Aptos Tools; a Look at ANS and Aptos Explorer
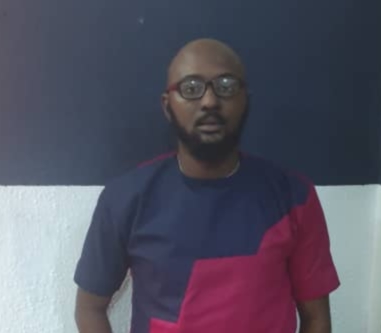
Introduction
The Aptos tooling ecosystem makes the journey across the Aptos blockchain easier and more accessible. The Aptos Name Service (ANS) and the Aptos Explorer are tools designed to simplify users' interactions with the blockchain.
Aptos Name Service (ANS) represents long blockchain addresses into easy-to-remember names. Instead of dealing with a lengthy public key, users can now use simple ".apt" names to interact with smart contracts and identify accounts.
The Aptos Explorer, on the other hand, is a tool for diving deeper into the blockchain. It presents a clean, user-friendly interface that allows you to track events happening on Aptos, from transactions and blocks to accounts and contracts. Whether you're checking the status of a transaction or exploring blockchain data, the Aptos Explorer provides transparency and ease of use.
In this post, we’ll explore how to integrate the ANS SDK into your dapp and effectively use the Aptos Explorer to enhance your understanding and interaction with the Aptos blockchain.
Aptos Naming System
Aptos blockchain addresses are long strings of letters and numbers that represent a blockchain account. ANS allows users to replace these long addresses with easy-to-read names by registering the address for some time, similar to how the Ethereum Naming Service (ENS) works.
To get started, a user checks if the domain name they want to purchase is available. They make payments using the Aptos native coin, APT, to register the name if it is available. The registered .apt domain name points to the user's address and can be used instead of the long address. A user can also create a sub-domain from their primary domain. The sub-domain points to an address and resolves to that address. ANS domains and sub-domains do expire, and their owners should renew them to avoid losing access. The image illustration in Figure 1 below explains this process.
Figure 1: An Illustration of ANS domain registration
How to Integrate ANS into a Dapp
The ANS SDK is part of the Aptos SDK. To get started install the Typescript SDK @aptos-labs/ts-sdk
. We will use the console to demonstrate some of the ANS SDK
functionalities.
Create a new npm project and install Aptos Typescript SDK
by running
Then create a index.js
file and paste the code below into the file;
npm i @aptos-labs/ts-sdk
import { Aptos, AptosConfig, Network, Account, Ed25519PrivateKey, Hex } from "@aptos-labs/ts-sdk";
import fs from 'fs';
import { join } from 'path';
const config = new AptosConfig({ network: Network.TESTNET });
const aptosLocal = new Aptos(config);
let wizardDomain = "wizard.apt";
let magicianSubDomain = "magician.wizard.apt"
const filePath = join(process.cwd(), 'account.json');
function bytesToHex(bytesList) {
return bytesList.map(byte => byte.toString(16).padStart(2, '0')).join('');
}
const writeAccount = (filePath, data) => {
const jsonData = JSON.stringify(data, null, 4);
try {
fs.writeFileSync(filePath, jsonData, 'utf8',)
} catch (error) {
console.log("error writing file ", error)
}
}
const readAccount = (filePath) => {
if (fs.existsSync(filePath)) {
try {
const jsonData = fs.readFileSync(filePath, "utf8");
const data = JSON.parse(jsonData);
return data;
} catch (error) {
return null
}
} else{
return null
}
}
async function createBlockChainAccount() {
const accountData = readAccount(filePath);
if (!accountData){
let adah = Account.generate();
let jason = Account.generate();
let timesCalled = 0;
while (timesCalled < 2 ){
//fund the account twice
await aptosLocal.fundAccount({
accountAddress: adah.accountAddress.toString(),
amount: 10_000_000_000
});
await aptosLocal.fundAccount({
accountAddress: jason.accountAddress.toString(),
amount: 10_000_000_000,
});
timesCalled++
}
const data = {
adahAccount: {
address: adah.accountAddress.toString(),
privateKey: adah.privateKey
},
jasonAccount: {
address: adah.accountAddress.toString(),
privateKey: adah.privateKey
}
}
writeAccount(filePath, data)
return { adah, jason }
} else {
const { adahAccount, jasonAccount } = accountData;
const { signingKey:adahSigningKey } = adahAccount.privateKey;
const { signingKey:jasonSigningKey } = adahAccount.privateKey;
const adahhexData = Object.values(adahSigningKey.data);
const jasonHexData = Object.values(jasonSigningKey.data);
const adahPrivateKey = new Ed25519PrivateKey(bytesToHex(adahhexData))
const jasonPrivateKey = new Ed25519PrivateKey(bytesToHex(jasonHexData))
const adah = Account.fromPrivateKey({privateKey: adahPrivateKey, address: adahAccount.address});
const jason = Account.fromPrivateKey({privateKey: jasonPrivateKey, address: jasonAccount.address});
return {adah, jason};
}
}
async function createWizardDomain(adah) {
let transaction = await aptosLocal.registerName({
sender: adah,
name: wizardDomain,
expiration: { policy: "domain" },
});
let pendingTxn = await aptosLocal.signAndSubmitTransaction({
signer: adah,
transaction,
});
await aptosLocal.waitForTransaction({ transactionHash: pendingTxn.hash });
}
async function createMagicianSubDomain(adah,jason) {
const transaction = await aptosLocal.registerName({
sender: adah,
name: magicianSubDomain,
expiration: {
policy: "subdomain:independent", // subdomain:follow-domain
expirationDate: Date.now() + 30 * 24 * 60 * 60 * 1000,
},
toAddress: jason.accountAddress.toString()
});
let pendingTxn = await aptosLocal.signAndSubmitTransaction({
signer: adah,
transaction,
});
await aptosLocal.waitForTransaction({ transactionHash: pendingTxn.hash });
}
async function main() {
//create blockchain account;
try {
console.log("creating and finding adah and jason account");
const {adah, jason} = await createBlockChainAccount();
console.log("finish funding adah and jason account");
//check for the availiability of a name
const checkDomain = await aptosLocal.ans.getName({ name: wizardDomain })
console.log("Domain", checkDomain);
if (!checkDomain) {
//register the domain name
await createWizardDomain(adah);
const wizardOwner = await aptosLocal.getOwnerAddress({ name: wizardDomain });
console.log("wizard domain owner: ", wizardOwner.toString());
console.log("Adah address: ", adah.accountAddress.toString());
console.log("Wizard domain registered");
await createMagicianSubDomain(adah, jason);
const magicianOwner = await aptosLocal.getOwnerAddress({ name: magicianSubDomain });
console.log("magician sub-domain owner: ", magicianOwner.toString());
console.log("Jason address: ", jason.accountAddress.toString());
console.log("Magician Sub domain domain registered");
} else {
console.log("domain already exist. chose another name");
}
} catch (error) {
console.log("error ", error)
}
}
main();
The code above demonstrates how to create a domain and a sub-domain using the SDK. At the top of the file, we initialize the Aptos
object connecting to the Aptos
Testnet. The ANS contract is not deployed to devnet
, which is why I am using the testnet network.
The createBlockChainAccount
creates and funds two test accounts from a faucet. You can’t get more than 1 APT
at an instance when funding an account and you need 1 APT
to register an ANS domain which leaves you short on the payment of the transaction gas fee. The createBlockChainAccount
function writes the created account into a json file and calls the funding API
twice to get enough APT
to execute the transactions.
The function createWizardDomain
accepts an account as a parameter. The domain name is registered using the registerName
function of the Aptos
object created at the top of the file. The expiration policy is set to domain; this expires after a year. When registering the domain name, you could register it with or without the .apt
suffix and If the domain name exists already an error will be thrown by the SDK.
let transaction = await aptosLocal.registerName({
sender: adah,
name: wizardDomain,
expiration: { policy: "domain" },
});
The createMagicianSubDomain
function creates a sub-domain from an already created domain. The adah
account creates the sub-domain and assigns it to the jason
account. The chosen expiration policy is subdomain:independent
, meaning the sub-domain's expiration date is separate from the primary domain's expiration date. Another policy, subdomain:follow-domain
, can be used for sub-domains, meaning the sub-domain expires when the primary domain expires.
const transaction = await aptosLocal.registerName({
sender: adah,
name: magicianSubDomain,
expiration: {
policy: "subdomain:independent", // subdomain:follow-domain
expirationDate: Date.now() + 30 * 24 * 60 * 60 * 1000,
},
toAddress: jason.accountAddress.toString()
});
The SDK also provides different well-named functions used to operate ANS
in our dapp. For example functions like, getName
, getAccountNames
, getAccountDomains
, getDomainSubdomains
etc.
Test the code above by running the file using;
node index.js
and you will get a result similar to the figure below
Figure 2
You can use this example as a starting point to explore other functionalities listed in the SDK.
Aptos Explorer
Aptos
Explorer is a graphical interface tool used to analyse the Aptos
blockchain. The explorer's core purpose is to provide real-time access to data from the blockchain which includes transaction data, smart contracts code, resources, coins and tokens, blocks etc.
You can use the Explorer’s search tab to find information about an account address or a transaction hash. Entering an account address into the search bar yields results as shown in Figure 3
below.
Figure 3
In Figure 3
, there is a “Transaction” tab containing information about all the transactions made by an address. Clicking on each transaction opens up a detailed view of the transaction.
Figure 4
We can see a broad overview of a transaction in the transaction detailed tab. The “Overview” tab on the transaction detail page as shown in Figure 4
, gives information about the address of the transaction sender, the smart contract, the function called in the smart contract, the gas fee and other information relating to a transaction.
Also on the transaction details page, there’s information about the balance change between the addresses in the transaction. For example, sending a transaction to register a domain name using ANS
will cost the sender the amount for registering the domain name and the gas used. So the balance changes; the sender account gets subtracted gas fee + domain registration amount
and the smart contract gets the registration fee as shown in Figure 5
.
Figure 5
Still, on the transaction detail page, we can analyze the events emitted by the transaction and the transaction payload. This helps to give insight into the workings of a smart contract and understanding what is going on at each function call of the contract. Lastly, on the transaction details page, we can also view the state changes that a transaction caused in the blockchain via the “Payload” tab.
Taking a closer look at Figure 3 above, after the “Transaction” tab you will see the “Coins”, “Tokens”, “Resources”, “Modules” and “Info” tabs. The “Coins” and “Tokens” show all the coins and tokens owned by the address. The “Resources” tab lists all the move resources found at that address and the “Modules” tab shows the smart contract deployed at the address. Finally, the Info tab shows the sequence number of the account address, which is the number of transactions that have been sent from that address and the authentication key of the address.
As we can see the explorer provides a comprehensive overview of a user account. Users can view account balances, recent transactions, and owned smart contracts. Developers and users alike can quickly inspect an account’s activity and understand its interaction with the broader network. This is particularly useful for keeping track of dApp accounts or assessing the activities of large token holdings accounts ( whales ).
The Explorer could also provide information about validators. You can see a detailed analysis of each network validator, its location, the overall health of the network and detailed information about individual blocks within the blockchain. This includes the number of transactions per block, the time at which a block was confirmed, and the block height.
The Aptos
Explorer provides a rich vein of information about the Aptos blockchain and it is a critical infrastructure that supports the transparency, accountability, and usability of the Aptos
network.
Concluding Words
Tools are meant to simplify our tasks, and in this post, we explored two designed to make adopting the Aptos ecosystem seamless. Aptos Name Service (ANS) personalizes blockchain addresses, allowing users to register their addresses with custom names, adding a personal touch and style to blockchain transactions.
Meanwhile, the Aptos Explorer is your go-to tool for diving into the finer details of the Aptos blockchain. Whether you’re an experienced user or just getting started, its intuitive, graphical interface makes navigating blockchain data easy and accessible for everyone.
In a rapidly evolving ecosystem like Aptos, having tools like ANS and Aptos Explorer simplifies usability and fosters broader adoption by breaking down the complexities of blockchain technology making it easier for anyone to join and thrive in the Aptos Ecosystem.
Thank you for reading.
Subscribe to my newsletter
Read articles from Osikhena Oshomah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
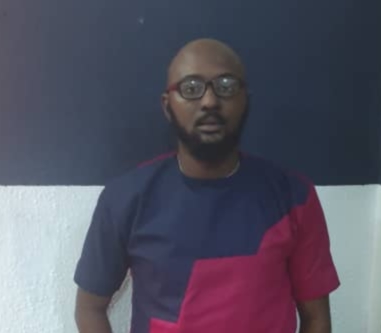
Osikhena Oshomah
Osikhena Oshomah
Javascript developer working hard to master the craft.