Deep Dive into Functional Programming in JavaScript: Higher Order Functions and Immutability

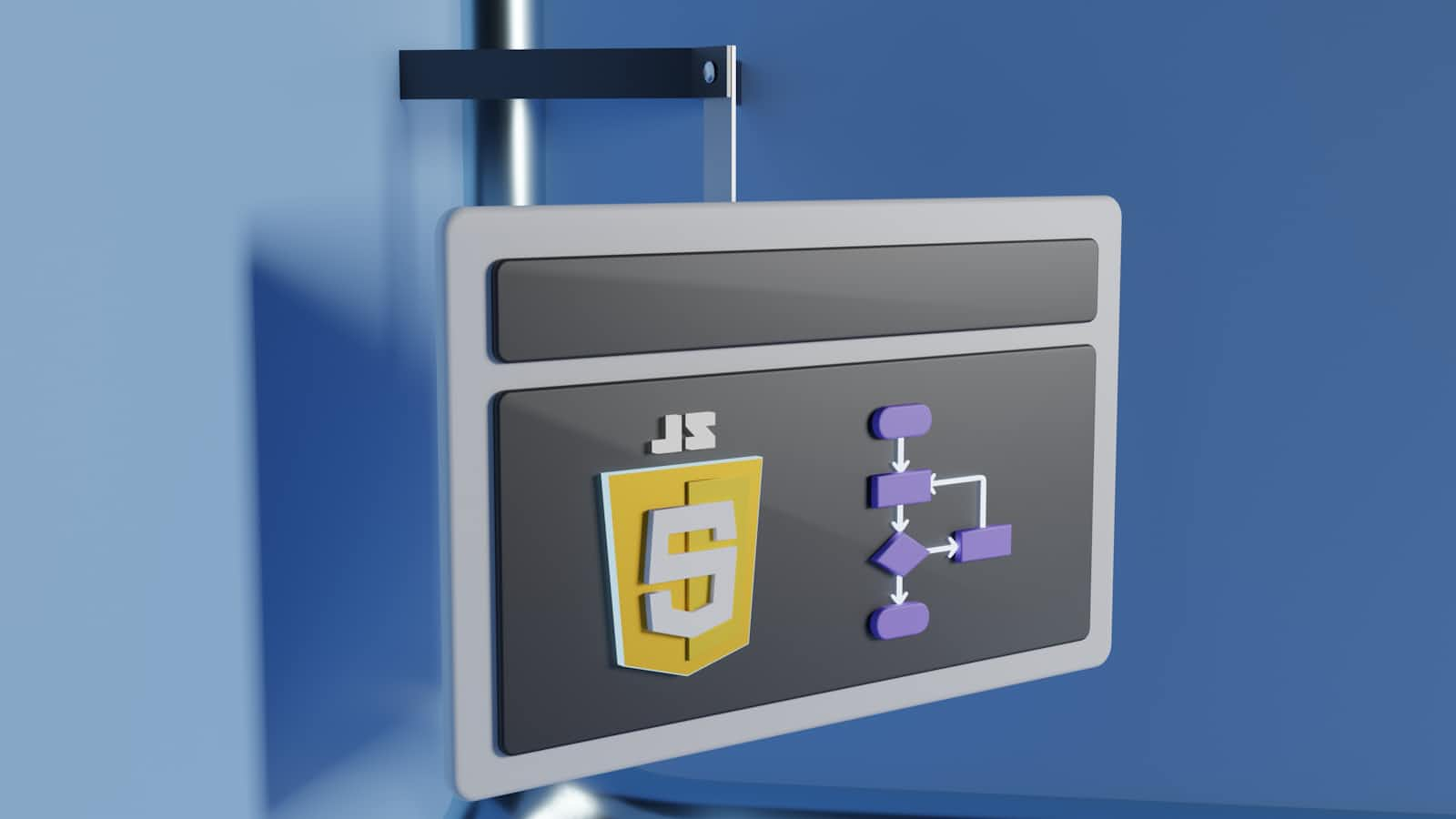
Functional programming has gained significant traction in the JavaScript world. Well Known for improving readability, reducing bugs, and making code more predictable, functional programming centers around pure functions, immutability, and avoiding shared state. This article will focus on two fundamental aspects of functional programming in JavaScript: higher-order functions and immutability.
Let's explore how these concepts can transform the way we approach coding in JavaScript ;
At its core, functional programming (FP) is a declarative paradigm—expressing the logic of computation without describing its control flow. In JavaScript, FP emphasizes writing functions that are pure (no side effects) and focusing on immutability to prevent unintended changes in program state.
Higher-Order Functions: The Power of Functions That Manipulate Functions
A higher-order function (HOF) is any function that either takes one or more functions as arguments or returns a function as its result. Higher-order functions provide a powerful way to abstract common operations, making your code concise and expressive.
Why Higher-Order Functions?
Higher-order functions are useful for handling tasks such as iteration, data transformation, and asynchronous operations. By abstracting these tasks, higher-order functions allow you to define the what rather than the how of your code, improving readability and maintainability.
Examples of Higher-Order Functions in JavaScript
JavaScript has built-in HOFs like map
, filter
, and reduce
. Let’s examine each:
Using map
for Data Transformation
The map
function iterates over an array, applies a transformation function to each element, and returns a new array.
// Scenario: Converting an array of prices from USD to EUR
const pricesUSD = [29.76, 41.85, 46.5];
const conversionRate = 0.89;
const pricesEUR = pricesUSD.map(price => (price * conversionRate).toFixed(2));
console.log(pricesEUR); // [26.49,37.25,41.38]
Here, map
allows us to apply the conversion function to each price without mutating the original pricesUSD
array, demonstrating both immutability and transformation.
Filtering Data with filter
The filter
function lets us create a new array based on specific criteria, filtering out elements that don't match.
// Scenario: Filtering out even numbers
const numbers = [1, 2, 3, 4, 5, 6];
const oddNumbers = numbers.filter(num => num % 2 !== 0);
console.log(oddNumbers); // [1, 3, 5]
reduce
offers powerful ways to accumulate values, and with a bit of creativity, it can handle complex transformations as well.
Immutability: Ensuring Predictable Code
In functional programming, immutability is the concept of not modifying existing data structures. Instead, you create new structures with updated values. Immutability reduces bugs by making it clear when data has changed and what caused the change.
Why Immutability?
Mutable state can lead to unexpected behaviors, especially in larger applications. By enforcing immutability, functional programming allows you to maintain a predictable flow of data, which simplifies debugging and testing.
How to Achieve Immutability in JavaScript
JavaScript has several ways to enforce immutability:
Object Spread and Array Spread Syntax
Array and Object Methods (map, filter, reduce)
Libraries: Popular libraries like Immutable.js or Immer offer additional tools for immutability.
Immutability in Action: An Example
Suppose we have a list of users and want to update one user’s age without modifying the original array.
// Original data
const users = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 }
];
// Updating Bob's age immutably
const updatedUsers = users.map(user =>
user.name === 'Bob' ? { ...user, age: 31 } : user
);
console.log(updatedUsers);
// [
// { name: 'Alice', age: 25 },
// { name: 'Bob', age: 31 },
// { name: 'Charlie', age: 35 }
// ]
Here, we used the map
function to create a new array with Bob’s age updated. This approach keeps the original users
array intact, adhering to the principle of immutability.
Avoiding Mutation in Nested Objects
For complex objects, it’s essential to handle nested data carefully. Using libraries like Immer can simplify this by letting you write mutable code that works immutably under the hood.
// Using Immer for deep immutability
import produce from 'immer';
const state = {
user: {
name: 'Alice',
age: 25,
address: { city: 'New York', zip: '10001' }
}
};
const updatedState = produce(state, draft => {
draft.user.address.city = 'Los Angeles';
});
console.log(state.user.address.city); // "New York"
console.log(updatedState.user.address.city); // "Los Angeles"
Immer allows us to “mutate” draft
, producing a new, immutable state without changing the original.
Combining Higher-Order Functions with Immutability
Imagine a scenario where you have a list of transactions and need to calculate the total amount spent on a specific category.
const transactions = [
{ amount: 50, category: 'Groceries' },
{ amount: 20, category: 'Transport' },
{ amount: 100, category: 'Groceries' },
{ amount: 60, category: 'Entertainment' }
];
const totalGroceries = transactions
.filter(txn => txn.category === 'Groceries')
.reduce((sum, txn) => sum + txn.amount, 0);
console.log(totalGroceries); // 150
In this example, filter
isolates the grocery transactions, and reduce
sums their amounts. This combination of higher-order functions with immutable methods achieves our goal efficiently and keeps our original data untouched.
Best Practices and Conclusion
Functional programming with JavaScript’s higher-order functions and immutability fosters a clean, predictable codebase. Here are some final best practices:
Use Higher-Order Functions Wisely: They’re powerful but can lead to confusing code if overused. Use them where they simplify the logic.
Favor Immutability: While not always feasible, immutability can greatly enhance data predictability, especially in asynchronous and state-heavy applications.
Leverage Libraries for Complex Immutability Needs: Libraries like Immer provide robust solutions when working with deeply nested data.
Incorporating functional programming principles takes some adaptation but offers significant long-term benefits in maintainability, readability, and reduced bugs. As you continue your journey with JavaScript, try incorporating higher-order functions and immutability into your code and experience the difference they make!
Happy coding!
Subscribe to my newsletter
Read articles from Adedeji Agunbiade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
