Boost Your Problem-Solving Skills with Binary Search Techniques

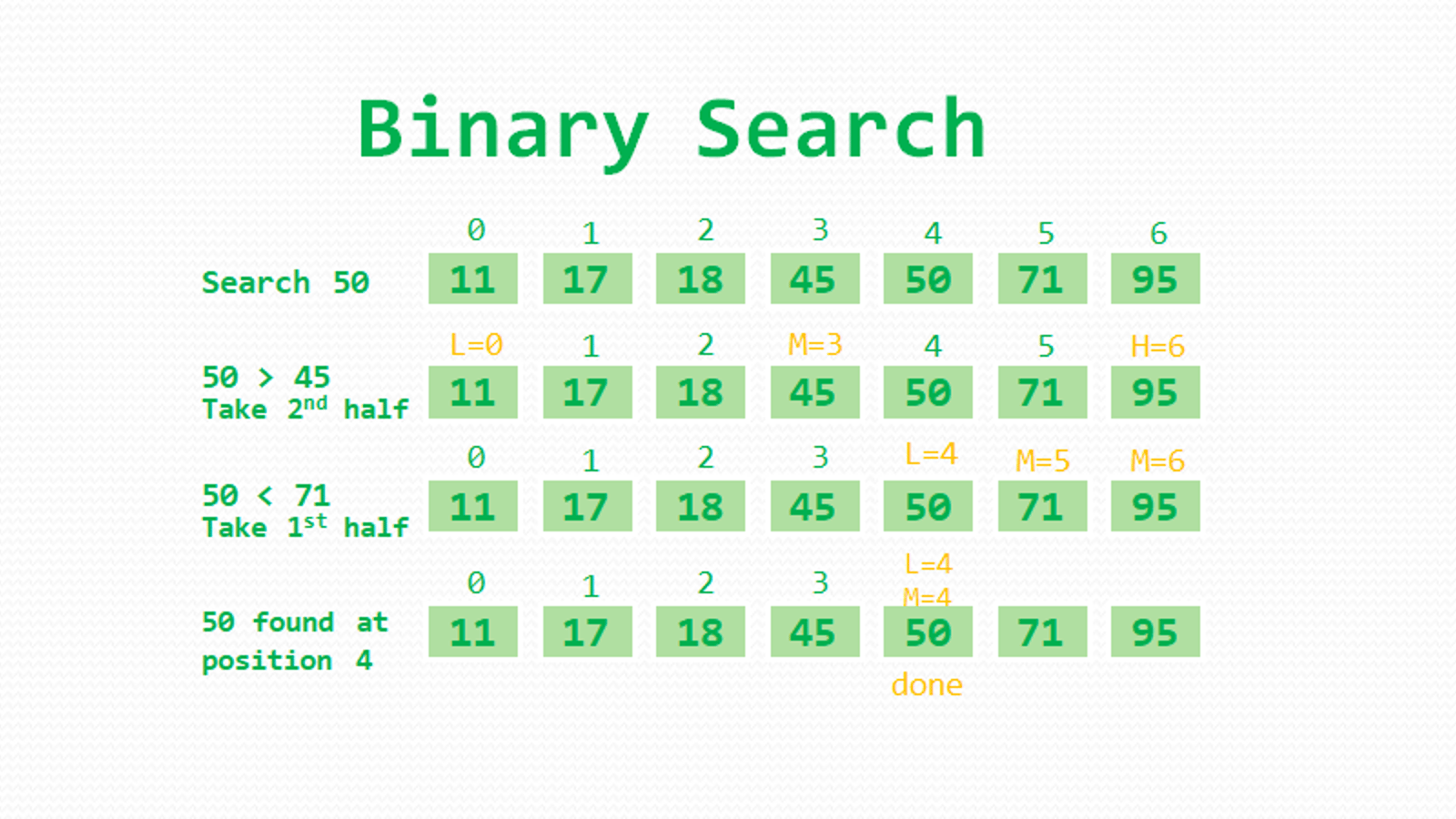
Binary Search is one of the most powerful and efficient techniques for performing operations on sorted data. It drastically reduces the time complexity of many search problems, allowing them to run in O(log(n)) time instead of O(n) or even O(n²). This efficiency makes it invaluable for optimizing search functions in a wide range of applications.
Let’s dive into how Binary Search can improve our approach to searching for an element in a sorted array.
The Naive Search Method
Let's first try a straightforward approach to searching for a target element in a sorted array.
nums: list[int] = [11,17,18,45,50,71,95]
target: int = 16
def find_the_target(nums: list[int], target: int) -> int:
# Returns the index of the target if found
for i in range(len(nums)):
if nums[i] == target:
return i
return -1
In this naive approach, we loop through each element in the array to find the target. This method has a time complexity of O(n), which is manageable for small arrays but becomes inefficient as the array size increases.
Improving with Binary Search
Now let’s use Binary Search to optimize this function. Binary Search is possible here because the array is sorted, allowing us to narrow down our search range with each step, rather than checking each element sequentially.
nums: list[int] = [11,17,18,45,50,71,95]
target: int = 16
def find_the_target(nums: list[int], target: int) -> int:
i, j = 0, len(nums) - 1
while i <= j:
mid = (i + j) // 2
if nums[mid] == target:
return mid
elif nums[mid] > target:
j = mid - 1
else:
i = mid + 1
return -1
Why Is Binary Search More Efficient?
At first glance, Binary Search might look more complex than our naive approach. However, it’s significantly more efficient, especially for large datasets.
Consider this scenario:
Suppose we have a list of 1,000 elements and we want to find a target near the end of the list, say 999.
Using the naive approach, we’d need to make 999 comparisons to find the target.
With Binary Search, however, we only need about 10 comparisons to locate the element or determine that it’s missing from the list.
Binary Search works by repeatedly halving the search range, meaning that even with a massive dataset, the number of comparisons grows slowly (logarithmically) compared to the list size.
What if the Target is Not in the Array?
If the target isn’t present in the array, the naive approach would require O(n) time to verify this. With Binary Search, however, it only takes O(log(n)) steps to confirm the absence of the target. This improvement becomes especially important when working with very large data sets, as it saves significant processing time and resources.
Key Takeaways
Binary Search operates with O(log(n)) time complexity, making it highly efficient for large, sorted datasets.
It significantly reduces the number of operations needed compared to a linear search.
Binary Search requires a sorted array to function correctly—if the data is unsorted, a sorting step is required before applying Binary Search.
Binary Search is an essential tool for problem-solving in computer science, providing a simple yet efficient solution to many search-related tasks. Embracing Binary Search can elevate your programming skills and open up opportunities to solve complex problems with ease.
Subscribe to my newsletter
Read articles from Romjan D. Hossain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Romjan D. Hossain
Romjan D. Hossain
Exploring new/old technologies every day. Its just amazing.....!