes, database updates can be challenging to roll back, especially in live production environments where downtime isn’t an option. Setting up a CI/CD pipeline for database changes enables safe schema migrations, minimizes downtime, and allows teams to maintain database versioning effectively.
In this article, we’ll explore how to create CI/CD pipelines that manage database changes, ensure safe migrations, handle rollbacks, and use tools like Flyway and Liquibase for automated database versioning.
The Need for CI/CD in Database Schema Changes
A CI/CD pipeline for database changes provides a controlled and automated way to deploy schema updates. This approach brings many benefits:
Consistency: Ensures that all environments (development, staging, production) stay synchronized.
Safety: Reduces the risk of human error by automating processes.
Speed: Allows faster delivery of updates while ensuring stability.
Rollback Capability: Supports fast rollback in case of errors, helping to avoid downtime and data loss.
When managed correctly, a CI/CD pipeline can deploy schema changes just as safely and efficiently as code changes.
Setting Up a Database CI/CD Pipeline
A CI/CD pipeline for database changes typically involves these steps:
Plan and Test the Schema Changes
Use Migration Tools for Version Control
Validate Changes with Pre-Deployment Checks
Deploy Schema Changes Automatically
Automate Rollbacks for Fast Recovery
Let’s dive deeper into each step and see how to configure it.
1. Planning and Testing Schema Changes
The first step in a database CI/CD pipeline is carefully planning and testing schema changes. Changes should be tested on a replica of the production database to ensure they work as expected. Focus on:
Backward Compatibility: Make changes that are backward-compatible whenever possible, so they don’t disrupt older versions of the application.
Decoupling: For major changes, use multiple phases, such as deploying a new column or table, migrating data, and then deprecating old structures.
For example, instead of renaming a column directly, add the new column, migrate data, update the application to use the new column, and then remove the old one.
2. Using Migration Tools for Version Control
Database migration tools like Flyway and Liquibase enable version control for database changes, allowing each schema change to be tracked and applied consistently across environments.
Flyway
Flyway works by managing migration scripts, which are applied sequentially to bring the database to the latest state. It uses a naming convention (like V1__Create_users_table.sql
) to track migrations.
Example Flyway Migration Setup:
-- V1__Create_users_table.sql
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(50),
email VARCHAR(100)
);
In the CI/CD pipeline, Flyway checks for new migrations and applies them automatically. Flyway also offers migrate
(to apply new migrations) and undo
(for rollbacks).
Liquibase
Liquibase provides more flexibility with XML, YAML, JSON, or SQL-based changesets. Each changeset is a versioned migration and can be tagged for rollback or selective execution.
Example Liquibase Changeset:
databaseChangeLog:
- changeSet:
id: 1
author: jdoe
changes:
- createTable:
tableName: users
columns:
- column:
name: id
type: SERIAL
autoIncrement: true
constraints:
primaryKey: true
- column:
name: username
type: VARCHAR(50)
- column:
name: email
type: VARCHAR(100)
Both Flyway and Liquibase can be integrated into CI/CD pipelines to automatically apply changes, manage versioning, and enable rollbacks.
3. Validating Changes with Pre-Deployment Checks
To prevent issues during deployment, validate database changes in a staging environment. Here are essential pre-deployment checks:
Syntax Check: Ensure SQL syntax is correct.
Data Integrity: Run tests to confirm data remains intact after the migration.
Performance Testing: Test queries and indexing to prevent performance degradation.
Dependency Check: Ensure new changes don’t break dependent services or applications.
Automated tests should run as part of the CI pipeline to catch issues early. CI tools like Jenkins or GitHub Actions can trigger these validations before merging schema changes into production.
4. Deploying Schema Changes Automatically
In the CD stage, deploy changes to production automatically but cautiously, following best practices:
Deploy During Off-Peak Hours: If possible, schedule schema changes during times of low traffic.
Limit Transaction Size: For large databases, split migrations into smaller transactions to minimize locking and performance impact.
Monitor in Real-Time: Watch for any unusual performance issues or errors immediately after deployment.
In Jenkins, a CI/CD pipeline might look like this:
pipeline {
agent any
stages {
stage('Validate Schema Changes') {
steps {
sh 'flyway validate'
}
}
stage('Apply Migrations') {
steps {
sh 'flyway migrate'
}
}
stage('Run Post-Deployment Tests') {
steps {
sh 'npm test'
}
}
}
}
This pipeline runs validation, applies migrations, and tests the application after deployment.
5. Automating Rollbacks for Fast Recovery
Rollbacks are vital when a migration causes issues. Tools like Flyway and Liquibase support rollback scripts:
Flyway Undo Migrations: Flyway lets you create undo migrations, which are applied in reverse when a rollback is triggered.
Liquibase Rollbacks: Liquibase has rollback tags, enabling you to undo changesets selectively.
Example of a rollback in Flyway:
-- V1__Create_users_table.sql
CREATE TABLE users ( ... );
-- V1__Drop_users_table.sql (undo)
DROP TABLE users;
Example of a rollback in Liquibase:
databaseChangeLog:
- changeSet:
id: 1
author: jdoe
changes:
- createTable:
tableName: users
rollback:
- dropTable:
tableName: users
These rollbacks should be automated within the CI/CD pipeline, allowing you to revert changes seamlessly if needed.
Best Practices for Safe Schema Migrations
Use Idempotent Scripts: Ensure scripts can be rerun without issues. For instance, use
IF EXISTS
orIF NOT EXISTS
clauses to avoid errors.Backup Critical Data: Perform regular database backups, especially before significant migrations.
Ensure Version Synchronization: Use a migration tool to track the database schema version to prevent mismatches across environments.
Monitor and Log: Use monitoring tools to watch for errors, latency, or performance drops immediately after schema changes.
Wrapping Up
Implementing a CI/CD pipeline for database changes enhances the consistency, safety, and speed of schema updates. By planning changes carefully, using migration tools like Flyway and Liquibase, and following best practices for automation and rollback, teams can confidently deploy database updates with minimal risk and downtime. This approach empowers development teams to innovate and scale, keeping their databases stable and secure as their applications evolve.
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
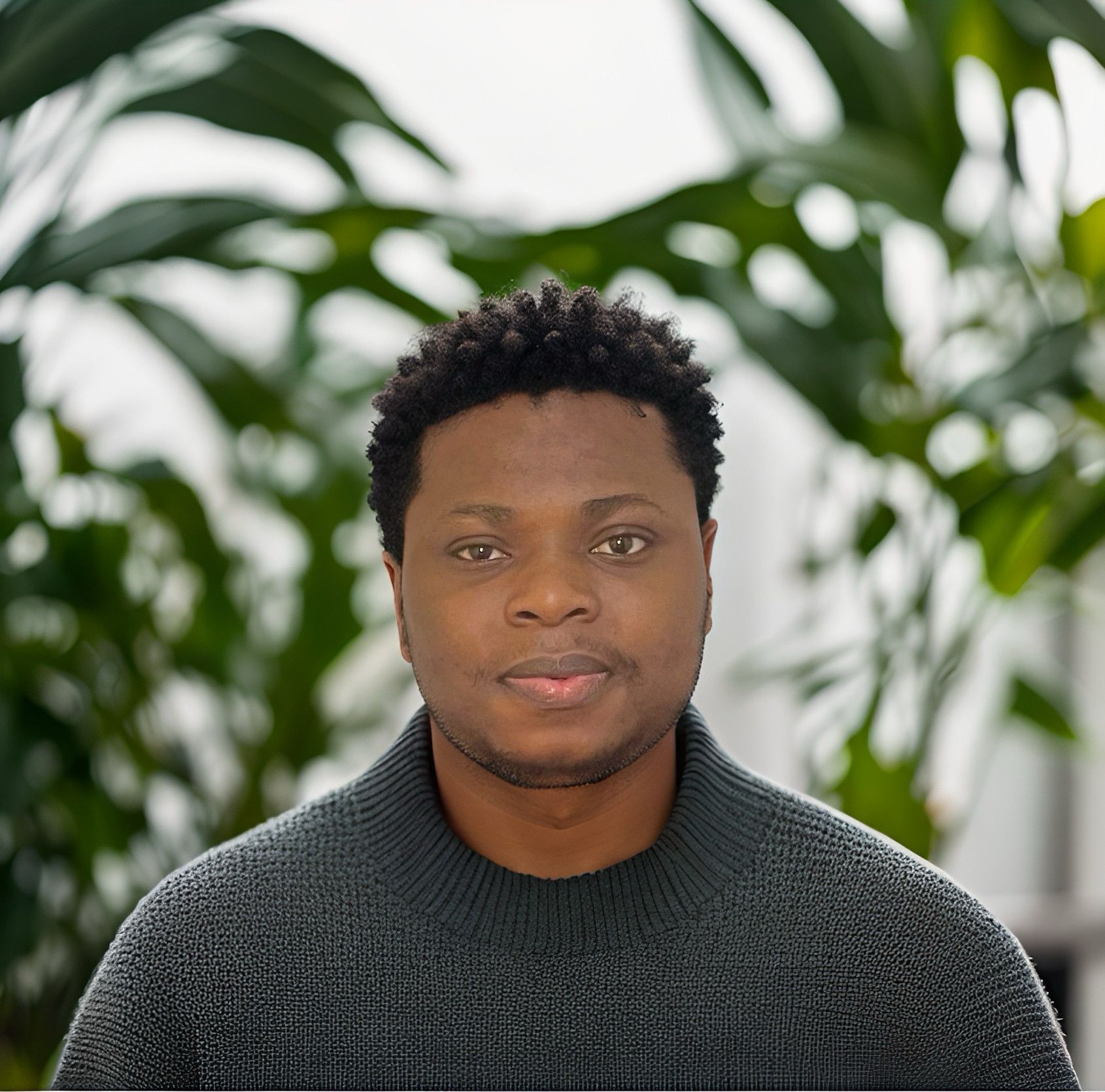